Technical Comparison between String and StringBuilder in Java
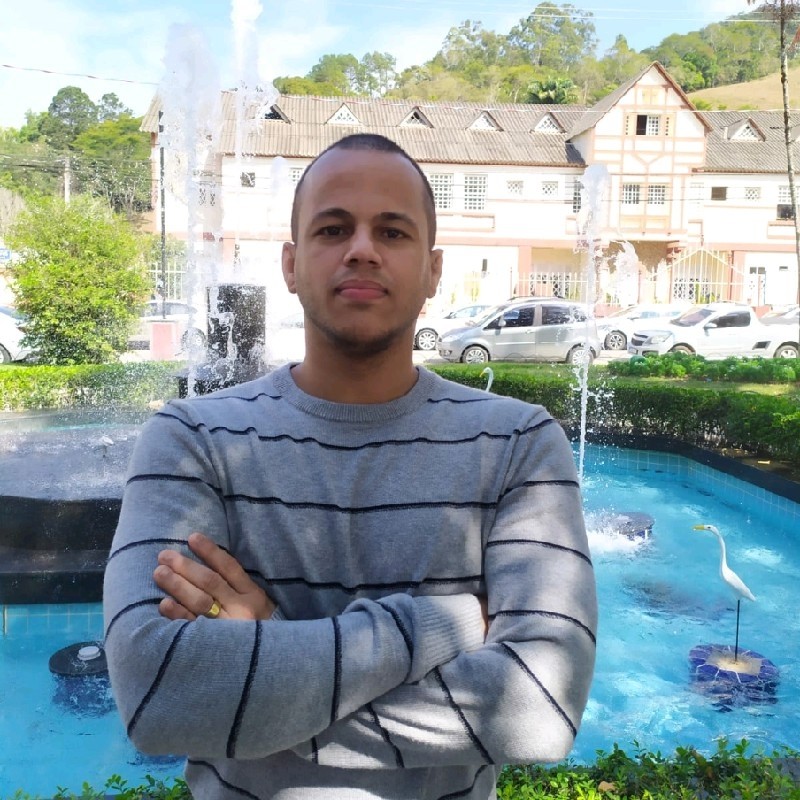
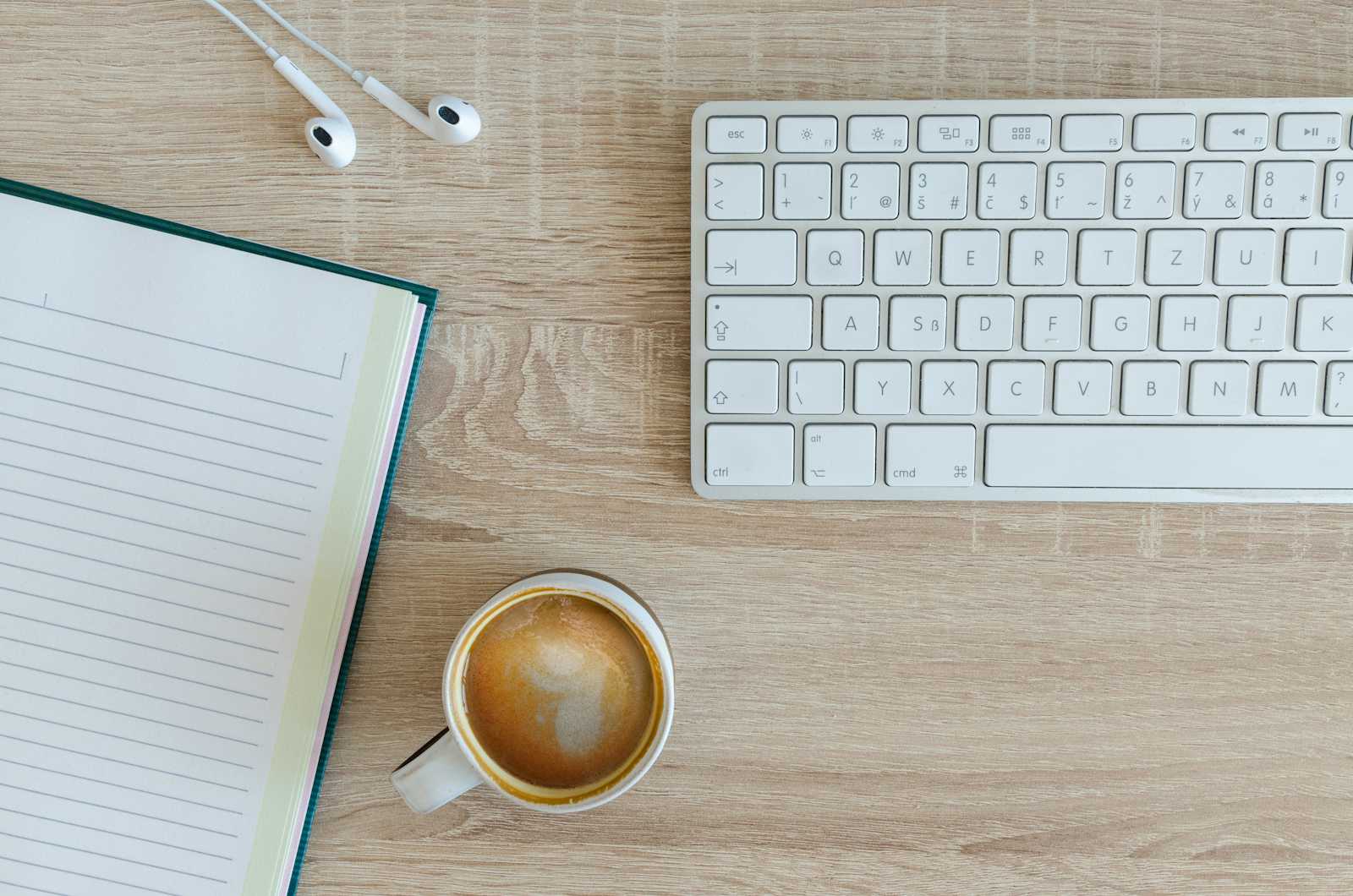
Introduction
In Java software development, the choice between String
and StringBuilder
can significantly impact the efficiency and performance of an application. Both classes have distinct characteristics and appropriate use cases. In this article, we will delve into the technical aspects of String
and StringBuilder
, explore their differences, and internal workings, and provide practical examples.
String
The String
class in Java represents a sequence of characters and is immutable. This means that once created, the character sequence cannot be altered. Let's explore more about the technical characteristics of String
:
Immutability
The immutability of Strings
ensures that once an instance is created, its value cannot be changed. Every operation that modifies the String
creates a new instance. This is useful for data security and integrity, especially in multi-threaded environments.
String Pool
Java uses a string pool to save memory. The String Pool
is a set of strings stored in memory that are reused. When a new String
is created, the JVM checks the pool to see if a string with the same value already exists. If it does, the reference to that string is returned, avoiding the creation of new objects.
String Usage Examples
Example 1: Simple Concatenation
String s1 = "Hello";
String s2 = "World";
String s3 = s1 + " " + s2;
System.out.println(s3); // Output: Hello World
Example 2: Concatenation in Loop
String result = "";
for (int i = 0; i < 1000; i++) {
