Exploring Solidity: Creating a Simple Identity Smart Contract
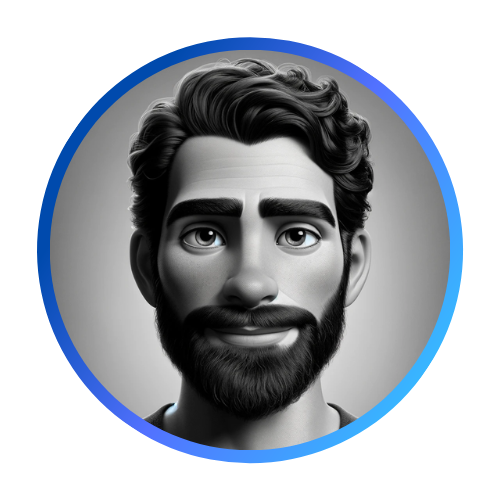
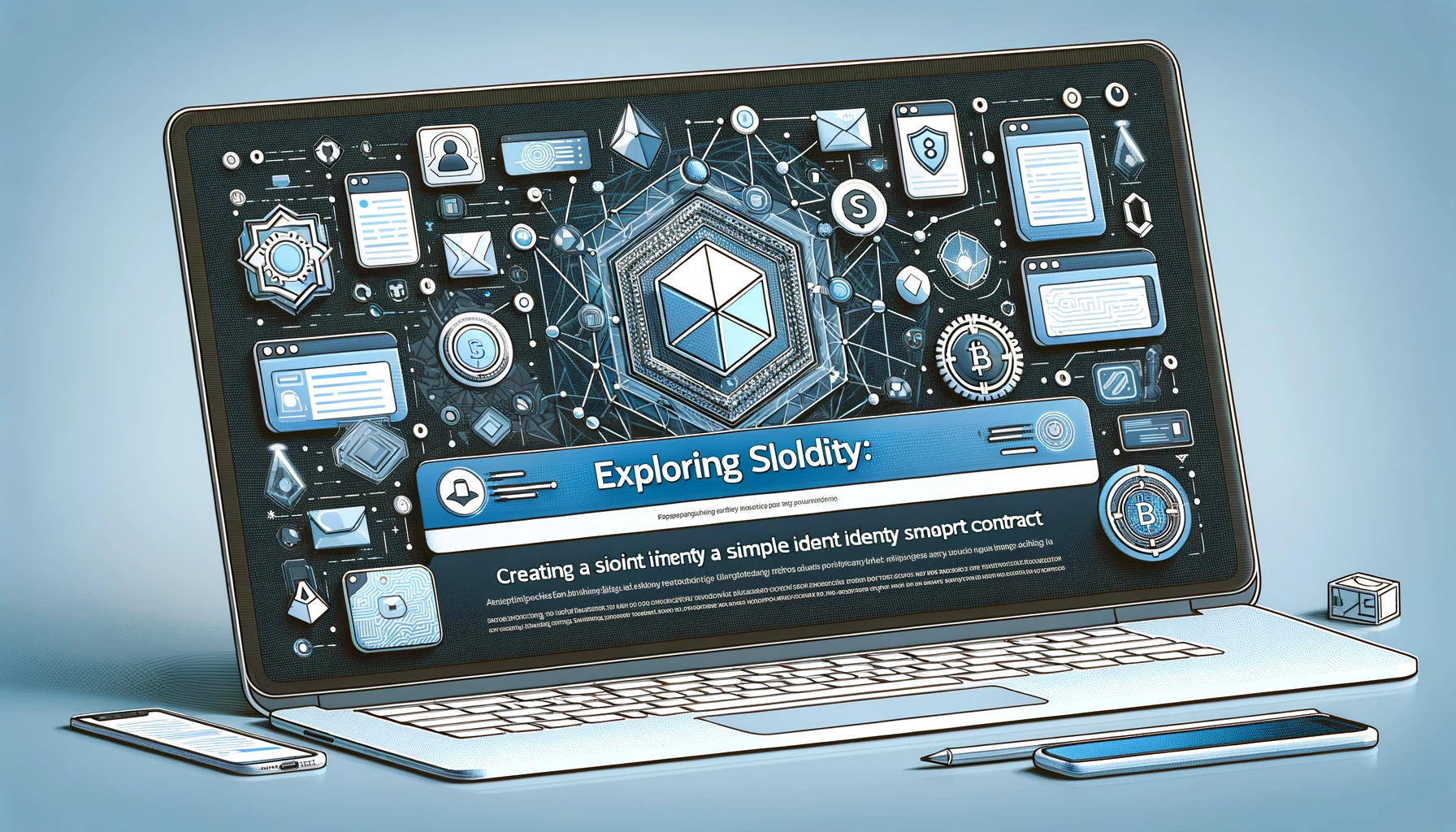
As part of my journey into blockchain development, I've been working on writing smart contracts using Solidity. Today, I'd like to share a simple smart contract I've created, called Identity
. This contract allows you to store and retrieve a person's name and age, as well as update the age.
