useCallback vs. useMemo in React
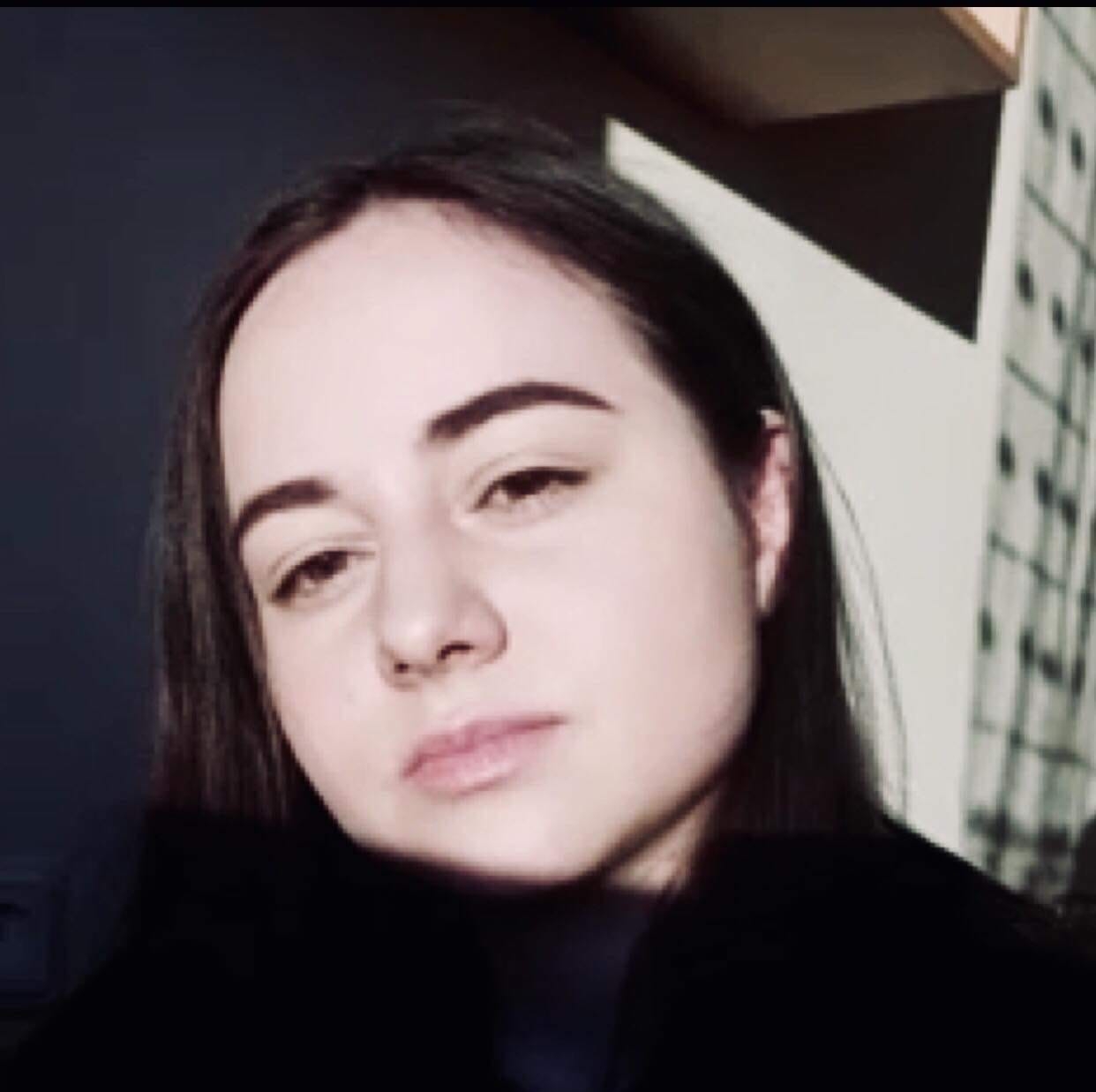
Table of contents
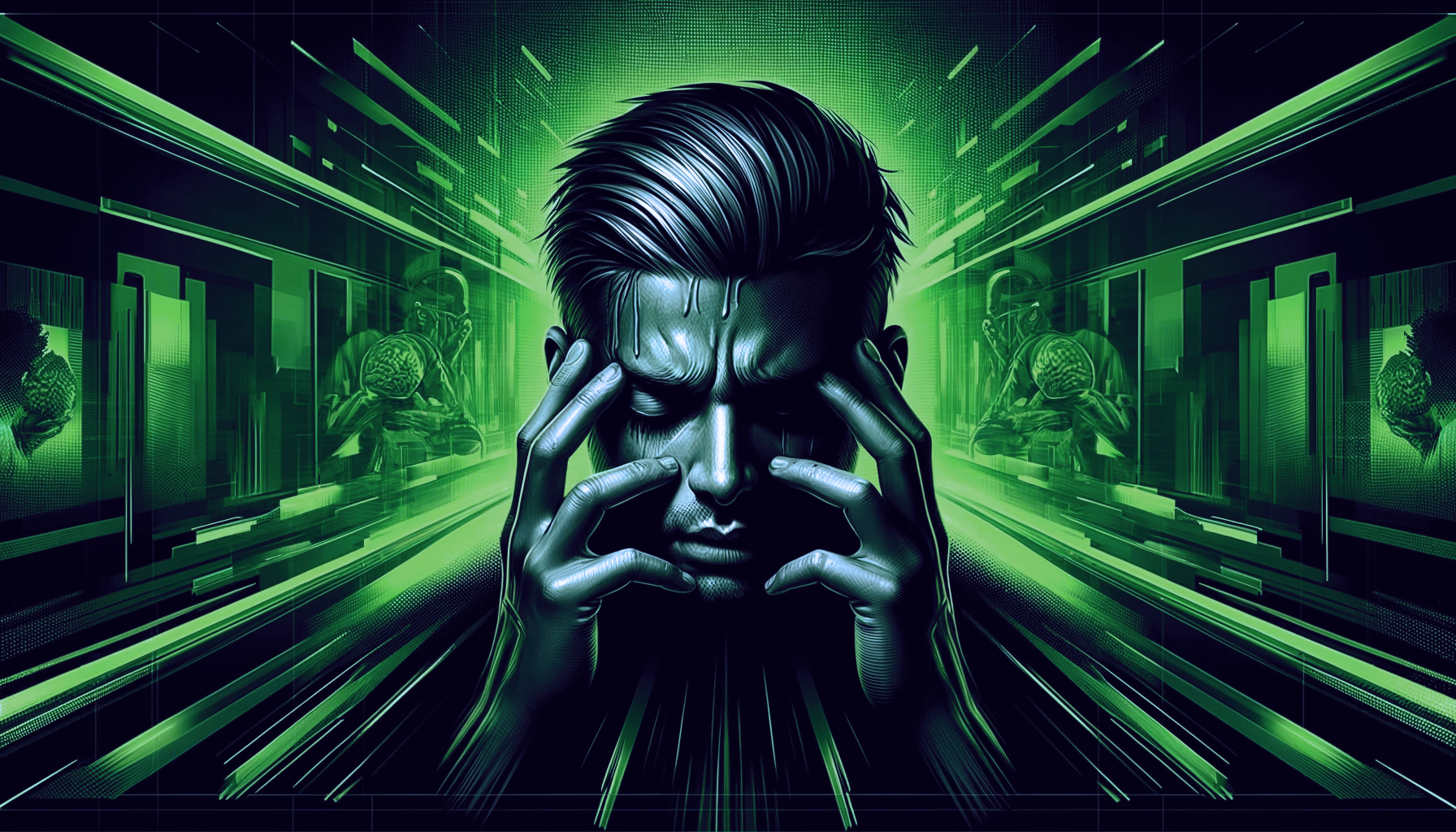
React offers plenty of hooks for various use cases. useMemo
and useCallback
are widely used hooks in the React community to solve performance issues. While they might seem similar, it's crucial to understand the key differences in how they function. Let’s go through each to understand the basics.
useMemo Hook
The useMemo
hook is really useful when dealing with expensive calculations. It memoizes the result of a function call and returns the cached result when the dependencies have not changed. For example, let’s say you have to map through a very large array to find a specific number. Each time the number you are looking for might change, so the mapping is necessary. But if the number you need to find is the same as before, this expensive mapping should be avoided. This is where useMemo
helps. See the example below:
In this example, useMemo
avoids running the expensive computation if the target
number hasn't changed. The dependency array [target]
ensures that the computation runs only when target
changes.
useCallback Hook
useCallback
is a bit more complex and tricky to use. Unlike useMemo
, which memoizes the result of a function call, useCallback
memoizes the function itself. To understand why this is useful, let's delve into how React works. Every time a component re-renders, React creates new instances of functions, which means function references change even if the logic inside them remains the same.
This behavior can cause unnecessary re-renders in child components that rely on these functions. useCallback
helps React remember the function between re-renders, preventing unnecessary updates:
In this example, the increment
function is memoized using useCallback
, so Child
does not re-render unnecessarily when the Parent
component re-renders. The dependency array []
ensures that the function reference remains the same across re-renders.
