Day 14 - Python Data Types and Data Structures for DevOps
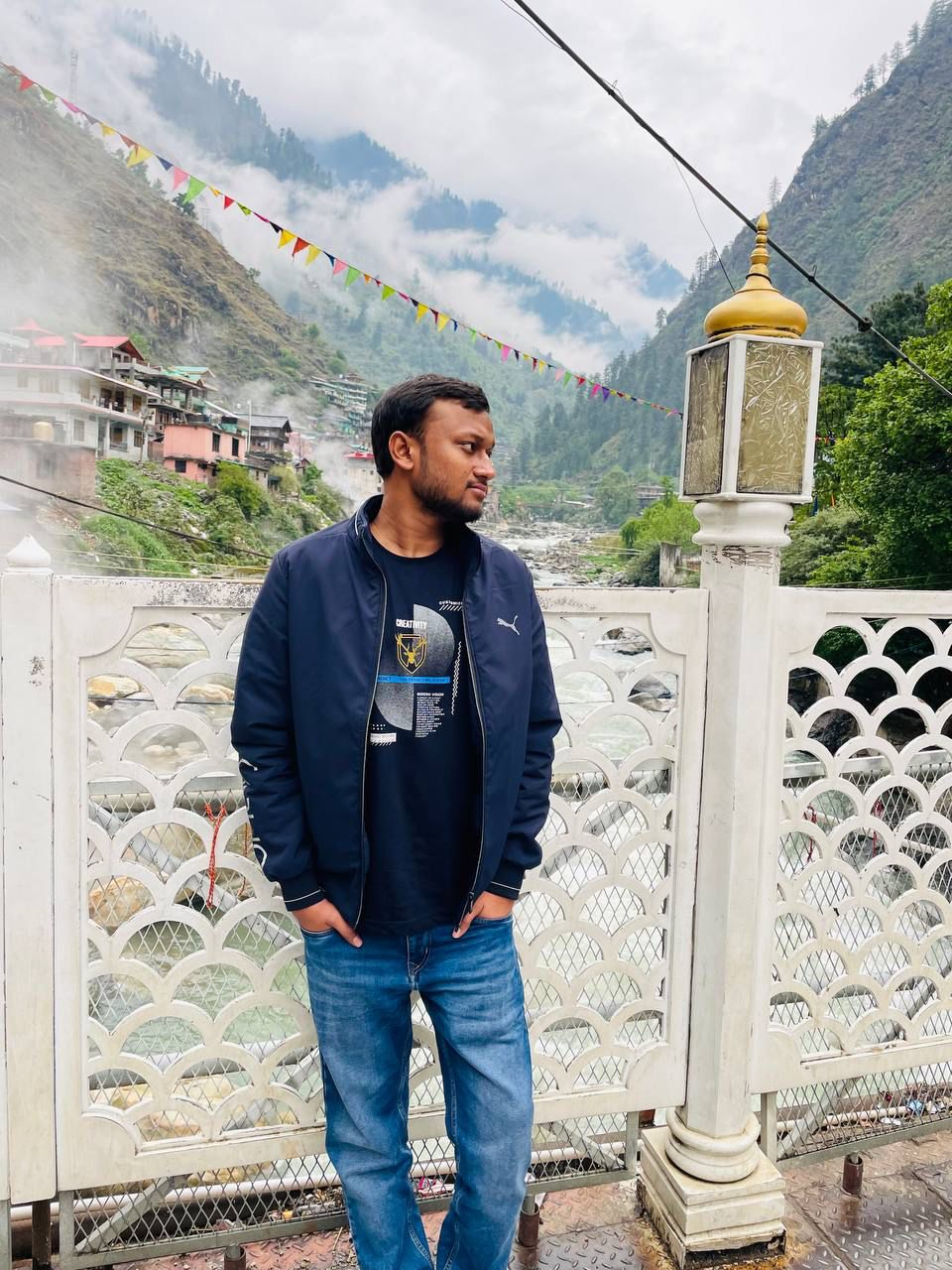
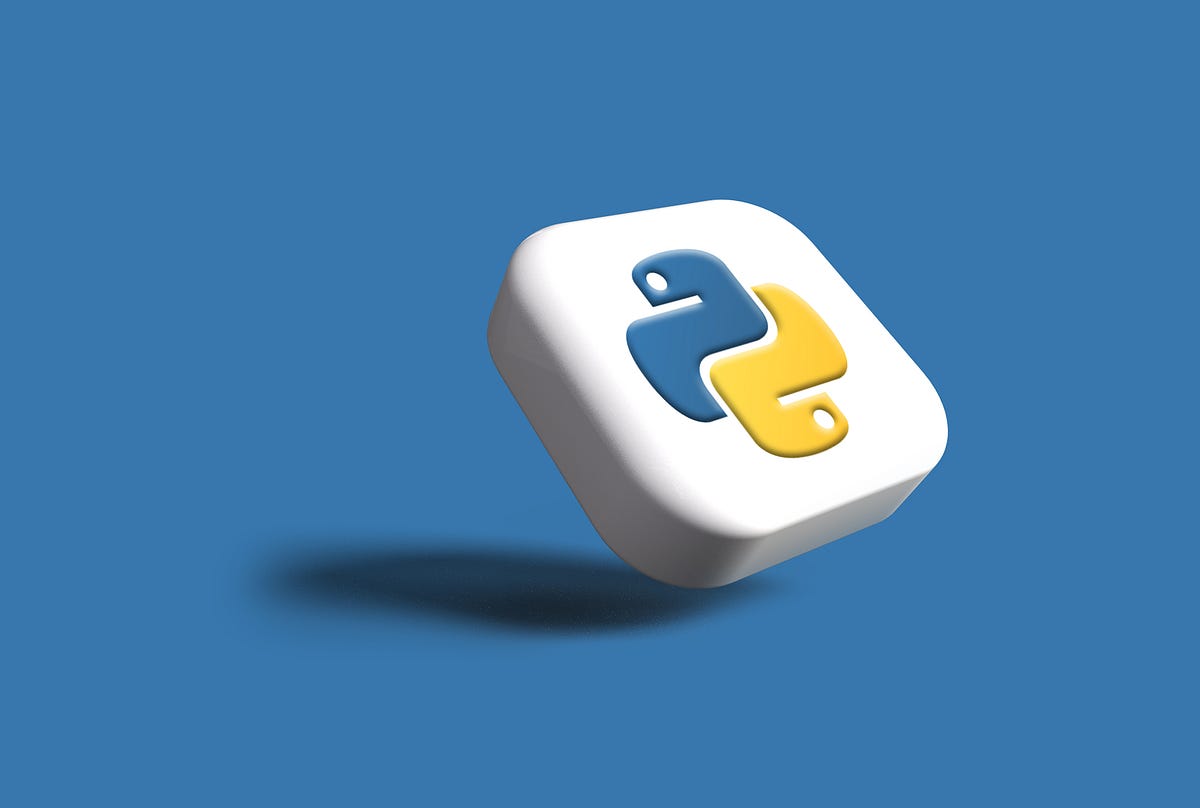
Introduction
Python is a versatile language that is extensively used in the DevOps world for automating tasks, managing infrastructure, and more. In this blog, we will explore Python data types and data structures, focusing on Lists, Tuples, Sets, and Dictionaries. Understanding these fundamental concepts is crucial for efficient data handling and manipulation in your DevOps scripts.
Data Types
Python, being a dynamically typed language, supports various data types. These data types are essentially classes, and the variables are instances of these classes. Some of the primary data types include:
Numeric: Includes integers, floats, and complex numbers.
Sequential: Includes strings, lists, and tuples.
Boolean: Represents True or False values.
Set: An unordered collection of unique elements.
Dictionaries: Key-value pairs for storing data.
Data Structures
Data structures are vital for organizing data efficiently. Let's discuss three common data structures: Lists, Tuples, and Sets.
