Understanding Cargo in Rust: Project Management Made Easy
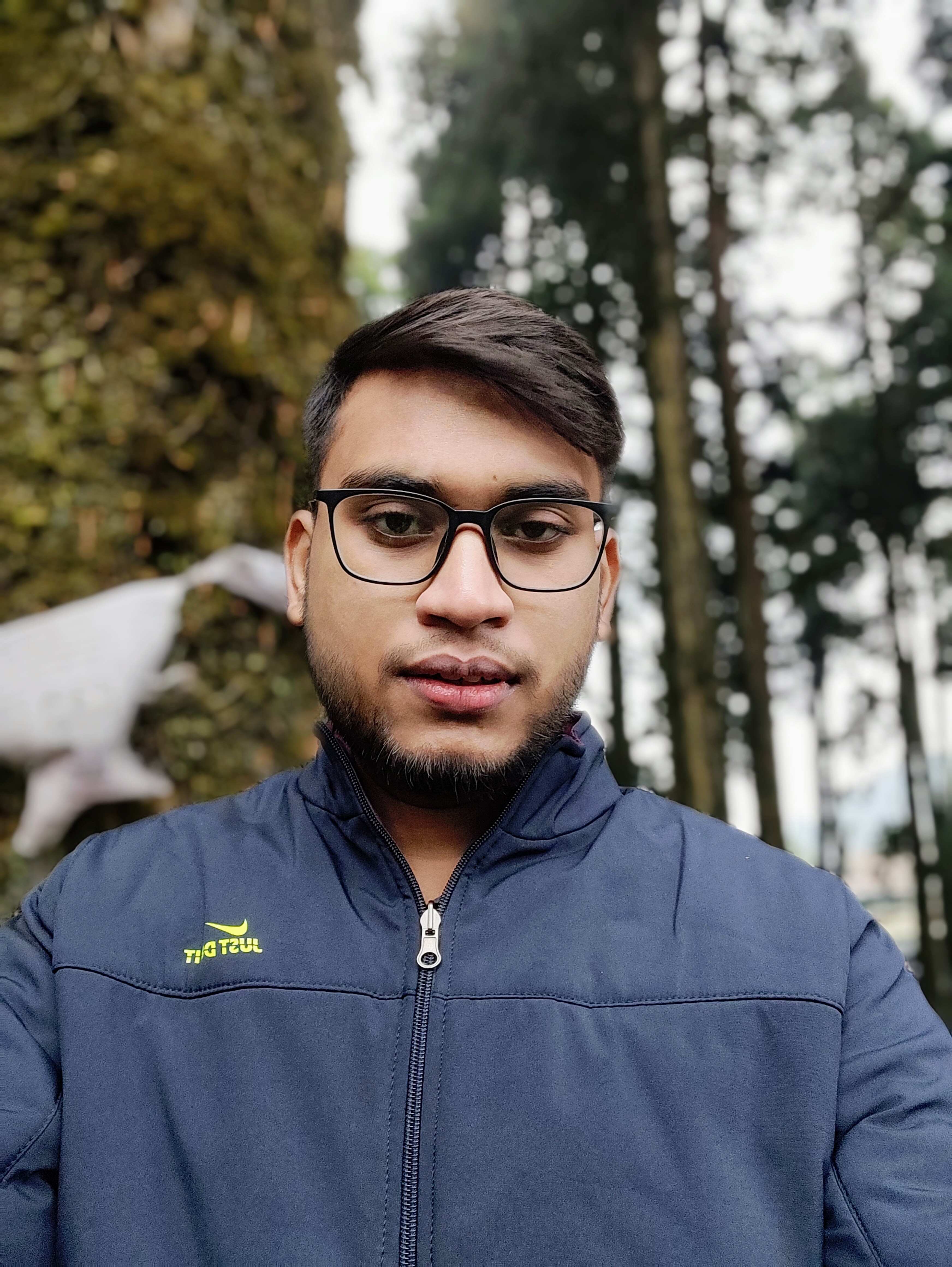
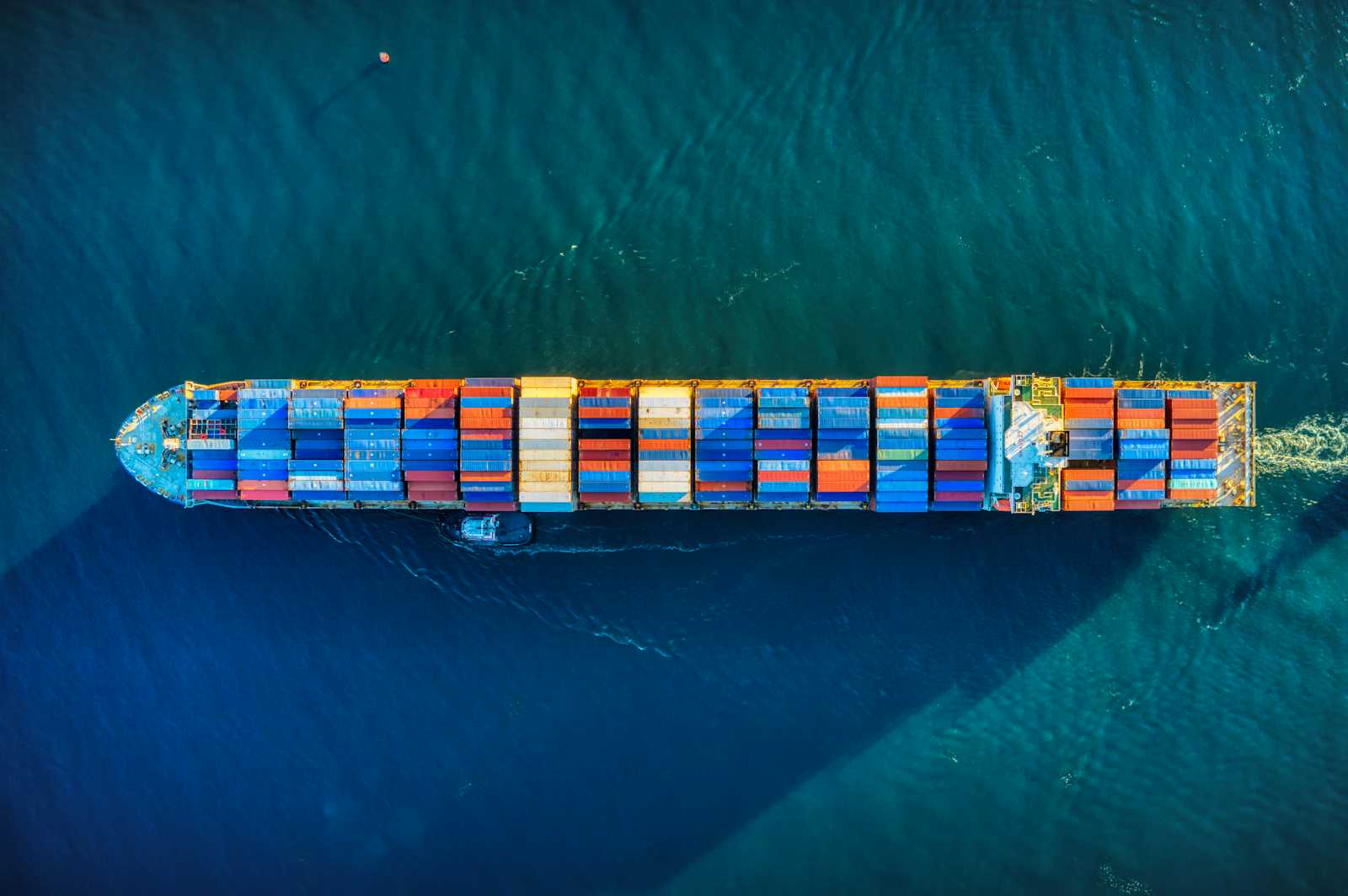
Introduction to Cargo in Rust
Rust is a systems programming language known for its performance, reliability, and memory safety. One of the essential tools in the Rust ecosystem is Cargo, which is the build system and package manager for Rust. In this blog, we will explore what Cargo is, how to use it, and some common issues you might encounter.
What is Cargo?
Cargo is Rust’s build system and package manager. Most Rustaceans (the term used to refer to members of the Rust programming language community) use this tool to manage their Rust projects. It handles tasks such as building your code, downloading the libraries your code depends on (dependencies), and much more. Essentially, Cargo makes the process of developing Rust applications smoother and more efficient.
Why is Cargo Important?
Project Management: It organizes your project structure, making it easier to manage code.
Dependency Management: It automatically fetches and updates your project dependencies.
Build System: It compiles your code and ensures that it runs correctly.
Testing: It helps you run tests on your code to ensure it works as expected.
Getting Started with Cargo
Installing Cargo
Cargo is typically installed as part of the Rust installation process. If you haven't installed Rust yet, you can do so by visiting rust-lang.org and following the installation instructions. Once Rust is installed, Cargo should be available as well.
To verify the installation, open your terminal and run:
cargo --version
This should display the version of Cargo installed on your system.
Creating a New Project
Creating a new Rust project with Cargo is straightforward. Use the following command:
cargo new my_project
cd my_project
This command creates a new directory called my_project
with the following structure:
|-- src
| |-- main.rs
|-- .gitignore
|-- Cargo.toml
Project Structure
src/main.rs: The main source file for your project.
