Building Rock-Solid Flutter Apps: A Guide to Testing & Quality Assurance
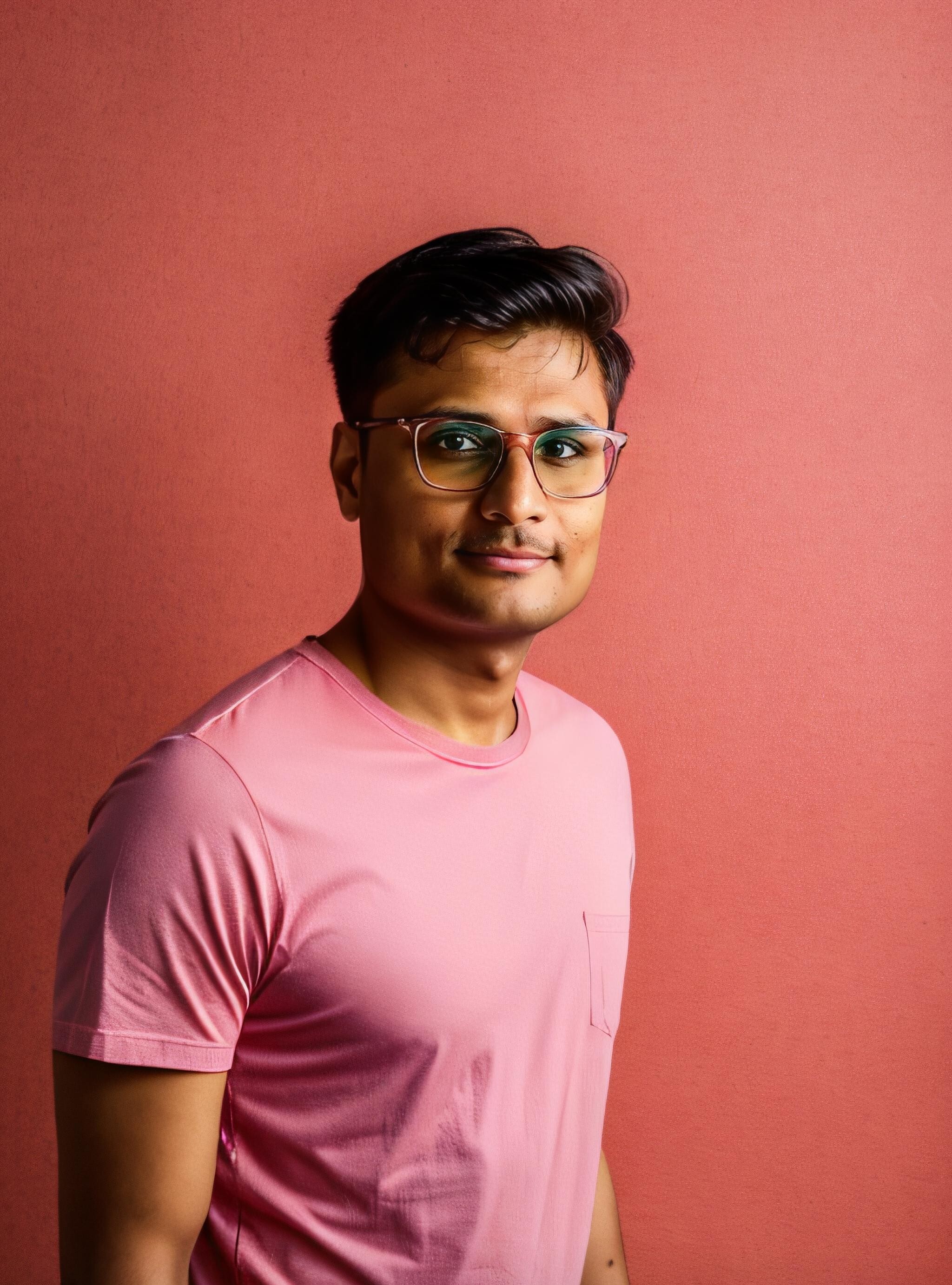
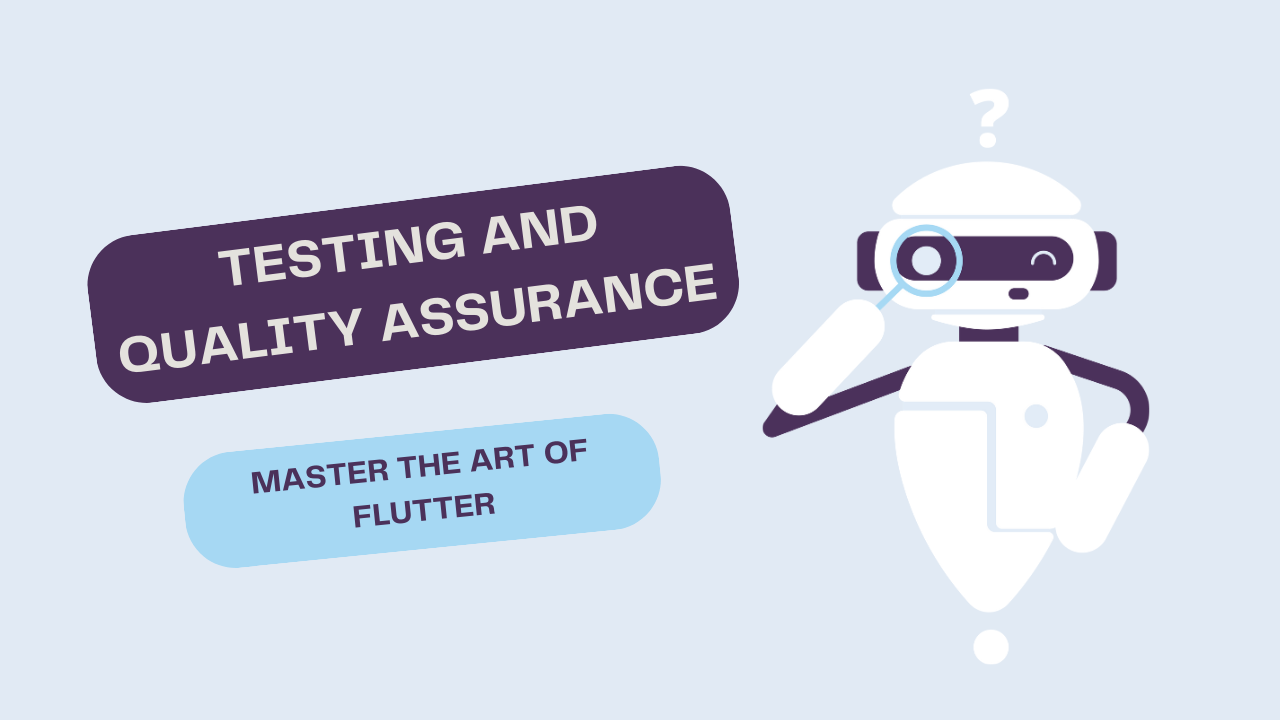
Testing is the cornerstone of crafting high-quality Flutter apps. In this article, we’ll delve into the world of Flutter testing and quality assurance, equipping you with the knowledge and techniques to build robust and user-friendly apps.
Writing Unit Tests for Flutter Apps
Unit tests are used to test individual units or components of our code in isolation. In Flutter, we can write unit tests to verify the behavior of specific functions, classes, or modules. These tests help us ensure that our code is working correctly and producing the expected results.
For example, let’s say we have a function called calculateSum()
that calculates the sum of two numbers. We can write a unit test to verify its functionality.
void main() {
test('Calculate sum test', () {
expect(calculateSum(2, 3), equals(5));
});
}
int calculateSum(int a, int b) {
return a + b;
}
In this example, we are testing the calculateSum()
function to ensure that it correctly adds two numbers and returns the expected result. The expect()
function is used to define the assertion and verify that the actual result matches the expected result.
