Demystifying Object-Oriented Programming (OOP) Concepts
Table of contents
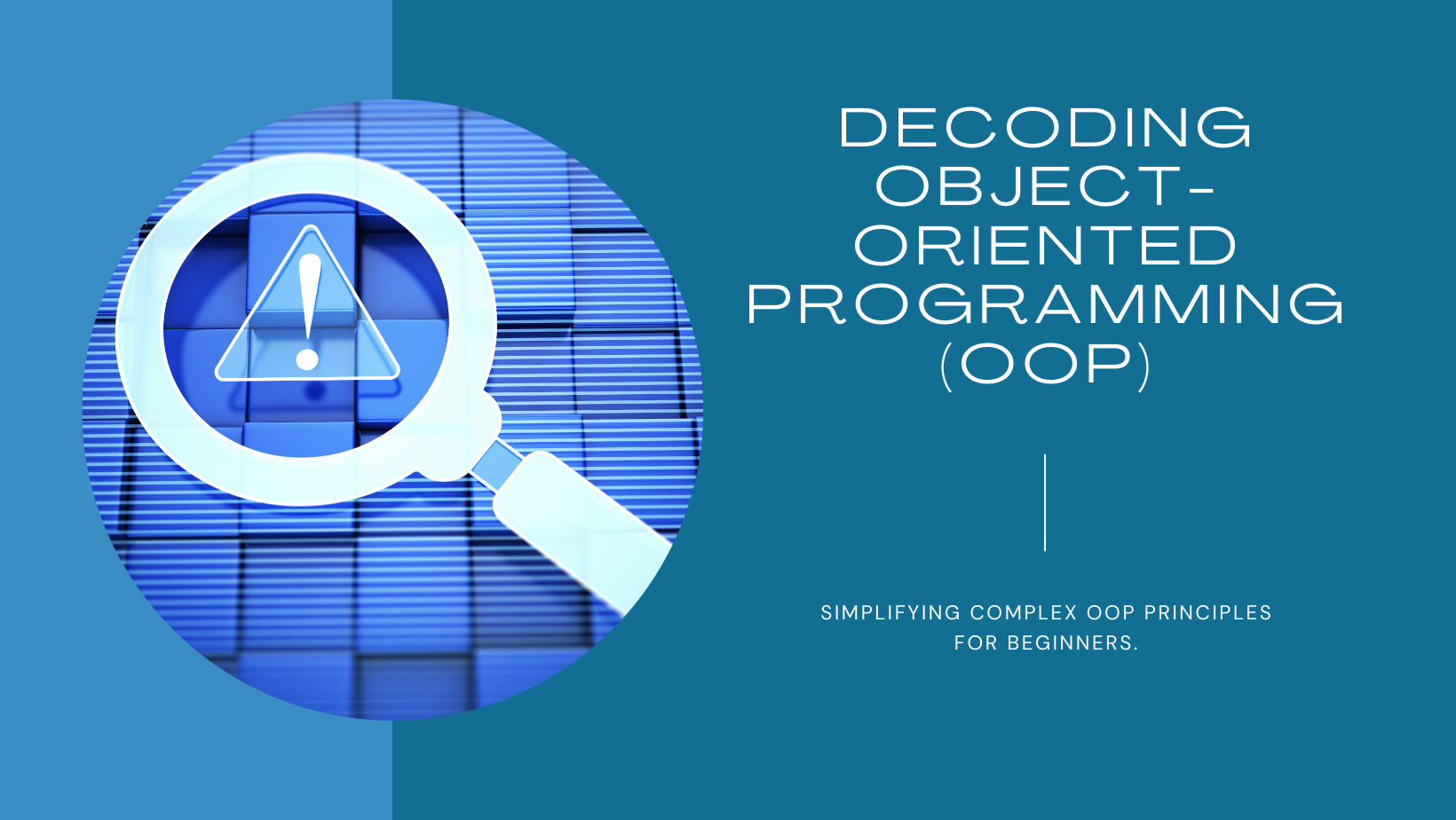
Object-Oriented Programming (OOP) is a fundamental concept in software development, yet it can be intimidating for beginners. In this post, we'll break down the basics of OOP, exploring its core principles, benefits, and practical applications.
What is Object-Oriented Programming?
OOP is a programming paradigm that revolves around the concept of objects and classes. It's a way of designing and organizing code to make it more modular, reusable, and efficient.
Key OOP Concepts:
Classes: A blueprint or template that defines the properties and behavior of an object.
Objects: Instances of classes, with their own set of attributes (data) and methods (functions).
Inheritance: A mechanism where a child class inherits properties and behavior from a parent class.
Polymorphism: The ability of an object to take on multiple forms, depending on the context.
Encapsulation: Hiding internal implementation details of an object from the outside world.
Abstraction: Focusing on essential features and hiding non-essential details.
Benefits of OOP:
Modularity: OOP promotes code organization and reusability.
Easier Maintenance: OOP makes it easier to modify and extend existing code.
Improved Code Readability: OOP encourages self-documenting code.
Faster Development: OOP enables developers to build on existing classes and objects.
Real-World Analogy:
Think of a car as an object. The car class would define properties like color, model, and year, as well as methods like startEngine() and accelerate(). You can create multiple car objects, each with its own attributes and behavior.
Practical Applications:
Game Development: OOP is ideal for creating game characters, objects, and environments.
Simulation Software: OOP is used in simulations, like flight simulators or weather forecasting systems.
Web Development: OOP is applied in web frameworks like Ruby on Rails and Django.
Code Samples:
Python
# Define a Car class
class Car:
def __init__(self, color, model, year):
self.color = color
self.model = model
self.year = year
def startEngine(self):
print("Vroom!")
def accelerate(self):
print("Speeding up!")
# Create a car object
my_car = Car("Red", "Toyota", 2022)
# Call methods on the object
my_car.startEngine() # Output: Vroom!
my_car.accelerate() # Output: Speeding up!
Java
// Define a Car class
public class Car {
private String color;
private String model;
private int year;
public Car(String color, String model, int year) {
this.color = color;
this.model = model;
this.year = year;
}
public void startEngine() {
System.out.println("Vroom!");
}
public void accelerate() {
System.out.println("Speeding up!");
}
}
// Create a car object
Car my_car = new Car("Red", "Toyota", 2022);
// Call methods on the object
my_car.startEngine(); // Output: Vroom!
my_car.accelerate(); // Output: Speeding up!
JavaScript
// Define a Car class
class Car {
constructor(color, model, year) {
this.color = color;
this.model = model;
this.year = year;
}
startEngine() {
console.log("Vroom!");
}
accelerate() {
console.log("Speeding up!");
}
}
// Create a car object
const my_car = new Car("Red", "Toyota", 2022);
// Call methods on the object
my_car.startEngine(); // Output: Vroom!
my_car.accelerate(); // Output: Speeding up!
Conclusion:
Object-Oriented Programming is a powerful concept that simplifies software development. By understanding classes, objects, inheritance, polymorphism, encapsulation, and abstraction, you'll be better equipped to write efficient, modular, and maintainable code. Embrace OOP and take your programming skills to the next level!
#alx9jacommunity #t22 #alxfellowship #alx_africa
Subscribe to my newsletter
Read articles from Oladele David Temidayo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Oladele David Temidayo
Oladele David Temidayo
I am Oladele David a software developer and tech enthusiast based in Lagos Nigeria. I'm passionate about sharing and acquiring knowledge, documentation, Open-source projects and communities.