What does serializeUser & deserializeUser do?
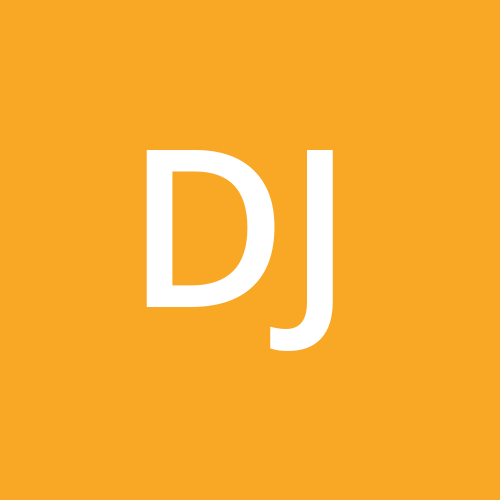
Looking at these methods in a simple and straightforward way, you can simply say that serializeUser stores the identifying data for the logged-in user inside the passport variable. Then deserializeUser uses that information to populate the request variable (usually req.user) with the user information that it pulls from the database or where ever else you stored it.
passport.serializeUser((user, done) => {
done(null, user._id)
})
passport.deserializeUser(function(id, done) {
User.findById(id).then(function(user){
done(null, user);
}).catch(function(err){
done(err, null);
})
});
These methods are basically in charge of maintaining the session data of the user for your application.
Serialization and deserialization are essentially about coding information in a way that it can be efficiently stored in a computer system and later retrieved.
The way this can be achieved is by using an id, that way you don't need to pass around all the data for an item/user. You just use an identifier and when you need specific data that identifier can be used to fetch the required information.
Knowing this permits us to understand how Passport handles the user's session data. You know that serialize needs to store the id for your user information. While deserialize needs to access the storage place of that information and pull the data that your application needs. You also know that the user id can be found in req.session.passport (most likely in a variable called user) and the user data can be found at req.user.
So if your application needs to tell whether a user is an administrator or a regular user then you can include that information in the data that deserialize uses.
If you are saving the user information from Google or Microsoft and will be using your own identifier and not the id provided by these companies then you need to pass that variable to serialize. Which may mean you will need an extra lookup if your pages uses those other companies for authentication.
References:
https://stackoverflow.com/questions/27637609/understanding-passport-serialize-deserialize https://stackoverflow.com/questions/16047570/passport-js-store-information-in-session
Subscribe to my newsletter
Read articles from Dianne De Jesus directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
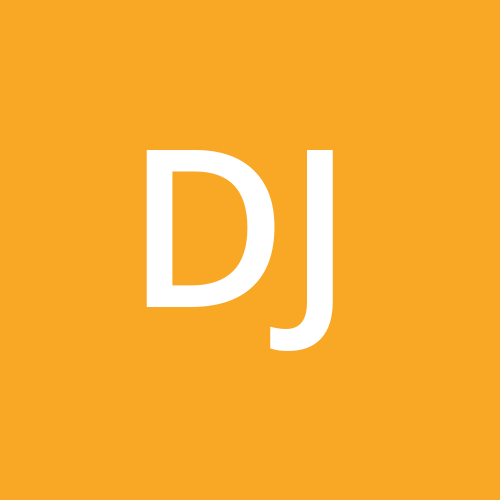