Session 2 : A Guide to Operators, If-Else Statements, and Loops
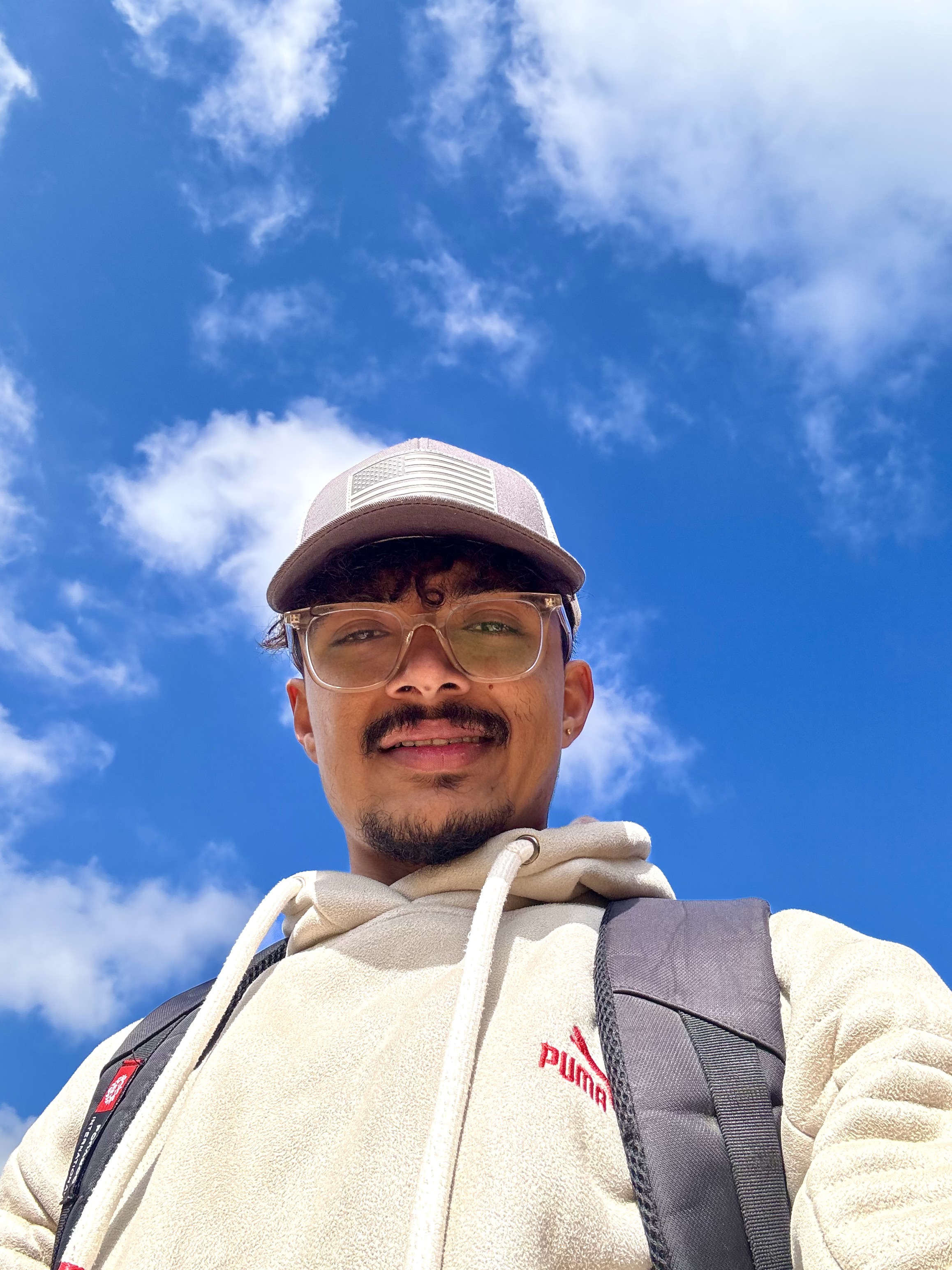
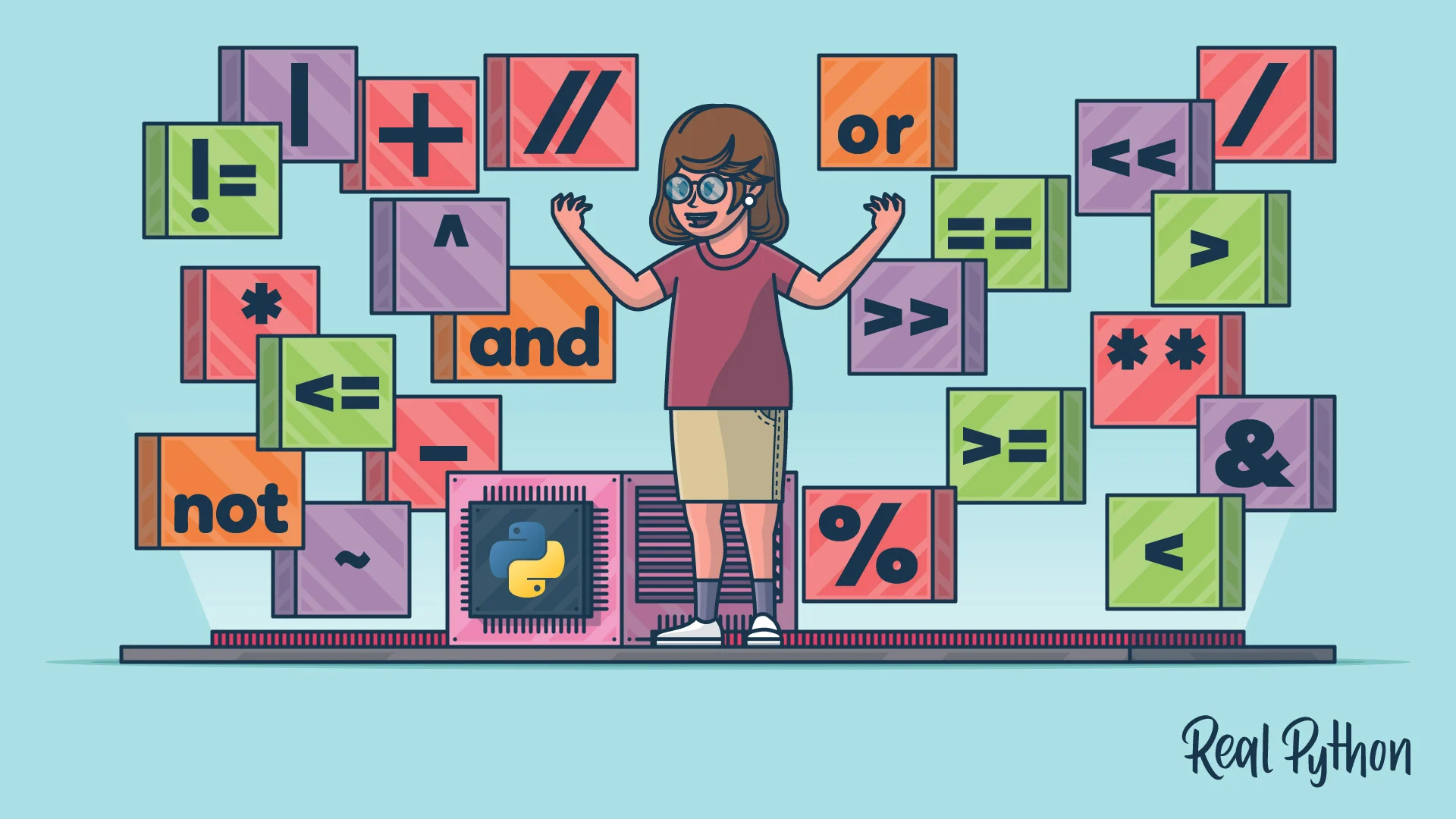
In this post, we will learn about operators, loops, and conditional expressions, which are fundamental components in Python.
Just imagine, you'd like to,
Manipulate data: Operators enable you to perform calculations, comparisons, and assignments, like "calculate the 5 power 7 ? ".
