What is Data Structure in Python? – Types, Classifications, & Applications
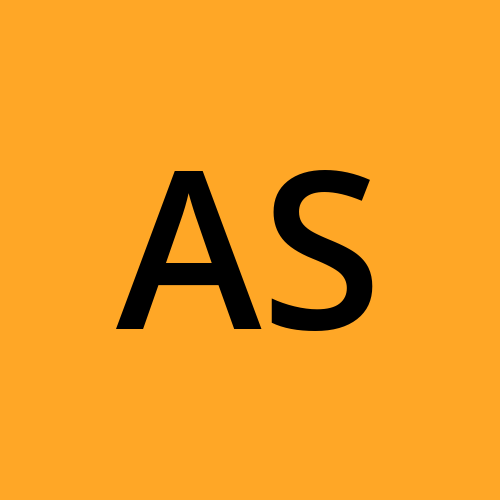
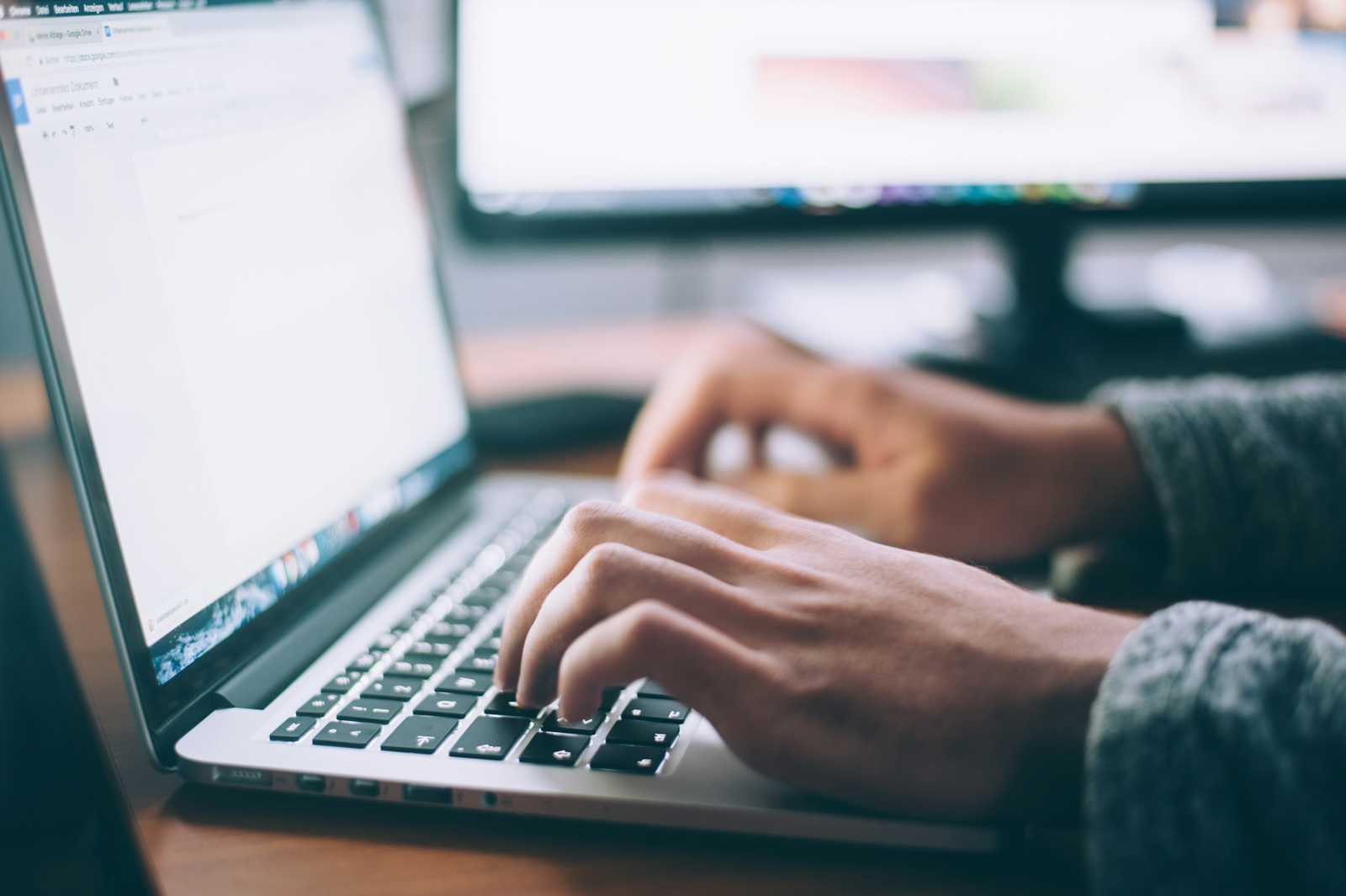
In programming, data structures are the fundamental building blocks that organize and store information efficiently. Just like a toolbox holds various instruments for specific tasks, Python offers a rich set of data structures, each designed to excel at handling different kinds of data and operations.