Integration Testing
Integration testing focuses on testing the interaction between different components of an app. It ensures that the various parts of our app work together seamlessly. In Flutter, we can write integration tests to verify that different screens, widgets, or modules are integrated correctly and functioning as expected.
For instance, let’s consider a scenario where we have a login screen and a home screen. We want to test the flow from the login screen to the home screen. We can write an integration test to simulate this flow.
void main() {
testWidgets('Login to home screen test', (WidgetTester tester) async {
await tester.pumpWidget(MyApp());
// Simulate login process
await tester.enterText(find.byType(TextField).first, 'username');
await tester.enterText(find.byType(TextField).last, 'password');
await tester.tap(find.text('Login'));
await tester.pump();
// Verify that we are on the home screen
expect(find.text('Welcome to the Home Screen'), findsOneWidget);
});
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Column(
children: <Widget>[
TextField(decoration: InputDecoration(labelText: 'Username')),
TextField(decoration: InputDecoration(labelText: 'Password')),
RaisedButton(
child: Text('Login'),
onPressed: () {
// Login logic
},
),
],
),
),
);
}
}
In this example, we are testing the integration between the login screen and the home screen. We simulate the login process by entering the username and password, tapping the login button, and verifying that we are on the home screen.
UI Testing
UI testing, also known as widget testing in Flutter, focuses on testing the visual and interactive aspects of our app’s user interface. It ensures that our app’s UI components, such as widgets and layouts, are rendered correctly and respond appropriately to user interactions.
Let’s consider a scenario where we have a simple app with a button that increments a counter when tapped. We can write a UI test to verify this behavior.
void main() {
testWidgets('Counter increment test', (WidgetTester tester) async {
await tester.pumpWidget(MyApp());
// Verify initial counter value
expect(find.text('0'), findsOneWidget);
// Tap the button
await tester.tap(find.byIcon(Icons.add));
await tester.pump();
// Verify that the counter value has incremented
expect(find.text('1'), findsOneWidget);
});
}
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
int counter = 0;
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(counter.toString()),
IconButton(
icon: Icon(Icons.add),
onPressed: () {
setState(() {
counter++;
});
},
),
],
),
),
),
);
}
}
In this example, we are testing the UI of a counter app in Flutter. We simulate tapping the add button and verify that the counter value is incremented correctly.
Best Practices for Flutter Testing
To ensure effective testing and quality assurance in Flutter, consider the following best practices:
Write tests early: Start writing tests as early as possible in the development process to catch issues early and ensure code reliability.
Test all possible scenarios: Cover different use cases, edge cases, and scenarios to ensure that your app works correctly in various situations. For example, if you have a form in your app, make sure to test scenarios where all fields are filled, some fields are empty, or invalid data is entered.
Use mock dependencies: When testing components that depend on external services or APIs, use mock dependencies to isolate the component being tested and ensure consistent results.
For instance, if your app makes API calls, you can use a mock API client during testing to simulate the responses and avoid hitting the actual API.Automate testing: Set up automated testing pipelines to run tests regularly, ensuring continuous integration and quick feedback on code changes.
You can use tools like Flutter Driver or integration with CI/CD platforms to automate your tests and run them on different devices or simulators.Monitor test coverage: Keep track of your test coverage to ensure that critical parts of your app are thoroughly tested.
There are various code coverage tools available for Flutter, such as lcov and coverage, that can help you measure the effectiveness of your tests.
By following these best practices and utilizing the various testing techniques available in Flutter, you can enhance the quality and reliability of your apps.
In the next article, we’ll learn how to adapt Flutter apps to different platforms, specifically focusing on Android and iOS. We will discuss the key differences between these platforms and learn how to design platform-specific user interfaces. Keep fluttering!
