Building a REST API with Flask: A Step-by-Step Guide
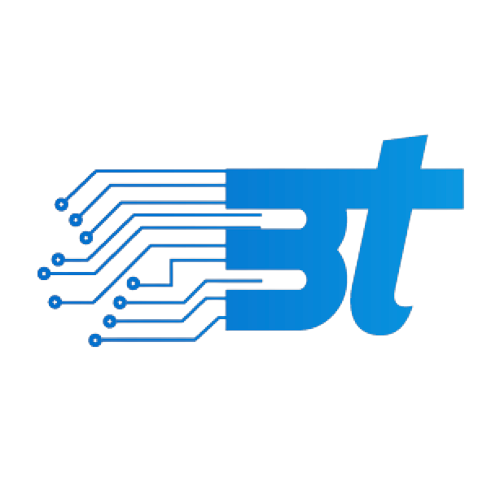
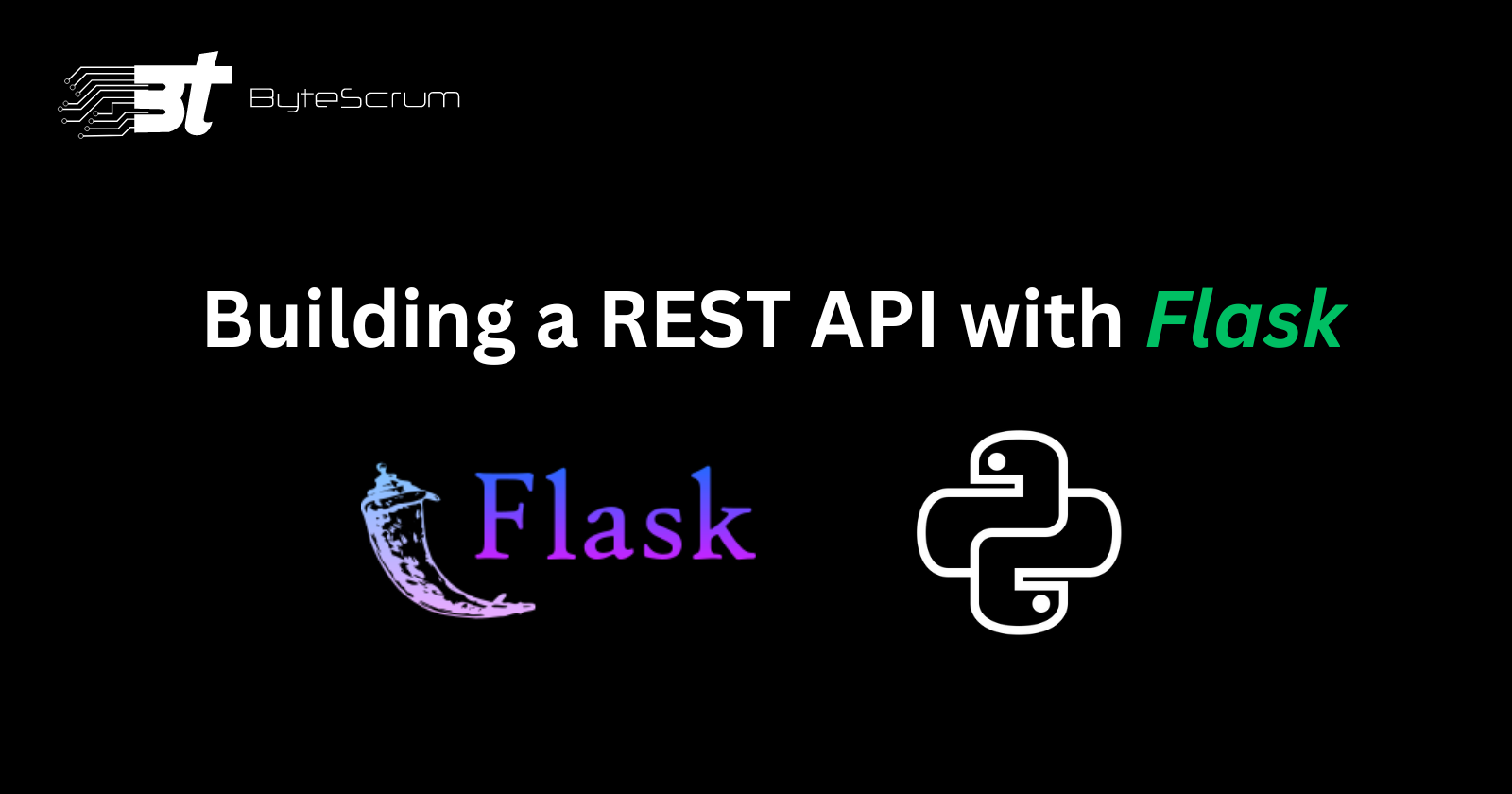
Introduction
Flask is a lightweight and flexible Python web framework that makes it easy to build web applications, including REST APIs. In this guide, we'll walk through the process of creating a REST API using Flask, covering everything from setting up the project to implementing endpoints and testing the API.
