Keep Your Data Organized: An Easy Introduction to Data Partitioning (SQL)
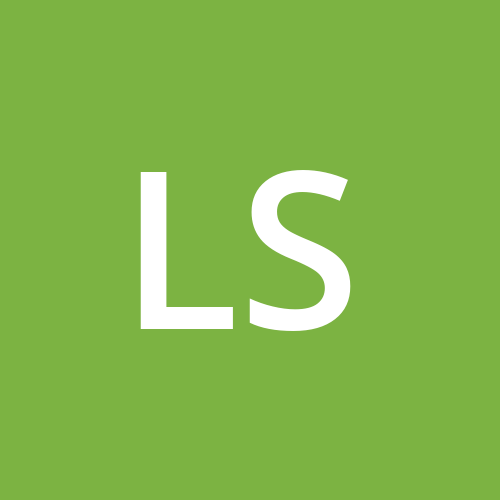
Think of partitioning like organizing your closet by seasons. Instead of having all your clothes jumbled together, you divide them into sections: summer, winter, spring, and fall. This way, when you need a summer outfit, you only look in the summer section. It’s faster and more efficient. Partitioning your data does the same thing for your database.
So, we can say - data partitioning is a technique used in databases to divide large tables into smaller, more manageable pieces, called partitions. This approach can significantly improve performance, scalability, and manageability, especially when dealing with large datasets.
Why Use Data Partitioning?
Performance Improvement: By breaking a large table into smaller partitions, queries that access a subset of data can be executed faster because they scan only relevant partitions instead of the entire table.
Manageability: Maintenance tasks like backups, restores, and data purges can be performed more efficiently on smaller partitions rather than on a single large table.
Scalability: Partitioning helps in handling large volumes of data, making it easier to scale databases horizontally.
Types of Partitioning in MySQL
Range Partitioning: Data is divided based on a range of values. For example, partitioning a sales table by year.
PARTITION BY RANGE (YEAR(order_date)) ( PARTITION p0 VALUES LESS THAN (2000), PARTITION p1 VALUES LESS THAN (2010), PARTITION p2 VALUES LESS THAN (2020), PARTITION p3 VALUES LESS THAN MAXVALUE );
List Partitioning: Similar to range partitioning but based on a predefined list of values.
PARTITION BY LIST (country) ( PARTITION usa VALUES IN ('USA'), PARTITION canada VALUES IN ('Canada'), PARTITION uk VALUES IN ('UK'), PARTITION other VALUES IN ('Germany', 'France', 'Australia', 'Other') );
Hash Partitioning: Data is divided based on a hash function. This is useful for evenly distributing data across partitions.
PARTITION BY HASH(customer_id) PARTITIONS 4;
Key Partitioning: Similar to hash partitioning but uses MySQL’s internal function to generate the partition key.
PARTITION BY KEY(customer_id) PARTITIONS 4;
How to Implement Data Partitioning
Let’s walk through a basic example of range partitioning on a sales
table.
Step 1: Create a Partitioned Table
CREATE TABLE sales (
sale_id INT AUTO_INCREMENT,
sale_date DATE,
amount DECIMAL(10, 2),
PRIMARY KEY (sale_id, sale_date)
)
PARTITION BY RANGE (YEAR(sale_date)) (
PARTITION p0 VALUES LESS THAN (2018),
PARTITION p1 VALUES LESS THAN (2019),
PARTITION p2 VALUES LESS THAN (2020),
PARTITION p3 VALUES LESS THAN (2021),
PARTITION p4 VALUES LESS THAN (2022),
PARTITION p5 VALUES LESS THAN MAXVALUE
);
In this example:
The
sales
table is partitioned by the year of thesale_date
.Each partition holds data for a specific range of years.
Step 2: Insert Data
INSERT INTO sales (sale_date, amount) VALUES ('2017-05-15', 100.00);
INSERT INTO sales (sale_date, amount) VALUES ('2018-03-20', 200.00);
INSERT INTO sales (sale_date, amount) VALUES ('2019-07-22', 150.00);
Step 3: Query the Partitioned Table
When you query the table, MySQL uses partition pruning to access only the relevant partitions:
SELECT * FROM sales WHERE sale_date BETWEEN '2018-01-01' AND '2019-12-31';
This query will primarily access the partitions for 2018 and 2019 which are p0
and p1
.
EXPLAIN SELECT * FROM sales WHERE sale_date BETWEEN '2018-01-01' AND '2019-12-31';
The EXPLAIN
output might show that the query scans p0
and p1
partitions.
Managing Partitions
Adding Partitions: Add new partitions as data grows.
ALTER TABLE sales ADD PARTITION ( PARTITION p6 VALUES LESS THAN (2023) );
Dropping Partitions: Remove old partitions to archive or delete data.
ALTER TABLE sales DROP PARTITION p0;
Potential Challenges
Query Performance: Queries that do not use the partition key may not benefit from partition pruning, potentially leading to full partition scans.
Complexity: Designing the right partitioning strategy requires understanding your data access patterns.
Maintenance: Regular maintenance is needed to manage partitions effectively, including splitting, merging, and monitoring partition sizes.
Conclusion
Mastering data partitioning in MySQL is a crucial step toward optimizing your database for performance and scalability. By effectively dividing your data into partitions, you can enhance query speeds, streamline maintenance tasks, and better manage large volumes of data. Whether you're using range, list, or hash partitioning, the key is to start with a straightforward approach and refine your strategy as you gain deeper insights into your data and usage patterns. With thoughtful implementation, data partitioning can transform your database into a more efficient and robust system.
Subscribe to my newsletter
Read articles from Liton Chandra Shil directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
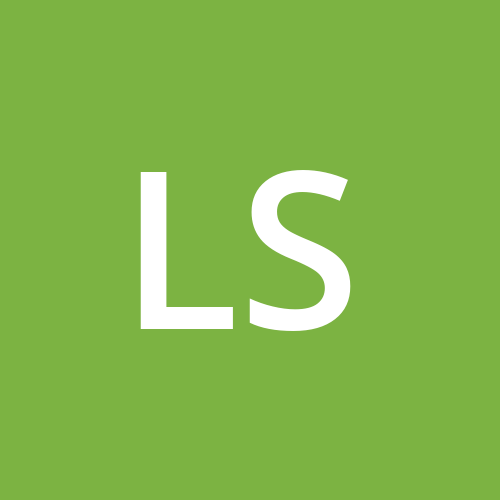