Project Architecture Diagram

Table of contents
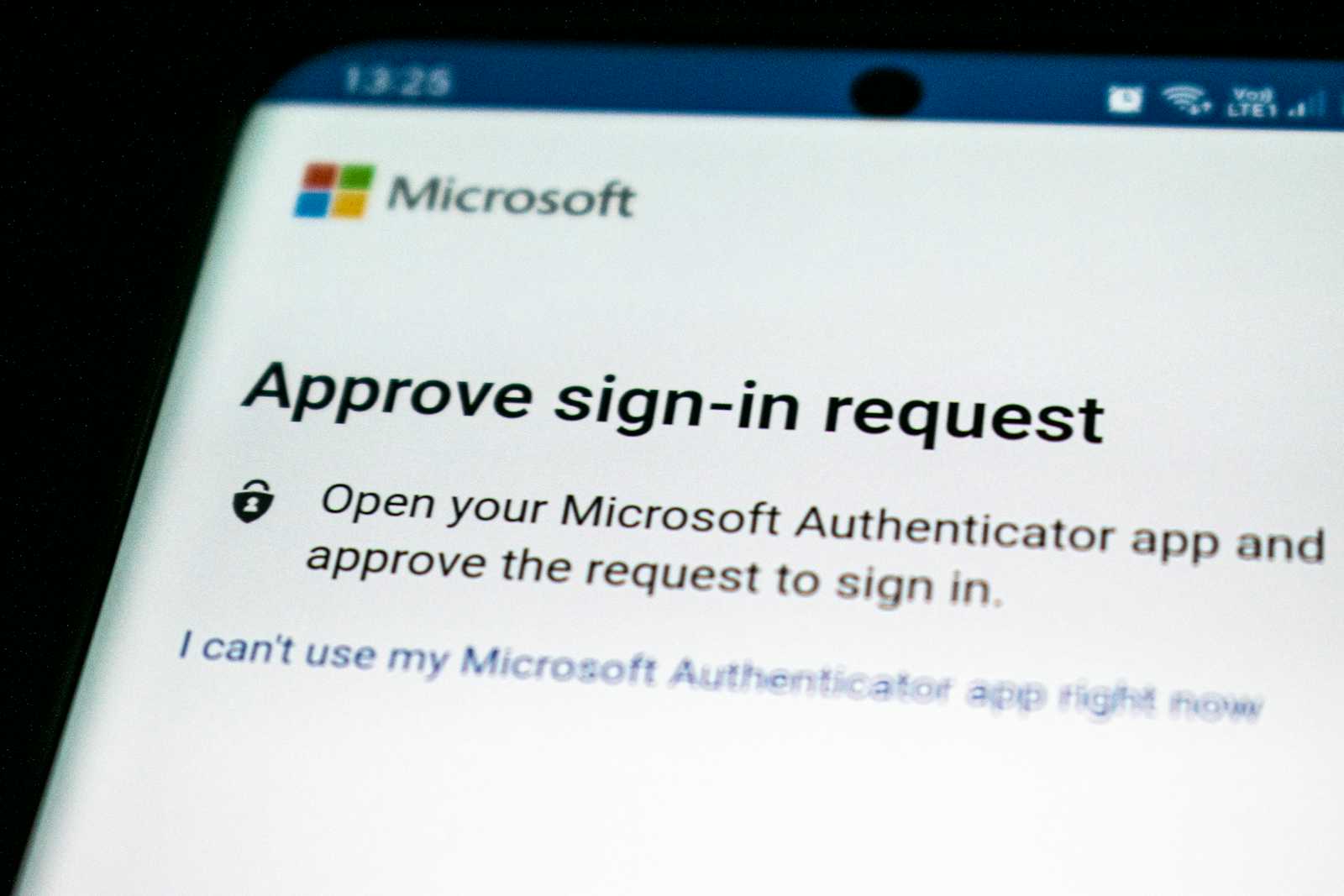
(Angular 17, ASP.NET 8, SQL Server 2022, and JWT authentication)
High-Level Project Architecture Diagram
+--------------------+
| Client (UI) |
| (Angular 17 App) |
+---------+----------+
|
v
+-----------------------+
| API Gateway |
| (ASP.NET 8) |
+----------+------------+
|
v
+------------------------------------------------+
| Backend |
| +-----------------+ +------------------+ |
| | Authentication |<----->| Business Logic | |
| | Service | | Services | |
| +-------+---------+ +---------+--------+ |
| | | |
| v v |
| +-----------------+ +------------------+ |
| | Token Service |<----->| Data Access | |
| | (JWT) | | Layer (DAL) | |
| +-------+---------+ +---------+--------+ |
| | | |
+------------------------------------------------+
|
v
+-----------------------+
| Database Layer |
| (SQL Server 2022) |
+-----------------------+
Explanation of the Architecture
Client (UI) - Angular 17 App:
Responsibility: Provides the user interface and handles user interactions.
