Technical Interview Challenge using React.js
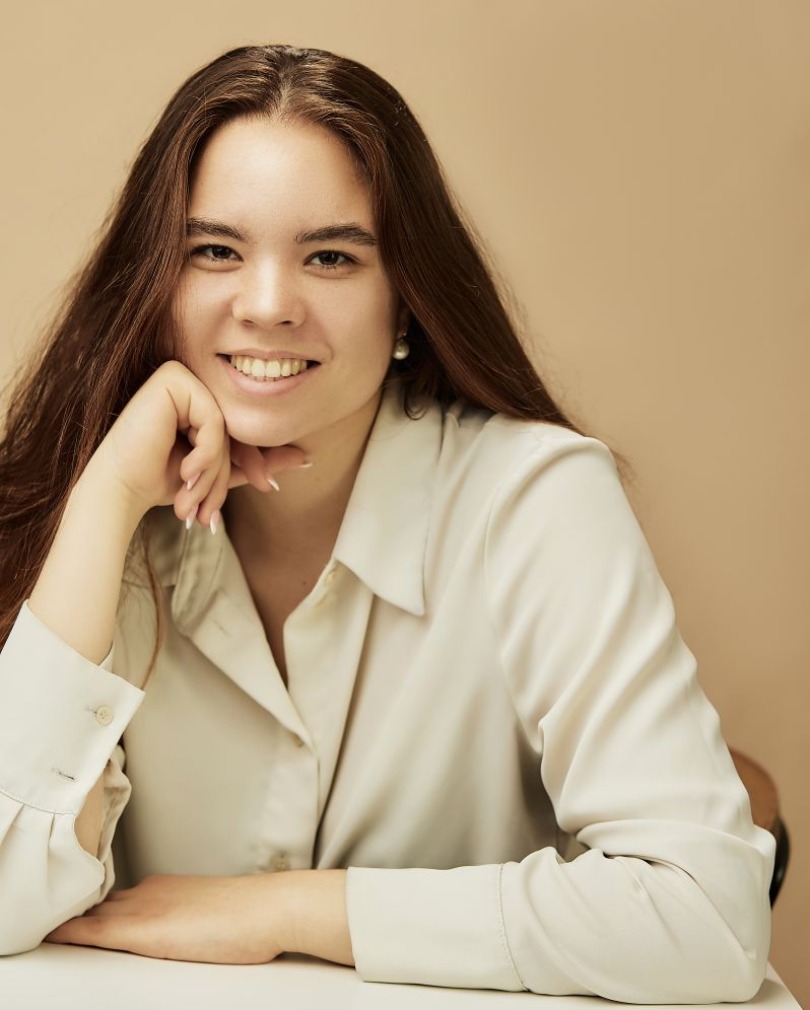
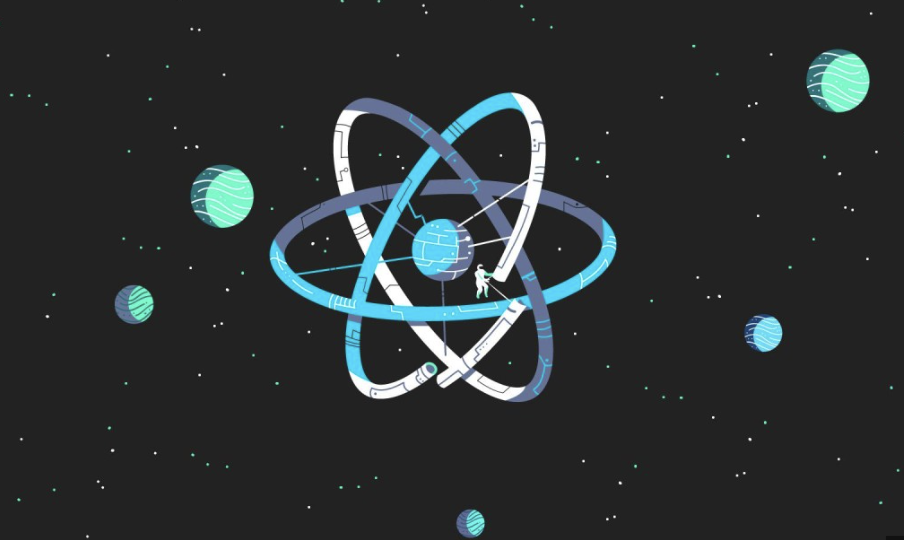
In a recent front-end interview, I was given a technical challenge involving several key concepts in React.js:
Conditional rendering
Managing a loading state in a React.js component
Using the map() method to render elements to the UI
Error handling and performance: HTTP request abortion and
clearTimeout()
The Challenge
Fetch an API (JSON file) to display movie details, show a "Loading..." message for 2 seconds before fetching completes, and test it.
Conditional Rendering
Conditional rendering displays components based on certain conditions. For our "Loading..." message, we check the isLoading
variable. If true
, "Loading..." is displayed; otherwise, the movie list is rendered.