Make decisions: If-else conditions let your program make choices based on data, like "if it's raining, take an umbrella".
Repeat tasks: Loops help you perform actions multiple times, like "printing I love you 5 times".
Mastering these fundamentals concept will help you write efficient, logical, and readable code, to become a good Python programmer!
Operators
In python, operators are special symbols or keywords which are used to perform operations on variables and values. Let's learn different types of operators in python,
Arithmetic Operators
We used arithmetic operators for basic mathematical operations like,
+
: Addition-
: Subtraction*
: Multiplication/
: Division%
: Modulus (remainder of division)**
: Exponentiation (power of)//
: Integer Division (returns, the nearest integer lower than the result)print(5+6) # 11 print(5-6) # -1 print(5*6) # 30 print(5/2) # 2.5 print(5//2) # 2 print(5%2) # 1 print(5**2) # 25
Relational Operators
We used relational operators to compare two values and return result in boolean.
==
: Equal to!=
: Not equal to>
: Greater than<
: Less than>=
: Greater than or equal to<=
: Less than or equal toprint(4>5) # False print(4<5) # True print(4>=5) # True print(4<=5) # True print(4==4) # True print(4!=4) # False
Logical Operators
Logical operators are used to combine conditional statements.
and
: Returns True if both statements are trueor
: Returns True if one of the statements is truenot
: If True return False | If False return Trueprint(1 and 0) # O [False] print(0 or 1) # 1 [True] print(not 1) # False
Bitwise Operators
We use bitwise operators only to operate on bits or binary digits.
&
: AND|
: OR^
: XOR (exclusive OR)~
: NOT (inverts all the bits)<<
: Left shift>>
: Right shiftprint(2&3) # 2 print(2|3) # 3 print(2^3) # 1 print(~3) #-4 print(4>>2) # 1 print(5<<2) # 20
In data science, bitwise operators are not commonly used may be we used & (AND) ,| (OR)
operators for image processing , computer vision projects. But, typically more relevant in low-level programming, system programming. To understand more : Link
Assignment Operators
Assignment operators
=
is used to assign a value to a variable.a = 2 # assign value 2 to variable a a += 2 # means: a = a + 2 print(a) # 4
Identity Operators
Identity operators are used to check if two values are located at same memory location or not.
is
: True if the operands are identical (refer to the same object)is not
: True if the operands are not identical (do not refer to the same object)a = 10 b = 10 c = a l1 = [1,2,3] l2 = [1,2,3] print(a is b) # True print(a is c) # True print(c is b) # True print(l1 is l2) # False
Here, l1
and l2
have same list but they are not identical because it store both in different memory location although they have same list.
Membership Operators
Membership operators are used to check if a sequence is presented in or not.
in
: True if value found in a sequencenot in
: True if value not found in a sequenceprint('P' in 'Python') # True print('P' in 'python') # False print(1 not in [1,2,3,4,5]) # False
Now that you've learned the basics of operators in Python, it's time to put that knowledge into practice.
Task:WAP to find the sum of 3 digits number given by the user. Eg. Input: 345 | Output: 12
If-Else Condition
In Python, if-else statements are essential control flow tools that enable you to make decisions based on specific conditions. Here’s a simple example to understanding if-else statements in Python.
age = 15
if age >= 18:
print("You are eligible for driving license")
else:
print("You are a minor.")
# Output: You are a minor.
Here, if-else
statement allows you to execute one block of code if the condition is True and another block of code if the condition is False. Since age
is 15, the condition age >= 18
is False, so the message "You are a minor." is printed.
Moreover, for more complex decision-making, we use elif
("else if") to check multiple conditions. It lets you test several conditions in sequence until one is found to be true. Here's a simple login program to check whether the user input email and password are valid or not.
# valid_email -> datadiary@gmail.com
# password -> datadiary
email = input('enter email: ')
password = input('enter password: ')
if email == 'datadiary@gmail.com' and password == 'datadiary':
print('Welcome')
# if email valid but password wrong
elif email == 'datadiary@gmail.com' and password != 'datadiary':
# tell the user
print('Incorrect password')
# Reenter password
password = input('enter password again: ')
if password == 'datadiary':
print('Welcome,finally!')
else:
print('password invalid')
else:
print('No correct input.')
