gRPC API: Enhancing Remote Procedure Calls Explained
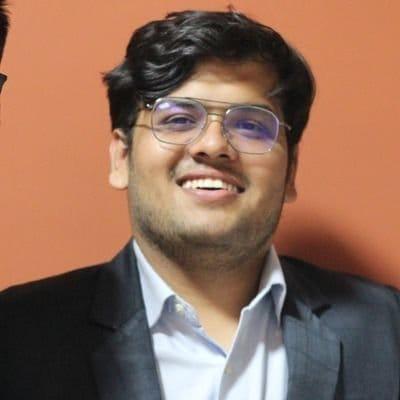
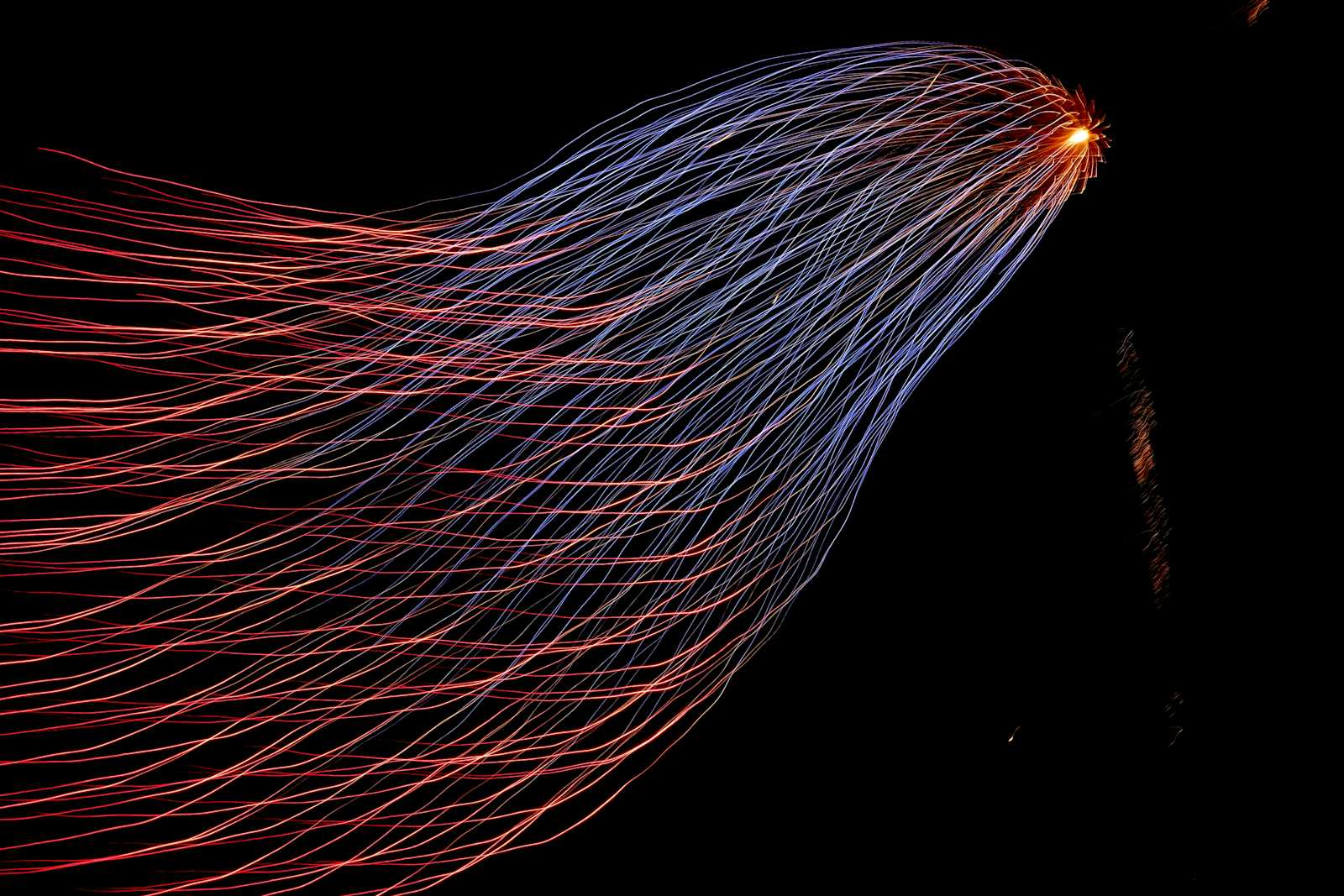
Introduction
I am excited to share this comprehensive blog series on gRPC, a powerful framework for efficient and high-performance remote procedure calls (RPC) across various tech stacks. This blog has been in development for quite some time, and I am thrilled to present an in-depth overview of gRPC, its core features, and how it compares to other widely-used communication protocols like REST, SOAP, WebSockets, and GraphQL.
