Unleashing the Power of Arrays in JavaScript: A Comprehensive Guide
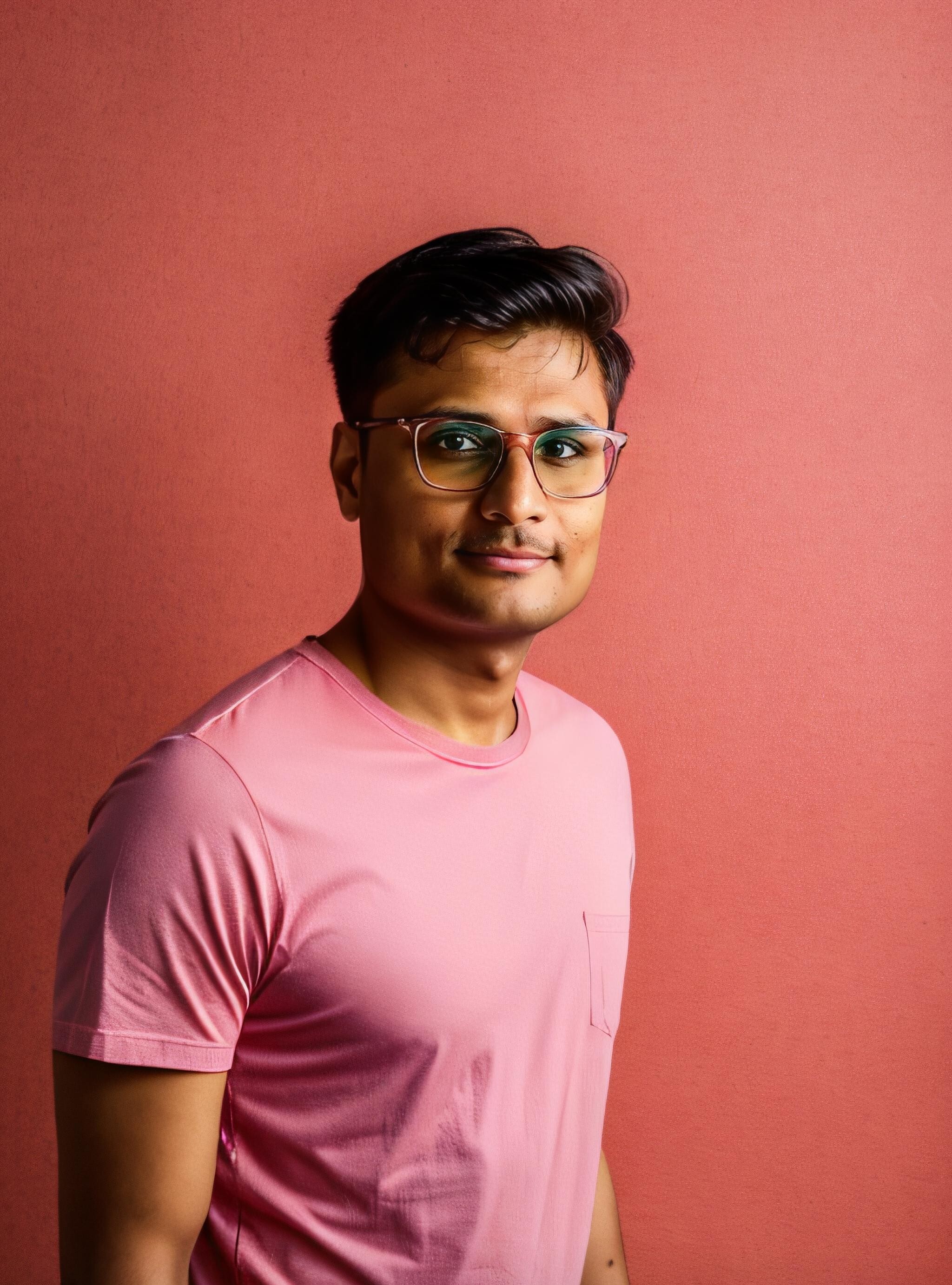
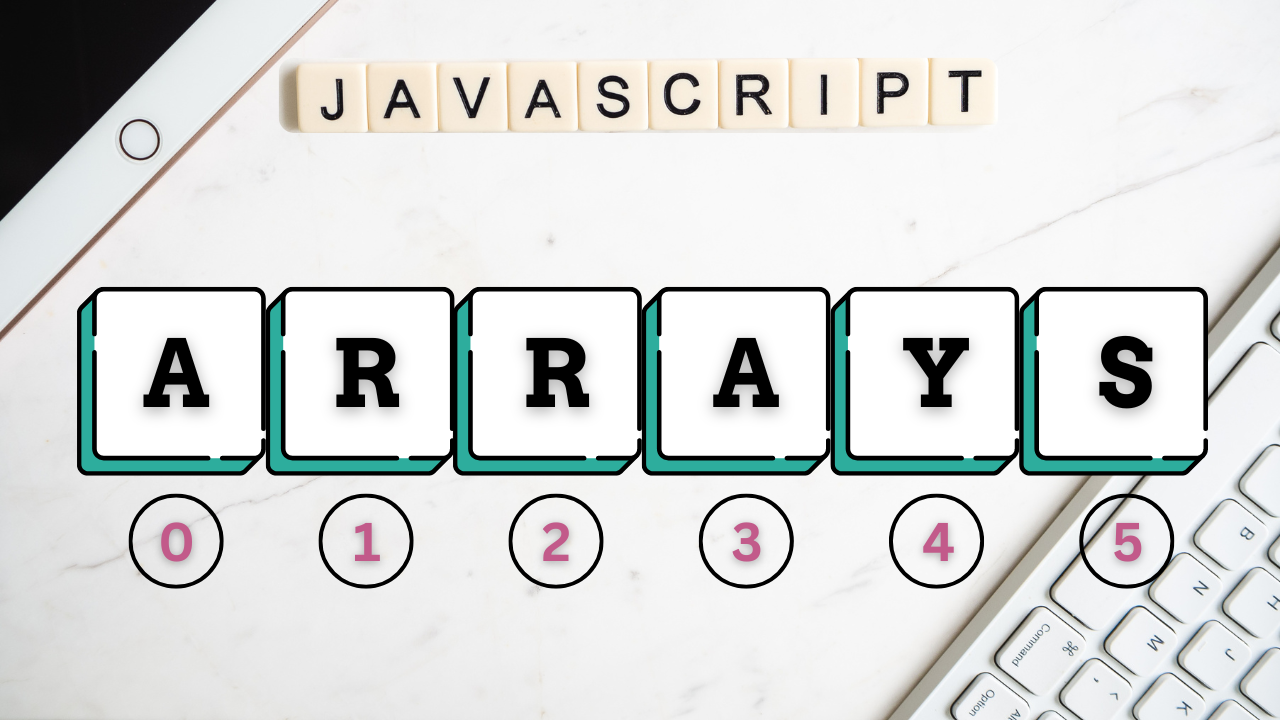
Arrays are data structures that allow you to store and manipulate collections of values. They are widely used in programming for organizing and working with related data.
We’ll build a practical web application example to demonstrate these array concepts in action. We’ll learn how to search for movies, add them to a watchlist, and filter movies based on ratings, all while utilizing the power of arrays in JavaScript.
So, get ready to dive deep into the world of arrays and unlock their potential in your JavaScript projects!
Creating and initializing arrays
In JavaScript, an array is a collection of values or elements.
You can create an array using the array literal notation, which involves enclosing the elements within square brackets
[]
.
// Creating an empty array
let fruits = [];
// Creating an array with initial values
let numbers = [1, 2, 3, 4, 5];
let mixed = [true, "hello", 42, null];
- You can also create an array using the
Array
constructor.
let numbers = new Array(1, 2, 3, 4, 5);
// Creates an array with 3 empty slots
let emptyArray = new Array(3);
Array properties and methods
- The
length
property returns the number of elements in an array.
let fruits = ["apple", "banana"];
console.log(fruits.length); // Output: 2
The
push()
method adds one or more elements to the end of an array.The
pop()
method removes the last element from an array.
let fruits = ["apple", "banana"];
fruits.push("orange"); // ["apple", "banana", "orange"]
let lastFruit = fruits.pop(); // ["apple", "banana"]
The
unshift()
method adds one or more elements to the beginning of an array.The
shift()
method removes the first element from an array.
