Dynamic Routes in Next.js

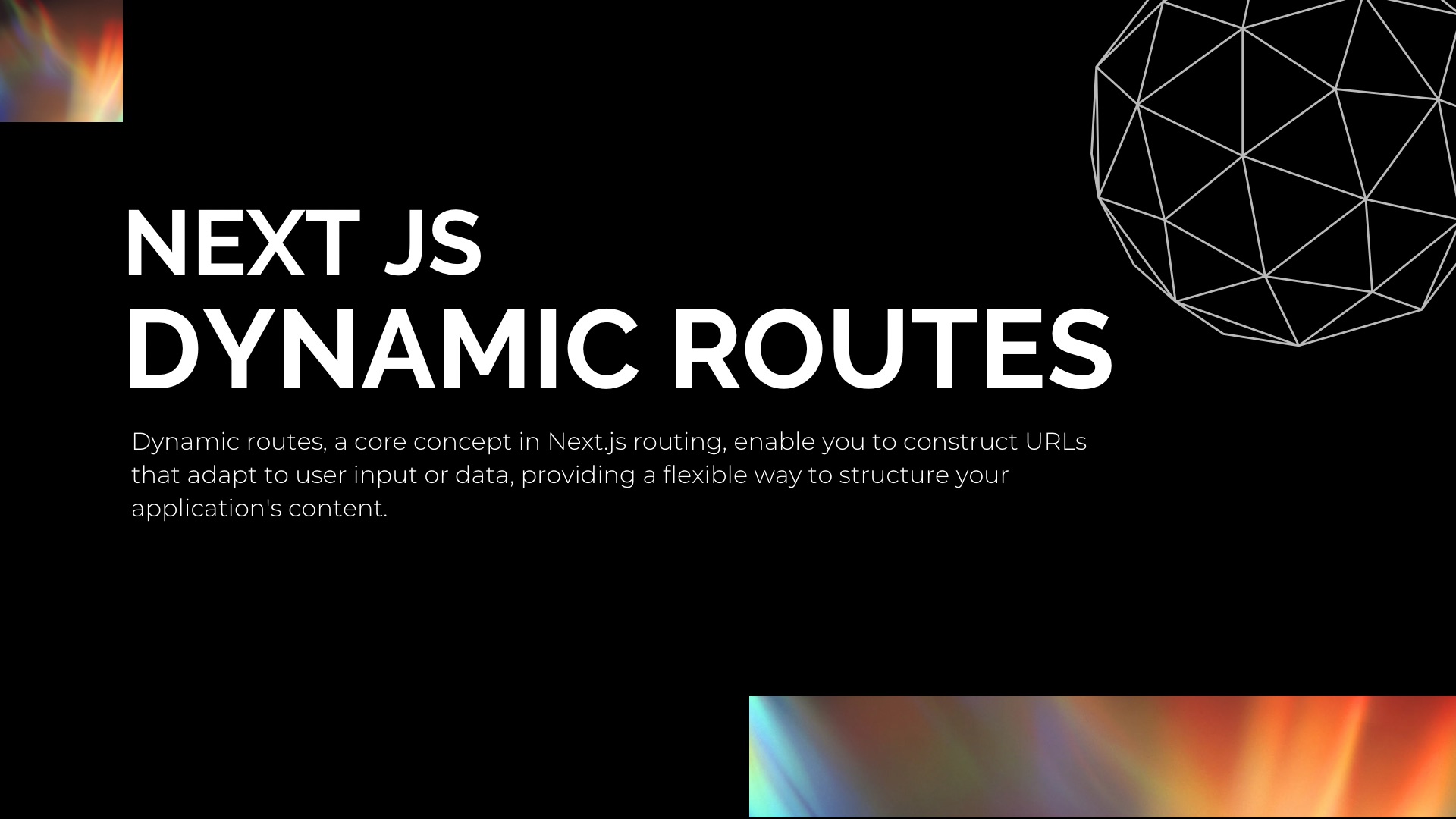
Introduction
Next.js, a popular React framework, offers a robust routing system that empowers you to create dynamic and user-friendly experiences within your web applications. Dynamic routes, a core concept in Next.js routing, enable you to construct URLs that adapt to user input or data, providing a flexible way to structure your application's content.