Now, try to solve one question on if-else statement,
Task :WAP that determines the category of a person based on their given age.
"Child"
if the age is 0-12"Teenager"
if the age is 13-19"Adult"
if the age is 20-59"Senior"
if the age is 60 or above
Modules
Python Module is a file that contains built-in functions, classes, and variables. There are many Python modules, each with its specific work. In this section, we are going to learn about math, keywords, random and datetime modules. To know more about python modules you can check on python documentation page.
Math
The math module provides access to a variety of mathematical functions and constants.
math.sqrt(x)
: Returns the square root ofx
.math.pow(x, y)
: Returnsx
raised to the power ofy
.math.pi
: The constant π (pi).math.sin(x)
,math.cos(x)
,math.tan(x)
: Trigonometric functions.math.log(x, base)
: Returns the logarithm ofx
to the specifiedbase
.import math print(math.sqrt(190) # 13.784 print("Value of pi:", math.pi) # 3.1416
Keywords
Keyword module provides a simple way to check for Python keywords, which are reserved words that cannot be used as identifiers (variable names, function name etc.
import keyword print('Total keyword in python: ', len(keyword.kwlist)) # 35 print(keyword.kwlist) # ['False', 'None', 'True', 'and', 'as', 'assert', 'async', 'await', 'break', 'class', 'continue', 'def', 'del', 'elif', 'else', 'except', 'finally', 'for', 'from', 'global', 'if', 'import', 'in', 'is', 'lambda', 'nonlocal', 'not', 'or', 'pass', 'raise', 'return', 'try', 'while', 'with', 'yield']
Random
The random module is used to generate random numbers and perform random operations. It is useful for tasks like simulations, gaming, and random sampling.
random.random()
: Returns a random float between 0.0 and 1.0.random.randint(a, b)
: Returns a random integer betweena
andb
(inclusive).random.shuffle(seq)
: Shuffles the sequenceseq
in place.import random print(random.randint(1,100)) # 3 numbers = [1,2,3,4,5] random.shuffle(numbers) print(numbers) # [1,2,5,3,4]
Datetime
The datetime module supplies classes for manipulating dates and times. It is useful for applications that require date and time calculations.
datetime.date
(y, m, d)
: Represents a date.datetime.time(hrs, min, sec)
: Represents a time.datetime.datetime(year, month, day, hour, minute, second)
: Represents both date and time.datetime.datetime.now
()
: Returns the current local date and time.import datetime print(datetime.datetime.now()) # 2024-05-23 12:29:11.942735
Task:
Write a Python program that checks if a given word is a Python keyword.
Write a Python program that generates and prints a random password. The password should be 8 characters long and can include uppercase letters, lowercase letters, and digits.
Loops
Python provides two types of loops: for loops and while loops. In this section, we'll explore both types of loops,
For loop
A for loop is used to iterate over a sequence (like a list, tuple, dictionary, set, or string) and execute a block of code for each item in the sequence.
for i in range(1,2,3,4,5): print(i) # 1 # 2 # 3 # 4 # 5 # range() is often used to generate a sequence of numbers.
While loop
A while loop executes a block of code as long as a specified condition is true.
i = 1 while i <= 4: print(i) i += 1 # 1 # 2 # 3 # 4
Above, while loop continues to execute as long as
i
is less than or equal to 4. The value ofi
is incremented by 1 in each iteration until the condition is no longer satisfied.
Task:
Write a Python program that takes an integer n as input and prints the multiplication table for n up to 10.
The current population of a town is 10000. The population of the town is increasing at the rate of 10% per year. You have to write a program to find out the population at the end of each of the last 10 years.
If you have any questions on any topic covered in this blog post, feel free to leave a comment below. I'm here to help you on your learning journey!
