Day 70 - Terraform Modules

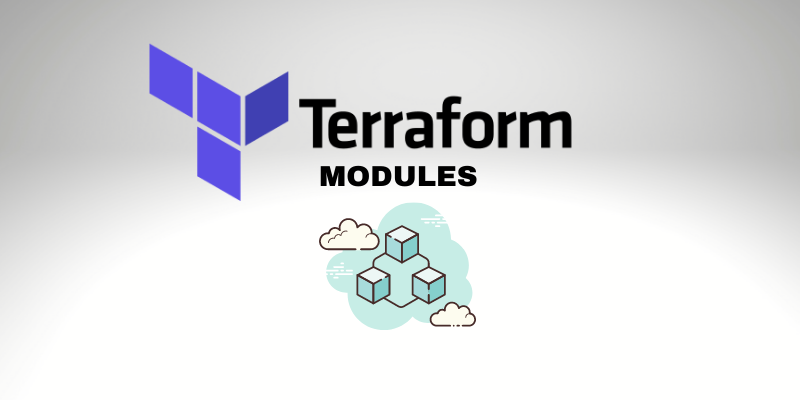
Modules are containers for multiple resources that are used together. A module consists of a collection of .tf and/or .tf.json files kept together in a directory
A module can call other modules, which lets you include the child module's resources into the configuration in a concise way.
Modules can also be called multiple times, either within the same configuration or in separate configurations, allowing resource configurations to be packaged and re-used.
Below is the format on how to use modules:
Creating a AWS EC2 instance:
resource "aws_instance" "server-instance" {
# Define number of instance
instance_count = var.number_of_instances
# Instance Configuration
ami = var.ami
instance_type = var.instance_type
subnet_id = var.subnet_id
vpc_security_group_ids = var.security_group
# Instance Tagsid
tags = {
Name = "${var.instance_name}"
}
}
The code provided is an example of a Terraform configuration file that creates an EC2 instance in AWS using the aws_instance resource. Here is a brief description of the different sections in this code:
resource "aws_instance" "server-instance": This line declares a new resource of type aws_instance with the name "server-instance".
instance_count = var.number_of_instances: This line sets the number of instances to create based on the value of the number_of_instances variable which will be defined in Server Module Variables section.
ami = var.ami: This line sets the ID of the Amazon Machine Image (AMI) to use for the instance based on the value of the ami variable.
instance_type = var.instance_type: This line sets the instance type of the instance based on the value of the instance_type variable.
subnet_id = var.subnet_id: This line sets the ID of the subnet where the instance will be launched based on the value of the subnet_id variable.
vpc_security_group_ids = var.security_group: This line sets the security group(s) that will be associated with the instance based on the value of the security_group variable.
tags = { Name = "${var.instance_name}" }: This line sets a tag with the key "Name" and the value of the instance_name variable on the instance.
Server Module Variables:
variable "number_of_instances" {
description = "Number of Instances to Create"
type = number
default = 1
}
variable "instance_name" {
description = "Instance Name"
}
variable "ami" {
description = "AMI ID"
default = "ami-xxxx"
}
variable "instance_type" {
description = "Instance Type"
}
variable "subnet_id" {
description = "Subnet ID"
}
variable "security_group" {
description = "Security Group"
type = list(any)
}
The code provided is an example of a Terraform configuration file that defines several variables that can be used to configure the creation of EC2 instances in AWS. Here is a brief description of each variable:
number_of_instances: This variable defines the number of EC2 instances to create. It has a default value of 1 if no value is provided.
instance_name: This variable is used to set the name of the EC2 instance. It does not have a default value, meaning that the user must provide a value for this variable.
ami: This variable is used to set the ID of the Amazon Machine Image (AMI) to use for the EC2 instance. It has a default value of "ami-xxxx" if no value is provided.
instance_type: This variable is used to set the instance type of the EC2 instance. It does not have a default value, meaning that the user must provide a value for this variable.
subnet_id: This variable is used to set the ID of the subnet where the EC2 instance will be launched. It does not have a default value, meaning that the user must provide a value for this variable.
security_group: This variable is used to set the security group(s) that will be associated with the EC2 instance. It has a type of list(any) to allow the user to specify one or more security groups. It does not have a default value, meaning that the user must provide a value for this variable.
