React JS Beginner to Advanced series (7)
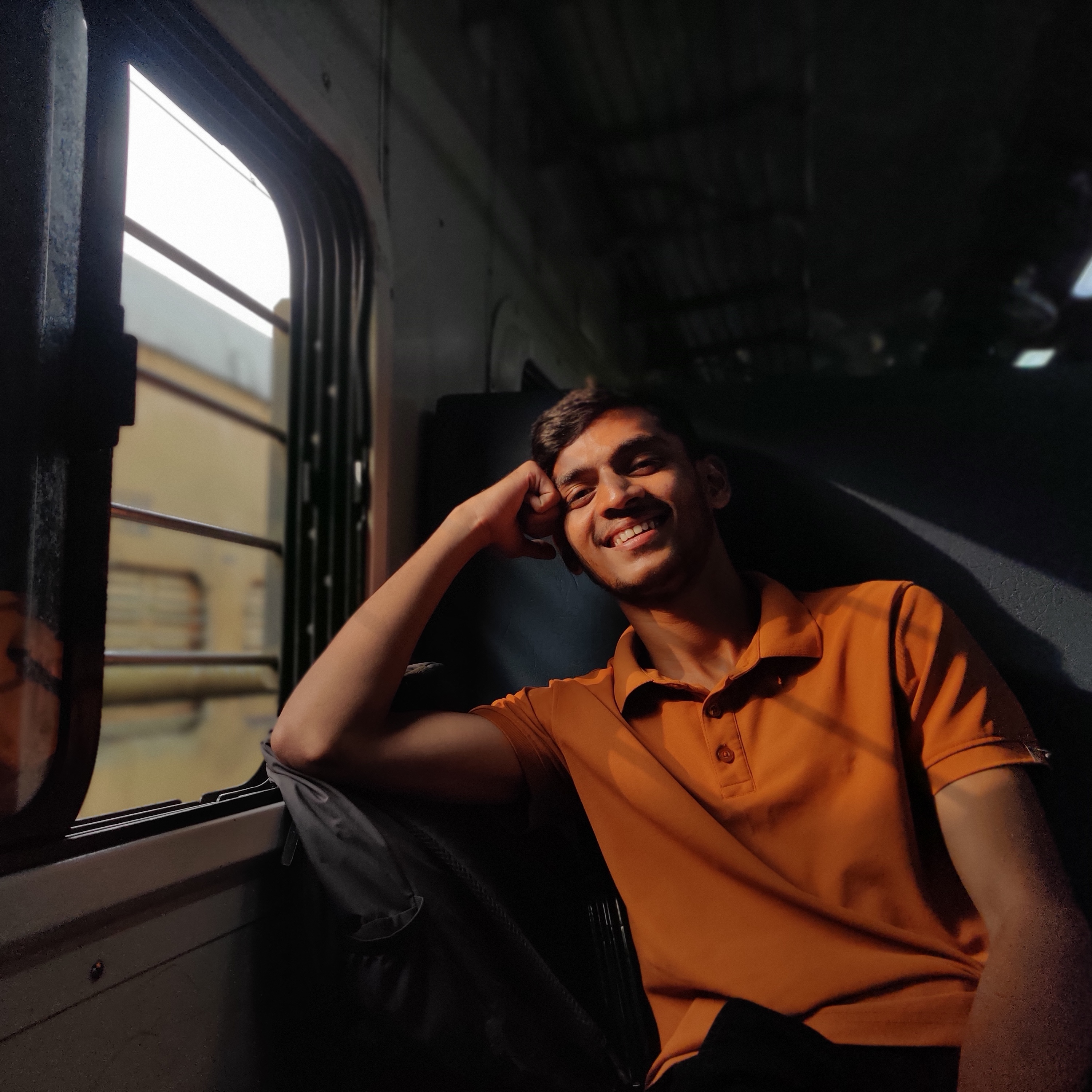
3 min read
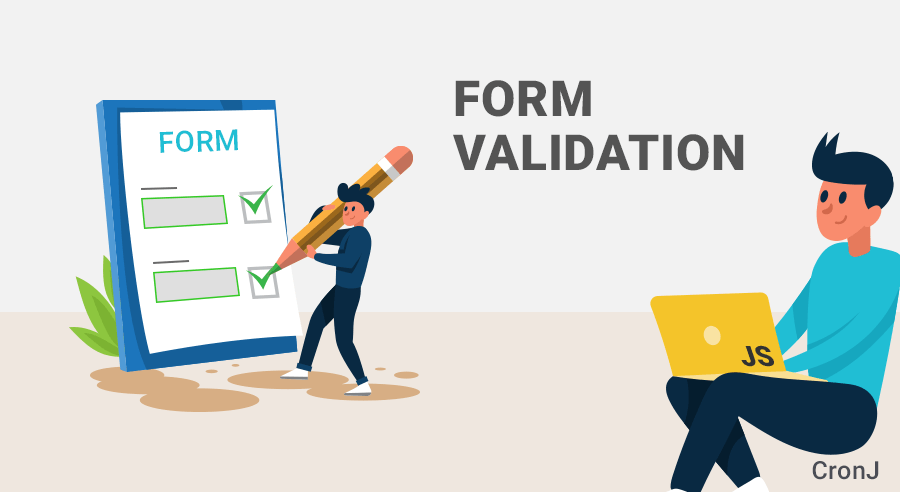
This is a 7th blog of this react series, if you didn't check out the previous one, go check now on my profile: ReactJS Beginner to Advanced Series
After discovering the previous one, we discuss "form validations" in ReactJS.