Happy coding and happy learning! 🚀
Subscribe to my newsletter
Read articles from Manoj Paudel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
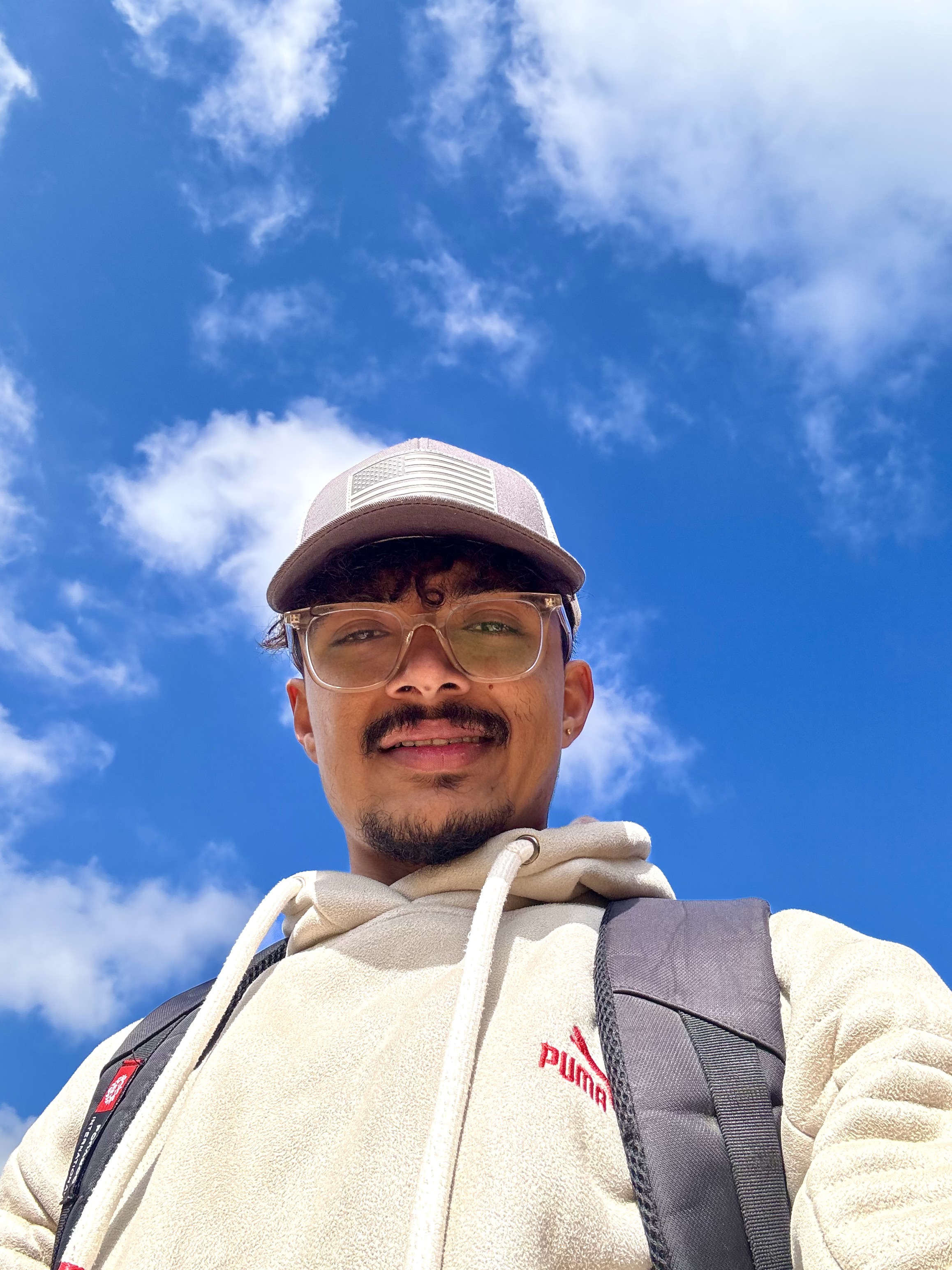
Manoj Paudel
Manoj Paudel
Hey there! I'm Manoj Paudel, a third-year Computer Science student diving into the world of data science. I'm excited to share my learning journey through my blogs.