Server module Output:
output "server_id" {
description = "Server ID"
value = aws_instance.server-instance.id
}
The code provided is an example of a Terraform configuration file that creates an EC2 instance in AWS using the aws_instance resource and outputs its ID using the output section. Here is a brief description of the different sections in this code:
output "server_id": This line declares a new output named "server_id".
value = aws_instance.server-instance.id: This line sets the value of the output to the ID of the EC2 instance created earlier with the aws_instance resource. The format aws_instance.server-instance.id refers to the attribute id of the resource aws_instance named "server-instance".
Task-01
Explain the below in your own words.
1.Write about different modules Terraform.
Terraform is a popular open-source infrastructure as code (IAC) tool that allows you to manage and provision cloud resources across multiple cloud providers. One of the key features of Terraform is its modular architecture, which allows you to break down your infrastructure code into smaller, reusable components called modules. This makes it easier to manage complex infrastructures and promote code reuse.
There are different types of modules in Terraform, including:
1.Root modules: The root module is the top-level module that serves as the entry point for your entire Terraform configuration. It typically includes your provider configuration and any variables that are needed to define your infrastructure resources.
2. Child modules: A child module is a sub-module that is nested within the root module or another child module. It can be used to group related resources and configurations together for easier management.
3. Published Modules: A published module in Terraform refers to a module that has been shared with the community through the Terraform Module Registry. The Terraform Module Registry is a public repository where users can share their modules with others to promote code reuse and collaboration..
Each module contains a set of resources and configurations that can be managed independently. This makes it easier to manage and test your infrastructure code, and promotes code reuse across your organization. You can create your own custom modules, or use existing modules from the Terraform Module Registry, which is a public repository of Terraform modules contributed by the community.
2.Difference between Root Module and Child Module.
The main difference between a root module and a child module in Terraform is their role in the overall configuration.
A root module is the top-level module of a Terraform configuration. It serves as the entry point for your entire Terraform configuration and typically includes your provider configuration and any variables that are needed to define your infrastructure resources. A root module is responsible for initializing Terraform, configuring providers, and declaring resources.
On the other hand, a child module is a sub-module that is nested within the root module or another child module. It is used to group related resources and configurations together for easier management. A child module is typically created to encapsulate a set of resources that perform a specific function. Child modules are reusable components that can be used in multiple root modules.
Child modules can be defined within a root module using a module block, which specifies the source of the module and any input variables that need to be set. The source can be a local file path or a remote location, such as a version control system or a Terraform Module Registry.
Another difference between root modules and child modules is their visibility of variables. A root module can declare and reference variables that are visible to all child modules, while a child module can declare and reference variables that are only visible within that module.
- Is modules and Namespaces are same? Justify your answer for both Yes/No
No, modules and namespaces are not the same concept, although they may share some similarities in certain contexts.
A module in Terraform is a self-contained unit of infrastructure code that encapsulates a set of related resources and can be reused across multiple configurations. Modules help to promote code reuse, modularity, and abstraction in Terraform, allowing users to manage complex infrastructures more easily.
On the other hand, a namespace is a way of organizing names or identifiers in a way that avoids naming conflicts. Namespaces are commonly used in programming to avoid naming conflicts between different modules, functions, or variables. They provide a way to group related names together under a common prefix or label.
While both modules and namespaces are used for organization and abstraction, they serve different purposes in different contexts. Modules in Terraform are used to organize and reuse infrastructure code, while namespaces are used to organize and avoid naming conflicts between different identifiers in programming.
Thank you for reading!
Subscribe to my newsletter
Read articles from Nikhil Yadav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Nikhil Yadav
Nikhil Yadav
I am a highly motivated and enthusiastic individual completed B.Tech from Savitribai Phule University, Pune . With a strong interest in DevOps and Cloud technologies, I am eager to kick-start my career in this domain. Although I do not have much professional experience, I possess a willingness to learn, excellent problem-solving skills, and a passion for technology. I am committed to contributing my best to any team I work with.