Python GUI Development with Tkinter: Building Interactive Applications
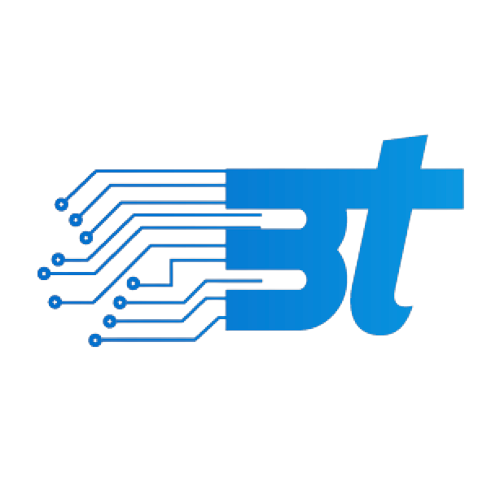
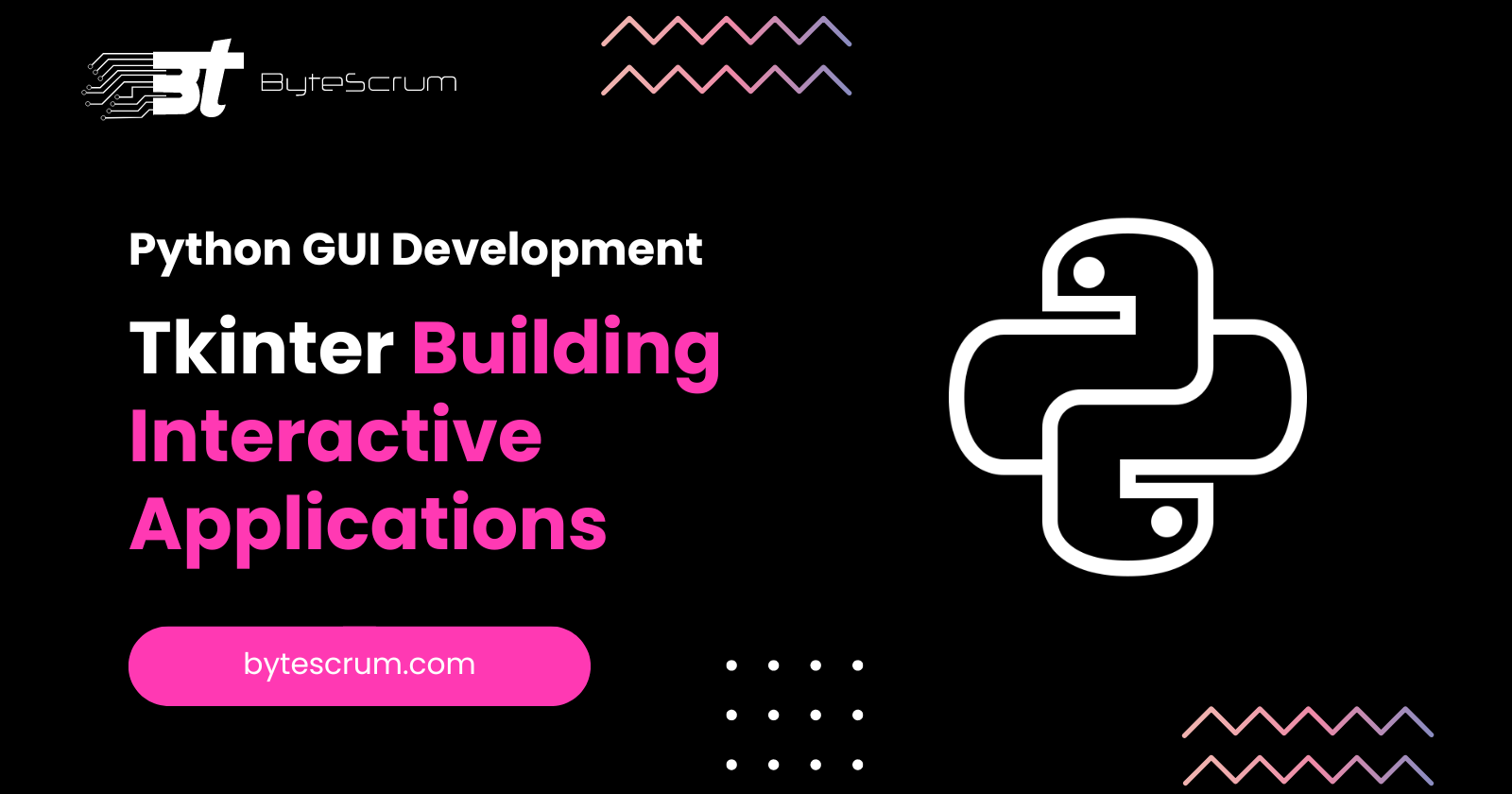
Graphical User Interfaces (GUIs) are essential for creating interactive applications that enhance user experience. Python’s Tkinter library is a powerful tool for building desktop applications with a native look and feel. In this guide, we'll explore how to use Tkinter to develop a variety of GUI applications, from simple forms to more complex interfaces.
