Custom Image Widget: File Image, Network Image, SVG Image, and Asset Image can support this widget with Image Shape
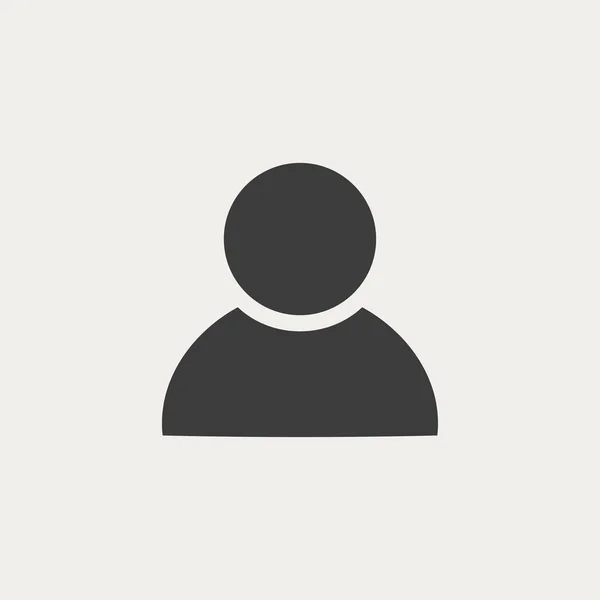
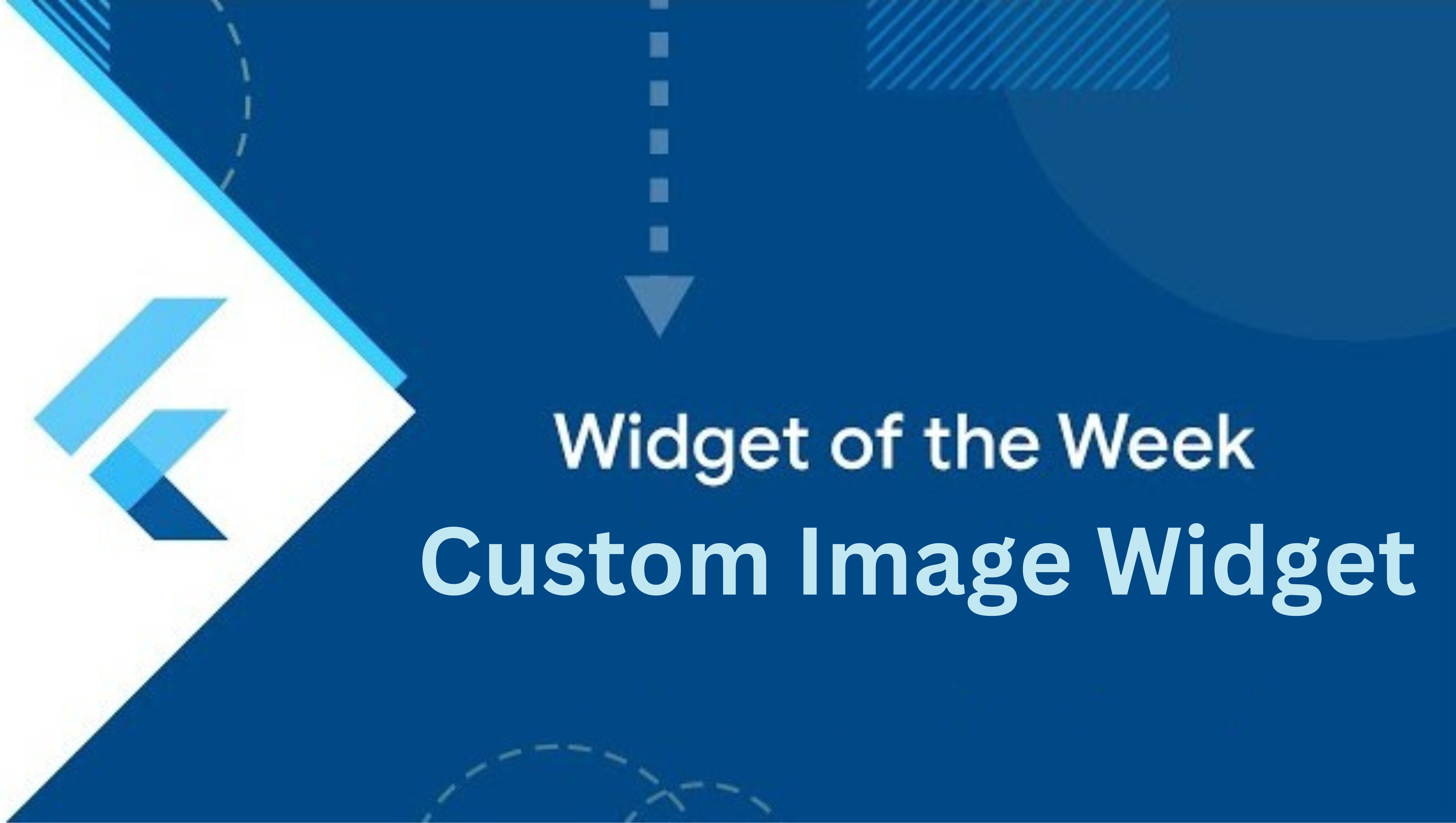
"In Flutter development, images play a pivotal role in user engagement and visual appeal. With the customImageBuilderWidget
function, you can elevate your app's image-handling capabilities to new heights. This blog explores the power and flexibility of this custom image widget, offering insights into its features and usage scenarios.
