Using DOMPurify in Nuxt 3 for Secure HTML Sanitization
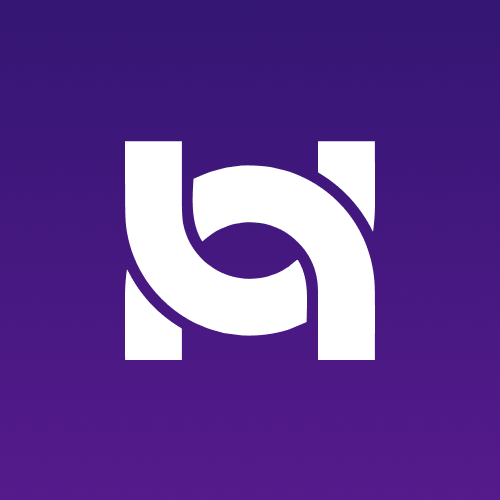
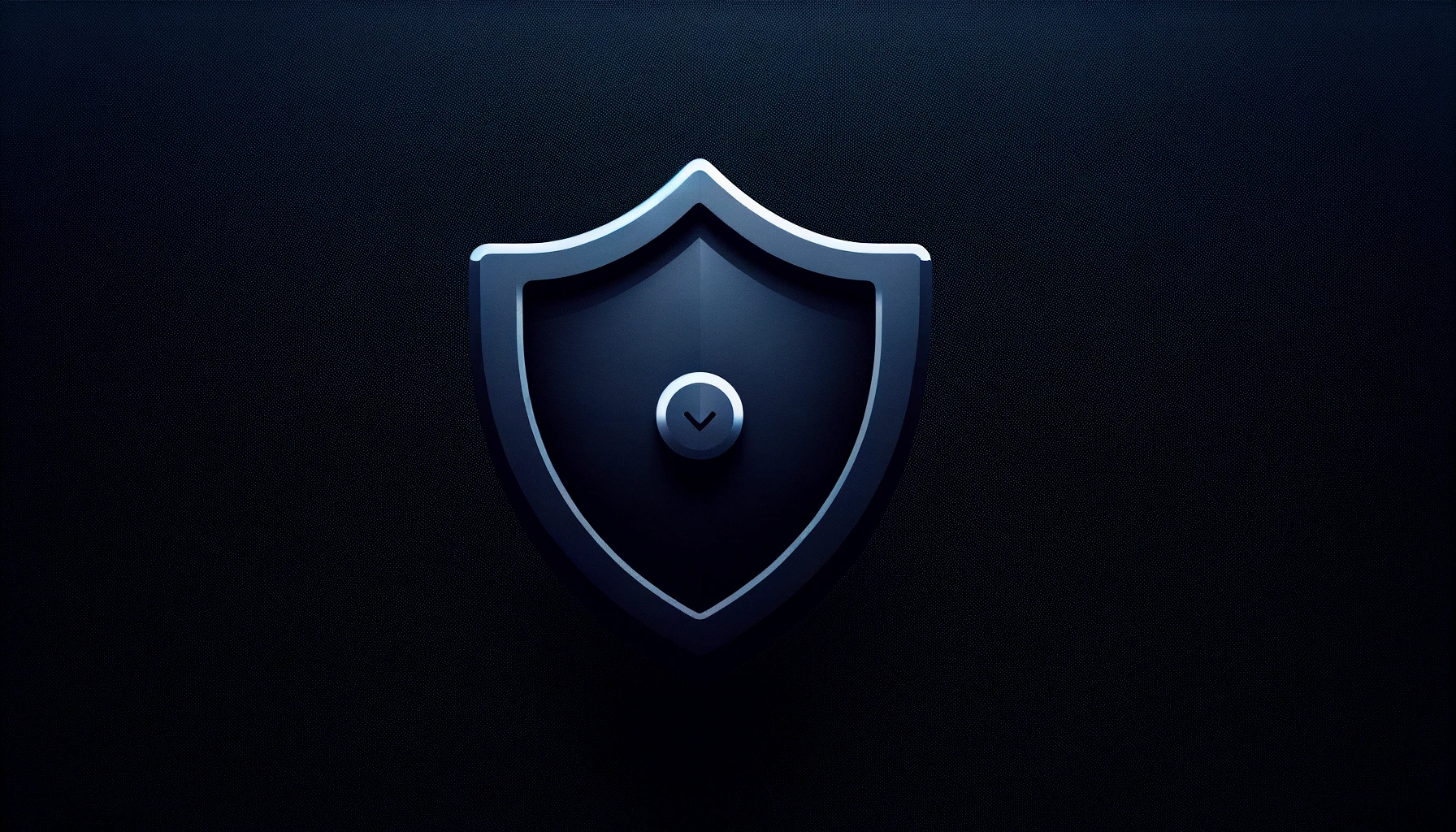
Security is everything when it comes to building web applications. XSS attacks can ruin your day, but thankfully, we have DOMPurify to sanitize HTML content. Let's see how to get DOMPurify up and running in a Nuxt 3 application!