Understanding these structures is paramount for writing effective and efficient Python programs. This blog post provides a foundational understanding of data structures in Python.
As you delve deeper into programming, explore additional resources to grasp more advanced structures like graphs and heaps. Remember, effectively utilizing data structures is a cornerstone of becoming a proficient Python programmer.
What is a Data Structure in Python?
In Python, a data structure is a specialized format used to organize, store, access, and manipulate data. It defines how data elements are arranged in memory and how they relate to each other. Choosing the right data structure for your program is crucial for its efficiency and functionality.
Think of it like this: if data were a collection of tools, a data structure would be the toolbox that holds them. Different tools are suited for different tasks, and data structures work the same way.
Lists are great for storing items in a specific order, like a shopping list, while dictionaries are perfect for storing key-value pairs like phonebooks.
Key Classifications of Data Structures in Python
Python data structures fall into two categories: linear and non-linear. Linear structures like lists and stacks arrange elements in a sequence, accessed by position. Non-linear structures like dictionaries and sets don't have a strict order and use keys or pointers for retrieval. Here is the description of the same:
Linear Data Structures
These structures arrange elements in a sequential order, similar to beads on a string. You can access elements based on their position (index) in the sequence. Here are some common examples:
Lists: Ordered, mutable collections that can hold various data types. They're flexible and often used for general-purpose storage of sequences needing modification. (Example: [apple, banana, cherry] )
Tuples: Ordered, immutable collections similar to lists, but their elements cannot be changed after creation. Tuples are ideal for storing fixed data sets that shouldn't be modified. (Example: (10, 20, 30) )
Stacks: LIFO (Last-In-First-Out) data structure. The element added last is accessed first, like a stack of plates. Stacks are commonly used to implement undo/redo functionality or manage function calls. (Example: Imagine a stack of plates where you can only take the top one off)
Queues: FIFO (First-In-First-Out) data structure. The added element is accessed first, similar to a line of waiting people. Queues are useful for simulating tasks in a printer queue or processing messages in a network. (Example: Imagine a line of people where the first person in line gets served first)
Non-Linear Data Structures
These structures' elements are not arranged sequentially. They can have complex relationships and are accessed using keys or pointers. Here are some key examples:
Sets: Unordered collections of unique elements. Sets help store collections where duplicates are not allowed and for checking for memberships. (Example: {apple, banana, cherry} - notice duplicates are automatically removed)
Dictionaries: Unordered collections of key-value pairs. Imagine an address book where names are keys and phone numbers are values. Dictionaries are efficient for retrieving data based on unique keys. (Example: {"Alice": "123-456-7890", "Bob": "987-654-3210"})
Trees: Hierarchical data structures with a parent-child relationship between elements. They are used for efficient searching and sorting (e.g., binary search trees). Imagine an organizational chart where the CEO is the root and employees branch out from there.
Applications of Data Structures in Python
The power of data structures in Python comes into play when you need to organize and manipulate data efficiently. Here are some real-world applications of different data structures:
Lists
Storing shopping lists or to-do lists where the order matters. Keeping track of student grades in a class. Representing social media follower lists.
Tuples
Defining fixed data like coordinates (x, y) on a graph. Storing employee records with elements like (name, ID, department).
Stacks
Implementing undo/redo functionality in text editors or image editing software. Managing function calls during program execution.
Queues
Simulating tasks in a printer queue where documents are processed in the order they are received. Handling messages in a network where messages are delivered in the order they are sent.
Sets
Checking for plagiarism by finding duplicate entries in a list of submitted assignments. Identifying unique words in a text document.
Dictionaries
Building phonebook applications where names are keys and phone numbers are values. Storing user profiles on a website where usernames are keys and profiles are values. Implement configuration settings for an application where settings names are keys and values are settings options.
