The Power of useState: Dynamic State Handling in React
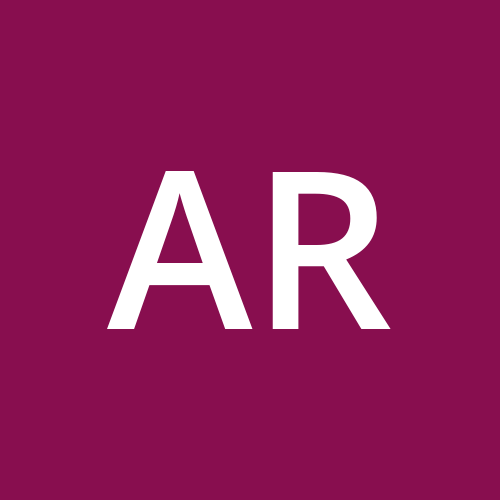
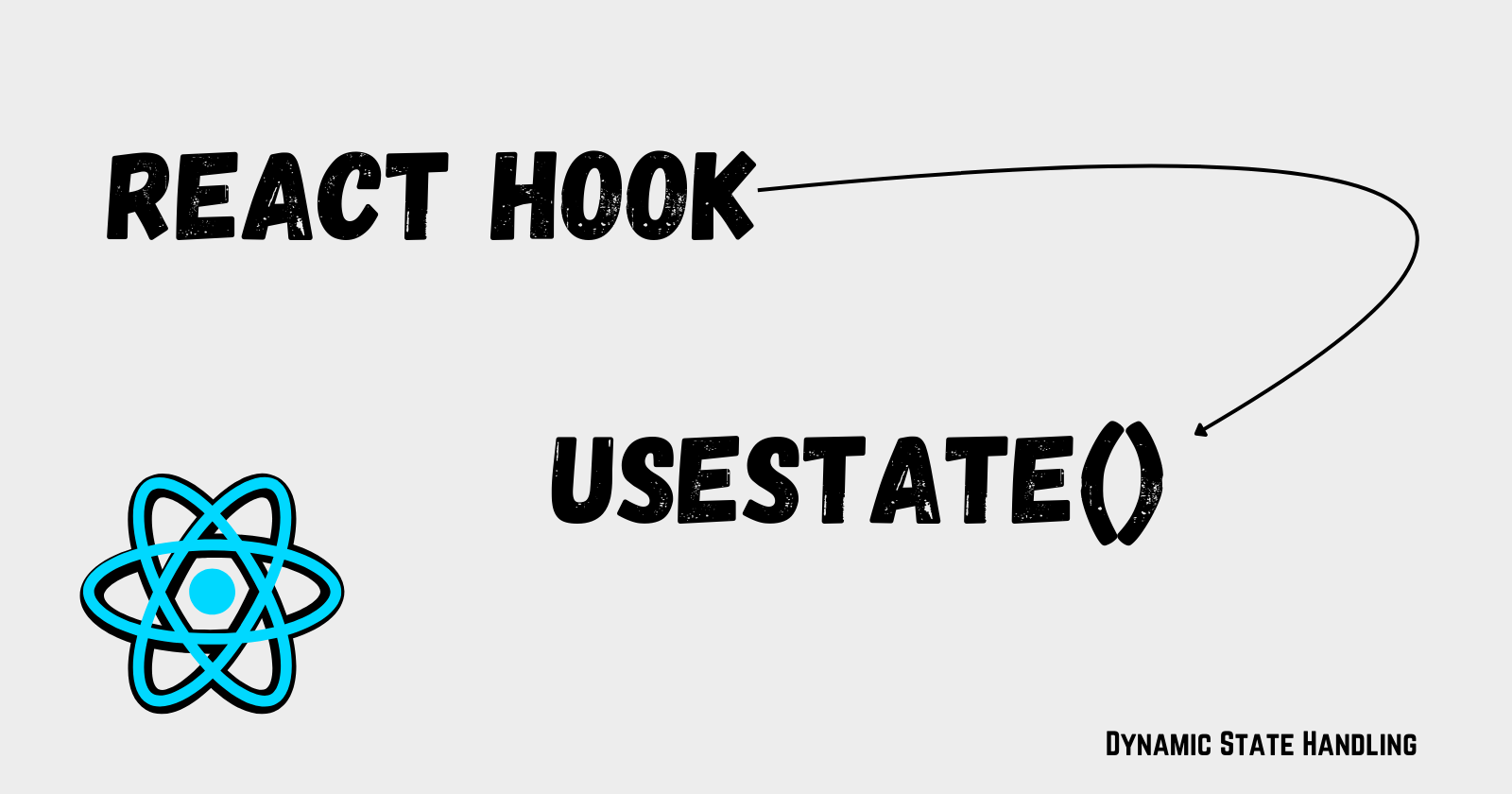
React has become a leading library for building user interfaces, largely due to its powerful state management capabilities. Managing state is essential for creating dynamic and interactive web applications. The introduction of hooks in React 16.8 significantly changed how developers handle state in functional components. Among the various hooks, the useState
hook is fundamental for adding state to functional components.
