Understanding Threads and Processes in Node.js: A Comprehensive Guide

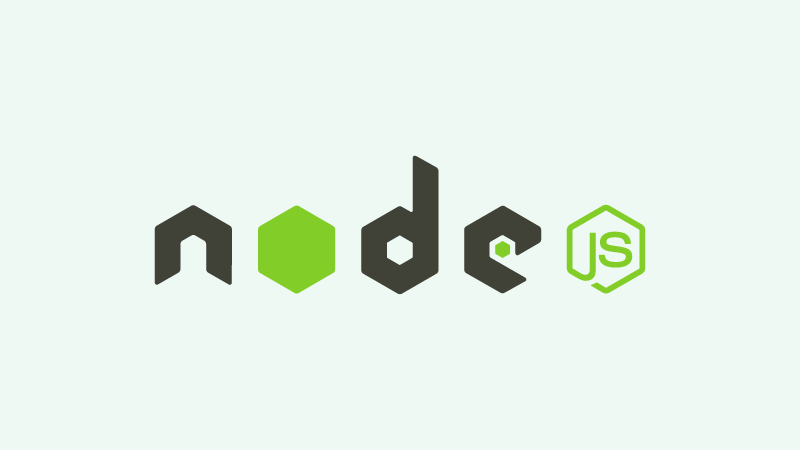
In Node.js, understanding how threads and processes work is crucial for building efficient and scalable applications. Let’s dive into the details of threads, processes, and their interaction with the libuv library.
Threads and Processes
Threads
A thread in Node.js is a separate execution context within a single process. It’s a lightweight, independent unit of processing that can run in parallel with other threads within the same process.
Each thread resides within the process memory and has its own execution pointer. However, they share the heap of the process.
Threads are essential for handling concurrent tasks efficiently. They allow us to perform multiple operations simultaneously, improving overall performance.
Processes
A process represents an independent program execution in the operating system. It has its own memory space, file descriptors, and other resources.
In Node.js, each Node process runs in a single thread by default. However, Node.js itself is multithreaded and provides hidden threads through the libuv library.
Libuv handles I/O operations like reading files from disk or making network requests. It allows your code to make I/O requests without blocking the main thread1
Libuv: The Heart of Node.js
What is Libuv?
Libuv is a C library originally written for Node.js to abstract non-blocking I/O operations.
It enables an event-driven asynchronous I/O model, allowing the CPU and other resources to be used simultaneously while still performing I/O operations efficiently.
Libuv facilitates a callback-based approach for I/O and other activities, preventing wastage of system resources.
Key features of libuv include handling services like File System, DNS, network, child processes, pipes, signal handling, polling, and streaming.
Event Loop
The event loop is at the core of libuv. It uses a single-threaded asynchronous I/O approach.
When a network request or any other asynchronous operation occurs, a callback is registered.
The event loop listens for incoming I/O and emits events for each request.
After successful execution, the registered callback is enqueued in the event queue and executed by the main thread.
Libuv caches the current time during each iteration of the loop to minimize frequent system calls
Thread Pool
While libuv doesn’t use threads for asynchronous tasks, it employs a thread pool for operations that can’t be done asynchronously at the OS level.
For example, libuv uses threads to make synchronous file system (fs) calls asynchronous.
-
Examples
Asynchronous File Read
const fs = require('fs'); fs.readFile('example.txt', 'utf8', (err, data) => { if (err) { console.error('Error reading file:', err); } else { console.log('File content:', data); } });
In this example, the file read operation is non-blocking, thanks to libuv.
HTTP Server
const http = require('http'); const server = http.createServer((req, res) => { res.writeHead(200, { 'Content-Type': 'text/plain' }); res.end('Hello, Node.js!'); }); server.listen(8080, () => { console.log('Server listening on port 8080'); });
The HTTP server handles multiple client requests concurrently using libuv’s event loop.
Conclusion
Understanding threads, processes, and libuv is essential for writing efficient Node.js applications. By leveraging asynchronous I/O and libuv’s features, you can build high-performance systems that scale gracefully.
Remember, Node.js empowers you to create powerful applications by harnessing the underlying magic of threads and processes! 🚀🌟
Subscribe to my newsletter
Read articles from Vishwajit Vm directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vishwajit Vm
Vishwajit Vm
Hi there, I'm Vishwajit vm, a skilled Laravel and PHP developer. With years of experience in the field, I have a proven track record of delivering high-quality projects on time and within budget. I am passionate about using my expertise in Laravel and PHP to create innovative and efficient web applications that meet the unique needs of each project. I am always open to collaboration and am eager to work with others on any project related to Laravel and PHP. My goal is to deliver the best possible results for each project, using my deep understanding of the framework and its capabilities to create custom solutions that meet the specific needs of each project. In my free time, I enjoy pursuing my hobbies of photography, cycling, and playing video games. I also have a YouTube channel where I share my knowledge and insights on Laravel and PHP development, offering valuable tips and advice to others in the field. If you're looking for a skilled Laravel and PHP developer who is passionate about their work and dedicated to delivering the best possible results, feel free to reach out to me at vishwajitmall50@gmail.com or call me at 91-8920352115. I'm always happy to discuss potential projects and collaborations.