By understanding these applications and choosing the right data structure for the task, you can write Python programs that are functional and perform optimally. As your programming journey progresses, you'll encounter even more intricate applications of data structures in various domains.
Mastering these data structures will unlock the potential to write robust, adaptable, and efficient Python programs.
Best Practices for Data Structures in Python
Choosing the right data structure is crucial for writing efficient and Pythonic code. Here are some best practices to keep in mind:
Select the Right Tool for the Job
Understand the characteristics of your data: size, data type, and the operations you need to perform on it. Don't be afraid to experiment with different structures to see which performs best for your specific task.
Leverage Pythonic Features
Utilize built-in functions and methods specific to each data structure. For example, use list.append() to add elements to a list or dict.get() to retrieve values from a dictionary. Employ list comprehensions and dictionary comprehensions for concise and efficient data manipulation.
Prioritize Clarity and Readability
Choose descriptive variable names that reflect the data structure's contents. Use comments to explain complex logic or the purpose of specific data structures within your code.
Optimize for Performance
For frequently accessed data, consider using efficient data structures like dictionaries for fast lookups by key. If you need to preserve the order of elements and avoid duplicates, sets are a good choice.
Be mindful of time and space complexity when choosing a data structure for large datasets.
Utilize Built-in Modules When Necessary
Python offers libraries like collections that provide specialized data structures like deque for efficient queues or defaultdict for dictionaries with default values.
Consider Mutability
If your data needs to remain unchanged, opt for immutable structures like tuples. This prevents accidental modifications and improves code safety.
Profile Your Code
Use profiling tools to identify bottlenecks in your code related to data structures. This can help you optimize your choice and usage.
Stay Updated
Python is constantly evolving, so keep yourself updated on new data structures and functionalities that might be better suited for your needs.
By following these best practices, you'll write Python code that is not only efficient but also clear, maintainable, and leverages the strengths of different data structures.
Frequently Asked Questions
What are some common examples of data structures in Python?
Lists store ordered collections of items, dictionaries store key-value pairs, sets store unique elements, stacks follow LIFO (Last-In-First-Out) order, and queues follow FIFO (First-In-First-Out) order.
What are some applications of data structures in Python?
Data structures are used everywhere! Lists store shopping lists, dictionaries power phonebook apps, sets check for duplicate entries, stacks manage function calls, and queues simulate printer queues.
Why are data structures important in Python programming?
Understanding data structures empowers you to write efficient and organized Python programs. Choosing the right structure optimizes how your program handles and retrieves data, leading to better performance and cleaner code.
Conclusion
By understanding these applications and choosing the right data structure in Python for the task, you can write Python programs that are not only functional but also perform optimally. As your programming journey progresses, you'll encounter even more intricate applications of data structures in various domains.
Ready to take your Data Science skills to the next level?
Data structures are the building blocks of efficient and powerful Python programs, essential for any aspiring Data Scientist. But navigating the complexities of lists, dictionaries, and beyond can feel overwhelming.
Pickl.AI's Python for Data Science course empowers you to understand linear and non-linear data structures in Python. With this knowledge, you'll be able to confidently choose the right data structure for any task, optimize your code for efficiency, and ultimately unlock a broader range of Data Science concepts and applications.
Don't let data structures impede your progress. Enroll in Pickl.AI's course today and empower yourself to become a proficient Data Scientist.
Subscribe to my newsletter
Read articles from Alex Smith directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
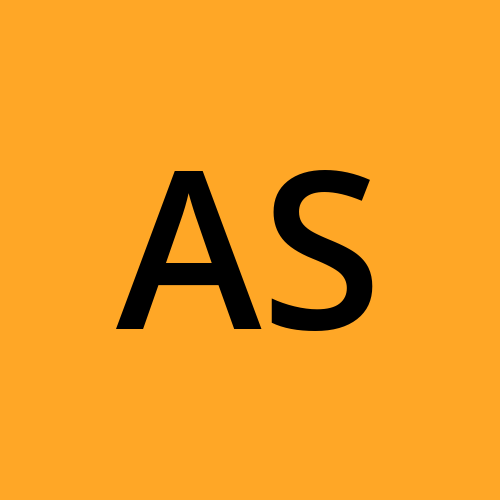
Alex Smith
Alex Smith
i am professional data science expert