Understanding state in React
React state is a built-in object that allows components to store and manage dynamic data. It represents the component's local data that can change over time. Whenever it changes, the component re-renders. It plays a crucial role in how React components render and update the user interface (UI).
Unlike normal JavaScript variables, which don't automatically update the UI when their values change, state ensures that changes are reflected in the UI. This makes state essential for creating dynamic user interfaces. However, you can't directly modify the state value. Instead, React provides a tool called the useState
hook.
Dive into the useState Hook
Imagine you have a toy car that can change colors. You need a way to tell the car when to change its color and what color to change to. In React, a hook is like a special button you can press to tell your toy car to change its color. Functions starting with "use" are Hooks.
One of those hooks is useState() which lets you create and update state variables within functional components, enabling your UI to stay in sync with state changes. The useState hooks returns an array with two values, a state and a function to update it. You should never update the state directly. Always use the function when you need to update the state.
Let's see the most basic React example there is - the Counter:
import React, { useState } from 'react';
function Counter() {
const [number, setNumber] = useState(0);
function update() {
number = number + 1;
}
return (
<div>
<p>{number}</p>
<button onClick={update}>Increment</button>
</div>
);
}
export default Counter;
This is a simple component the goal of which is to update the number state when the user clicks on the Increment button. The update function is responsible for the update of the state. But what do you think will happen here? the state variable number
will increment as expected, but this change will not be reflected in the UI. Why this does not work?
Bypassing React's State Management: When you directly modify the state variable (
number = number + 1
), you are bypassing React's state management system. React doesn't know that the state has changed because you're not using the state setter function (setNumber
).No Re-render Triggered: React relies on the state setter function to know when to re-render a component. By directly modifying the state, React is not notified of any change, so it doesn't trigger a re-render. As a result, the UI will not be updated to reflect the new state.
State Out of Sync: The internal state managed by React (
number
) will remain unchanged, while your local variable (number
) might be updated. This causes the state and UI to go out of sync, leading to unexpected behavior.
To ensure that state changes are properly managed and the UI reflects these changes, you should use the state setter function provided by useState
.
function update(){
setNumber(num => num + 1);
}
The state setter function (setNumber
) provided by the useState
hook updates the state and notifies React of the change. This triggers a re-render of the component, ensuring the UI reflects the new state. We use it to keep the state and UI synchronized, avoiding direct state manipulation which React can't detect and won't cause a re-render.
Immutability in React
React enforces immutability for state updates for this very reason. When you call setNumber(number + 1)
, React does not modify the original number
directly. Instead, it creates a new state value and updates its internal state with this new value. This approach ensures predictable state changes and helps maintain a consistent UI.
If you’re interested in understanding more about immutability and why it is crucial in React, you can read my recent blog post on this topic here.
Understanding the Asynchronous Nature of useState
It's also important to remember that the useState
function works asynchronously. This means it doesn't update the state right away when you call it. Instead, React schedules the state update to happen during the next render cycle.
What Does Asynchronous State Update Mean?
When you call the state update function (e.g., setNumber
), React doesn’t immediately change the state and re-render the component. Instead, it marks the state for update and handles the actual update and re-rendering process in the background. This approach allows React to optimize performance by batching multiple state updates together and reducing the number of re-renders.