The Smart Contract
Here's the complete code for the Identity
contract:
// SPDX-License-Identifier: MIT
pragma solidity >=0.5.0 <0.9.0;
contract Identity {
string private name;
uint private age;
constructor() public {
name = "Laljan";
age = 24;
}
function getName() public view returns (string memory) {
return name;
}
function getAge() public view returns (uint) {
return age;
}
function setAge(uint _age) public {
age = _age;
}
}
Understanding the Code
Let's break down the different parts of the contract:
License and Version Pragma:
// SPDX-License-Identifier: MIT pragma solidity >=0.5.0 <0.9.0;
The SPDX license identifier ensures that the license type is clear. The pragma directive specifies that the code is written for Solidity versions between 0.5.0 (inclusive) and 0.9.0 (exclusive).
Contract Definition:
contract Identity {
This line declares a new contract named
Identity
.State Variables:
string private name; uint private age;
These variables store the name and age of the person. They are marked as
private
to restrict direct access from outside the contract.Constructor:
constructor() public { name = "Laljan"; age = 24; }
The constructor initializes the state variables with default values. It is executed only once when the contract is deployed.
Getters:
function getName() public view returns (string memory) { return name; } function getAge() public view returns (uint) { return age; }
These functions allow external users to read the values of
name
andage
.Setter:
function setAge(uint _age) public { age = _age; }
This function allows the age to be updated. It takes a new age as an input and updates the state variable
age
.
Deploying and Interacting with the Contract
To deploy and interact with this contract, you can use Remix, an online Solidity IDE. Here are the steps:
Open Remix: Go to Remix.
Create a New File: Create a new file and name it
Identity.sol
. Copy and paste the contract code into this file.Compile the Contract: Click on the "Solidity Compiler" tab and compile the contract. Ensure that the compiler version matches the pragma statement in your contract.
Deploy the Contract: Go to the "Deploy & Run Transactions" tab, select
Identity
from the dropdown menu, and deploy the contract.Interact with the Contract: Once deployed, you can interact with the contract using the provided interface. You can call
getName
andgetAge
to retrieve the current values and usesetAge
to update the age.
Conclusion
This simple Identity
contract is a great starting point for learning Solidity and smart contract development. It covers basic concepts like state variables, functions, visibility, and contract deployment. As I continue my journey, I'll be exploring more complex contracts and sharing my progress. Stay tuned for more updates!
Written by ljb630 | Trying to find my place in the web3 community! | Technical Writer & Researcher | Team Lead
Subscribe to my newsletter
Read articles from ljb630 directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
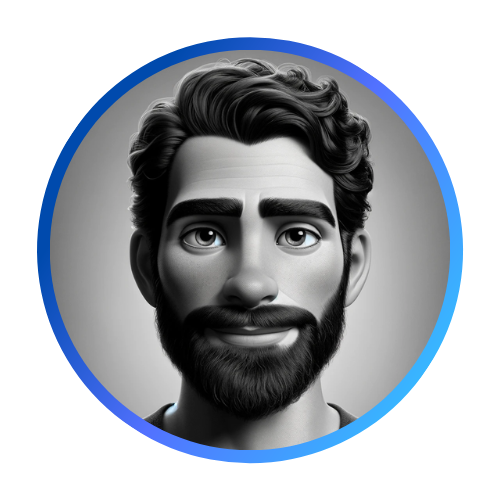
ljb630
ljb630
Trying to find my place in the web3 community! | Technical Writer & Researcher | Team Lead