Lists
Lists are ordered, mutable collections of items. They are flexible, allowing mixed data types and duplicate entries.
my_list = [1, 'Python', 3.14, True]
my_list.append('DevOps')
print(my_list) # Output: [1, 'Python', 3.14, True, 'DevOps']
Tuples
Tuples are similar to lists but are immutable, meaning their content cannot be changed after creation. They are useful for read-only collections of data.
my_tuple = (1, 'Python', 3.14, True)
print(my_tuple) # Output: (1, 'Python', 3.14, True)
Sets
Sets are unordered collections of unique items. They do not allow duplicates and are useful for membership testing and eliminating duplicates.
my_set = {1, 'Python', 3.14, True, 1}
print(my_set) # Output: {1, 'Python', 3.14, True}
Working with Dictionaries
Dictionaries in Python are collections of key-value pairs. They are highly optimized for retrieval operations.
fav_tools = {
1: "Linux",
2: "Git",
3: "Docker",
4: "Kubernetes",
5: "Terraform",
6: "Ansible",
7: "Chef"
}
print(fav_tools[3]) # Output: Docker
Managing Cloud Providers List
In DevOps, managing lists of cloud service providers is a common task. Here’s how to add a new provider to the list and sort it.
cloud_providers = ["AWS", "GCP", "Azure"]
cloud_providers.append("Digital Ocean")
cloud_providers.sort()
print(cloud_providers) # Output: ['AWS', 'Azure', 'Digital Ocean', 'GCP']
Difference Between List, Tuple, and Set
List
Mutable: Elements can be changed or updated.
Ordered: Maintains the order of insertion.
Allows Duplicates: Can contain duplicate elements.
Syntax: Defined using square brackets
[]
.
my_list = [1, 2, 3, 4, 5]
Tuple
Immutable: Once created, elements cannot be changed or updated.
Ordered: Maintains the order of insertion.
Allows Duplicates: Can contain duplicate elements.
Syntax: Defined using parentheses
()
.
my_tuple = (1, 2, 3, 4, 5)
Set
Mutable: Elements can be added or removed.
Unordered: Does not maintain any order.
No Duplicates: Cannot contain duplicate elements.
Syntax: Defined using curly braces
{}
.
my_set = {1, 2, 3, 4, 5}
Hands-on Examples
List Example
my_list = [1, 2, 3, 4, 5]
my_list.append(6) # Adding an element
print(my_list) # Output: [1, 2, 3, 4, 5, 6]
Tuple Example
my_tuple = (1, 2, 3, 4, 5)
# Tuples are immutable, so we cannot add or remove elements
print(my_tuple) # Output: (1, 2, 3, 4, 5)
Set Example
my_set = {1, 2, 3, 4, 5}
my_set.add(6) # Adding an element
print(my_set) # Output: {1, 2, 3, 4, 5, 6}
Dictionary Tasks
Creating and Using a Dictionary
Let's create the given dictionary and use dictionary methods to print the favorite tool by using keys.
fav_tools = {
1: "Linux",
2: "Git",
3: "Docker",
4: "Kubernetes",
5: "Terraform",
6: "Ansible",
7: "Chef"
}
# Print the favorite tool for key 3 (Docker)
print(fav_tools[3]) # Output: Docker
Working with Lists of Cloud Service Providers
Initial List and Adding a New Provider
Let's create a list of cloud service providers, add "Digital Ocean" to it, and sort the list alphabetically.
cloud_providers = ["AWS", "GCP", "Azure"]
# Add Digital Ocean to the list
cloud_providers.append("Digital Ocean")
# Sort the list alphabetically
cloud_providers.sort()
print(cloud_providers) # Output: ['AWS', 'Azure', 'Digital Ocean', 'GCP']
Conclusion
Understanding Python data types and data structures is fundamental for efficient data handling in DevOps. Lists, Tuples, Sets, and Dictionaries each have unique characteristics and use cases that can help optimize your scripts and applications. Keep practicing and exploring these concepts to enhance your DevOps skills!
Thank you for reading our DevOps blog post. We hope you found it informative and helpful. If you have any questions or feedback, please don't hesitate to contact us.
I hope this helps!