Consider the following scenario:
function Counter() {
const [number, setNumber] = useState(0);
function update() {
setNumber(number + 1);
console.log(number); // This will log the old state, not the updated one
}
return (
<div>
<p>{number}</p>
<button onClick={update}>Increment</button>
</div>
);
}
In this example, when you click the button, you might expect the console.log
statement to print the updated value of number
. However, because setNumber
is asynchronous, console.log(number)
will still log the old state value. The new state value will only be reflected in the next render cycle.
Why Does React Use Asynchronous State Updates?
Performance Optimization: React batches multiple state updates together to minimize the number of re-renders. This means if you call
setNumber
multiple times in quick succession, React may group these updates and process them in a single re-render. This improves performance by reducing the computational cost of updating the UI multiple times.Consistency: Asynchronous updates allow React to manage state changes in a predictable manner, ensuring that the state and UI remain consistent throughout the application.
Smooth User Experience: By handling updates efficiently, React ensures a smoother user experience, avoiding unnecessary UI refreshes and maintaining responsive interfaces.
Understanding the asynchronous nature of useState
helps developers write more predictable and efficient code. It’s crucial to be aware of this behavior to avoid confusion and ensure that state-dependent logic is implemented correctly.
Understanding Functional State Updates in React
When you use the functional form of the state setter, setNumber(number => number + 1)
, it may seem like the state updates "right away," but it actually works within the same asynchronous framework. Here's why it appears different:
Access to the Latest State
The functional form setNumber(number => number + 1)
provides the latest state value at the time the update function is executed. This ensures that each state update is based on the most current state, which is particularly useful in cases where multiple updates are made in quick succession.
Asynchronous Processing
Even with the functional form, the state update is still asynchronous. React schedules the state update and re-render for the next render cycle, but because the functional form correctly accesses the latest state, it can chain multiple updates correctly.
Example to Illustrate:
function Counter() {
const [number, setNumber] = useState(0);
function incrementTwice() {
setNumber(number + 1);
setNumber(number + 1); // Both calls use the initial number value
}
function incrementTwiceWithFunction() {
setNumber(number => number + 1);
setNumber(number => number + 1); // Each call uses the updated state value
}
return (
<div>
<p>{number}</p>
<button onClick={incrementTwice}>Increment Twice</button>
<button onClick={incrementTwiceWithFunction}>Increment Twice with Function</button>
</div>
);
}
incrementTwice
: This callssetNumber(number + 1)
twice in succession. SincesetNumber
is asynchronous, both calls use the initial value ofnumber
. Ifnumber
starts at0
, both calls will set it to1
, and you end up withnumber
being1
.incrementTwiceWithFunction
: This uses the functional form, so eachsetNumber
call gets the most recent state. The first call incrementsnumber
to1
, and the second call increments it to2
. Thus,number
correctly ends up being2
.
Avoiding Common Pitfalls
1. Direct State Mutation
Pitfall: Directly modifying the state variable, such as number = number + 1
, instead of using the state setter function.
Solution: Always use the state setter function provided by useState
to update the state. This ensures that React is aware of the state change and can trigger a re-render.
// Incorrect
function update() {
number = number + 1;
}
// Correct
function update() {
setNumber(number + 1);
}
2. Ignoring Immutability
Pitfall: Mutating state directly, which can lead to unpredictable behavior and difficult-to-debug issues.
Solution: Ensure that you create new state objects or arrays instead of mutating existing ones. Use the spread operator or Object.assign
for objects, and methods like concat
or the spread operator for arrays.
// Incorrect
const newItems = items;
newItems.push(newItem);
setItems(newItems);
// Correct
setItems([...items, newItem]);
3. Handling Asynchronous State Updates Correctly
Pitfall: Assuming state updates are synchronous and immediately reflected in the UI, or updating state based on the current state without using the functional form, which can cause stale state issues (refers to a scenario where a component is working with an outdated or incorrect version of the state).
Solution: Remember that state updates are asynchronous. Use the functional form of the state setter to ensure you always work with the most recent state, and be aware that the state will update in the next render cycle.