What is Form Validation ?
- Form validation is a technical process where a web-form checks if the information provided by a user is correct. This process is useful when a user needs to input information that meets specific rules and requirements.
- For example, when registering for a website, form validation ensures that the user provides all the required details and the information is accurate. If the user doesn't meet the requirements, the form data won't be accepted or stored on the server.
Form Validations in ReactJs:
- In a React project, you can implement user input form validations in various ways. Here are some common methods:
=> HTML5 Form Validation:
- Use built-in HTML5 validation attributes like required, pattern, minLength, maxLength, type, etc.
<form>
<input type="text" required pattern="\d+" title="Only numbers are allowed" />
<input type="submit" />
</form>
=> Manual Validation in Component State:
- Use React's state to manage form values and validation errors.
import React, { useState } from 'react';
const MyForm = () => {
const [values, setValues] = useState({ name: '' });
const [errors, setErrors] = useState({});
const handleChange = (e) => {
setValues({ ...values, [e.target.name]: e.target.value });
};
const handleSubmit = (e) => {
e.preventDefault();
const validationErrors = {};
if (!values.name) {
validationErrors.name = 'Name is required';
}
setErrors(validationErrors);
if (Object.keys(validationErrors).length === 0) {
// Form is valid, submit data
}
};
return (
<form onSubmit={handleSubmit}>
<input name="name" value={values.name} onChange={handleChange} />
{errors.name && <span>{errors.name}</span>}
<input type="submit" />
</form>
);
};
export default MyForm;
=> Using Controlled Components:
- Manage the form input values through the component’s state and perform validation during input change or form submission.
import React, { useState } from 'react';
const MyForm = () => {
const [email, setEmail] = useState('');
const [emailError, setEmailError] = useState('');
const validateEmail = (value) => {
const regex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
return regex.test(value);
};
const handleChange = (e) => {
setEmail(e.target.value);
if (!validateEmail(e.target.value)) {
setEmailError('Invalid email format');
} else {
setEmailError('');
}
};
return (
<form>
<input type="email" value={email} onChange={handleChange} />
{emailError && <span>{emailError}</span>}
<input type="submit" disabled={emailError} />
</form>
);
};
export default MyForm;
=> Using Validation Libraries:
- Utilize libraries like Formik and Yup for more advanced form handling and validation.
npm install formik yup
import React from 'react';
import { Formik, Form, Field, ErrorMessage } from 'formik';
import * as Yup from 'yup';
const validationSchema = Yup.object().shape({
name: Yup.string().required('Name is required'),
email: Yup.string().email('Invalid email format').required('Email is required'),
});
const MyForm = () => (
<Formik
initialValues={{ name: '', email: '' }}
validationSchema={validationSchema}
onSubmit={(values) => {
console.log(values);
}}
>
{() => (
<Form>
<div>
<Field name="name" />
<ErrorMessage name="name" component="div" />
</div>
<div>
<Field name="email" />
<ErrorMessage name="email" component="div" />
</div>
<button type="submit">Submit</button>
</Form>
)}
</Formik>
);
export default MyForm;
=> Using Third-Party Validation Libraries:
- Libraries like react-hook-form provide extensive form validation capabilities.
import React from 'react';
import { useForm } from 'react-hook-form';
const MyForm = () => {
const { register, handleSubmit, formState: { errors } } = useForm();
const onSubmit = data => console.log(data);
return (
<form onSubmit={handleSubmit(onSubmit)}>
<input {...register('name', { required: 'Name is required' })} />
{errors.name && <span>{errors.name.message}</span>}
<input type="submit" />
</form>
);
};
export default MyForm;
Check out this personal project of mine on form validations in React:
More to Go:
- Wrapping up this blog here. In the next blog of this series, we'll cover the different methods of API calling and requests-respones between client and server in React. Stay tuned for more insightful content!