1. Getting Started with Tkinter
Tkinter comes bundled with Python, making it easy to start building GUIs. The following example demonstrates a basic Tkinter window.
1.1. Creating a Basic Window:
This script creates a simple window with a title and dimensions.
import tkinter as tk
def create_window():
window = tk.Tk()
window.title("Simple Tkinter Window")
window.geometry("400x300")
window.mainloop()
# Usage
create_window()
1.2. Adding Widgets:
Widgets are the building blocks of a Tkinter application. This example adds a label and a button to the window.
import tkinter as tk
def create_window():
window = tk.Tk()
window.title("Simple Tkinter Window")
window.geometry("400x300")
label = tk.Label(window, text="Hello, Tkinter!")
label.pack(pady=10)
button = tk.Button(window, text="Click Me", command=lambda: label.config(text="Button Clicked"))
button.pack(pady=10)
window.mainloop()
# Usage
create_window()
2. Building Forms with Tkinter
Forms are common in GUI applications for collecting user input. Tkinter provides various widgets to create forms, including labels, entry fields, and buttons.
2.1. Creating a Simple Form:
This example demonstrates a basic form with labels and entry fields.
import tkinter as tk
def create_form():
window = tk.Tk()
window.title("Simple Form")
window.geometry("400x300")
tk.Label(window, text="Name:").grid(row=0, column=0, padx=10, pady=10)
name_entry = tk.Entry(window)
name_entry.grid(row=0, column=1, padx=10, pady=10)
tk.Label(window, text="Email:").grid(row=1, column=0, padx=10, pady=10)
email_entry = tk.Entry(window)
email_entry.grid(row=1, column=1, padx=10, pady=10)
def submit_form():
name = name_entry.get()
email = email_entry.get()
print(f"Name: {name}, Email: {email}")
submit_button = tk.Button(window, text="Submit", command=submit_form)
submit_button.grid(row=2, column=1, padx=10, pady=10)
window.mainloop()
# Usage
create_form()
3. Advanced Widgets and Layouts
Tkinter offers advanced widgets like Listbox
, Treeview
, and Canvas
, along with layout managers like pack
, grid
, and place
for more complex interfaces.
3.1. Using theListbox
Widget:
This script demonstrates how to create and interact with a Listbox
.
import tkinter as tk
def create_listbox():
window = tk.Tk()
window.title("Listbox Example")
window.geometry("400x300")
listbox = tk.Listbox(window)
listbox.pack(pady=20)
items = ["Item 1", "Item 2", "Item 3", "Item 4"]
for item in items:
listbox.insert(tk.END, item)
def on_select(event):
selected_item = listbox.get(listbox.curselection())
print(f"Selected: {selected_item}")
listbox.bind('<<ListboxSelect>>', on_select)
window.mainloop()
# Usage
create_listbox()
3.2. Creating aTreeview
Widget:
Treeview
is useful for displaying hierarchical data. This example shows how to create a simple Treeview
.
