Topic: 13 Understanding Data Storage in Flutter

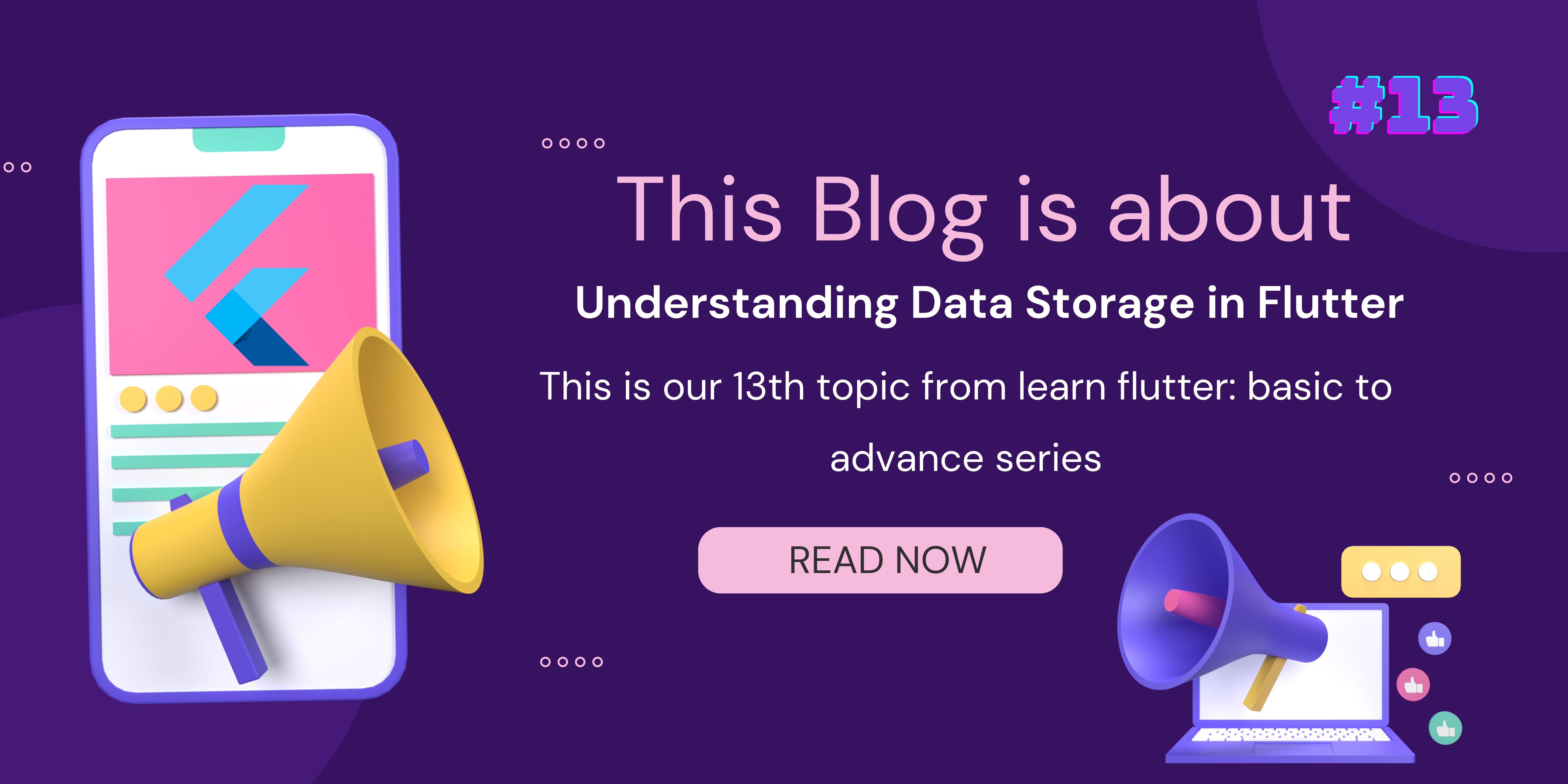
Hello devs, So in today's article, we talk about different types of data storage in Flutter. Flutter offers several options for storing data locally on the device. Each option has its strengths and use cases, so it's essential to choose the right one based on your specific requirements.
