Daily Hack #day47 - Java Streams: Summarizing Your Data
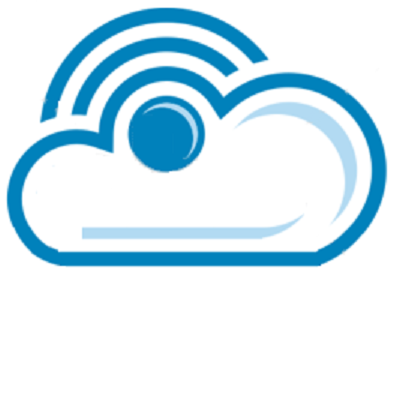
2 min read
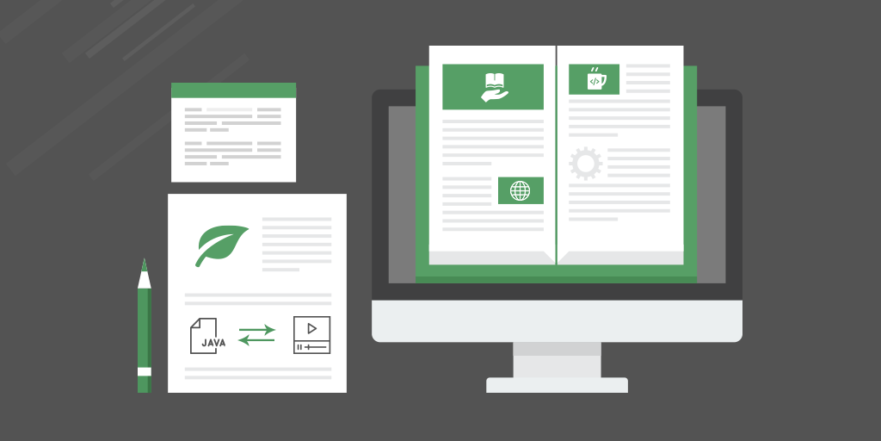
The reduce
operation accumulates elements within a stream into a single result.
This is useful for calculating various statistics or summarizing your data. Here’s an example of finding the total price of all products:
int totalPrice = products.stream()
.mapToInt(product -> product.getPrice()) // Map to int stream of prices
.reduce(0, Integer::sum); // Reduce using sum function
This code first maps the product stream to an IntStream
containing just the prices.
Then, reduce with the Integer::sum
method efficiently calculates the sum of all prices, providing the total cost.
Here is a code example you can copy/modify at your own pace to see the above concept in action:
import java.util.ArrayList;
import java.util.List;
class Product {
private String name;
private int id;
private double price;
public Product(String name, int id, double price) {
this.name = name;
this.id = id;
this.price = price;
}
public double getPrice() {
return price;
}
}
public class ProductSum {
public static void main(String[] args) {
// Create a list of products
List<Product> products = new ArrayList<>();
products.add(new Product("Product 1", 1, 10.0));
products.add(new Product("Product 2", 2, 20.0));
products.add(new Product("Product 3", 3, 30.0));
// Calculate the sum of all product prices using Streams API
double totalPrice = products.stream()
.mapToDouble(Product::getPrice)
.reduce(0, Double::sum);
System.out.println("Total price of all products: " + totalPrice);
}
}
In this program:
- We define a
Product
class with three fields:name
,id
, andprice
. - We create a list of
Product
objects and populate it with some sample data. - We use the
stream()
method on theproducts
list to obtain a stream ofProduct
objects. - We use the
mapToDouble()
method to map eachProduct
object to its price (double
value). - We use the
sum()
terminal operation to calculate the sum of all the prices in the stream. - Finally, we print the total price of all products.
0
Subscribe to my newsletter
Read articles from Cloud Tuned directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
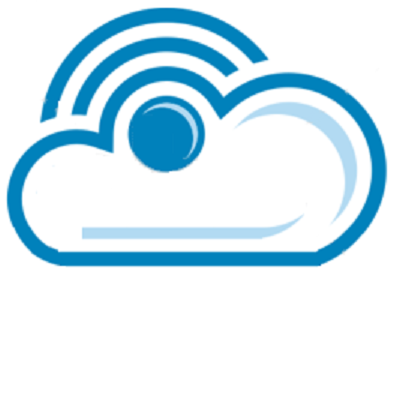