result += "text";
}
System.out.println(result.length()); // Output: 4000
Internal Working
In Example 2, each iteration of the loop creates a new String
object, which is inefficient in terms of memory and performance. The JVM uses the String Pool
to manage string reuse, but frequent concatenation operations can lead to the creation of many temporary objects.
StringBuilder
The StringBuilder
class is mutable and designed for use when a string needs to be modified frequently. Unlike String
, StringBuilder
allows the modification of its character sequence without creating new objects, resulting in better performance for certain operations.
Mutability
StringBuilder
allows direct modification of the string content, avoiding the creation of new objects and saving memory. It is especially useful in loops or frequent concatenation operations.
Usage and Benefits
StringBuilder
is more efficient in terms of memory and performance for intensive string manipulation operations, such as concatenating many strings in a loop.
StringBuilder Usage Examples
Example 1: Simple Concatenation
StringBuilder sb = new StringBuilder();
sb.append("Hello");
sb.append(" ");
sb.append("World");
System.out.println(sb.toString()); // Output: Hello World
Example 2: Concatenation in Loop
StringBuilder result = new StringBuilder();
for (int i = 0; i < 1000; i++) {
result.append("text");
}
System.out.println(result.length()); // Output: 4000
Internal Working
In Example 2, StringBuilder
directly modifies its internal buffer, avoiding the creation of new objects for each concatenation, resulting in a much more efficient operation.