Pitfalls
While useMemo
and useCallback
are useful for optimizing performance, they should be used judiciously. These hooks themselves consume computation power to determine whether the memoized values should be used. Therefore, if the computation or re-rendering cost is not significant, using these hooks might not be worth it.
In Addition
Dependencies: Always specify dependencies correctly. Incorrect dependencies can lead to stale values or unnecessary re-renders.
Testing: Ensure you test your components thoroughly when using these hooks, as incorrect usage can lead to subtle bugs.
Profiling: Use React's built-in Profiler or other performance profiling tools to identify if
useMemo
anduseCallback
actually provide a performance benefit.
These are the key points to understand when using useMemo
and useCallback
in React. Properly utilized, they can significantly enhance your application's performance.
Subscribe to my newsletter
Read articles from Ugne Adomaityte directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
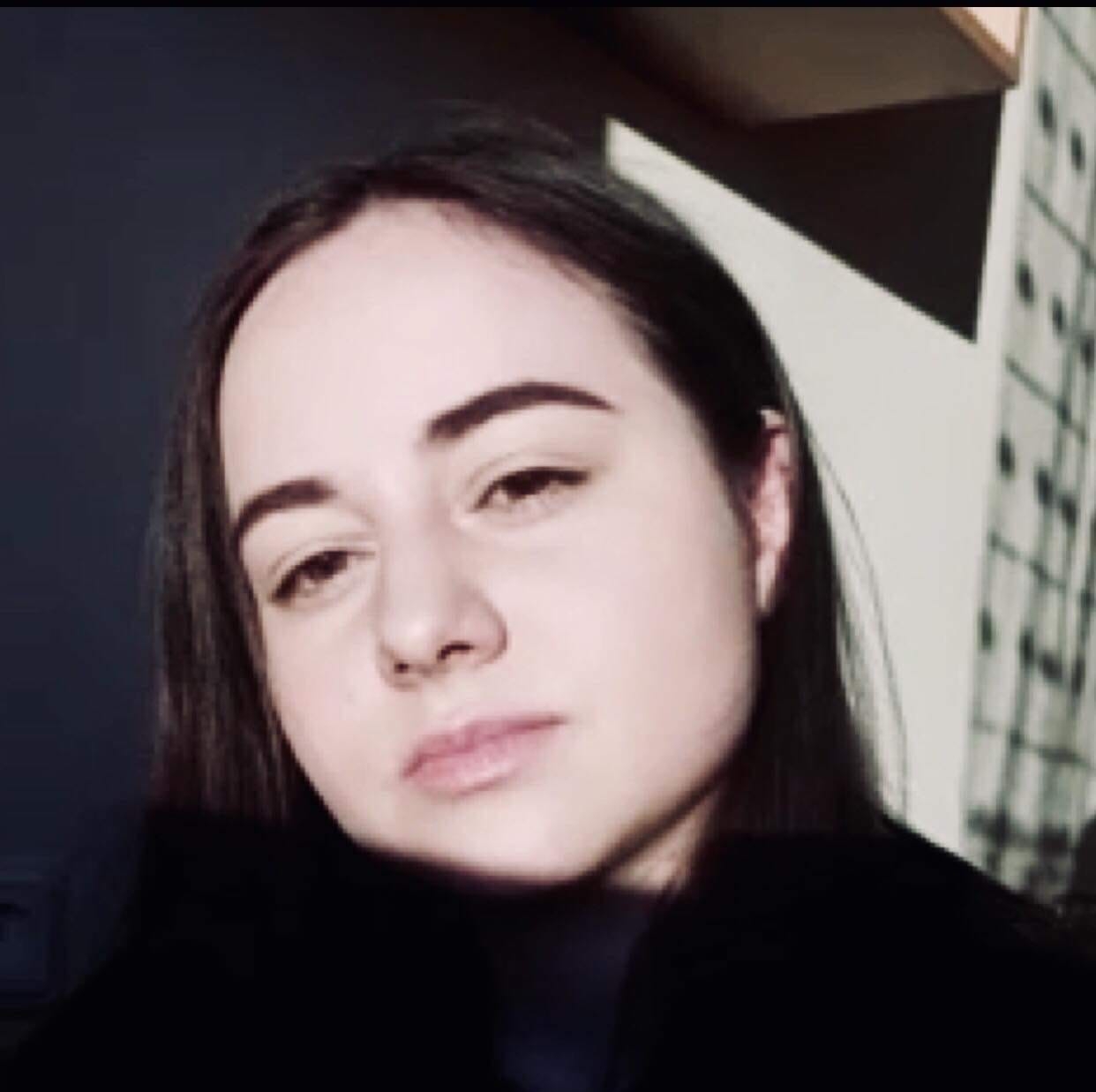