Alright devs, First we talk about small-level data storage.
Shared Preferences
Shared Preferences is a simple key-value storage solution provided by the Flutter framework. It allows you to store small amounts of data persistently on the device. Shared Preferences is ideal for storing simple data types like strings, integers, booleans, and floats. It's lightweight, easy to use, and accessible across app restarts.
How Shared Preferences Work in Flutter
Shared Preferences works asynchronously, meaning that data is stored and retrieved without blocking the main UI thread. This ensures that your app remains responsive even when accessing stored data.
Under the hood, Shared Preferences uses platform-specific implementations to store data. On Android, it uses SharedPreferences, while on iOS, it uses NSUserDefaults. This allows Shared Preferences to provide a consistent API for accessing stored data across both platforms.
Using Shared Preferences in Flutter
Using Shared Preferences in Flutter is straightforward. Here's a step-by-step guide on how to use Shared Preferences to store and retrieve data in your application:
- Add Dependency: First, you need to add the shared_preferences package to your pubspec.yaml file.
dependencies:
flutter:
sdk: flutter
shared_preferences: ^2.0.7
- Import Package: Import the shared_preferences package in your Dart file where you want to use Shared Preferences.
import 'package:shared_preferences/shared_preferences.dart';
- Saving Data: To save data to Shared Preferences, you need to obtain an instance of SharedPreferences and then use it to store data.
void saveData() async {
SharedPreferences prefs = await SharedPreferences.getInstance();
prefs.setString('username', 'john_doe');
}
- Reading Data: To retrieve data from Shared Preferences, you again need to obtain an instance of SharedPreferences and then use it to retrieve the stored data.
void readData() async {
SharedPreferences prefs = await SharedPreferences.getInstance();
String? username = prefs.getString('username');
print('Username: $username');
}
Benefits of Using Shared Preferences
Shared Preferences offers several benefits for data storage in Flutter applications:
Simplicity: Shared Preferences provides a simple and straightforward API for storing and retrieving data.
Asynchronous: Shared Preferences works asynchronously, ensuring that data access operations do not block the main UI thread.
Persistence: Data stored with Shared Preferences persists across app restarts, making it suitable for storing user preferences and settings.
Cross-Platform: Shared Preferences works consistently across both Android and iOS platforms, providing a unified data storage solution.
Shared Preferences is a valuable tool for storing small amounts of data persistently in Flutter applications. Whether you need to store user preferences, authentication tokens, or app settings, Shared Preferences offers a lightweight and efficient solution. By following the simple steps outlined in this article, you can leverage Shared Preferences to enhance the functionality and user experience of your Flutter apps.
Okay, now we moving on to medium-level data storage.
SQLite Database
SQLite is a lightweight, embedded SQL database engine that provides a full SQL implementation. It's widely used in mobile and desktop applications due to its simplicity, reliability, and efficiency. In Flutter, SQLite is commonly used for storing structured data, such as user profiles, shopping cart items, or any other form of relational data.
How SQLite Works in Flutter
Flutter provides a plugin called sqflite
for interacting with SQLite databases. This plugin allows you to perform CRUD (Create, Read, Update, Delete) operations on your database, execute SQL queries, and manage transactions. Under the hood, sqflite
uses platform-specific implementations to interact with the SQLite database engine, ensuring consistent behavior across different platforms.
Using SQLite Database in Flutter
Using SQLite in Flutter involves several steps, including creating and opening a database, defining tables and columns, and performing data manipulation operations. Here's a basic guide on how to use SQLite in Flutter:
- Add Dependency: First, you need to add the sqflite package to your pubspec.yaml file.
dependencies:
flutter:
sdk: flutter
sqflite: ^2.0.0+3
- Import Package: Import the sqflite package in your Dart file where you want to use SQLite.
import 'package:sqflite/sqflite.dart';
- Creating and Opening a Database: Use the
openDatabase
function to create and open a SQLite database.
