NestJS; A Beginner's Guide
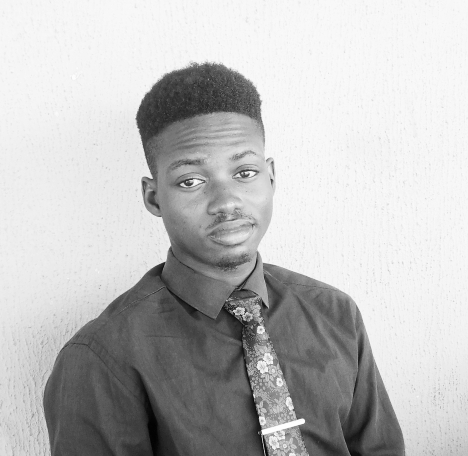
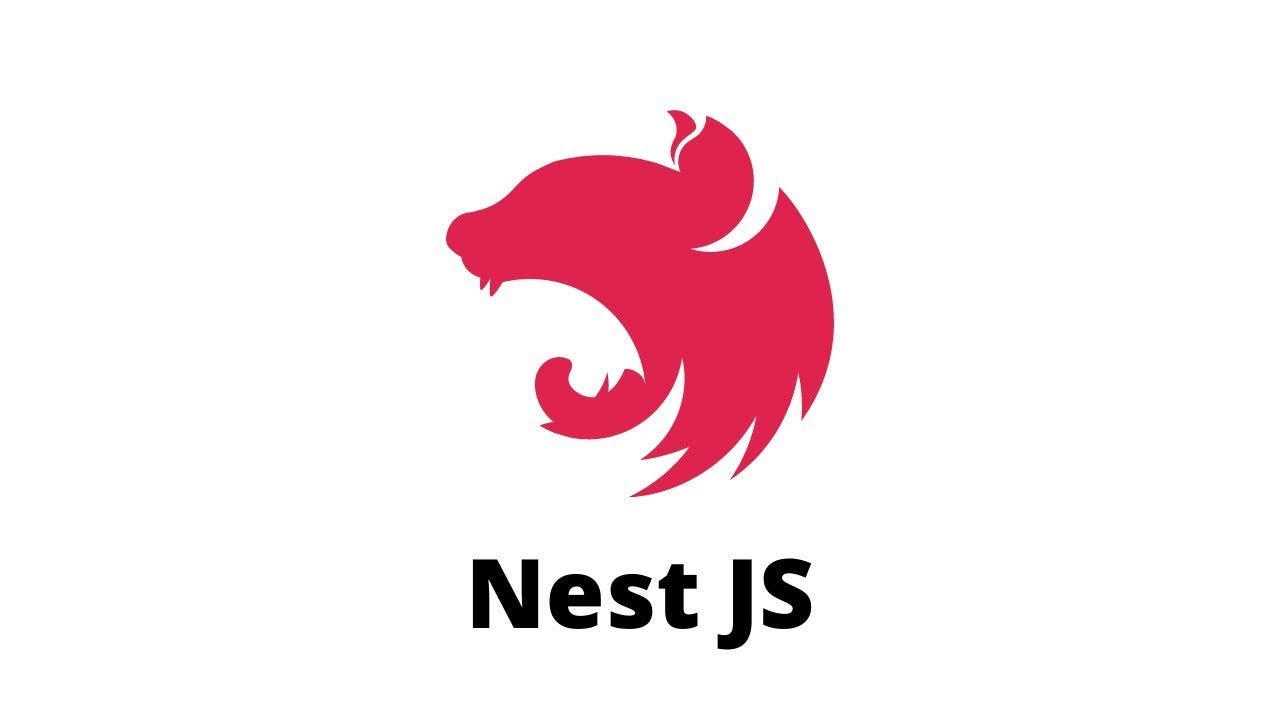
Introduction
NestJS is a progressive Node.js framework for building efficient and scalable server-side applications. It uses TypeScript by default and is heavily inspired by Angular, bringing familiar concepts such as dependency injection and a modular architecture to the backend. Whether you're new to backend development or experienced with Node.js, NestJS offers a robust platform for creating well-structured and maintainable applications.
In this guide, we'll walk you through setting up a basic NestJS project and building a simple REST API. By the end of this tutorial, you'll have a solid foundation for further exploring the framework's powerful features.
What You'll Learn After This Tutorial
How to install and set up a NestJS project
Understanding the project structure
Creating controllers and services
Building a simple REST API
Running and testing your NestJS application
Key Terms to Understand Before Proceeding
NestJS: A framework for building server-side applications with Node.js and TypeScript.
TypeScript: A typed superset of JavaScript that compiles to plain JavaScript.
Controller: A class in NestJS that handles incoming HTTP requests and returns responses.
Service: A class that handles business logic and data access in NestJS.
Module: A class that organizes related controllers and services in a NestJS application.
Body
Setting Up Your NestJS Project
Installing NestJS CLI
First, you'll need to install the NestJS CLI (Command Line Interface) globally using npm (Node Package Manager). Open your terminal and run the following command:
npm install -g @nestjs/cli
Creating a New Project
With the CLI installed, you can create a new NestJS project by running:
nest new my-nestjs-app
The CLI will prompt you to choose a package manager (npm or yarn). Select your preferred option and wait for the dependencies to be installed.