1. Setting Up Your Environment
Before we start building the API, we need to set our development environment. This involves installing Flask and creating a virtual environment to manage our project dependencies.
1.1. Install Flask:
First, ensure you have Python installed. Then, you can install Flask using pip:
pip install Flask
1.2. Create a Virtual Environment:
Create a virtual environment to isolate your project dependencies:
python -m venv venv
Activate the virtual environment:
- On Windows:
venv\Scripts\activate
- On macOS and Linux:
source venv/bin/activate
2. Creating a Basic Flask Application
2.1. Project Structure:
Create a new directory for your project and set up the following structure:
my_flask_api/
├── app.py
├── requirements.txt
└── venv/
2.2. Basic Flask Application:
In app.py
, write the basic setup for a Flask application:
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/')
def home():
return jsonify({'message': 'Welcome to my API!'})
if __name__ == '__main__':
app.run(debug=True)
Run the application:
python app.py
Open your browser and navigate to http://127.0.0.1:5000/
to see the welcome message.
3. Building RESTful Endpoints
3.1. Define the API Structure:
For this example, we'll build a simple API to manage a collection of books. Each book will have an ID, title, author, and publication date.
3.2. Create the Data Model:
For simplicity, we'll use an in-memory list to store our books. In a real-world application, you would use a database.
books = [
{'id': 1, 'title': '1984', 'author': 'George Orwell', 'published': '1949-06-08'},
{'id': 2, 'title': 'To Kill a Mockingbird', 'author': 'Harper Lee', 'published': '1960-07-11'}
]
3.3. Implement CRUD Operations:
Create (POST):
@app.route('/books', methods=['POST'])
def add_book():
new_book = request.get_json()
books.append(new_book)
return jsonify(new_book), 201
Read (GET):
Get all books:
@app.route('/books', methods=['GET'])
def get_books():
return jsonify(books)
Get a book by ID:
@app.route('/books/<int:book_id>', methods=['GET'])
def get_book(book_id):
book = next((book for book in books if book['id'] == book_id), None)
if book:
return jsonify(book)
else:
return jsonify({'error': 'Book not found'}), 404
Update (PUT):
@app.route('/books/<int:book_id>', methods=['PUT'])
def update_book(book_id):
book = next((book for book in books if book['id'] == book_id), None)
if book:
data = request.get_json()
book.update(data)
return jsonify(book)
else:
return jsonify({'error': 'Book not found'}), 404
Delete (DELETE):
@app.route('/books/<int:book_id>', methods=['DELETE'])
def delete_book(book_id):
global books
books = [book for book in books if book['id'] != book_id]
return jsonify({'message': 'Book deleted'})
4. Testing the API
4.1. Manual Testing with Postman or cURL:
You can use tools like Postman or cURL to manually test the API endpoints. For example, to get all books:
curl http://127.0.0.1:5000/books
4.2. Automated Testing with Unit Tests:
Create a new file test_
app.py
to write unit tests for your API using the unittest
module:
import unittest
from app import app
class FlaskTestCase(unittest.TestCase):
def setUp(self):
self.app = app.test_client()
self.app.testing = True
def test_home(self):
result = self.app.get('/')
self.assertEqual(result.status_code, 200)
self.assertIn('Welcome to my API!', result.get_data(as_text=True))
def test_get_books(self):
result = self.app.get('/books')
self.assertEqual(result.status_code, 200)
self.assertIn('1984', result.get_data(as_text=True))
# Additional tests for POST, PUT, DELETE...
if __name__ == '__main__':
unittest.main()
Run the tests:
python test_app.py
Conclusion
Happy Coding!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
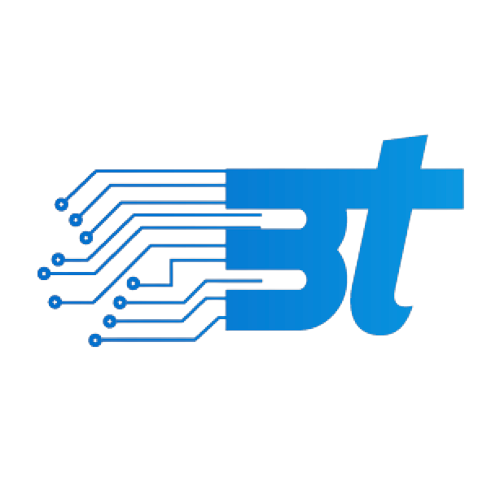
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.