let fruits = ["apple", "banana"];
fruits.unshift("kiwi"); // ["kiwi", "apple", "banana"]
let firstFruit = fruits.shift(); // ["apple", "banana"]
The
concat()
method merges two or more arrays and returns a new array.The
slice()
method returns a shallow copy of a portion of an array.
let fruits1 = ["apple", "banana"];
let fruits2 = ["orange", "kiwi"];
let allFruits = fruits1.concat(fruits2); // ["apple", "banana", "orange", "kiwi"]
let someFruits = allFruits.slice(1, 3); // ["banana", "orange"]
- The
splice()
method can add or remove elements from an array.
let fruits = ["apple", "banana", "orange"];
fruits.splice(1, 1, "mango", "mango"); // ["apple", "mango", "mango", "orange"]
The
indexOf()
method returns the first index at which a given element is found in the array, or -1 if it's not present.The
lastIndexOf()
method returns the last index at which a given element is found in the array, or -1 if it's not present.
console.log(fruits.indexOf("mango")); // Output: 1
console.log(fruits.lastIndexOf("mango")); // Output: 2
The
forEach()
method executes a provided function once for each element of the array.The
map()
method creates a new array with the results of calling a provided function on every element of the array.The
filter()
method creates a new array with all elements that pass the test implemented by the provided function.The
reduce()
method applies a function against an accumulator and each element of the array to reduce it to a single value.
let numbers = [1, 2, 3, 4, 5];
numbers.forEach(function(num) {
console.log(num * 2);
});// Output: 2, 4, 6, 8, 10
let doubledNumbers = numbers.map(function(num) {
return num * 2;
});// [2, 4, 6, 8, 10]
let evenNumbers = numbers.filter(function(num) {
return num % 2 === 0;
});// [2, 4]
let sum = numbers.reduce(function(accumulator, num) {
return accumulator + num;
}, 0);// 15
Array destructuring
- Array destructuring is a way to unpack values from an array into distinct variables.
let fruits = ["apple", "banana", "orange"];
let [firstFruit, secondFruit, thirdFruit] = fruits;
console.log(firstFruit);// Output: "apple"
console.log(secondFruit);// Output: "banana"
console.log(thirdFruit);// Output: "orange"
Spread operator with arrays
- The spread operator (
...
) allows you to spread the elements of an array into individual elements.
let fruits1 = ["apple", "banana"];
let fruits2 = ["orange", "kiwi"];
let allFruits = [...fruits1, ...fruits2];
console.log(allFruits); // Output: ["apple", "banana", "orange", "kiwi"]
// Copying an array
let originalFruits = ["apple", "banana", "orange"];
let copiedFruits = [...originalFruits];
console.log(copiedFruits); // Output: ["apple", "banana", "orange"]
Example (Movie recommendation app)
let’s create a simple web application that demonstrates the different Array concepts and methods in a real-world context. We’ll build a movie recommendation app that allows users to search for movies, add movies to their watchlist, and filter movies based on ratings.
Here’s the HTML structure for our web app:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Movie Recommendation App</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<h1>Movie Recommendation App</h1>
<input type="text" id="searchInput" placeholder="Search for movies...">
<button id="searchBtn" class="button">Search</button>
<h2>Search Results</h2>
<ul id="searchResults"></ul>
<h2>Watchlist</h2>
<ul id="watchlist"></ul>
<h2>Top Rated Movies</h2>
<ul id="topRatedMovies"></ul>
<script src="app.js"></script>
</body>
</html>
Here is the CSS for styling our web app.