Consider a counter component:
import React, { useState } from 'react';
function Counter() {
const [number, setNumber] = useState(0);
function update() {
setNumber(prevNumber => prevNumber + 1);
console.log(number); // This will log the old state, not the updated one
}
return (
<div>
<p>{number}</p>
<button onClick={update}>Increment</button>
</div>
);
}
export default Counter;
4. Updating Specific Object Property
Pitfall: Modifying just a property of an object instead of creating a new object reference.
When updating a property of an object in state, it's important to create a new object. If you directly modify a property, it can lead to unexpected behavior.
import { useState } from "react";
export default function App() {
const [user, setUser] = useState({
name: "John",
age: 25,
});
// Incorrect way to update property of user state
const changeName = () => {
setUser((user) => (user.name = "Arthur")); // This overwrites the user object
};
return (
<div className="App">
<p>User: {user.name}</p>
<p>Age: {user.age}</p>
<button onClick={changeName}>Change name</button>
</div>
);
}
When you click the button, instead of updating just the name
, this will overwrite the entire user
object with the string "Arthur".
Correct Approach:
To update a specific property, create a new object using the spread operator:
javascriptCopy codeimport { useState } from "react";
export default function App() {
const [user, setUser] = useState({
name: "John",
age: 25,
});
// Correct way to update property of user state using spread operator
const changeName = () => {
setUser((user) => ({ ...user, name: "Arthur" }));
};
return (
<div className="App">
<p>User: {user.name}</p>
<p>Age: {user.age}</p>
<button onClick={changeName}>Change name</button>
</div>
);
}
Incorrect:
setUser((user) => (
user.name
= "Arthur"))
overwrites theuser
state with a string.Correct:
setUser((user) => ({ ...user, name: "Arthur" }))
creates a new object, updating only thename
property while keeping the other properties unchanged.
5. Not Defining a Default Value for useState
Pitfall: Failing to define a default value for useState
, which can lead to type errors or unexpected behavior when the state is used before it is set.
Solution: Always provide a sensible default value when initializing state with useState
. This ensures that your component can handle its state consistently from the start and prevents type errors.
Consider a component that manages a list of items:
javascriptCopy codeimport React, { useState } from 'react';
function ItemList() {
const [items, setItems] = useState(); // No default value
function addItem(item) {
setItems([...items, item]); // TypeError: items is undefined
}
return (
<div>
<ul>
{items && items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
<button onClick={() => addItem('New Item')}>Add Item</button>
</div>
);
}
export default ItemList;
Explanation: Without a default value, items
is initially undefined
. When you try to spread items
in setItems([...items, item])
, it results in a TypeError because you cannot spread undefined
.
const [items, setItems] = useState([]); // Default value is an empty array
function addItem(item) {
setItems([...items, item]); // Works correctly because items is always an array
}
Default Value: useState([])
initializes items
as an empty array. This ensures items
is always defined and can be safely used in the component.
Consistent State Handling: With a default value, you avoid type errors and ensure the state is always in a consistent and expected format.
Conclusion
State management using useState
is a fundamental aspect of building dynamic and interactive web applications with React. By understanding the common pitfalls and how to avoid them, you can write more efficient, predictable, and maintainable code. Always use the state setter function, respect immutability, use functional updates when necessary, manage state wisely, and remember that useState
is asynchronous. By following these best practices, you'll be well on your way to mastering state management in React.
If you liked this post, please give it a like and share it with others who might find it helpful!
Subscribe to my newsletter
Read articles from Artur directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
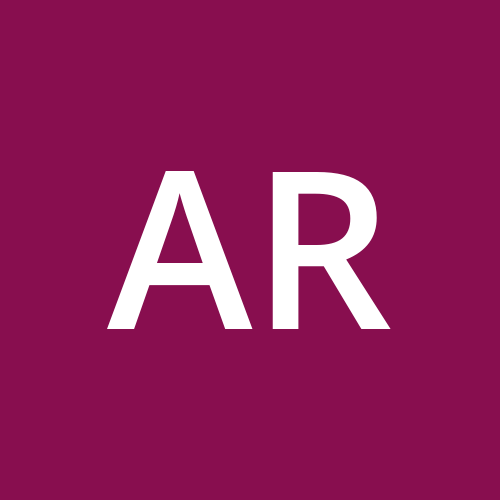