Discover how to seamlessly integrate various image types, including network images, asset images, and memory images, into your Flutter app. Learn to dynamically adjust image dimensions, apply custom shapes such as rectangles and circles, and add stylish border radii for that extra touch of finesse.
Whether you're fetching images from URLs, loading assets from your project, or rendering images from memory, this widget provides a comprehensive solution. With built-in support for SVG images and error handling, it ensures a smooth and reliable image viewing experience for your users.
Join us as we delve into the intricacies of image rendering in Flutter and unlock the full potential of the customImageBuilderWidget
. Elevate your app's visuals and create captivating user experiences that leave a lasting impression."
import 'dart:convert';
import 'dart:io';
import 'dart:typed_data';
import 'package:cached_network_image/cached_network_image.dart';
import 'package:flutter/material.dart';
import 'package:flutter_svg/flutter_svg.dart';
class CommonWidget {
static Widget customImageBuilderWidget({
required String image,
double? width,
double? height,
BoxFit fit = BoxFit.cover,
Widget? placeholder,
BorderRadius? borderRadius,
BoxShape shape = BoxShape.rectangle,
Color? color,
}) {
Widget buildLoadingPlaceholder() {
return const Center(child: CircularProgressIndicator());
}
Widget buildErrorPlaceholder() {
return const Icon(Icons.error);
}
Widget applyBorderRadius(Widget child) {
if (borderRadius != null) {
return ClipRRect(
borderRadius: borderRadius,
child: child,
);
} else {
return child;
}
}
Widget applyShape(Widget child) {
if (shape == BoxShape.circle) {
return ClipOval(child: child);
} else {
return applyBorderRadius(child);
}
}
if (image.startsWith('http') || image.startsWith('https')) {
// Network image
if (image.endsWith('.svg')) {
return applyShape(
SvgPicture.network(
image,
width: width,
height: height,
fit: fit,
placeholderBuilder: (BuildContext context) => placeholder ?? buildLoadingPlaceholder(),
colorFilter: color != null ? ColorFilter.mode(color, BlendMode.srcIn) : null,
),
);
} else {
return applyShape(
CachedNetworkImage(
imageUrl: image,
width: width,
height: height,
fit: fit,
placeholder: (context, url) => placeholder ?? buildLoadingPlaceholder(),
errorWidget: (context, url, error) => buildErrorPlaceholder(),
errorListener: (value) => buildErrorPlaceholder(),
),
);
}
} else if (image.startsWith('asset')) {
// Asset image
if (image.endsWith('.svg')) {
return applyShape(
SvgPicture.asset(
image,
width: width,
height: height,
fit: fit,
colorFilter: color != null ? ColorFilter.mode(color, BlendMode.srcIn) : null,
placeholderBuilder: (BuildContext context) => placeholder ?? buildLoadingPlaceholder(),
),
);
} else {
return applyShape(
Image.asset(
image,
width: width,
height: height,
fit: fit,
errorBuilder: (context, error, stackTrace) => buildErrorPlaceholder(),
),
);
}
} else if (image.startsWith('memory')) {
// Memory image
final split = image.split(',');
if (split.length == 2) {
final base64String = split[1];
final Uint8List bytes = base64.decode(base64String);
return applyShape(
Image.memory(
bytes,
width: width,
height: height,
fit: fit,
errorBuilder: (context, error, stackTrace) => buildErrorPlaceholder(),
),
);
} else {
// Invalid memory image URL
return buildErrorPlaceholder();
}
} else {
return applyShape(
Image.file(
File(image),
fit: fit,
width: width,
height: height,
color: color,
errorBuilder: (context, error, stackTrace) => buildErrorPlaceholder(),
),
);
}
}
}
Subscribe to my newsletter
Read articles from Meet Dabhi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
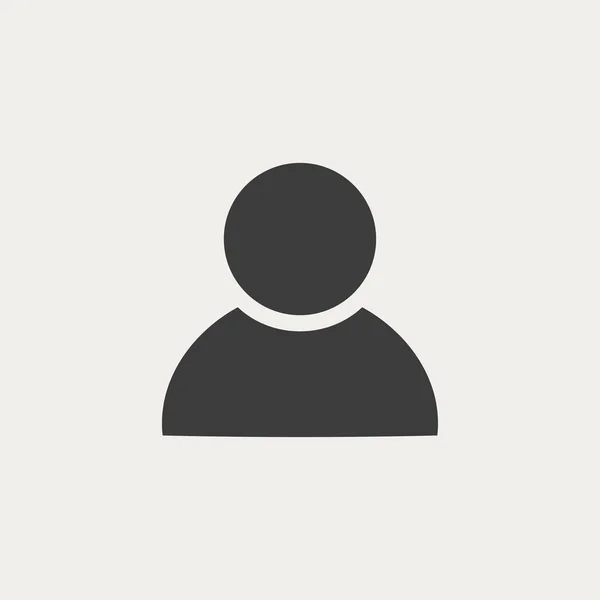
Meet Dabhi
Meet Dabhi
Welcome to Flutter Hub! I'm Meet Dabhi, a Flutter developer with 3+ years of experience in creating beautiful and functional mobile applications (Android, IOS, Web, MacOS, etc.) My journey with Flutter began in 3+, and since then, I've been dedicated to mastering this powerful framework. I created Flutter Hub to share my knowledge, insights, and the latest trends in the Flutter community. Through this blog, I aim to provide valuable resources for both beginners and seasoned developers, helping them navigate the ever-evolving world of Flutter development.