body {
background-color: #1B2430;
color: white;
font-family: Arial, sans-serif;
margin: 20px;
}
h1 {
color: #D6D5A8;
}
h2 {
color: #816797;
}
input {
padding: 5px;
font-size: 16px;
border-radius: 5px;
border: none;
margin-bottom: 10px;
} :focus {
outline: none;
}
ul {
list-style-type: none;
padding: 0;
}
li {
margin-bottom: 10px;
}
.button {
padding: 5px;
font-size: 16px;
background-color: #D6D5A8;
color: black;
border: none;
padding: 5px 10px;
cursor: pointer;
margin-left: 10px;
border-radius: 5px;
}
And here’s the JavaScript code (app.js
) that demonstrates various array concepts and methods:
// Sample movie data
const movies = [
{ title: 'The Shawshank Redemption', rating: 9.3 },
{ title: 'The Godfather', rating: 9.2 },
{ title: 'The Dark Knight', rating: 9.0 },
{ title: 'Pulp Fiction', rating: 8.9 },
{ title: 'Forrest Gump', rating: 8.8 },
{ title: 'Inception', rating: 8.8 },
{ title: 'The Matrix', rating: 8.7 },
{ title: 'Interstellar', rating: 8.6 },
{ title: 'The Departed', rating: 8.5 },
{ title: 'Fight Club', rating: 8.8 }
];
// Creating and initializing arrays
let watchlist = [];
let topRatedMovies = [];
// Array properties and methods
const searchInput = document.getElementById('searchInput');
const searchBtn = document.getElementById('searchBtn');
const searchResults = document.getElementById('searchResults');
const watchlistElement = document.getElementById('watchlist');
const topRatedMoviesElement = document.getElementById('topRatedMovies');
searchBtn.addEventListener('click', function() {
const searchTerm = searchInput.value.toLowerCase();
const filteredMovies = movies.filter(movie =>
movie.title.toLowerCase().includes(searchTerm)
);
searchResults.innerHTML = '';
filteredMovies.forEach(movie => {
const li = document.createElement('li');
li.textContent = `${movie.title} (Rating: ${movie.rating})`;
const addToWatchlistBtn = document.createElement('button');
addToWatchlistBtn.textContent = 'Add to Watchlist';
addToWatchlistBtn.addEventListener('click', function() {
watchlist.push(movie);
renderWatchlist();
});
addToWatchlistBtn.classList.add('button');
li.appendChild(addToWatchlistBtn);
searchResults.appendChild(li);
});
});
function renderWatchlist() {
watchlistElement.innerHTML = '';
watchlist.forEach(movie => {
const li = document.createElement('li');
li.textContent = `${movie.title} (Rating: ${movie.rating})`;
watchlistElement.appendChild(li);
});
}
// Array destructuring
const [firstMovie, secondMovie, ...otherMovies] = movies;
console.log('First movie:', firstMovie);
console.log('Second movie:', secondMovie);
console.log('Other movies:', otherMovies);
// Spread operator with arrays
const topRatedMoviesCopy = [...movies];
topRatedMovies = topRatedMoviesCopy.filter(movie => movie.rating >= 9.0);
topRatedMoviesElement.innerHTML = '';
topRatedMovies.forEach(movie => {
const li = document.createElement('li');
li.textContent = `${movie.title} (Rating: ${movie.rating})`;
topRatedMoviesElement.appendChild(li);
});
This is how our web app looks like.
Now, let’s understand the web application example code.
// Sample movie data
const movies = [
{ title: 'The Shawshank Redemption', rating: 9.3 },
{ title: 'The Godfather', rating: 9.2 },
{ title: 'The Dark Knight', rating: 9.0 },
{ title: 'Pulp Fiction', rating: 8.9 },
{ title: 'Forrest Gump', rating: 8.8 },
{ title: 'Inception', rating: 8.8 },
{ title: 'The Matrix', rating: 8.7 },
{ title: 'Interstellar', rating: 8.6 },
{ title: 'The Departed', rating: 8.5 },
{ title: 'Fight Club', rating: 8.8 }
];
// Creating and initializing arrays
let watchlist = [];
let topRatedMovies = [];
Here I have created an array (“movies”), containing sample movie data with titles and ratings. We also initialize two empty arrays: watchlist
and topRatedMovies
.
// Array properties and methods
const searchInput = document.getElementById('searchInput');
const searchBtn = document.getElementById('searchBtn');
const searchResults = document.getElementById('searchResults');
const watchlistElement = document.getElementById('watchlist');
const topRatedMoviesElement = document.getElementById('topRatedMovies');
Here we select dom elements using getElementById
and store them in variables.
searchBtn.addEventListener('click', function() {
const searchTerm = searchInput.value.toLowerCase();
const filteredMovies = movies.filter(movie =>
movie.title.toLowerCase().includes(searchTerm)
);
...
