Getting Started with C++: Essential Concepts (Part 1)
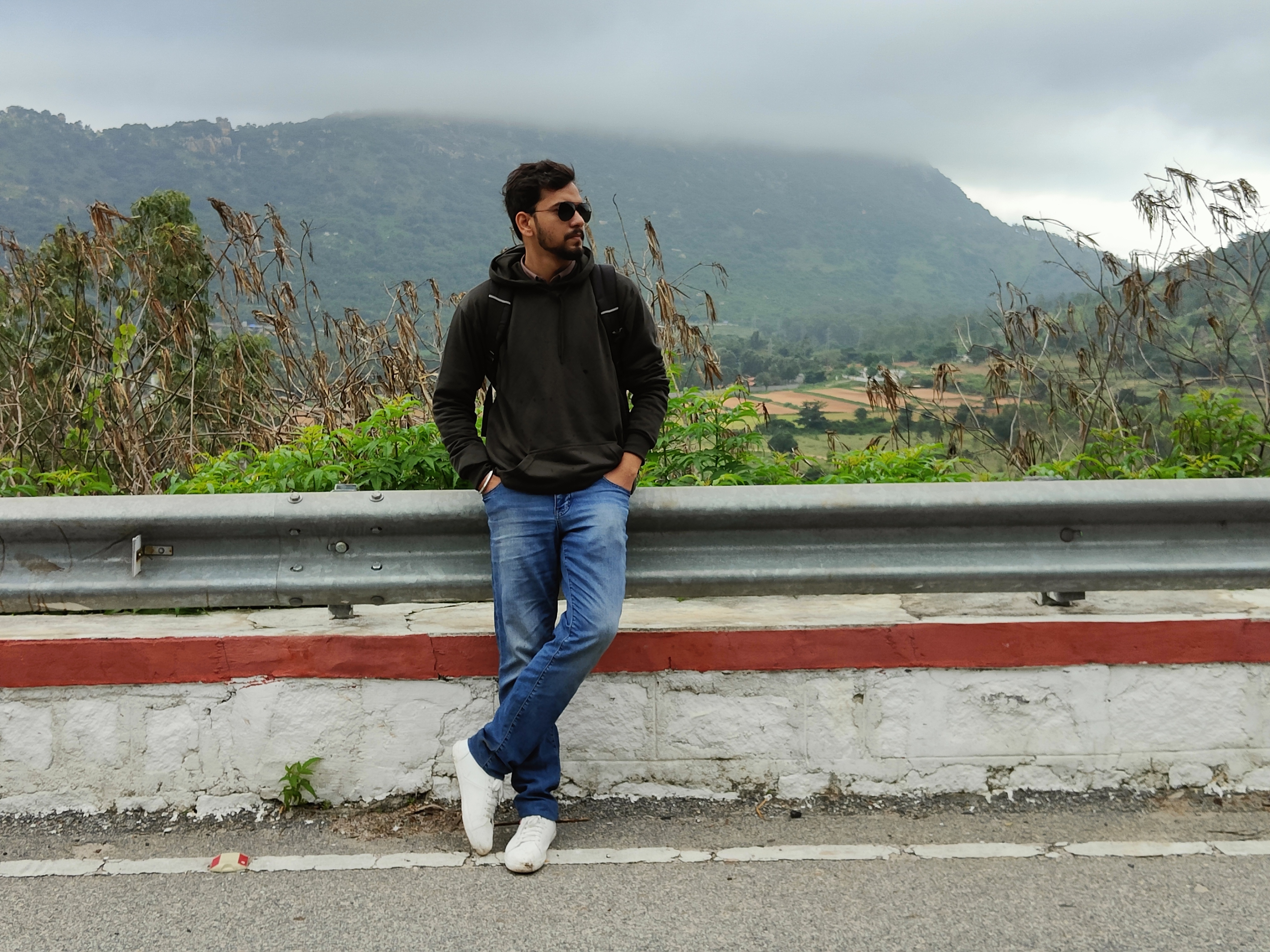
What is a Programming Language?
A Programming Language is a computer language that is used by programmers(Developers) to communicate with computers. It is a set of instructions written in any specific language (C, C++, Java, Python) to perform specific tasks. To communicate with computers we need to convert programming language into a Machine understandable format. This is done using a Compiler.
A compiler is a set of programs that translates source programs into machine code. C++ is a high-level general-purpose programming language created by Danish Computer scientist Bjarne Stroustrup.
Why do we need it?
Performance: Efficiency and speed
Control over system resources and memory
Object Oriented programming
Use of C++:
System Software Development
Game Development
Real-time Systems
First Program: Let's Write "Hello World"
Let's Break down the above code one by one:
line 1: #include is a header file that contains function declarations, macro definitions, and other necessary details that the current file needs to compile and run correctly. <iostream> is a standard C++ file that provides facilities for standard libraries and input/output operations.
line 2: It acts as a dictionary that allows you to use names from the standard C++ library (like 'cin', 'court' etc).
line 3: It is the starting point of the code [curly brackets -> '{}', Parentheses -> '()' ]. All the programs should be written within the curly brackets. In the programming world, we call it as "Scope of the program".
line 4: cout is used to print. '<<' is called an insertion operator. It inserts the data into the standard output stream. With the double inverted commas cout will print as it is. 'endl' is an output manipulator. It stands for 'end line'. This will move the cursor to the next line. ';'(semicolon) is used to terminate the statements. Each statement must end with a semicolon which indicates the end of the statement.
return 0: It represents the successful execution of the program. If not 0 it shows unsuccessful execution.
All about Variables and Datatypes
Variables are used to store data that can be manipulated during the execution of the program.
Example:
Declaration: This is where you define the type and name of the variable.