Understanding Dynamic Routes
In contrast to static routes (like /about
), dynamic routes employ placeholders within the URL structure. These placeholders, enclosed in square brackets []
, represent segments that can vary based on user interaction or data. For instance, a dynamic route for product pages might look like this:
/products/[productId]
Here, [productId]
is the dynamic segment, which can take on different values (e.g., /products/123
, /products/456
) to display distinct product details.
Benefits of Dynamic Routes
Scalability: Dynamic routes enable you to efficiently manage data-driven content. With a single route pattern, you can handle numerous items or resources without creating individual static routes.
Flexibility: They provide a dynamic URL structure that can seamlessly adapt to changing data or user interactions.
Improved User Experience: Dynamic routes often translate to cleaner and more intuitive URLs for users,enhancing website navigation.
Creating Dynamic Routes in Next.js
Next.js leverages a file-based routing system, where the structure of your project's pages
directory directly maps to the application's URL structure. To create a dynamic route:
Create a Folder with Square Brackets: Within your
pages
directory, create a folder named with square brackets surrounding the dynamic segment name. For example,pages/products/[productId].js
.Component File (Optional): Inside the folder, you can create a component file (e.g.,
products/[productId].js
) that will render the content for this dynamic route.
Accessing Dynamic Route Parameters
Within your component file, you can access the dynamic segment values using the useRouter
hook provided by Next.js:
JavaScript
import { useRouter } from 'next/router';
function ProductDetails() {
const router = useRouter();
const { productId } = router.query;
// Use productId to fetch product data or render content
}
The router.query
object contains key-value pairs representing the dynamic segments and their corresponding values. In this example, productId
can be used to fetch product information from an API or database, or to dynamically render the product details within the component.
Data Fetching with Dynamic Routes
Next.js offers two primary approaches for fetching data within dynamic routes:
getStaticProps: This function is executed during the build process, enabling pre-rendering of your dynamic pages with static data. It's ideal for content that doesn't change frequently (e.g., blog posts).
getServerSideProps: This function runs on the server for each request, allowing you to fetch the latest data before rendering the page for the user. It's suitable for content that requires real-time updates (e.g., product listings with dynamic pricing).
Choosing the Right Data Fetching Strategy
The choice between getStaticProps
and getServerSideProps
depends on your specific use case:
Use
getStaticProps
when you want:Improved SEO as pre-rendered pages are readily indexed by search engines.
Faster initial load times, as pages are already prepared at build time.
Use
getServerSideProps
when you need:The most up-to-date data for each request, ensuring users see the latest information.
User-specific content, such as personalized recommendations.
Further Considerations for Dynamic Routes
Optional Catch-All Routes: You can use
pages/[...slug].js
to capture any unmatched URL segments as an array inrouter.query.slug
.Dynamic Route Validation: Implement checks to ensure valid data is passed within the dynamic segments,preventing errors or unexpected behavior
Hope this will give you a brief understanding on Dynamic routes
Subscribe to my newsletter
Read articles from Narayana M V L directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Narayana M V L
Narayana M V L
I'm Narayana, a full-stack developer who navigates the intricate landscape of code with equal parts passion and precision. By day, I weave the magic of front-end development, painting pixel-perfect interfaces and bringing static pages to life. By night, I delve into the back-end's hidden depths, crafting the logic and infrastructure that makes it all tick. But for me, code is more than just lines and loops - it's a language of endless possibilities. That's why I don't just write code, I explore its frontiers. I delve into the newest tech trends, dissect them with a developer's eye, and then share my insights with my fellow adventurers in the code canyon. My writing is where I bridge the gap between the intricate machinery of development and the human language of understanding. I craft articles, tutorials, and even the occasional code haiku, demystifying complex concepts and igniting sparks of inspiration in developer minds. My target audience? You, the curious coder, the fearless explorer, the one who sees the beauty in both the user's click and the server's hum. I want to be your guide on this journey, a fellow traveler who can illuminate the path ahead, share the thrill of a new discovery, and maybe even help you debug that particularly nasty bug. So, whether you're a seasoned veteran or a wide-eyed newbie, welcome to my corner of the web. Grab a cup of your favorite caffeinated beverage, pull up a keyboard, and let's talk code, explore the frontiers, and build something amazing together. Beyond this core, you can personalize your bio further by: Adding specific technologies you're proficient in (e.g., React, Node.js) Mentioning any notable projects or accomplishments Sharing your writing platform or website Injecting your unique voice and personality Remember, your bio is a chance to make a first impression, so let it shine with your passion for code and your desire to connect with the developer community.