});
When the search button is clicked, we get the search term from the input field and use the filter
method to create a new array filteredMovies
containing movies whose titles include the search term.
searchBtn.addEventListener('click', function() {
...
searchResults.innerHTML = '';
filteredMovies.forEach(movie => {
const li = document.createElement('li');
li.textContent = `${movie.title} (Rating: ${movie.rating})`;
const addToWatchlistBtn = document.createElement('button');
addToWatchlistBtn.textContent = 'Add to Watchlist';
addToWatchlistBtn.addEventListener('click', function() {
watchlist.push(movie);
renderWatchlist();
});
addToWatchlistBtn.classList.add('button');
li.appendChild(addToWatchlistBtn);
searchResults.appendChild(li);
});
});
We then loop through filteredMovies
using forEach
and create list items for each movie. Finally, we append them to the searchResults
list.
For each movie in the search results, we create an “Add to Watchlist” button that, when clicked, adds the movie to the watchlist
array using the push
method.
function renderWatchlist() {
watchlistElement.innerHTML = '';
watchlist.forEach(movie => {
const li = document.createElement('li');
li.textContent = `${movie.title} (Rating: ${movie.rating})`;
watchlistElement.appendChild(li);
});
}
The renderWatchlist
function is called to update the watchlist display by creating list items for each movie in the watchlist
array.
// Array destructuring
const [firstMovie, secondMovie, ...otherMovies] = movies;
console.log('First movie:', firstMovie);
console.log('Second movie:', secondMovie);
console.log('Other movies:', otherMovies);
Here is the Array destructuring part. We use array destructuring to unpack the first two movies from the movies
array into separate variables: firstMovie
and secondMovie
. The rest of the movies are unpacked into a new array (otherMovies) using the rest syntax. We log these variables to the console to demonstrate array restructuring.
Now let’s look at how the Spread operator is used with arrays.
// Spread operator with arrays
const topRatedMoviesCopy = [...movies];
topRatedMovies = topRatedMoviesCopy.filter(movie => movie.rating >= 9.0);
topRatedMoviesElement.innerHTML = '';
topRatedMovies.forEach(movie => {
const li = document.createElement('li');
li.textContent = `${movie.title} (Rating: ${movie.rating})`;
topRatedMoviesElement.appendChild(li);
});
Here I have created a new array topRatedMoviesCopy
by spreading the elements of the movies
array using the spread operator.
We then use the filter
method on (topRatedMoviesCopy) to create a new array (topRatedMovies), containing only movies with a rating of 9.0 or higher.
Finally, we loop through topRatedMovies using (for Each) and create list items for each movie, appending them to the (topRatedMovies) list.
Creating and initializing arrays:
We start by creating an array
movies
containing sample movie data (title and rating).We also initialize two empty arrays:
watchlist
andhighlyRatedMovies
.
Array properties and methods:
We select DOM elements using
getElementById
and store them in variables.When the search button is clicked, we get the search term from the input field and use the
filter
method to create a new arrayfilteredMovies
containing movies whose titles include the search term (case-insensitive).We then loop through
filteredMovies
usingforEach
and create list items (li
) for each movie, appending them to thesearchResults
list.For each movie in the search results, we create an “Add to Watchlist” button that, when clicked, adds the movie to the
watchlist
array using thepush
method.The
renderWatchlist
function is called to update the watchlist display by creating list items for each movie in thewatchlist
array.
Array destructuring:
We use array destructuring to unpack the first two movies from the
movies
array into separate variablesfirstMovie
andsecondMovie
.The rest of the movies are unpacked into a new array
otherMovies
using the rest syntax (...
).We log these variables to the console to demonstrate array destructuring.
Spread operator with arrays:
We create a new array
highlyRatedMoviesCopy
by spreading the elements of themovies
array using the spread operator (...
).We then use the
filter
method onhighlyRatedMoviesCopy
to create a new arrayhighlyRatedMovies
containing only movies with a rating of 9.0 or higher.We loop through
highlyRatedMovies
usingforEach
and create list items for each movie, appending them to thehighlyRatedMovies
list.