Future<Database> openDatabase() async {
var databasesPath = await getDatabasesPath();
String path = join(databasesPath, 'example.db');
return openDatabase(path, version: 1,
onCreate: (Database db, int version) async {
await db.execute(
'CREATE TABLE users (id INTEGER PRIMARY KEY, name TEXT, age INTEGER)');
});
}
- Performing CRUD Operations: You can use various methods provided by the Database class to perform CRUD operations on your database.
// Inserting data into the database
void insertData() async {
Database database = await openDatabase();
await database.transaction((txn) async {
await txn.rawInsert(
'INSERT INTO users(name, age) VALUES("John Doe", 30)');
});
}
// Querying data from the database
void queryData() async {
Database database = await openDatabase();
List<Map> users = await database.rawQuery('SELECT * FROM users');
users.forEach((user) {
print('Name: ${user['name']}, Age: ${user['age']}');
});
}
Benefits of Using SQLite Database in Flutter
Using SQLite database in Flutter offers several benefits:
Reliability: SQLite is a highly reliable and well-tested database engine, ensuring data integrity and consistency.
Efficiency: SQLite is lightweight and efficient, making it suitable for mobile applications with limited resources.
Flexibility: SQLite supports a wide range of SQL features, including transactions, indexes, and constraints, giving you flexibility in how you structure and query your data.
Cross-Platform: SQLite works consistently across different platforms, allowing you to build cross-platform applications with ease.
SQLite database is a powerful tool for managing structured data in Flutter applications. Whether you're building a simple todo list app or a complex e-commerce platform, SQLite provides a reliable and efficient solution for storing and querying data. By understanding how SQLite works in Flutter and how to use it effectively, you can build robust and scalable applications that meet your data storage needs.
Alright devs, Now we explore our last Local Data storage in Flutter.
Hive
Hive is a lightweight and fast key-value database built specifically for Flutter. It's designed to be simple to use, highly performant, and versatile enough to handle a wide range of data storage needs. Hive is optimized for speed and memory usage, making it ideal for mobile applications with limited resources.
How Hive Works in Flutter
Hive uses a box-based storage model, where data is stored in boxes, each identified by a unique name. Each box can store key-value pairs, making it easy to organize and access your data. Hive supports various data types, including primitive types, lists, maps, and custom objects. It also provides built-in support for encryption and compression, ensuring the security and efficiency of your data storage.
Using Hive in Flutter
Using Hive in Flutter is straightforward and involves a few simple steps. Here's a basic guide on how to use Hive to store and retrieve data in your Flutter apps:
- Add Dependency: First, you need to add the hive package to your pubspec.yaml file.
yamlCopy codedependencies:
flutter:
sdk: flutter
hive: ^2.0.4
- Import Package: Import the hive package in your Dart file where you want to use Hive.
dartCopy codeimport 'package:hive/hive.dart';
- Initializing and Opening a Box: Use the
Hive.openBox
function to initialize and open a box for storing your data.
dartCopy codevoid openBox() async {
await Hive.initFlutter();
var box = await Hive.openBox('myBox');
}
- Storing and Retrieving Data: You can use the
put
andget
methods provided by the Box class to store and retrieve data.
dartCopy codevoid storeData() async {
var box = await Hive.openBox('myBox');
box.put('username', 'john_doe');
}
void retrieveData() async {
var box = await Hive.openBox('myBox');
String? username = box.get('username');
print('Username: $username');
}
Benefits of Using Hive in Flutter
Using Hive in Flutter offers several benefits:
Performance: Hive is highly performant, with fast read and write operations, making it suitable for use in mobile applications.
Simplicity: Hive provides a simple and intuitive API for storing and retrieving data, making it easy to use even for beginners.
Versatility: Hive supports various data types and provides flexibility in how you structure and organize your data.
Efficiency: Hive is optimized for memory usage, ensuring efficient utilization of device resources.
