String Interpolation: What's That All About?
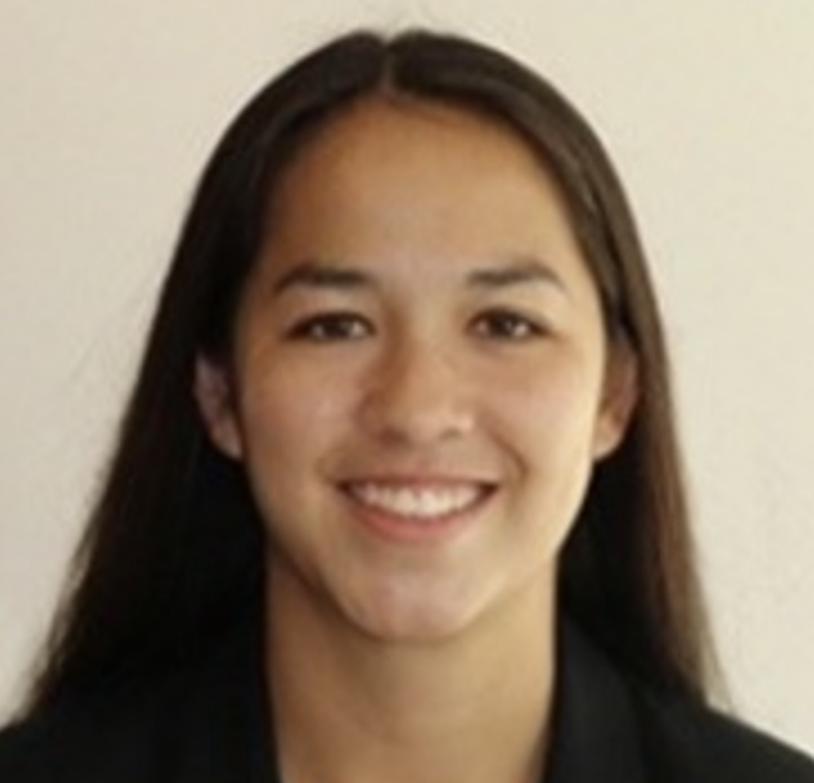
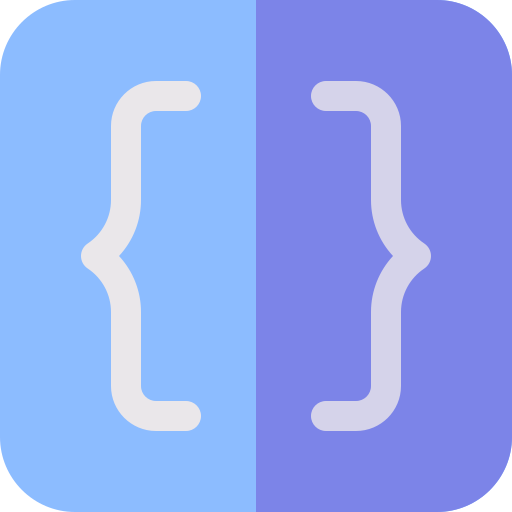
Today, we are going to discuss something commonly referred to in programming as string interpolation. Have you ever heard the word interpolate used in conversation? This usually refers to the addition of some sort of information or, more generally, dialogue, into a conversation. In programming, we use a similar phrase when we are working with strings and want to embed some sort of values or expressions into that string. For our purposes, we are going to be looking at code examples from JavaScript, but it is important to note that this concept is used widely across many programming languages.
Now, why is this even important? To answer this, let's consider a simple example. Perhaps we are developing a function to greet visitors at a zoo. This function at its most basic level might look something like this:
function greetVisitors(){
return 'Welcome to the zoo, James!'
}
console.log(greetVisitors()) // LOG: Welcome to the zoo, James!
We have successfully greeted a single visitor named James. That's great! Our next step is to make this function more dynamic by abstracting a visitor variable as a parameter for our function. As you might already have predicted, this also means that we must adjust our string to incorporate this variable, resulting in a function that looks something like this:
function greetVisitors(visitor){
return 'Welcome to the zoo, ' + visitor + '!'
}
console.log(greetVisitors('James')) // LOG: Welcome to the zoo, James!
console.log(greetVisitors('Kat')) // LOG: Welcome to the zoo, Kat!
When we look at this return statement, though it does function properly, there is a lot of code when we use the "+" operator and certainly room for error. Just imagine if we were working with a function that had multiple parameters and required us to include 5, 6, 7, or more "+" signs! That certainly would be a challenge to read and prone to error. If only we had a solution...
