Multi-Language Django Project in 12 Simple Steps(2024).
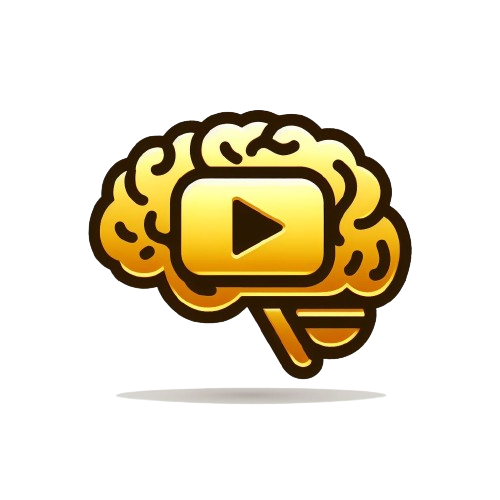
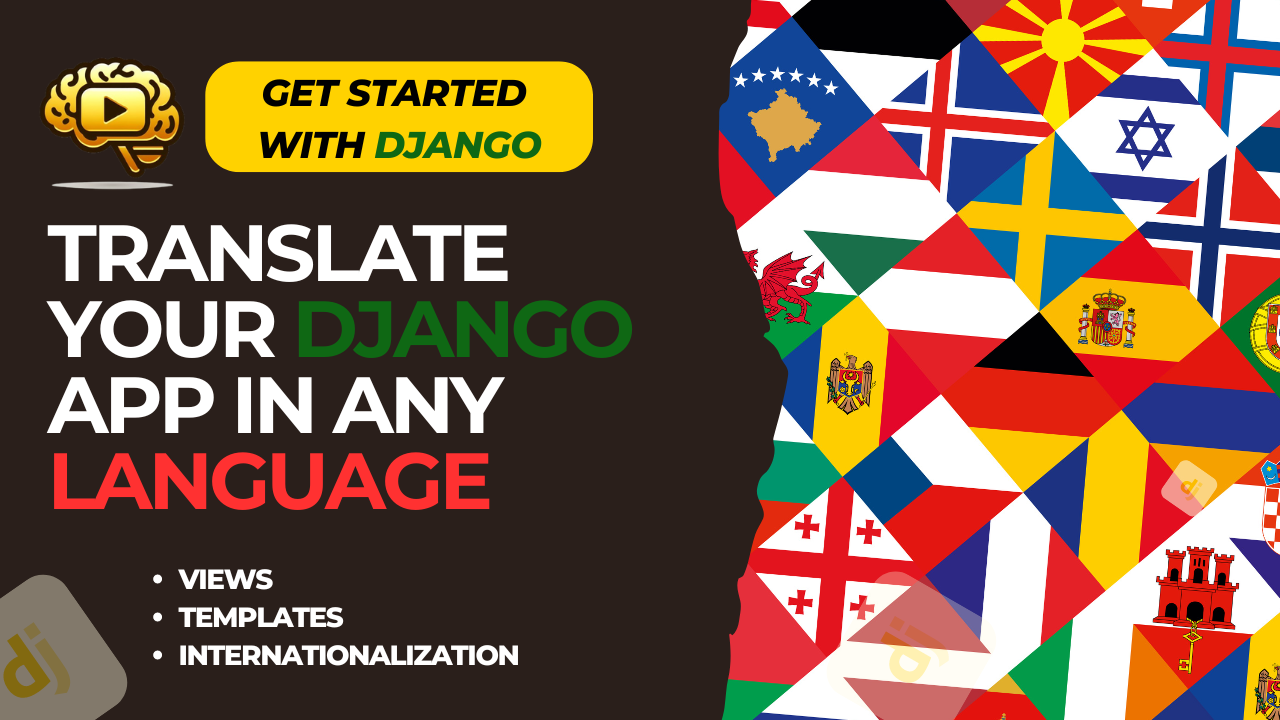
A Comprehensive Guide to Internationalization in Django
In today's interconnected world, reaching a global audience is crucial for the success of any web application. Internationalization (i18n) in Django allows developers to create applications that can be adapted to various languages and regions without significant changes to the codebase. In this tutorial, we'll walk through the steps to internationalize a Django project, covering everything from setting up a simple project to generating translation files and enabling language selection for users.
Table of Contents:
What is Internationalization?
Creating a Simple Django Project
Understanding
gettext
,gettext_lazy
, and{% trans "" %}
Loading Internationalization in Your HTML Templates
Creating a
locale
DirectoryAdding
LOCALE_PATHS
to the SettingsAdding Middleware to the Settings
Activating Internationalization
Adding Available Languages
Adding Internationalization to URLs
Generating and Compiling Translation Files
Enabling Language Selection for Users
1. What is Internationalization?
Internationalization, often abbreviated as i18n, is the process of designing and developing software applications that can be adapted to different languages and regions. It involves making your application locale-aware, enabling it to display content in multiple languages based on user preferences.
2. Creating a Simple Django Project
Let's start by creating a simple Django project to demonstrate internationalization:
django-admin startproject myproject
cd myproject
3. Understanding gettext
, gettext_lazy
, and {% trans "" %}
In Django, gettext
and gettext_lazy
are Python libraries used for internationalization, while {% trans "" %}
is a template tag used to mark strings for translation in templates.