Happy Learning✨
Subscribe to my newsletter
Read articles from Rahul Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
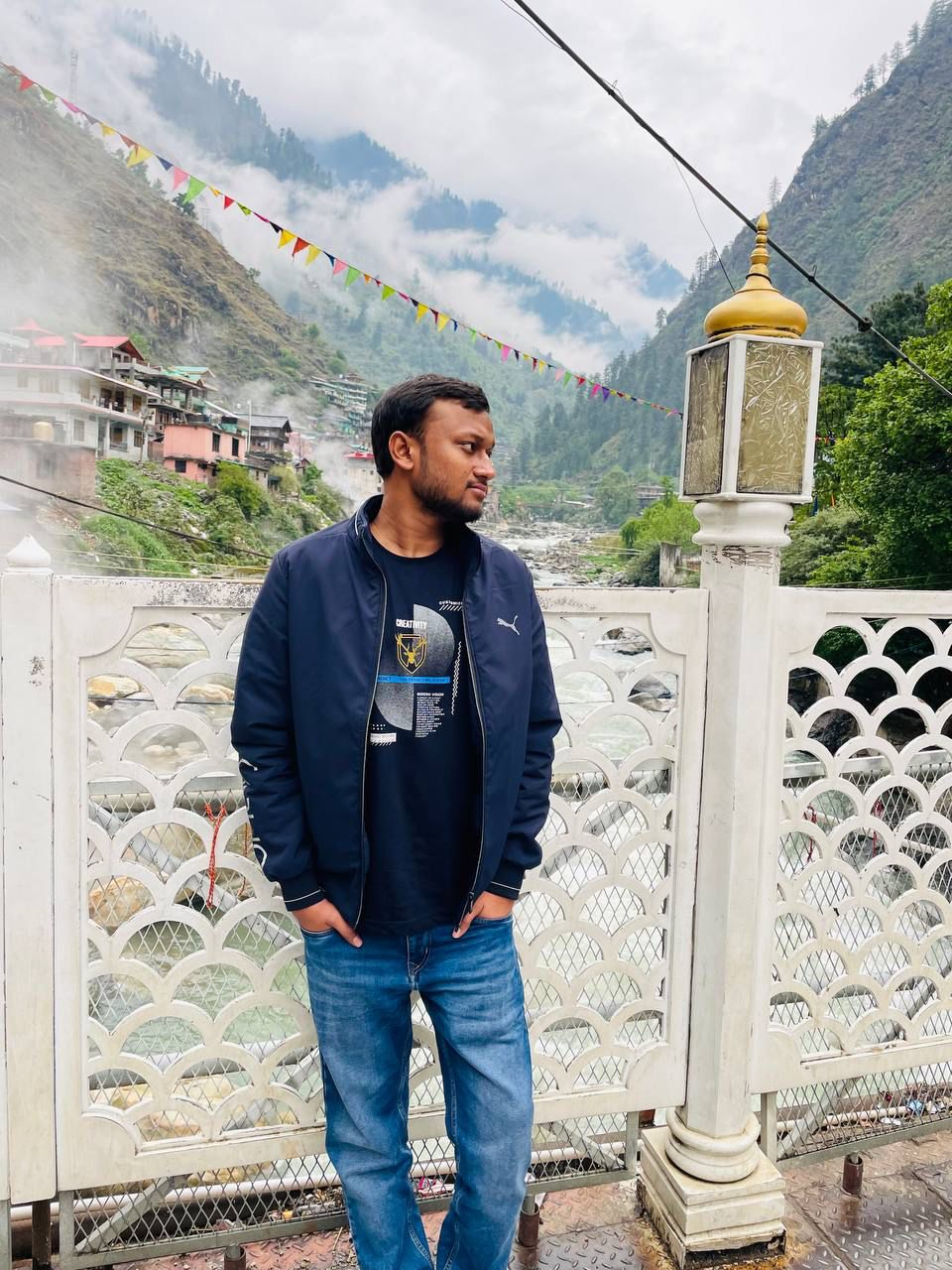
Rahul Gupta
Rahul Gupta
Hey there! 👋 I'm Rahul Gupta, a DevOps Engineer passionate about all things AWS DevOps Technology. Currently, on a learning adventure, I'm here to share my journey and Blogs in the world of cloud and DevOps. 🛠️ My focus? Making sense of AWS services, improving CI/CD, and diving into infrastructure as code. Whether you're fellow interns or curious enthusiasts, let's grow together in the vibrant DevOps space. 🌐 Connect with me for friendly chats, shared experiences, and learning moments. Here's to embracing the learning curve and thriving in the exciting world of AWS DevOps Technology!