0
Subscribe to my newsletter
Read articles from Aryan kadam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
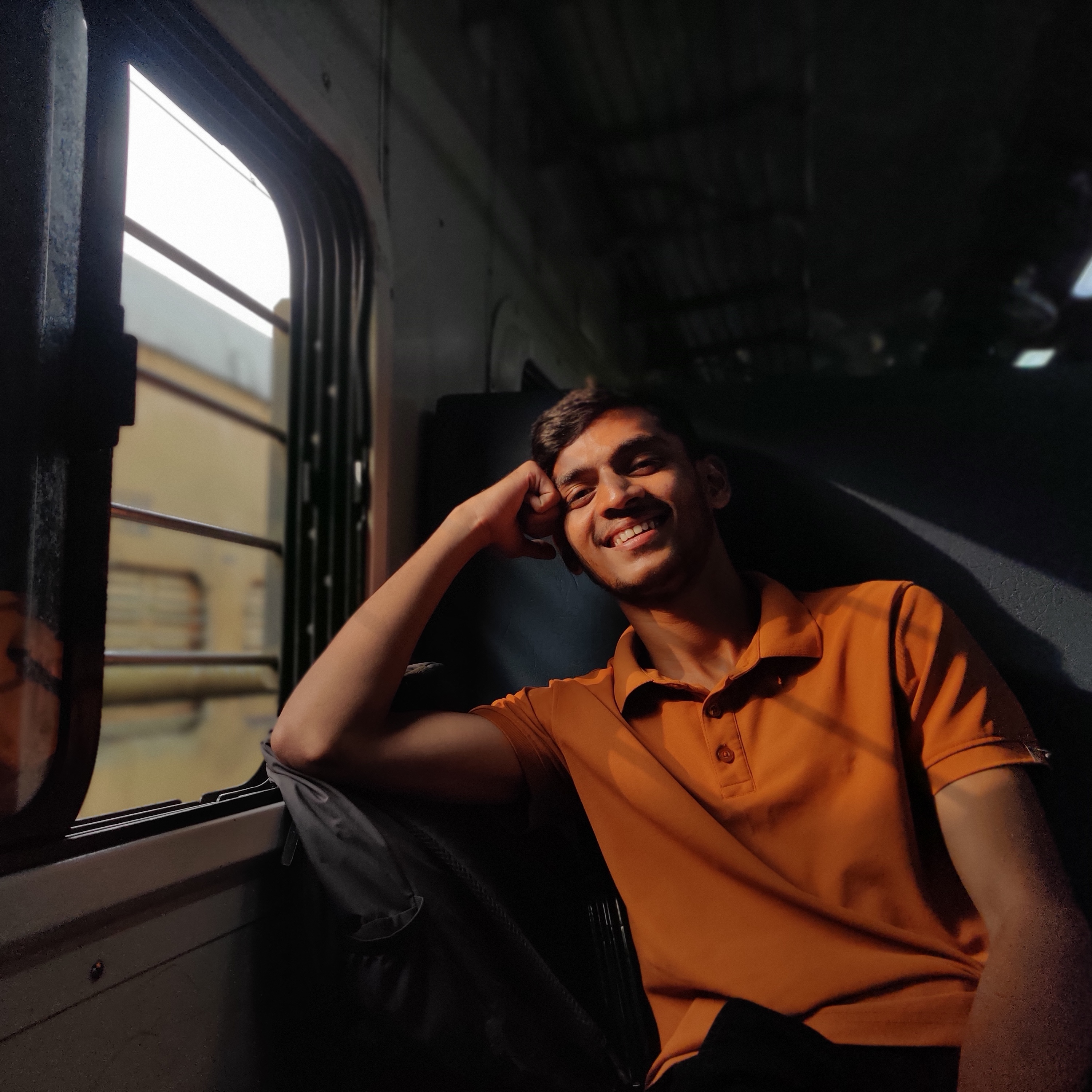
Aryan kadam
Aryan kadam
I am a student, coder, tech enthusiast, open source contributor, whatever you say.