<div>
{isLoading ? (
<p>Loading...</p>
) : (
movies.map((movie) => (
<div key={movie.imdbID}>
<h2>
{movie.Title} - {movie.Year}
</h2>
</div>
))
)}
Ï</div>
Managing a Loading State
Standard JS variables don't re-render React components. We use state variables instead. The isLoading
state shows a "Loading..." message before displaying movie data.
Our fetchData()
function handles fetching and aborting the request if needed. Initially, isLoading
is set to false
. When fetchData()
is called, it sets isLoading
to true
and fetches data. Once data is fetched, isLoading
is set back to false
.
Rendering with map()
The map()
method iterates over data and renders each item. It returns a new array of elements, which React then renders. Each element needs a unique key
for performance and rendering optimization.
movies.map((movie) => (
<div key={movie.imdbID}>
<h2>
{movie.Title} - {movie.Year}
</h2>
</div>
))
Error Handling and Performance
We use AbortController
to manage HTTP request abortion and clearTimeout()
to abort fetch requests. The fetchData()
function takes a controller as a parameter. If needed, the fetch request can be aborted using controller.signal
.
Errors are handled with a try...catch
block. Any fetching errors are caught, logged, and isLoading
is set to false
.
Conclusion
This exercise covered essential React.js concepts like testing, performance, and system design.
Also, the importance of effective state management and error handling in building robust applications.
Hope it helps ✌️
Subscribe to my newsletter
Read articles from Olga Nedelcu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
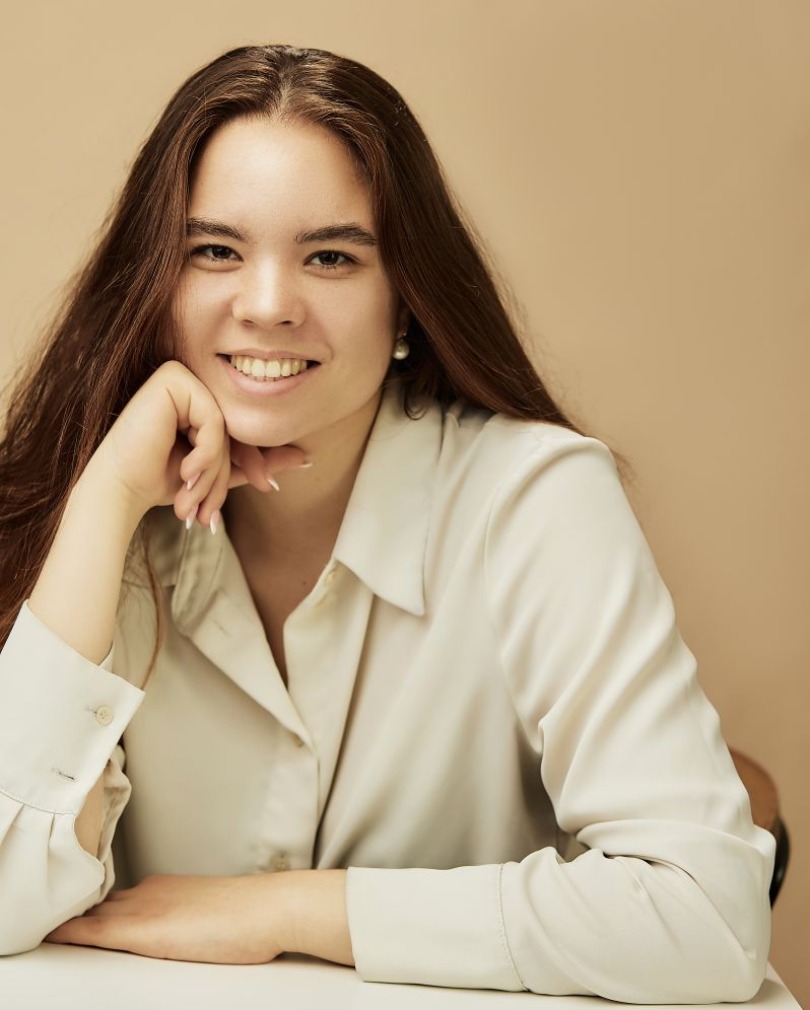
Olga Nedelcu
Olga Nedelcu
I'm a community-minded engineer based in Berlin, originally from Romania, and I grew up in sunny Spain. I combine the best of both communication and technical skills, with experience in both the engineering world and the business side of things. I'm proficient in a wide range of technologies like JavaScript, React.js, Redux, TypeScript, Node.js, HTML/CSS, Styled Components, SASS, and REST APIs. I love going beyond just writing code by sharing knowledge with my team through Communities of Practice (COPs), Employee Resource Groups (ERGs), and detailed technical documentation. My main interests are in frontend frameworks, web standards, accessibility (A11y), and Clean Code. My passion for programming started in high school when I built my first website. Since 2019, I've been working as a professional software developer, thriving in agile and iterative environments. Nearly five years later, I'm still excited to dive into code and collaborate with my colleagues. I'm very open and friendly, good at turning technical concepts into easy-to-understand information for everyone.