Understanding REST APIs: A Simple Guide
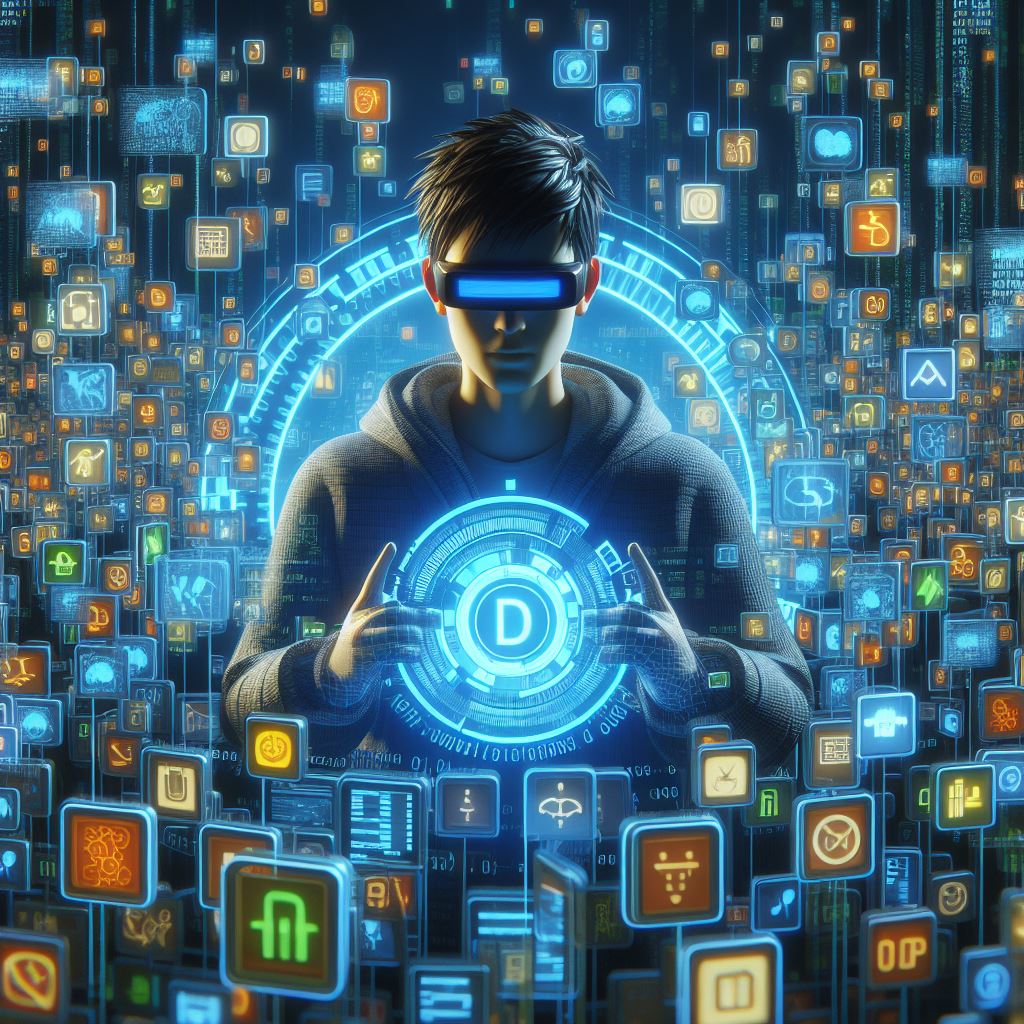
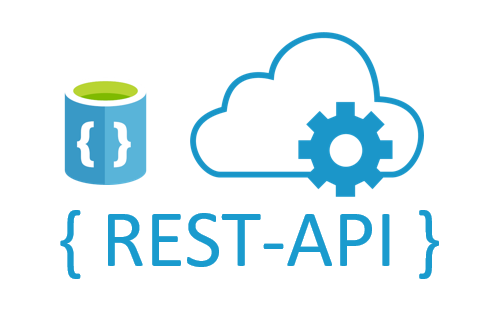
REST APIs, or representational state transfer application Programming Interfaces, are a set of rules and conventions for building and interacting with web services. They are based on the REST architecture style which was introduced by Roy Fielding in his doctoral dissertation. Here’s a detailed explanation of REST APIs:
Core principals of REST APIs
- Statelessness:
- Each request from a client to the server must contain all the information needed to understand and process the request. The server does not store any client context between requests. This means that each request is independent, and the server processes them without relying on any previous requests.
- Client-server Architecture:
- The client and server are separate entities that interact through the request-response model. The client is responsible for the user experience, while the server handles the data storage, business logic, and serving responses
- Uniform interface:
- REST APIs adhere to a uniform interface that simplifies and decouples the architecture, enabling each part to evolve independently. This interface is typically implemented through HTTP and involves standard methods such as GET, POST, PUT, PATCH, DELETE, and others
- Resource-based
- Resources (eg., users, posts, products) are the key abstraction in REST. Each resource is identified by a URI(Uniform Resource Identifier)for example ‘https://api.example.com/users/1’ might refer to a user with ID 1.
- Representation of Resources:
- Resources can be represented in different formats such as JSON, XML, or HTML. JSON is the most commonly used format due to its simplicity and readability. A client interacts with a resource by exchanging its representations.
- Stateless Operations
- Each operation on the resource (like reading, updating, or deleting) is performed in a stateless manner, relying solely on the information contained in the request itself.
- Cacheability
- Responses from the server can be marked as cacheable or non-cacheable enabling clients to cache responses and reduce the number of requests made to the server thus improving efficiency and performance.