import tkinter as tk
from tkinter import ttk
def create_treeview():
window = tk.Tk()
window.title("Treeview Example")
window.geometry("400x300")
tree = ttk.Treeview(window)
tree.pack(pady=20)
tree['columns'] = ('Name', 'Age')
tree.column("#0", width=0, stretch=tk.NO)
tree.column("Name", anchor=tk.W, width=120)
tree.column("Age", anchor=tk.W, width=120)
tree.heading("#0", text="", anchor=tk.W)
tree.heading("Name", text="Name", anchor=tk.W)
tree.heading("Age", text="Age", anchor=tk.W)
tree.insert('', 'end', iid=0, text="", values=('John Doe', '25'))
tree.insert('', 'end', iid=1, text="", values=('Jane Doe', '30'))
window.mainloop()
# Usage
create_treeview()
4. Event Handling and Callbacks
Handling events and defining callbacks are essential for interactive applications. This section demonstrates how to manage events in Tkinter.
4.1. Button Click Events:
This example shows how to handle button click events.
import tkinter as tk
def create_button_event():
window = tk.Tk()
window.title("Button Event Example")
window.geometry("400x300")
def on_button_click():
print("Button was clicked!")
button = tk.Button(window, text="Click Me", command=on_button_click)
button.pack(pady=20)
window.mainloop()
# Usage
create_button_event()
4.2. Binding Keyboard Events:
This script binds a keyboard event to a function.
import tkinter as tk
def create_keyboard_event():
window = tk.Tk()
window.title("Keyboard Event Example")
window.geometry("400x300")
def on_key_press(event):
print(f"Key Pressed: {event.char}")
window.bind('<KeyPress>', on_key_press)
window.mainloop()
# Usage
create_keyboard_event()
5. Creating Menus and Dialogs
Menus and dialogs enhance the functionality of GUI applications. Tkinter provides easy ways to create these elements.
5.1. Creating a Menu:
This example shows how to add a menu to a Tkinter application.
import tkinter as tk
def create_menu():
window = tk.Tk()
window.title("Menu Example")
window.geometry("400x300")
menubar = tk.Menu(window)
file_menu = tk.Menu(menubar, tearoff=0)
file_menu.add_command(label="Open")
file_menu.add_command(label="Save")
file_menu.add_separator()
file_menu.add_command(label="Exit", command=window.quit)
menubar.add_cascade(label="File", menu=file_menu)
edit_menu = tk.Menu(menubar, tearoff=0)
edit_menu.add_command(label="Undo")
edit_menu.add_command(label="Redo")
menubar.add_cascade(label="Edit", menu=edit_menu)
window.config(menu=menubar)
window.mainloop()
# Usage
create_menu()
5.2. Creating Dialog Boxes:
This script demonstrates how to create a simple message box.
import tkinter as tk
from tkinter import messagebox
def create_messagebox():
window = tk.Tk()
window.title("Message Box Example")
window.geometry("400x300")
def show_message():
messagebox.showinfo("Information", "This is a message box")
button = tk.Button(window, text="Show Message", command=show_message)
button.pack(pady=20)
window.mainloop()
# Usage
create_messagebox()
Conclusion
Happy Coding!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
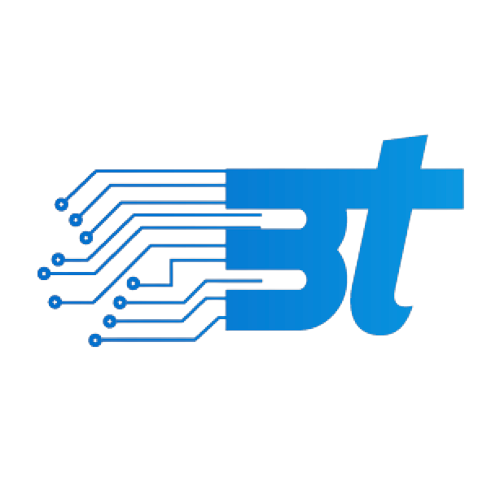
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.