4. Loading Internationalization in Your HTML Templates
To enable internationalization in your Django templates, load the i18n
template tags at the top of your HTML file:
{% load i18n %}
Also this is the html we are going to be using
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Internationalization Example</title>
<!-- Bootstrap CSS -->
<link href="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css" rel="stylesheet">
<style>
/* Custom CSS */
body {
background-color: #ffffff; /* White */
color: #6c757d; /* Light Grey */
}
.navbar {
background-color: #ffd700; /* Golden Yellow */
}
.footer {
background-color: #ffd700; /* Golden Yellow */
position: fixed;
bottom: 0;
width: 100%;
}
</style>
</head>
<body>
<!-- Navbar -->
<nav class="navbar navbar-expand-lg navbar-light">
<div class="container">
<a class="navbar-brand" href="#">Internationalization</a>
<button class="navbar-toggler rounded-circle" type="button" data-toggle="collapse" data-target="#navbarSupportedContent" aria-controls="navbarSupportedContent" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarSupportedContent">
<ul class="navbar-nav ml-auto">
<li class="nav-item">
<a class="nav-link rounded-pill" href="#">Home</a>
</li>
<li class="nav-item">
<a class="nav-link rounded-pill" href="#">About</a>
</li>
<li class="nav-item">
<a class="nav-link rounded-pill" href="#">Contact</a>
</li>
<li class="nav-item">
<a class="nav-link rounded-pill" href="#">Language</a>
</li>
</ul>
</div>
</div>
</nav>
<!-- Main Content -->
<div class="container mt-5">
<div class="row">
<div class="col-lg-4 mb-4">
<div class="bg-light rounded p-3">
<h3 class="mb-3">English</h3>
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nullam vitae tristique elit.</p>
</div>
</div>
<div class="col-lg-4 mb-4">
<div class="bg-light rounded p-3">
<h3 class="mb-3">French</h3>
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nullam vitae tristique elit.</p>
</div>
</div>
<div class="col-lg-4 mb-4">
<div class="bg-light rounded p-3">
<h3 class="mb-3">Spanish</h3>
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nullam vitae tristique elit.</p>
</div>
</div>
</div>
</div>
<!-- Footer -->
<footer class="footer text-center py-3">
<div class="container">
<span>© 2024 Internationalization Example</span>
</div>
</footer>
<!-- Bootstrap JS -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js"></script>
</body>
</html>
5. Creating a locale
Directory
Create a directory named locale
at the level of your templates. This directory will hold the translation files for different languages.
6. Adding LOCALE_PATHS
to the Settings
In your Django project's settings file, add the following line to specify the location of the locale
directory:
LOCALE_PATHS = (
os.path.join(BASE_DIR, 'locale'),
)
7. Adding Middleware to Settings
Django provides middleware that helps manage language settings. Add the following middleware to your MIDDLEWARE
setting:
MIDDLEWARE = [
...
'django.middleware.locale.LocaleMiddleware',
...
]
8. Activating Internationalization
In your Django project's settings file, activate internationalization by setting USE_I18N
to True
:
USE_I18N = True
9. Adding Available Languages
Define the LANGUAGES
variable in your settings file to specify the list of available languages for translation:
from django.utils.translation import gettext_lazy as _
LANGUAGES = [
('en', _('English')),
('fr', _('French')),
# Add more languages as needed
]
10. Adding Internationalization to URLs
Include internationalization in your URL patterns by adding the following to your urls.py
:
from django.conf.urls.i18n import i18n_patterns
urlpatterns += i18n_patterns(
# Add your URL patterns here
)
11. Generating and Compiling Translation Files
To generate translation files for your project, use the following command:
django-admin makemessages -l <language_code>
After making translations, compile the translation files using:
django-admin compilemessages
12. Enabling Language Selection for Users
Add language selection options to your HTML templates to allow users to choose their preferred language. Use the following code snippet:
<select name="language" id="language">
{% get_current_language as current_language %}
{% get_available_languages as available_languages %}
{% get_language_info_list for available_languages as languages %}
{% for language in languages %}
<option value="{{ language.code }}" {% if language.code == current_language %}selected{% endif %}>
{{ language.name_local }} ({{ language.code }})
</option>
{% endfor %}
</select>
Conclusion
By following these steps, you can effectively internationalize your Django application, making it accessible to users worldwide. Internationalization not only enhances user experience but also opens up new opportunities for reaching diverse audiences. Embrace the power of internationalization to create inclusive and globally accessible web applications.
Subscribe to my newsletter
Read articles from Ndongmo christian directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
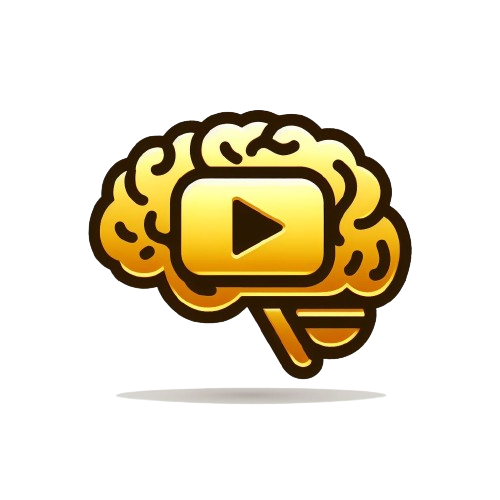
Ndongmo christian
Ndongmo christian
I am Christian,a Python developer, Content Creator from Hashnode. A Computer Engineering Student who loves Coding, Sharing of knowledge, Interacting with poeple. Also play Basketball, Music, Sweets etc