Components: Angular components, services, and modules.
Communication: Makes HTTP requests to the API Gateway.
API Gateway - ASP.NET 8:
Responsibility: Acts as an entry point for all client requests.
Components: Controllers, middleware for authentication and authorization.
Function: Validates and routes requests to appropriate backend services.
Backend Services:
Authentication Service:
Manages user authentication.
Issues JWT tokens upon successful login.
Token Service (JWT):
- Generates and validates JWT tokens.
Business Logic Services:
Encapsulates the core business logic.
Ensures separation of concerns.
Data Access Layer (DAL):
Handles database operations.
Uses repositories and unit of work patterns to interact with SQL Server 2022.
Database Layer - SQL Server 2022:
Responsibility: Stores and retrieves data.
Components: Tables, stored procedures, views, etc.
Communication: Interacts with the Data Access Layer to perform CRUD operations.
JWT Authentication Flow
User Login:
The client sends login credentials to the API Gateway.
The API Gateway forwards the request to the Authentication Service.
The Authentication Service validates the credentials and issues a JWT token if valid.
The JWT token is returned to the client.
Authenticated Requests:
The client includes the JWT token in the Authorization header of subsequent requests.
The API Gateway validates the token via the Token Service.
If valid, the request is forwarded to the appropriate backend service.
The backend service processes the request and interacts with the Data Access Layer if needed.
The response is sent back through the API Gateway to the client.
Clean Architecture Principles Applied
Separation of Concerns: Different layers (UI, API, Business Logic, DAL) handle distinct responsibilities.
Dependency Inversion: Higher-level modules (business logic) do not depend on lower-level modules (data access) but on abstractions.
Testability: Each layer can be tested independently, enhancing maintainability and scalability.
Modularity: Clear separation of modules allows for independent development and scaling of different parts of the application.
This architecture ensures a scalable, maintainable, and secure web application that leverages the strengths of Angular, ASP.NET, and SQL Server while adhering to clean architecture principles.
****************************************************************************
Detailed project architecture tree for an application using Angular 17, ASP.NET 8, SQL Server 2022, and JWT authentication, following clean architecture principles.
Project Architecture Tree
ProjectRoot/
│
├── Client/
│ ├── src/
│ │ ├── app/
│ │ │ ├── components/
│ │ │ ├── services/
│ │ │ ├── modules/
│ │ │ └── app.module.ts
│ │ ├── assets/
│ │ ├── environments/
│ │ └── main.ts
│ └── angular.json
│
├── Server/
│ ├── ApiGateway/
│ │ ├── Controllers/
│ │ ├── Middleware/
│ │ └── Program.cs
│ │
│ ├── Services/
│ │ ├── AuthenticationService/
│ │ │ ├── Interfaces/
│ │ │ ├── Models/
│ │ │ ├── Services/
│ │ │ └── AuthenticationService.cs
│ │ │
│ │ ├── TokenService/
│ │ │ ├── Interfaces/
│ │ │ ├── Models/
│ │ │ ├── Services/
│ │ │ └── TokenService.cs
│ │ │
│ │ ├── BusinessLogicService/
│ │ │ ├── Interfaces/
│ │ │ ├── Models/
│ │ │ ├── Services/
│ │ │ └── BusinessLogicService.cs
│ │ │
│ │ └── Shared/
│ │ ├── Utilities/
│ │ └── Constants/
│ │
│ ├── DataAccess/
│ │ ├── Repositories/
│ │ │ ├── Interfaces/
│ │ │ ├── Implementations/
│ │ │ └── Repository.cs
│ │ │
│ │ ├── UnitOfWork/
│ │ │ ├── Interfaces/
│ │ │ └── UnitOfWork.cs
│ │ │
│ │ └── DbContext/
│ │ └── ApplicationDbContext.cs
│ │
│ └── Core/
│ ├── Entities/
│ ├── ValueObjects/
│ ├── DTOs/
│ └── Exceptions/
│
└── Database/
├── Migrations/
├── SeedData/
└── Scripts/
Explanation of the Structure
Client (Angular 17):
src/app: Contains Angular components, services, and modules.