Hive is a powerful tool for simplifying data storage in Flutter applications. Whether you're building a simple todo list app or a complex e-commerce platform, Hive provides a reliable and efficient solution for storing and accessing your data. By understanding how Hive works and how to use it effectively, you can build robust and responsive applications that meet your data storage needs with ease.
So if sometimes you need to store data that also we get on another device then we use Firebase or Server. Let's explore Firebase data storage in Flutter.
Firebase Data Storage
Firebase Database is a cloud-hosted NoSQL database that allows you to store and sync data between your users in real-time. It's built on a hierarchical data structure, similar to JSON, making it flexible and scalable. Firebase Database offers real-time synchronization, offline capabilities, and powerful querying capabilities, making it ideal for building responsive and collaborative applications.
How Firebase Database Works in Flutter
Firebase Database provides a set of client SDKs for various platforms, including Flutter. The FlutterFire library provides a set of Flutter plugins that allow you to interact with Firebase services, including Firebase Database, from your Flutter applications. With FlutterFire, you can perform CRUD (Create, Read, Update, Delete) operations on your database, listen for real-time updates, and handle authentication and security.
Using Firebase Database in Flutter
Using the Firebase Database in Flutter involves several steps, including setting up a Firebase project, configuring authentication and security rules, and integrating Firebase SDK into your Flutter app. Here's a basic guide on how to use Firebase Database in Flutter:
Set Up Firebase Project: First, you need to set up a Firebase project in the Firebase console. Follow the instructions provided to create a new project and enable the Firebase Database.
Add Firebase to Your Flutter App: Next, add the necessary Firebase dependencies to your Flutter app by adding the
firebase_core
andfirebase_database
packages to your pubspec.yaml file.
dependencies:
flutter:
sdk: flutter
firebase_core: ^1.10.0
firebase_database: ^11.1.0
- Initialize Firebase: Initialize Firebase in your Flutter app by calling the
Firebase.initializeApp()
function in your main.dart file.
import 'package:firebase_core/firebase_core.dart';
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp();
runApp(MyApp());
}
- Perform CRUD Operations: You can now perform CRUD operations on your Firebase Database using the Firebase Database plugin. Here's an example of how to write data to the database:
import 'package:firebase_database/firebase_database.dart';
void writeData() {
DatabaseReference reference = FirebaseDatabase.instance.reference();
reference.child('users').set({'name': 'John Doe', 'age': 30});
}
Benefits of Using Firebase Database in Flutter
Using Firebase Database in Flutter offers several benefits:
Real-time Synchronization: Firebase Database provides real-time synchronization, allowing multiple clients to receive updates in real-time as the data changes.
Offline Capabilities: Firebase Database offers offline capabilities, allowing your app to continue functioning even when the device is offline.
Powerful Querying: Firebase Database provides powerful querying capabilities, allowing you to filter, sort, and limit data according to your requirements.
Scalability and Reliability: The Firebase Database is hosted on Google's infrastructure, providing scalability, reliability, and security out of the box.
Firebase Database is a powerful tool for building real-time, collaborative applications in Flutter. Whether you're building a chat app, a collaborative document editor, or a real-time dashboard, Firebase Database provides the features and capabilities you need to build responsive and engaging experiences for your users. By understanding how the Firebase Database works and how to integrate it into your Flutter app effectively, you can unlock the full potential of Firebase and build high-quality applications that delight your users.
Alright devs, This is the end of our blog i hope this blog helps you to easily understand Data storage in Flutter. Okay, then We catch up on our next topic: Navigation and Communication in Flutter.
Connect with Me:
Hey there! If you enjoyed reading this blog and found it informative, why not connect with me on LinkedIn? ๐ You can also follow my Instagram page for more mobile development-related content. ๐ฒ๐จโ๐ป Letโs stay connected, share knowledge and have some fun in the exciting world of app development! ๐
Subscribe to my newsletter
Read articles from Mayursinh Parmar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mayursinh Parmar
Mayursinh Parmar
๐ฑMobile App Developer | Android & Flutter ๐๐ก Passionate about creating intuitive and engaging apps ๐ญโจ Letโs shape the future of mobile technology!