Defining Constants in Flutter: Best Practices
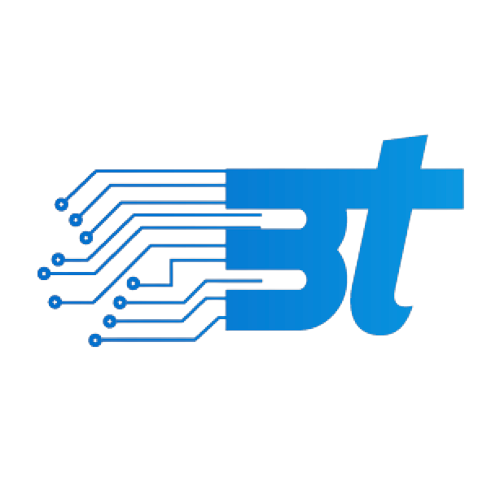
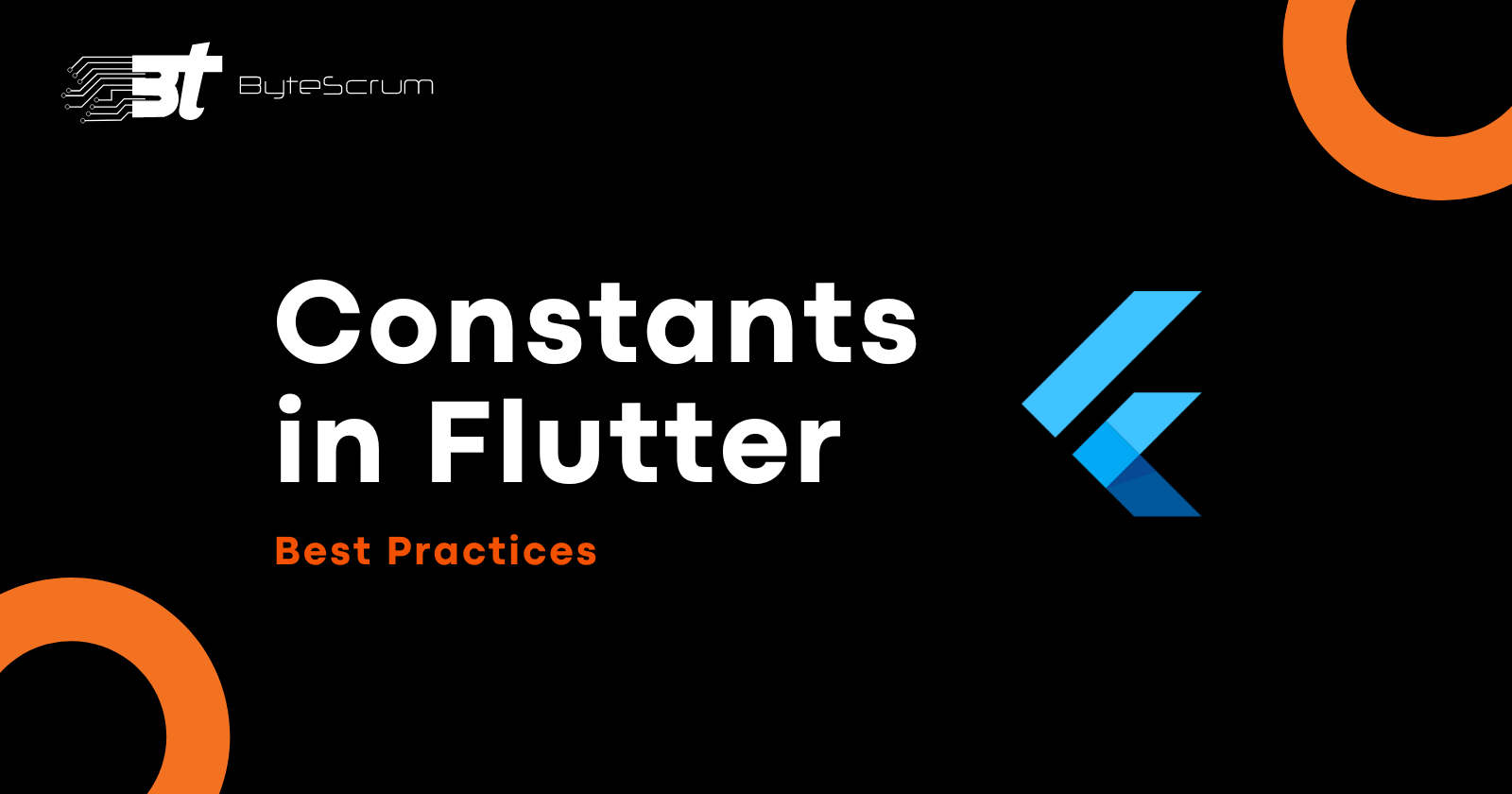
Introduction
When building a Flutter application, you often encounter values that remain unchanged throughout the app's lifecycle. These immutable values, known as constants, can be effectively managed to keep your code clean, efficient, and easy to maintain. This blog post explores the best practices for defining and using constants in Flutter.