Navigating the Project Structure
Once the project is created, navigate to the project directory:
cd my-nestjs-app
The generated project structure will look like this:
my-nestjs-app ├── src │ ├── app.controller.ts │ ├── app.controller.spec.ts │ ├── app.module.ts │ ├── app.service.ts │ └── main.ts ├── test │ └── app.e2e-spec.ts │ └── jest-e2e.json ├── .eslintrc.js ├── .prettierrc ├── nest-cli.json ├── package.json ├── tsconfig.build.json └── tsconfig.json
Creating Controllers and Services
Understanding Controllers
Controllers handle incoming requests and return responses to the client. Let's create a simple controller to handle a GET request.
In the
src
directory, openapp.controller.ts
and modify it as follows:import { Controller, Get } from '@nestjs/common'; import { AppService } from './app.service'; @Controller() export class AppController { constructor(private readonly appService: AppService) {} @Get() getHello(): string { return this.appService.getHello(); } }
Creating a Service
Services encapsulate the business logic of the application. They are typically used by controllers to process requests.
Open
app.service.ts
and modify it:import { Injectable } from '@nestjs/common'; @Injectable() export class AppService { getHello(): string { return 'Hello, World!'; } }
Connecting the Controller and Service
The
AppController
uses theAppService
to handle the logic for the GET request. The service methodgetHello
returns a simple string.
Building a Simple REST API
Creating a RESTful Endpoint
Let's create a new controller and service to handle a RESTful endpoint for managing users. First, generate a new module:
nest generate module users
Then, generate a new controller and service for the
users
module:nest generate controller users nest generate service users
Now, modify the
users.controller.ts
file:import { Controller, Get, Post, Body } from '@nestjs/common'; import { UsersService } from './users.service'; @Controller('users') export class UsersController { constructor(private readonly usersService: UsersService) {} @Get() findAll(): string { return this.usersService.findAll(); } @Post() create(@Body() createUserDto: any): string { return this.usersService.create(createUserDto); } }
And modify the
users.service.ts
file:import { Injectable } from '@nestjs/common'; @Injectable() export class UsersService { private readonly users = []; findAll(): string { return JSON.stringify(this.users); } create(user: any): string { this.users.push(user); return 'User created!'; } }
Running and Testing Your NestJS Application
Starting the Application
To start your NestJS application, run the following command in your project directory:
npm run start
Your application will be running at
http://localhost:3000
.Testing the API
You can use tools like Postman or curl to test your API endpoints. For example, to test the
GET /users
endpoint, send a GET request tohttp://localhost:3000/users
.To test the
POST /users
endpoint, send a POST request with a JSON body tohttp://localhost:3000/users
.
Conclusion
In this guide, we've covered the basics of setting up a NestJS project, creating controllers and services, and building a simple REST API. NestJS provides a powerful and flexible framework for developing server-side applications with Node.js and TypeScript. With a solid foundation in these core concepts, you're now ready to explore more advanced features and build robust applications with NestJS.
FAQ
Q: What is NestJS?
A: NestJS is a progressive Node.js framework for building efficient and scalable server-side applications using TypeScript.
Q: How do I install NestJS?
A: You can install NestJS by running npm install -g @nestjs/cli
to install the CLI globally, and then create a new project using nest new project-name
.
Q: What are controllers and services in NestJS?
A: Controllers handle incoming HTTP requests and return responses, while services encapsulate the business logic of the application and are typically used by controllers.
Q: How do I run a NestJS application?
A: You can run a NestJS application by navigating to your project directory and executing npm run start
.
Q: What tools can I use to test my API endpoints?
A: You can use tools like Postman or curl to send HTTP requests to your API endpoints and test their responses.
Subscribe to my newsletter
Read articles from Adeoye David directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
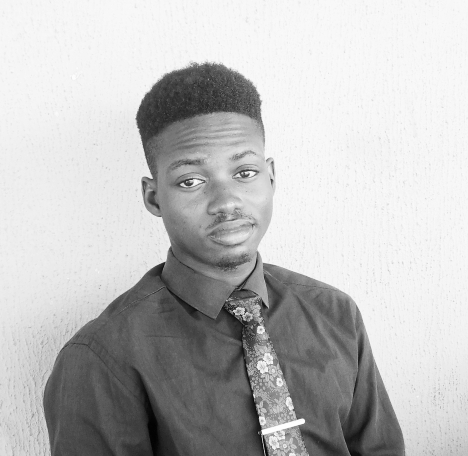
Adeoye David
Adeoye David
I'm David, a passionate backend developer and avid technical writer dedicated to crafting clean, efficient, and scalable software solutions. With a deep-rooted love for code and a knack for communicating complex technical concepts in a clear and concise manner, I thrive in the intersection of technology and writing. As a backend developer, I specialize in building robust and reliable server-side applications using technologies like Node.js, Express.js, and MongoDB. Whether it's architecting scalable APIs, optimizing database performance, or implementing secure authentication mechanisms, I'm always up for the challenge of tackling backend complexities. Beyond my coding endeavors, I find immense joy in the art of technical writing. I believe that effective documentation is just as important as writing code—it empowers users, fosters collaboration, and ensures the long-term success of software projects. From comprehensive API guides to in-depth tutorials, I strive to create informative and accessible content that educates and inspires fellow developers. On this platform, you'll find a blend of my technical insights, coding adventures, and musings on software engineering best practices. Whether you're a seasoned developer seeking to deepen your knowledge or a newcomer navigating the world of backend development, I invite you to join me on this journey of exploration and discovery.