Initialization: This is where you initialize the initial value of the variable.
int variable = 5;
- 'int' - which type of data needs to be stored, 'variable' - the name of the block or space where you want to store the value, '5' - the data or value.
Categorization of Datatypes:
sizeOf() is a type of operator in C++ that is used to find how much space is taken by datatype. For every datatype, the amount of space that will be allocated in memory will vary depending on the machine bits level.
1 Byte = 8 Bits
Note: Boolean is taking 1 byte instead of 1bit because in memory 1 byte is the only addressable space.
User Input in C++
'cin' is an object of the iostream that allows you to read input.
Control flow statements
In C++ control flow statements allow you to manage the order of execution within your program.
- If condition: ' if(condition) ' allows you to execute a block of code conditionally based on whether a specified condition is true or false.
if-else: The 'if-else' condition allows you to execute different blocks of code based on whether a specified condition is true or false.
if-else if: if-else if condition statement allows you to handle multiple conditions in a structured way.
if-else if else:
Nested if: The nested if condition allows you to create multilayered conditions by placing an if statement inside another.
Switch-Case:
The switch case statement in C++ is a control flow statement that allows you to execute different blocks of code based on the value of an expression. It's an alternative to using a series of if-else statements when you need to compare a variable against multiple values.
Conditions about switch statements:
Expression type: valid for int/char/enum. Not valid for string and float.
Unique case value: The value of each case should be unique.
No range checking.
Fall through behavior: All use breaks after every case.
Ternary Operator
The ternary operator (also known as the conditional operator) provides a concise way to execute one of two expressions based on a condition.
In part 2 we will cover loops, functions, operators, etc. Thanks.
Continue reading...
Rishabh Kumar
Subscribe to my newsletter
Read articles from Rishabh Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
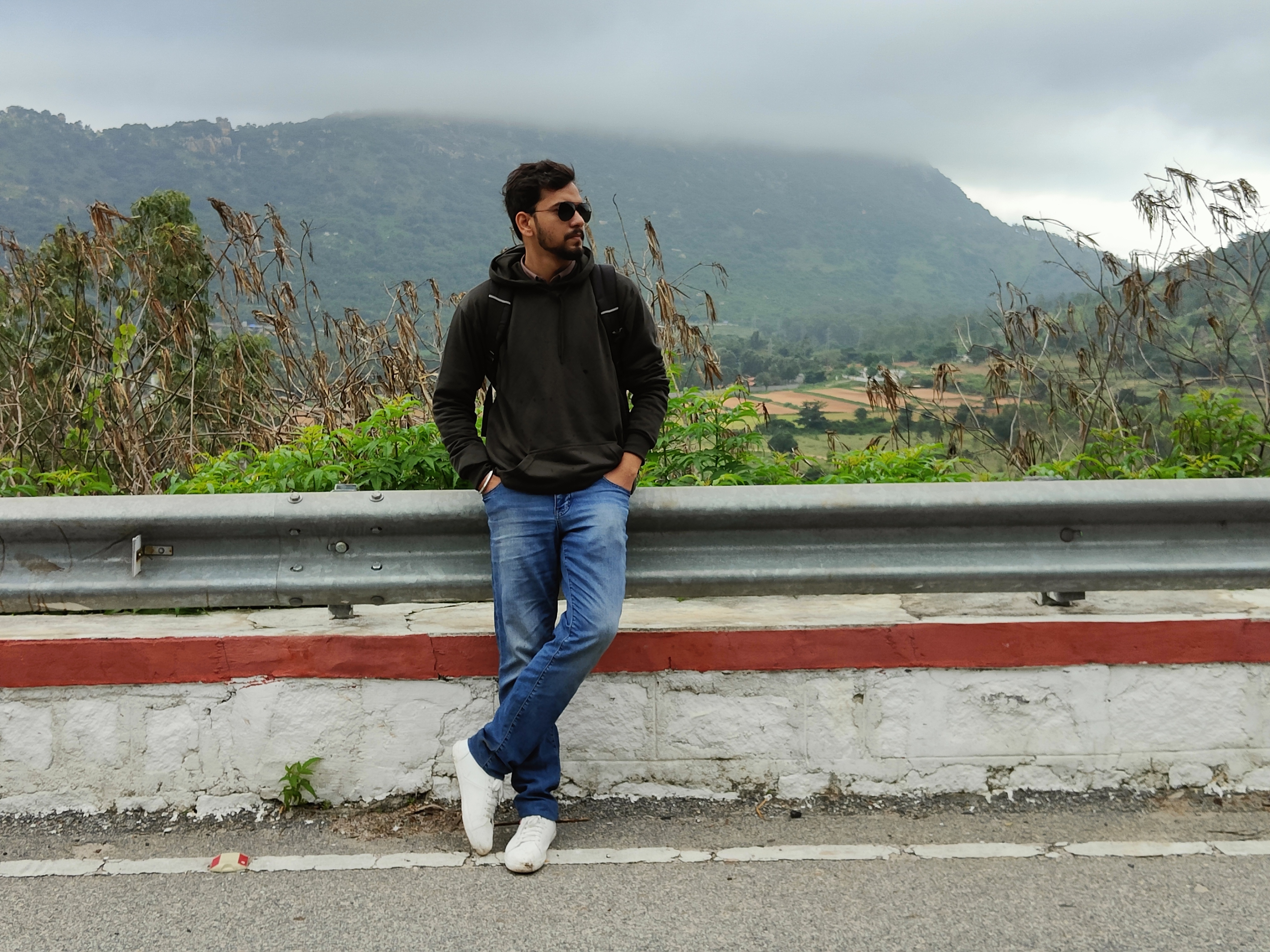