In this first installment, we will explore the unique advantages of gRPC, including its language-agnostic nature, efficient data serialization with Protocol Buffers, and the benefits of leveraging HTTP/2. We will also examine why RPC is a valuable tool for building scalable, high-performance distributed systems, and how it stands out from traditional protocols.
Stay tuned for the second part of this series, where we will take a deeper dive into the implementation of gRPC. We will walk through setting up a gRPC service, defining data structures using .proto files, and generating source code in various programming languages. Whether you are a seasoned developer or new to RPC, this series will equip you with the knowledge and tools to harness the full potential of gRPC in your projects. Let's get started!
One of the most notable features of gRPC is its complete language agnosticism, which means it can work seamlessly across different programming languages. This flexibility allows developers to build a server in one language and a client in another without any compatibility issues. For instance, you can create a server in Go and a client in Dart, or set up a server in Rust and have clients written in C++, Java, or Swift. The possibilities are extensive as gRPC supports many other languages as well, including Python, Ruby, and Node.js. The underlying Protocol Buffers specification is designed to be entirely language-neutral, which not only enhances its performance but also makes it incredibly versatile. This language neutrality ensures that data serialization and deserialization are efficient, leading to faster communication between services. As a result, gRPC is an excellent choice for building scalable, high-performance distributed systems that require interoperability across diverse tech stacks.
To get started with Protocol Buffers, you need to install the necessary tools. First, visit the Protocol Buffers installation page to download the appropriate package for your operating system. Once you have selected and downloaded the correct version, open your command prompt or terminal. Follow the instructions provided on the website to complete the installation process. Typically, you will need to run a command similar to the one below to install the Protocol Buffer compiler:
# Example command for installation
protoc --version
This command checks if the installation was successful and verifies the version of the Protocol Buffer compiler. Make sure to follow any additional setup steps specific to your operating system to ensure everything is configured correctly. Once installed, you can start defining your data structures using .proto
files and generate source code in your preferred programming languages. This setup is crucial for enabling efficient and language-neutral communication between your gRPC services.
Why do we need RPC when we already have REST, SOAP, WebSockets, and GraphQL?
Let's delve into the specifics to understand the unique advantages of RPC.
When a request is made from the browser, the browser handles the fetch request, maintains HTTP communication with the server, and manages security protocols using transport layer security (TLS). This entire process is facilitated by the HTTP library and SOAP client-based library, which are responsible for ensuring that the data is transmitted securely and reliably.
REST and SOAP are well-established protocols that allow for communication between different systems over HTTP. REST is known for its simplicity and statelessness, making it easy to use and scale. SOAP, on the other hand, is more rigid but offers built-in error handling and security features, making it suitable for enterprise-level applications.
WebSockets provide a full-duplex communication channel over a single, long-lived connection, which is ideal for real-time applications like chat apps and live updates. GraphQL, a more recent addition, allows clients to request exactly the data they need, reducing over-fetching and under-fetching of data.
However, RPC (Remote Procedure Call) offers a different approach. RPC allows a program to execute a procedure (subroutine) on a remote server as if it were a local call. This means that the complexities of network communication are abstracted away, making it easier for developers to build distributed systems. RPC frameworks like gRPC use Protocol Buffers for efficient serialization, which results in faster communication and smaller payloads compared to JSON or XML used in REST and SOAP.
gRPC uses Protocol Buffers to serialize data, resulting in binary and smaller data sizes. In contrast, REST uses JSON, which is a text-based format and generally results in larger data sizes. This makes gRPC more efficient in terms of data transmission and performance.
Moreover, RPC supports multiple programming languages, enabling seamless interoperability across diverse tech stacks. This is particularly beneficial in microservices architectures where different services might be written in different languages.
In summary, while REST, SOAP, WebSockets, and GraphQL each have their strengths, RPC provides a streamlined and efficient way to perform remote operations, making it a valuable tool in the developer's toolkit for building scalable and high-performance distributed systems.
Setup
Setting up an RPC framework like gRPC involves several steps to ensure efficient and seamless communication between different services. Here’s a detailed guide to help you get started:
Dart: In Dart, you can use the pub package GRPC
to add gRPC functionality to your applications. This package provides all the necessary tools and libraries to implement gRPC in your Dart projects.