Cargo.toml: The configuration file for your project where you specify dependencies and other metadata.
TOML (Tom’s Obvious, Minimal Language)
.gitignore: A default
.gitignore
file to avoid committing unnecessary files to version control.
Cargo Commands
Basic Commands
Creating a new project:
cargo new <project_name>
Building the project:
cargo build
Running the project:
cargo run
Managing Dependencies
Cargo makes it easy to manage dependencies for your project. You can add, remove, and update dependencies with simple commands.
Adding a dependency: (using
cargo-edit
extension)cargo add <dependency>
Removing a dependency:
cargo remove <dependency>
Updating dependencies:
cargo update
Building and Running Tests
Cargo also helps you manage tests for your project.
Running tests:
cargo test
Cargo.toml
Explained
The Cargo.toml
file is where you define the metadata for your project, including its dependencies. Here’s a breakdown of its important sections:
Overview of Cargo.toml
[package]: Contains metadata about your package, like its name, version, and authors.
[dependencies]: Lists your project’s dependencies.
[dev-dependencies]: Lists dependencies required for development and testing.
Example of Cargo.toml
[package]
name = "my_project"
version = "0.1.0"
authors = ["Your Name <you@example.com>"]
edition = "2018"
[dependencies]
serde = "1.0"
rand = "0.8"
[dev-dependencies]
tempfile = "3.1"
Adding Dependencies Manually
You can manually add dependencies to your Cargo.toml
file. For example, to add the serde
and rand
crates:
[dependencies]
serde = "1.0"
rand = "0.8"
After adding dependencies, run cargo build
to fetch and compile them.
Advanced Cargo Features
Workspaces
Cargo workspaces allow you to manage multiple packages within a single project. This is useful for large projects with many interdependent components.
To create a workspace, add a Cargo.toml
file at the root of your workspace with the following content:
[workspace]
members = [
"crate1",
"crate2",
]
Custom Build Scripts
You can customize the build process with a build.rs
file. This file is executed before your package is compiled and can be used to perform tasks such as code generation or setting environment variables.
Using Features
Features are optional dependencies that can be enabled or disabled. They allow you to customize your package for different use cases.
Example in Cargo.toml
:
[features]
default = ["serde"]
advanced = ["serde", "rand"]
Common Issues and Troubleshooting
Common Error Messages
"dependency not found": Ensure the dependency is spelled correctly and exists in the crates.io registry.
"build failed": Check the error message for details. It might be a syntax error or a missing dependency.
Frequently Asked Questions on the Internet
How do I add a local dependency in Cargo?
To add a local dependency, specify the path in
Cargo.toml
:[dependencies] my_local_crate = { path = "../my_local_crate" }
What is the difference between
dependencies
anddev-dependencies
?dependencies
are needed for running your application, whiledev-dependencies
are only required for development and testing.How do I resolve version conflicts in Cargo?
Cargo.lock helps ensure that everyone working on the project uses the same versions of dependencies. You can manually update or resolve conflicts in the
Cargo.toml
file.How to handle large projects with Cargo?
Use
workspaces
to manage multiple packages within a single project.Why does my Cargo build fail with a cryptic error message?
Look up the error message in the Rust error index or on StackOverflow for solutions.
Useful Resources
Official Rust Cargo Book: https://doc.rust-lang.org/cargo/index.html
StackOverflow: https://stackoverflow.com/questions/tagged/rust-cargo
Rust Community Forum: https://users.rust-lang.org/
Conclusion
Cargo is an essential tool for managing Rust projects. It simplifies project management, dependency handling, and the build process, making it easier to focus on writing code. By understanding and using Cargo effectively, you can streamline your development workflow and maintain a consistent project structure.
For further reading, check out the official Cargo documentation and other useful resources!
Goodbye for now! I hope this introduction to Cargo in Rust has been helpful and informative. Stay tuned for my next blog where we'll dive deeper into Rust programming and explore more advanced topics. If you have any questions or comments, feel free to leave them below.