Subscribe to my newsletter
Read articles from Vatsal Bhesaniya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
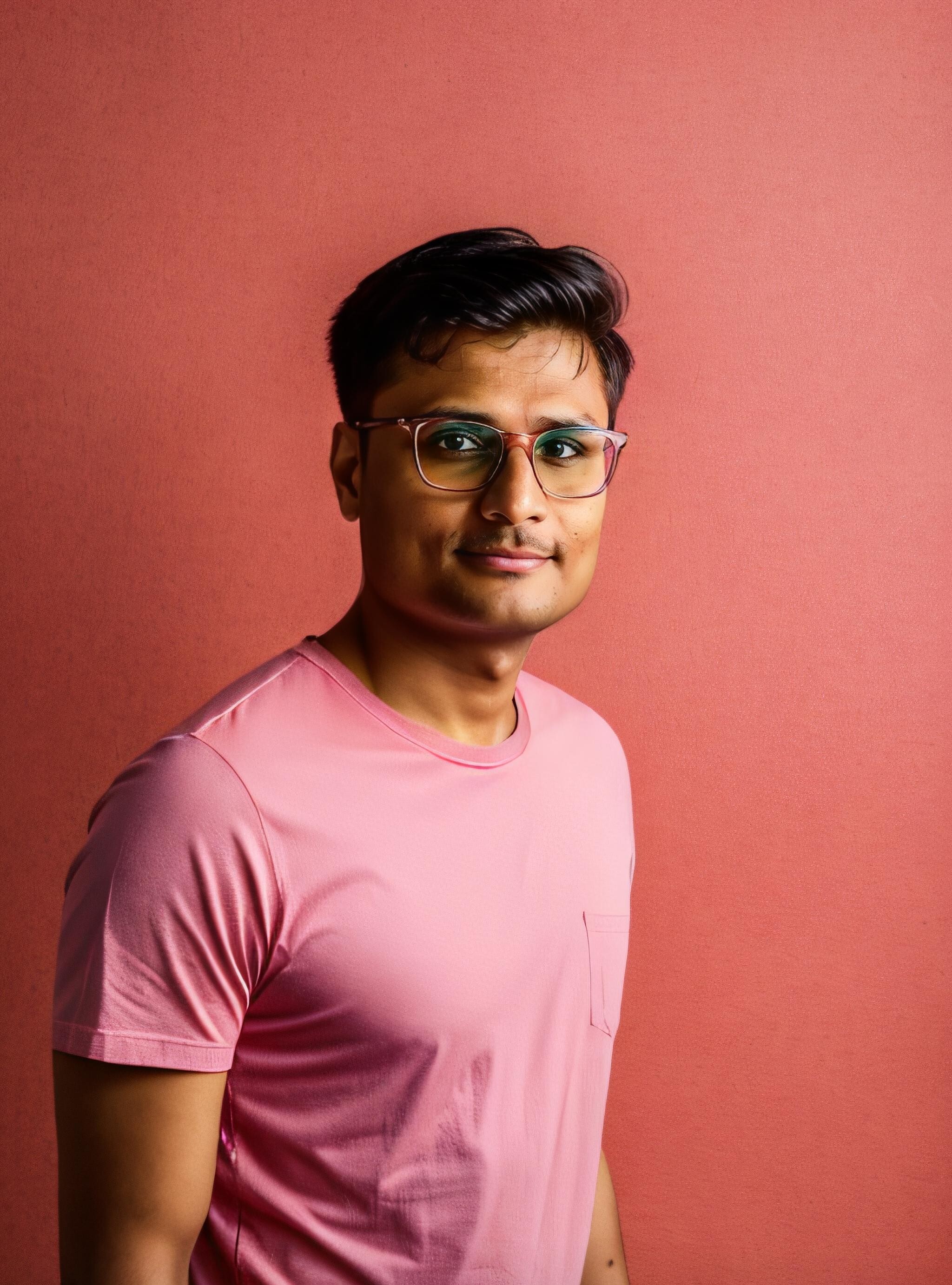