This is where string interpolation comes in! String interpolation is a way to dynamically embed values or expressions into a string. In this way, when we pass different values into those variables or adjust the expressions, this is reflected dynamically into our string output. As developers, we are always looking for ways to make our code more robust, efficient, and easy to follow, and string interpolation helps with all of these things.
Let's return to our example above and incorporate string interpolation. What we have done initially is employ something called concatenation, whereby we use the "+" operator to join two or more strings together. As a substitute for concatenation, we can instead perform string interpolation using backtick characters and curly braces (we refer to these as template literals) in order to achieve the same result:
function greetVisitors(visitor){
return `Welcome to the zoo, ${visitor}!`
}
console.log(greetVisitors('James')) // LOG: Welcome to the zoo, James!
console.log(greetVisitors('Kat')) // LOG: Welcome to the zoo, Kat!
You can see that our output is identical. Now at this point, you might be thinking, "Okay, that's nice, but is it really that helpful? We only saved a few keystrokes..." While you would be correct in the scenario we've shown above, just think about the various ways we could expand this concept of interpolation. Perhaps we have data on animals at the zoo that we'd like to incorporate into our strings:
const landAnimals = 15
const waterAnimals = 20
function announceAnimals(){
return `There are ${landAnimals + waterAnimals} animals to explore at our zoo!`
}
console.log(announceAnimals())
// LOG: There are 35 animals to explore at our zoo!
Here, we have included an expression within our template literals to help us display a single value representing the total number of animals at the zoo. There is no need to create a new variable and pass it through to our string, which is especially useful for one-off calculations. Taking this one step further, we can even call functions within our template literals:
const landAnimals = 15
const waterAnimals = 20
function announceAnimals(){
return `There are ${landAnimals + waterAnimals} animals to explore at our zoo!`
}
function greetVisitors(visitor){
return `Welcome to the zoo, ${visitor}! ${announceAnimals()}`
}
console.log(greetVisitors('James'))
// LOG: Welcome to the zoo, James! There are 35 animals to explore at our zoo!
Pretty cool, right?! There are many benefits of using string interpolation, the most significant of which is that it makes our code more concise and readable. Like concatenation, string interpolation allows for dynamic string construction. However, as we have seen, concatenation can become quite complicated and difficult to read as you stack more and more "+" operators on top of one another. Performing string interpolation with template literals is a powerful tool that not only improves readability, but also improves your efficiency in debugging.
By now you should have a solid understanding of what string interpolation is, how it can benefit us, and how it is used in our code. Be sure to test out the code examples above and try creating some interpolated strings on your own!
Subscribe to my newsletter
Read articles from Taylor Ng directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
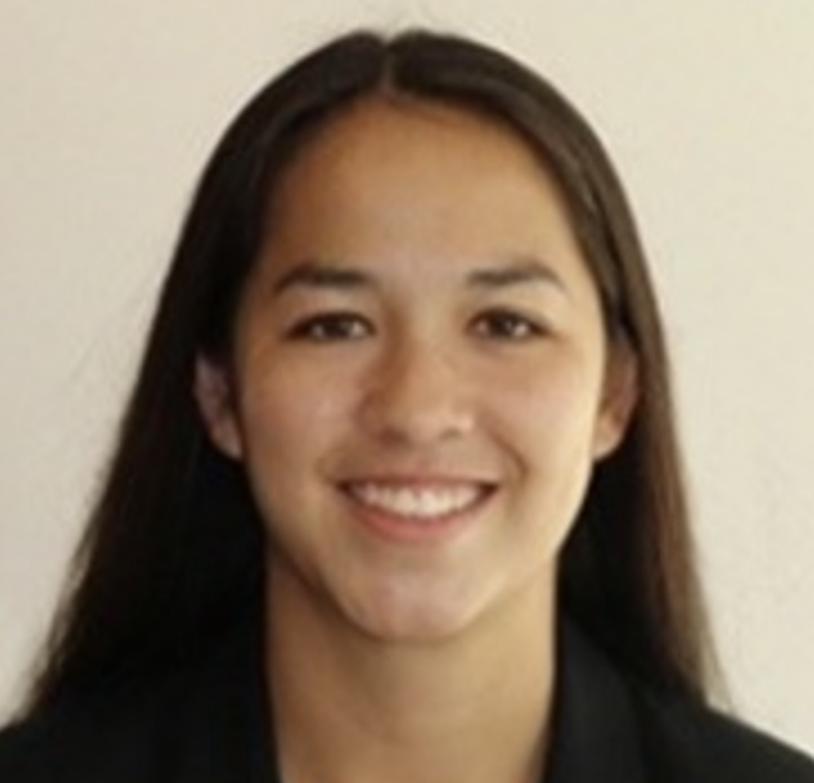