Exploring AWS Simple Queue Service (SQS): A Hands-On Guide

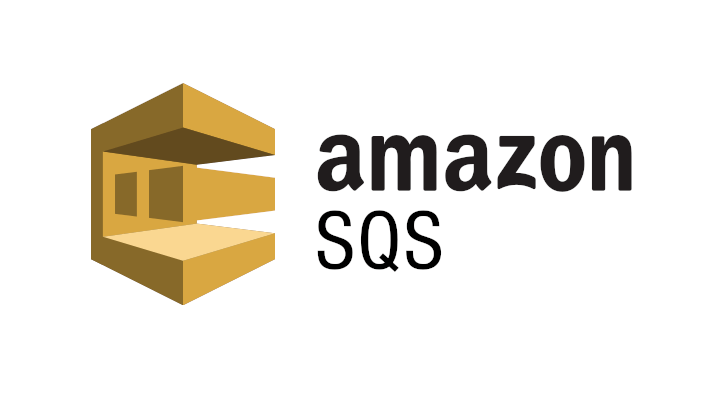
In today's fast-paced digital landscape, the need for efficient and reliable messaging systems has become paramount. Whether it's processing orders, managing workflows, or orchestrating microservices, businesses require a robust solution to handle message queues effectively. This is where Amazon Web Services (AWS) Simple Queue Service (SQS) comes into play.
Understanding AWS SQS
AWS SQS is a fully managed message queuing service that enables you to decouple and scale microservices, distributed systems, and serverless applications. It eliminates the complexity and overhead associated with managing message queues, allowing developers to focus on building scalable and resilient applications.
Key Features of AWS SQS:
Fully Managed: AWS SQS is a fully managed service, which means AWS handles the infrastructure, scalability, and availability of the message queues, allowing you to focus on application development.
Reliability: SQS ensures message durability by storing messages redundantly across multiple availability zones within a region, providing high availability and fault tolerance.
Scalability: SQS scales seamlessly with the volume of messages. It can handle any amount of throughput without requiring any manual intervention.
Flexible Message Queues: SQS offers two types of message queues: Standard Queue and FIFO (First-In-First-Out) Queue. Standard Queues provide best-effort ordering and high throughput, while FIFO Queues guarantee exactly-once processing and preserve the order of messages.
Security: SQS integrates with AWS Identity and Access Management (IAM) for fine-grained access control, allowing you to grant specific permissions to users or applications.
Visibility Timeout: SQS allows you to set a visibility timeout for messages. During this period, the message remains invisible to other consumers, enabling the consumer to process and delete the message without interference.
Hands-On Example: Sending and Receiving Messages with AWS SQS
Let's walk through a simple hands-on example to demonstrate how to send and receive messages using AWS SQS:
Step 1: Create an SQS Queue
Navigate to the AWS Management Console and open the SQS dashboard.
Click on "Create Queue" and choose the queue type (Standard or FIFO), then provide a name for your queue.
Configure the queue settings as per your requirements, such as message retention period, visibility timeout, etc.
Click on "Create Queue" to create your SQS queue.
Step 2: Send Messages to the Queue
import boto3
# Initialize SQS client
sqs = boto3.client('sqs', region_name='your_region')
# Send message to the queue
response = sqs.send_message(
QueueUrl='your_queue_url',
MessageBody='Hello from AWS SQS!'
)
print("Message sent:", response['MessageId'])
Step 3: Receive Messages from the Queue
# Receive message from the queue
response = sqs.receive_message(
QueueUrl='your_queue_url',
MaxNumberOfMessages=1
)
if 'Messages' in response:
message = response['Messages'][0]
print("Received message:", message['Body'])
# Delete the message from the queue
sqs.delete_message(
QueueUrl='your_queue_url',
ReceiptHandle=message['ReceiptHandle']
)
else:
print("No messages available")
Conclusion
AWS Simple Queue Service (SQS) provides a reliable, scalable, and fully managed messaging solution for building distributed applications. By decoupling components and leveraging SQS, developers can design resilient systems that can handle varying workloads and maintain high availability. With its ease of use and powerful features, SQS remains a cornerstone of AWS's messaging services, empowering businesses to innovate and scale their applications with confidence.
Subscribe to my newsletter
Read articles from Sumit Mondal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sumit Mondal
Sumit Mondal
Hello Hashnode Community! I'm Sumit Mondal, your friendly neighborhood DevOps Engineer on a mission to elevate the world of software development and operations! Join me on Hashnode, and let's code, deploy, and innovate our way to success! Together, we'll shape the future of DevOps one commit at a time. #DevOps #Automation #ContinuousDelivery #HashnodeHero