components: UI components of the application.
services: Angular services for business logic and API calls.
modules: Angular modules for organizing components and services.
assets: Static files like images, stylesheets, etc.
environments: Configuration files for different environments (development, production).
main.ts: Entry point for the Angular application.
angular.json: Angular project configuration file.
Server (ASP.NET 8):
ApiGateway: Handles incoming HTTP requests, routes them to appropriate services, and includes middleware.
Controllers: API controllers for handling client requests.
Middleware: Middleware components for request processing (e.g., authentication).
Services: Contains various service layers.
AuthenticationService: Handles user authentication logic.
Interfaces: Interface definitions for dependency injection.
Models: Data models related to authentication.
Services: Service implementations.
TokenService: Manages JWT token generation and validation.
BusinessLogicService: Contains the core business logic.
Shared: Common utilities and constants used across services.
DataAccess: Handles interactions with the database.
Repositories: Repository pattern implementations.
Interfaces: Interface definitions for repositories.
Implementations: Concrete implementations of repository interfaces.
UnitOfWork: Manages transactions.
Interfaces: Interface definitions for Unit of Work.
UnitOfWork.cs: Implementation of the Unit of Work pattern.
DbContext: Entity Framework DbContext for managing database connections and entities.
Core: Core entities, value objects, DTOs (Data Transfer Objects), and custom exceptions.
Entities: Business entities.
ValueObjects: Value objects that represent domain concepts.
DTOs: Data Transfer Objects for passing data between layers.
Exceptions: Custom exceptions for error handling.
Database (SQL Server 2022):
Migrations: Entity Framework migration files for managing database schema changes.
SeedData: Data seeding scripts for initializing the database.
Scripts: Additional SQL scripts for database setup and maintenance.
This architecture ensures a clean separation of concerns, maintainability, and scalability, following best practices of clean architecture and leveraging the strengths of Angular, ASP.NET, and SQL Server.
Explanation of the Structure with Commands
1. Client (Angular 17)
ng new client --routing --style css
cd client
ng generate module app-routing --flat --module=app
ng generate component example
ng generate service example
2. Server (ASP.NET 8)
dotnet new webapi -o ApiGateway
cd ApiGateway
dotnet new classlib -o ../Services/AuthenticationService
dotnet new classlib -o ../Services/TokenService
dotnet new classlib -o ../Services/BusinessLogicService
dotnet new classlib -o ../DataAccess
dotnet new classlib -o ../Core
dotnet sln add ApiGateway/ApiGateway.csproj
dotnet sln add Services/AuthenticationService/AuthenticationService.csproj
dotnet sln add Services/TokenService/TokenService.csproj
dotnet sln add Services/BusinessLogicService/BusinessLogicService.csproj
dotnet sln add DataAccess/DataAccess.csproj
dotnet sln add Core/Core.csproj
3. Database (SQL Server 2022)
dotnet new classlib -o Database
cd Database
mkdir Migrations SeedData Scripts
This structure provides a comprehensive and organized architecture for the application, following clean architecture principles and ensuring a clear separation of concerns.
Subscribe to my newsletter
Read articles from Saruf Ratul directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Saruf Ratul
Saruf Ratul
A Senior Software Engineer with five years of professional experience, specializing in fullstack development, Angular, Asp.Net, MySQL, Oracle, and AWS. A proven track record of managing large scale software engineering projects to support cloud deployments and integrations.