Go: To use gRPC in Go, run the command go get google.golang.org/grpc
. This will download and install the gRPC package, enabling you to start building gRPC services and clients in your Go applications.
Java: Java developers can use JARs from the Maven Central Repository to add gRPC support to their projects. By including the appropriate dependencies in your Maven pom.xml
file, you can easily integrate gRPC into your Java applications.
Kotlin: Similar to Java, Kotlin developers can also use JARs from the Maven Central Repository. By adding the necessary dependencies to your build configuration, you can leverage gRPC in your Kotlin projects.
Node: For Node.js, you can run npm install grpc
to add the gRPC package to your project. This package provides all the functionality needed to create gRPC clients and servers in a Node.js environment.
Objective-C: To integrate gRPC in Objective-C, add the gRPC-ProtoRPC
dependency to your podspec file. This will include the gRPC libraries in your project, allowing you to implement gRPC communication in your iOS applications.
C#: For C# developers, the easiest way to integrate gRPC is by using the NuGet package Grpc
. This package simplifies the process of adding gRPC support to your .NET applications, allowing you to quickly set up and start making remote procedure calls.
PHP: In PHP, you can run pecl install grpc
to install the gRPC extension. This extension enables you to use gRPC for remote procedure calls in your PHP applications.
Python: Python developers can run pip install grpcio
to install the gRPC package. This package provides the necessary tools to create gRPC clients and servers in Python.
Ruby: For Ruby, you can run gem install grpc
to add the gRPC gem to your project. This gem includes all the libraries needed to implement gRPC in your Ruby applications.
WebJS: For web applications using JavaScript, follow the grpc-web instructions to set up gRPC in your project. This will enable you to use gRPC for communication between your web clients and servers.
Messaging Format
gRPC uses Protocol Buffers (Protobuf) for data serialization. Protobuf is a highly efficient binary serialization format that results in smaller data sizes compared to text-based formats like JSON. This efficiency not only reduces the amount of data transmitted over the network but also speeds up the serialization and deserialization processes. Protobuf allows you to define your data structures in .proto files, which are then used to generate source code in various programming languages. This ensures that your data structures are consistent across different parts of your application, regardless of the language used.
HTTP Protocol
gRPC leverages HTTP/2 for its transport protocol. HTTP/2 provides several advantages over HTTP/1.1, including multiplexing, header compression, and improved performance. Multiplexing allows multiple requests and responses to be sent over a single connection simultaneously, reducing latency and improving throughput. Header compression reduces the size of HTTP headers, which can be significant in reducing the overhead for each request. These features combined make HTTP/2 a robust and efficient protocol for gRPC communication, leading to faster and more reliable interactions between clients and servers.
Communication
gRPC supports both Unary Client-Request and Bidirectional communication. Unary Client-Request allows a client to send a single request and receive a single response, similar to traditional HTTP requests. This is useful for simple, one-off interactions. On the other hand, Bidirectional communication enables continuous streaming of data between the client and server. This can be particularly useful for real-time applications where data needs to be continuously updated, such as live chat applications, real-time analytics, or collaborative tools. gRPC also supports client-side streaming and server-side streaming, providing flexibility in how data is transmitted.
Code Generation
gRPC uses the Native Protoc Compiler to generate code from .proto files. This compiler generates code in various programming languages, making it easier to implement gRPC services and clients across different tech stacks. By defining your service methods and message types in .proto files, you can automatically generate client and server code in languages like C++, Java, Python, Go, Ruby, and more. This not only speeds up development but also ensures that your gRPC services are consistent and compatible across different platforms. The generated code includes all the necessary boilerplate, allowing developers to focus on implementing the business logic.
Subscribe to my newsletter
Read articles from tarun directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
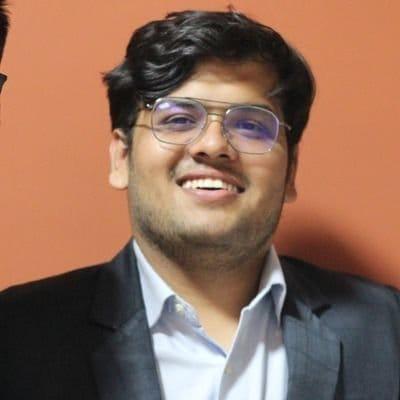
tarun
tarun
I am Seasoned web dev and in free time I sleep