Mastering Go: Guide to Type Declarations, Variables, and Constants
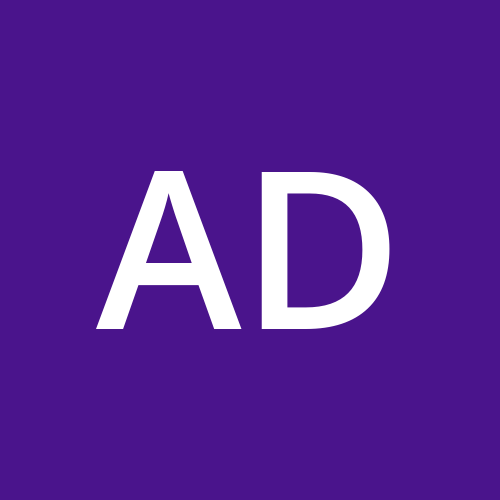
Welcome to the world of Go programming! In this blog post we will dive into the intricacies of type declarations, variables and constants, in Go. Having an understanding of these concepts is essential for any Go developer. Through our exploration we will shed light on the nuances that make Go an expressive language.
Type Declarations in Go; Building a Foundation for Clarity
An aspect of Go is its detailed approach to type declarations. Although it may appear verbose compared to languages this deliberate design choice prioritizes clarity over brevity. Lets delve into how Go handles type declarations and understand why this emphasis on verbosity can actually be beneficial.
Untyped Literals: Flexibility and Practicality
In Go, literals are untyped, providing a remarkable degree of flexibility. This means that you can use integer literals in floating-point expressions and assign integer literals to floating-point variables. This practicality stems from Go’s philosophy of avoiding forced type conversions until explicitly specified by the developer. We’ll discover how untyped literals contribute to a more versatile coding experience.
Declaring Variables in Go: Choose Wisely
Go offers multiple ways to declare variables, each with its own purpose and readability benefits. From the verbose var x int = 10
to the concise x := 10
, we’ll explore when to use each declaration style. Understanding these distinctions is crucial for effectively conveying your intent through code.
Choosing Between var and :=
The decision between var
and :=
often comes down to context and readability. Within functions, the :=
operator is prevalent for its brevity, while var
is preferred outside functions. We’ll discuss the situations where each style shines and offer insights into making your code clearer.
Avoiding Pitfalls with :=
While :=
is powerful, it has its limitations and potential pitfalls. We’ll explore scenarios where it’s advisable to use var
to avoid unintended consequences, such as variable shadowing. Additionally, we’ll touch on best practices for variable declarations in package-level blocks.
Immutability with const in Go
Go introduces the const
keyword for declaring immutable values. We’ll examine how constants differ from variables and explore the limitations and use cases of constants in Go. Understanding the role of const
is essential for maintaining a clean and efficient codebase.
Typed and Untyped Constants
Constants in Go can be typed or untyped, and choosing between them depends on the use case. We’ll discuss the scenarios where untyped constants offer more flexibility and delve into the situations where typed constants become necessary. The role of iota
in constant declarations will also be introduced.
Here’s what a typed constant declaration looks like:
const typedX int = 10
Here’s what an untyped constant declaration looks like:
const x = 10
Unused Variables: Go’s Unique Rule
Go enforces a unique rule: every declared local variable must be read. While the compiler’s unused variable check has limitations, adhering to this rule contributes to cleaner and more maintainable code. We’ll explore the rationale behind this rule and discuss how it aligns with Go’s goal of fostering collaborative coding.
func main() {
x := 10 // this assignment isn’t read!
x = 20 fmt.Println(x)
x = 30 // this assignment isn’t read!
}
Unused Constants and Package-Level Variables
Surprisingly, Go allows the creation of unread constants. We’ll investigate why this is permissible and the implications it has on code compilation. The discussion extends to package-level variables, emphasizing the potential challenges they introduce to code maintainability.
Naming Conventions: A Crucial Aspect of Readable Code
Good naming conventions are at the heart of readable and maintainable code. We’ll explore Go’s rules for naming variables and constants, emphasizing the importance of clear and descriptive names. From Unicode characters to camel case, we’ll cover the do’s and don’ts of naming identifiers in Go.
Short vs. Descriptive Names
Go encourages short variable names, especially within limited scopes, like for loops. We’ll discuss the rationale behind single-letter variable names and their role in maintaining code simplicity. Conversely, for package-level declarations, we’ll explore the need for more descriptive names to convey broader meanings.
Practical Exercises: Apply Your Knowledge
To solidify your understanding, we’ll conclude with practical exercises that allow you to apply the concepts covered in this blog. From variable assignments to constants and data type manipulations, these exercises will sharpen your Go programming skills.
Conclusion: Setting the Stage for Go Mastery
Congratulations on navigating through the intricate landscape of type declarations, variables, and constants in Go! Armed with this knowledge, you’re well on your way to mastering Go programming. In the next chapter, we’ll unravel the mysteries of composite types, including arrays, slices, maps, and structs. Get ready to explore the power and flexibility of Go’s composite data structures!
Subscribe to my newsletter
Read articles from Aman dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
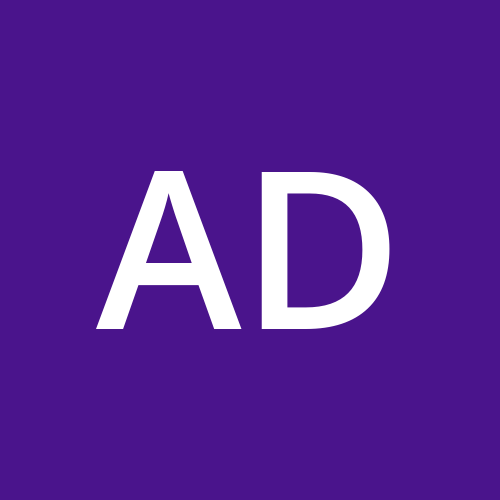