1. Using the const
Keyword
For simple, unchanging values like strings, numbers, and booleans, the const
keyword is your go-to solution. Constants defined this way are compile-time constants, meaning their values are fixed at compile-time and cannot be altered.
const String appTitle = 'My Flutter App';
const int maxUsers = 100;
const double pi = 3.14159;
2. Grouping Constants in a Class
To keep your constants organized, especially when they are related, define them within a class. This approach enhances readability and maintainability.
class Constants {
static const String appTitle = 'My Flutter App';
static const int maxUsers = 100;
static const double pi = 3.14159;
}
Usage:
For a set of related constants, consider using an enum
. Enums are particularly useful for defining a fixed set of related values, such as different environments or states.
Text(Constants.appTitle);
3. Using Enums for Related Constants
For a set of related constants, consider using an enum
. Enums are particularly useful for defining a fixed set of related values, such as different environments or states.
enum AppEnvironment {
development,
staging,
production
}
void main() {
const environment = AppEnvironment.development;
switch (environment) {
case AppEnvironment.development:
print('Development Environment');
break;
case AppEnvironment.staging:
print('Staging Environment');
break;
case AppEnvironment.production:
print('Production Environment');
break;
}
}
4. Defining Constant Widgets
In Flutter, you can define constant widgets to optimize performance. By marking widgets as const
, you ensure they are created only once and reused efficiently, reducing rebuilds.
const Text myConstantTextWidget = Text(
'Hello, Flutter!',
style: TextStyle(fontSize: 20),
);
Practical Example
Here's a practical example that demonstrates how to use constants in a Flutter application:
import 'package:flutter/material.dart';
class Constants {
static const String appTitle = 'My Flutter App';
static const Color primaryColor = Colors.blue;
}
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: Constants.appTitle,
theme: ThemeData(
primarySwatch: Constants.primaryColor,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(Constants.appTitle),
),
body: Center(
child: Text('Hello, world!'),
),
);
}
}
In this example, Constants
is a class that holds the app's title and primary color as static constants. This approach centralizes the constant values, making the code easier to manage and update.
Conclusion
const
keyword, grouping related constants in a class, utilizing enums for sets of related values, and defining constant widgets, you can ensure your Flutter app is efficient and easy to maintain.Happy coding!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
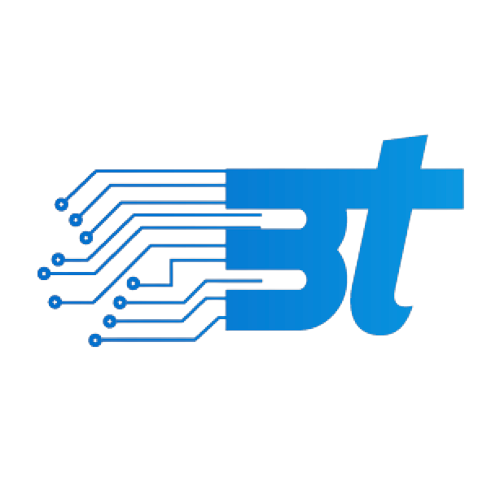
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.