Technical Comparison
Immutability vs Mutability
String: Immutable, suitable for constant text and security in multi-threaded contexts.
StringBuilder: Mutable, ideal for dynamic string construction and repetitive concatenation operations.
Memory Management
String: Uses the
String Pool
to save memory, but can be inefficient in intensive modification operations.StringBuilder: Avoids creating multiple temporary objects, resulting in better performance and memory usage.
Performance
String: Can be inefficient in concatenation loops due to the creation of new objects.
StringBuilder: Much more efficient in repetitive string modification operations.
Complex Example
Example 1: Text Manipulation with String
public class StringExample {
public static void main(String[] args) {
String text = "Initial Text";
for (int i = 0; i < 1000; i++) {
text = text.replace("Initial", "Modified");
}
System.out.println(text); // Output: Modified Text
}
}
Example 2: Text Manipulation with StringBuilder
public class StringBuilderExample {
public static void main(String[] args) {
StringBuilder text = new StringBuilder("Initial Text");
for (int i = 0; i < 1000; i++) {
int start = text.indexOf("Initial");
int end = start + "Initial".length();
text.replace(start, end, "Modified");
}
System.out.println(text.toString()); // Output: Modified Text
}
}
Memory Explanation
String: In the
String
example, each loop iteration creates a newString
object, resulting in higher memory consumption and possibly more frequent garbage collection pauses.StringBuilder: In the
StringBuilder
example, the internal buffer is modified directly, resulting in lower memory consumption and better overall performance.
Conclusion
The choice between String
and StringBuilder
depends on the specific needs of the application. For frequent concatenation or text modification operations, StringBuilder
is the better choice due to its efficiency. However, for constant and immutable text, String
is preferable for its security and simplicity.
By understanding the technical characteristics and appropriate use cases for each class, developers can optimize their Java applications for better performance and memory usage.
Subscribe to my newsletter
Read articles from André Felipe Costa Bento directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
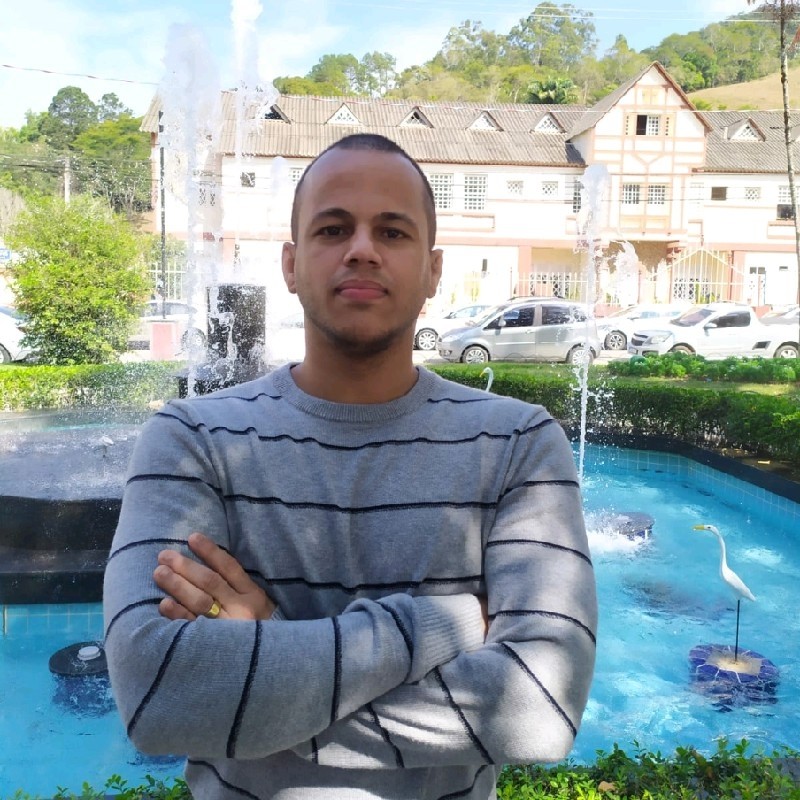
André Felipe Costa Bento
André Felipe Costa Bento
Fullstack Software Engineer.