So, we explored everything from creating and initializing them to mastering advanced techniques like destructuring and spread operators. It’s time to dive into another powerful data structure: objects. In the next article, we’ll explore the world of objects, which are used to store collections of key-value pairs and are fundamental for working with complex data structures and creating object-oriented programs.
Subscribe to my newsletter
Read articles from Vatsal Bhesaniya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
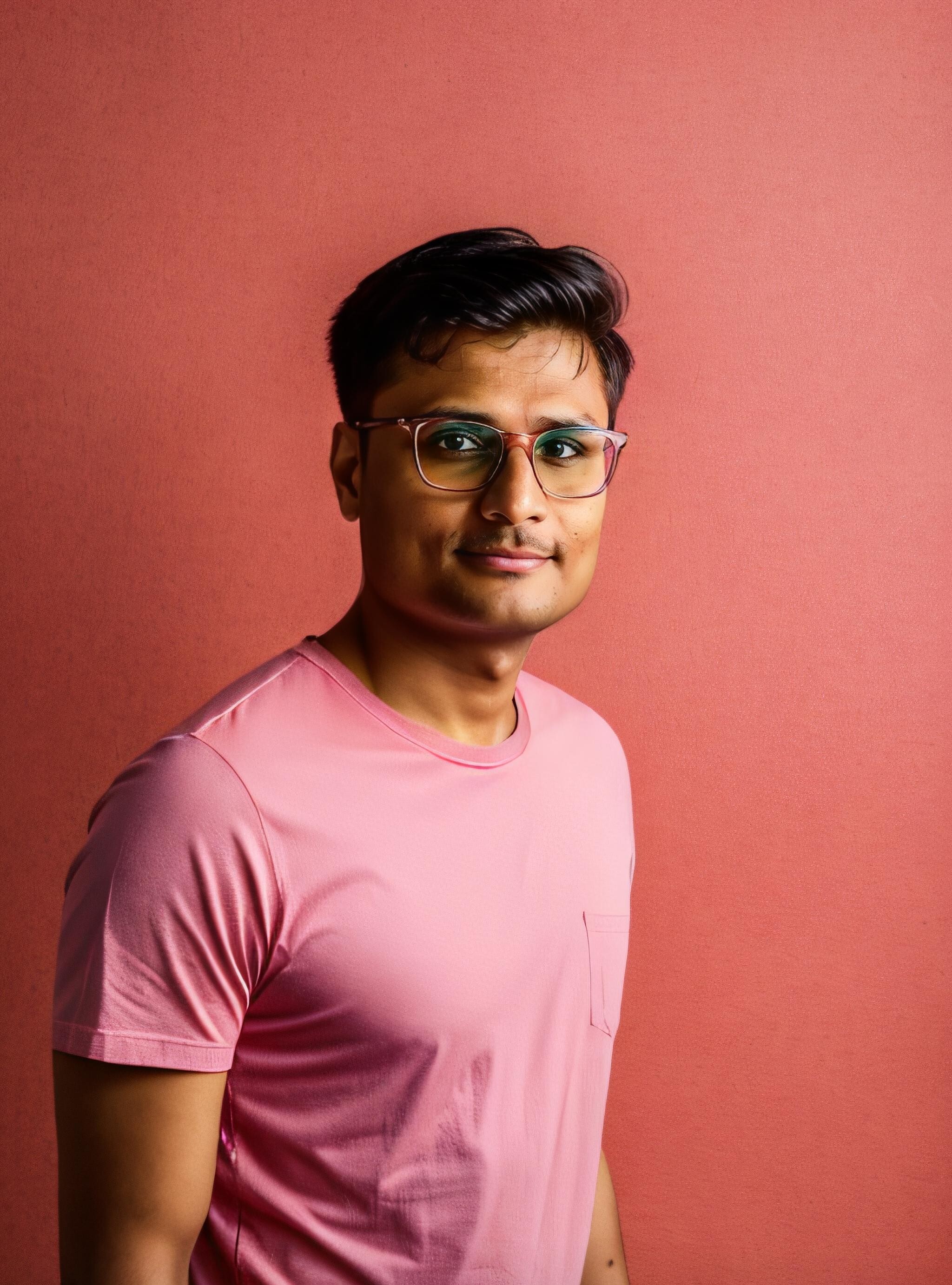