- Layered system
- The architecture can be composed of multiple layers each with its own responsibilities for example an API gateway, load balancer, and application servers can form different layers each focusing on specific tasks.
HTTP Methods in REST
- GET
Retrieves a resource or a collection of resources.
Example ‘GET/user/1’ retrieves the user with ID 1
- POST
Creates a new resource.
Example ‘POST/user’ with a JSON payload containing user details creates a new user.
- PUT
Updates an existing resource or creates it if it does not exist.
Example ‘PUT/user/1’ updates the user with ID 1.
- DELETE
Deletes a resource
Example ‘DELETE/user/1’ deletes the user with ID 1
- PATCH
Partially updates a resource
Example ‘PATCH/user/1’ with a JSON payload containing only the fields to update modifies the user with ID1.
Example of REST API workflow
- Client Request
- A client sends a request to the server to fetch a resource. For example,’ GET/products/1’ to fetch the product with ID 1.
- Server processing
- The server processes the request, interacting with the database or other services as needed.
- Responses
- The server sends back a response, usually in JSON format containing the requested resource or an error message if something went wrong.
REST API Best Practices
- Use proper HTTP status codes
Ensure the API returns the appropriate HTTP status codes such as 2000(ok),201(created),400(bad request)404(not found),500(internal server error), etc.
- Versioning
Implement versioning in your API to handle changes without breaking existing clients' common methods including using a version number in the URI(eg ‘/VI/users’) or in headers.
- Pagination
For endpoint that returns a large number of resources implement pagination to avoid performance issues and improve response times.
- Security
Secure your API using HTTPS and implement authentication and authorization mechanisms such as OAuth, JWT(JSON Web Tokens), or API keys.
- Documentation
Provide clear and comprehensive documentation for your API including endpoint requests/response formats and example use cases. Tools like Swagger/OpenAPI can help generate interactive documentation.
REST APIs are widely used due to their simplicity and flexibility they allow developers to create robust and maintainable web services that various clients, including web browsers, mobile applications, and other servers can easily consume.
Subscribe to my newsletter
Read articles from Reagan Mwangi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
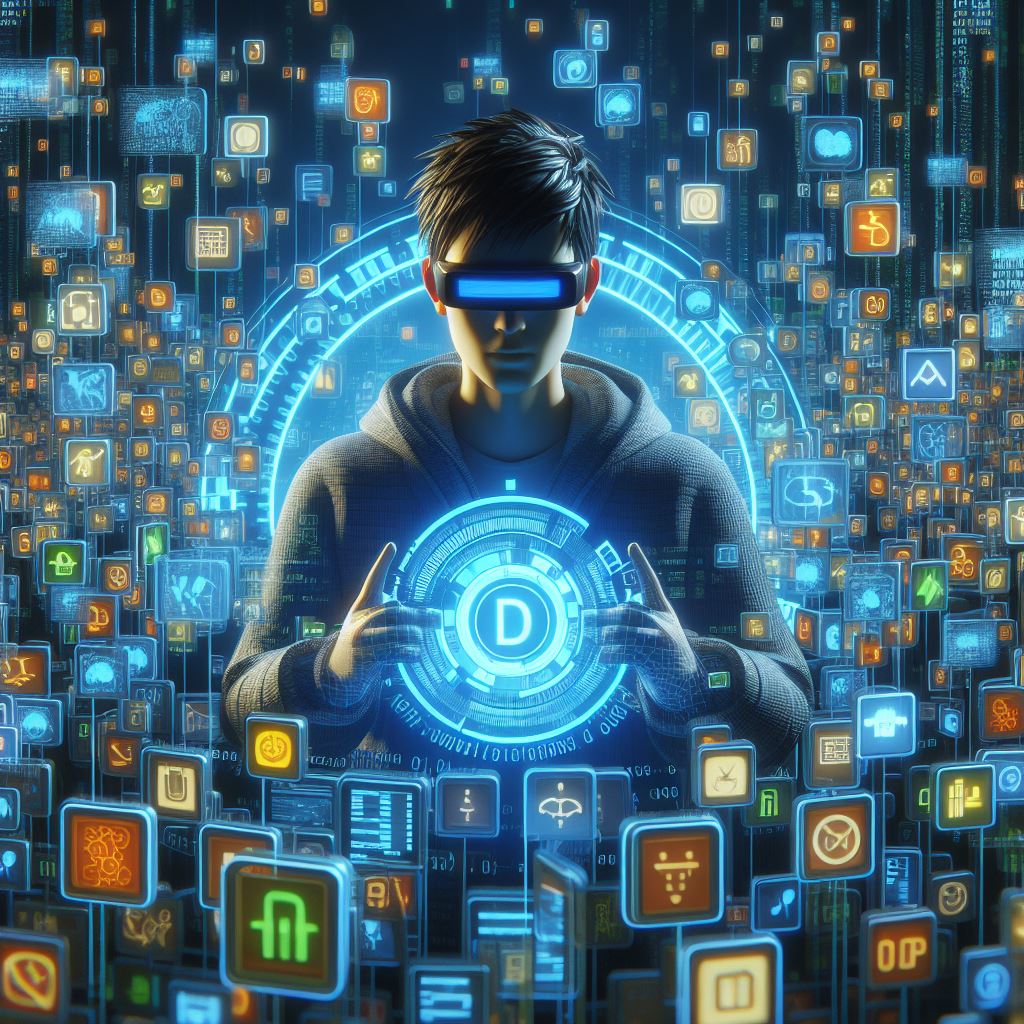