Installation
Let's start with the installation. We'll use pnpm here, but npm or yarn work just as well.
pnpm add dompurify jsdom
pnpm add -D @types/dompurify @types/jsdom
Creating the DOMPurify Plugin
Create a new plugin file /plugins/dompurify.js
and add the following code:
import DOMPurify from 'dompurify';
export default defineNuxtPlugin(async (nuxtApp) => {
let sanitize;
if (process.server) {
const { JSDOM } = await import('jsdom');
sanitize = DOMPurify(new JSDOM('').window).sanitize;
} else {
sanitize = DOMPurify.sanitize;
}
nuxtApp.provide('sanitizeHTML', sanitize);
});
Here's what's happening:
import DOMPurify: Imports the DOMPurify library.
defineNuxtPlugin: Defines a new Nuxt plugin.
if (process.server): On the server-side, uses the jsdom library to create a window object.
else: On the client-side, uses the regular DOMPurify interface.
nuxtApp.provide('sanitizeHTML', ...): Provides the sanitize function for use throughout the Nuxt application.
Using DOMPurify
Once the plugin is created and registered, we can use the sanitize function anywhere in the application.
Example 1: Using in script
<script setup>
const { $sanitizeHTML } = useNuxtApp();
const rawContent = "<p>Some potentially <strong onClick=\"alert('Hack!')\">dangerous</strong> HTML content</p>";
const sanitizedContent = $sanitizeHTML(rawContent);
</script>
Example 2: Using in template
<script setup>
const rawContent = "<p>Some potentially <strong onClick=\"alert('Hack!')\">dangerous</strong> HTML content</p>";
</script>
<template>
<div v-html="$sanitizeHTML(rawContent)"></div>
</template>
Summary
DOMPurify is like a bodyguard for your HTML content, keeping harmful scripts at bay. By integrating DOMPurify into your Nuxt 3 application and using the $sanitizeHTML
function, you ensure your app is better protected against XSS attacks. I hope this blog post helps you get DOMPurify up and running in your own project!
If you have any questions or need further assistance, feel free to leave a comment below. Happy coding!
Subscribe to my newsletter
Read articles from Rane Hyper directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
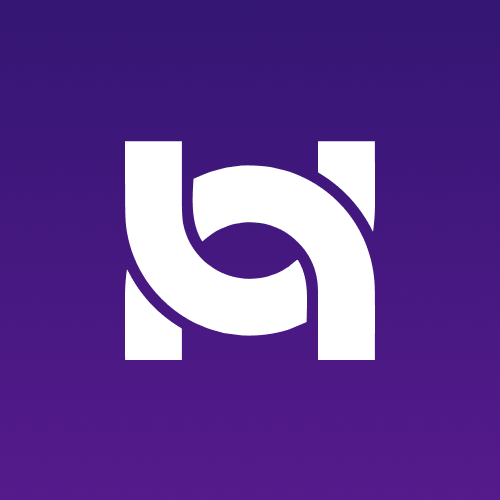
Rane Hyper
Rane Hyper
Founder of Hyperlink