Subscribe to my newsletter
Read articles from SOUMITRA-SAHA directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
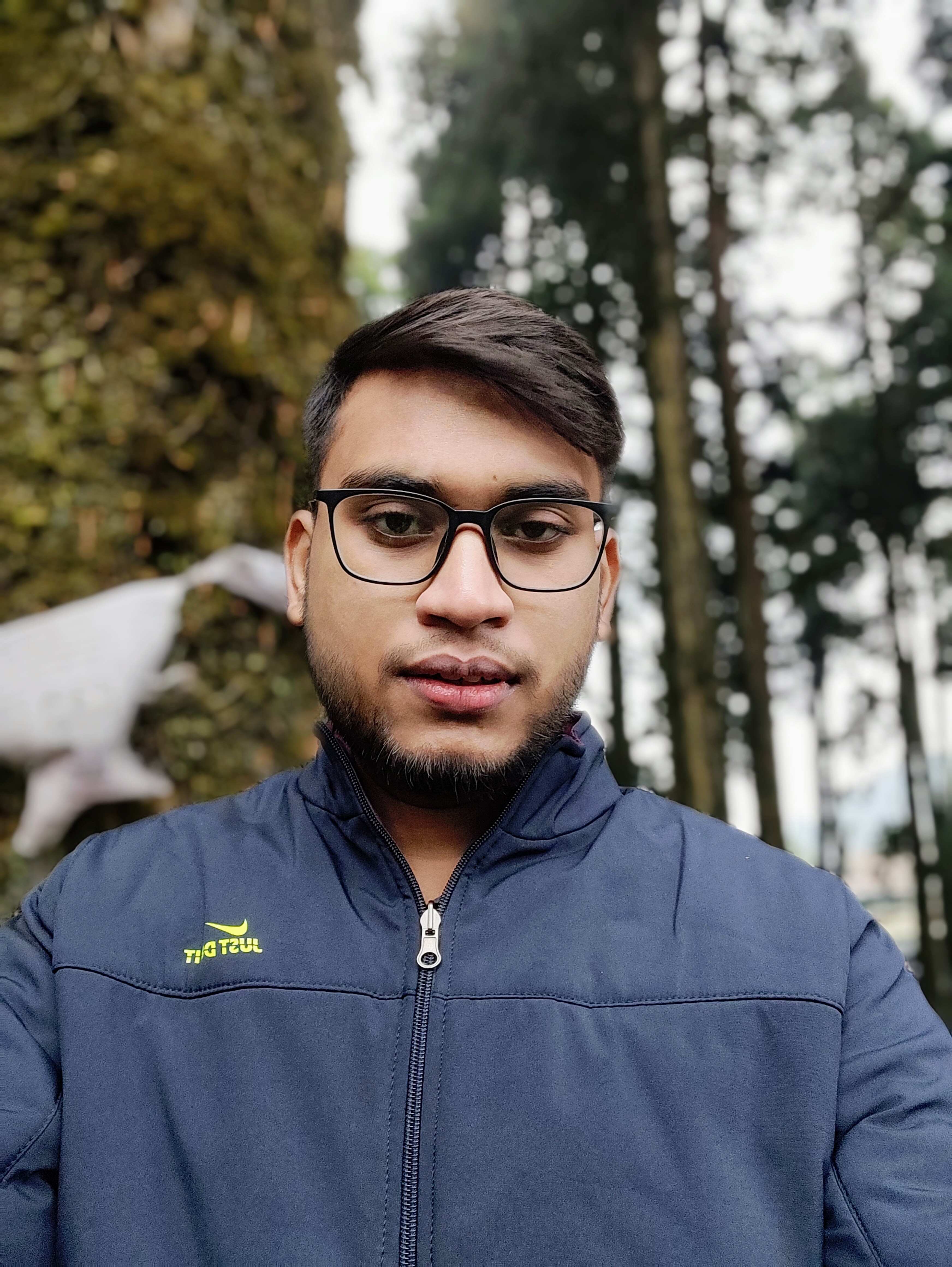
SOUMITRA-SAHA
SOUMITRA-SAHA
About Me I am a Full-Stack Developer with approximately two years of experience in the industry. My expertise spans both front-end and back-end development, where I have worked extensively to build and maintain dynamic, responsive web applications. Technical Skills Front-End Development: HTML, CSS, JavaScript, Typescript Frameworks: React, NextJs, Remix, Vite Libraries: TailwindCSS, Bootstrap Tools: Webpack, Babel Back-End Development: Languages: Typescript, Node.js, Python, Go, Rust Frameworks: NextJS, Remix, Express.js, Django, Go (Gin), Rust Databases: PostgreSQL, MySQL, MongoDB Tools: Docker, Kubernetes DevOps: Version Control: Git, GitHub CI/CD: Jenkins, CircleCI Infrastructure as Code: Terraform Cloud Services: AWS, Lamda Other Skills: RESTful API Development Test-Driven Development (TDD), (vitest, jest, Cypress) Agile Methodologies Personal Interests I am passionate about learning new technologies and keeping up with industry trends. I enjoy exploring innovative solutions to complex problems and continuously seek opportunities to expand my expertise. In my free time, I engage with the developer community, contributing to open-source projects and participating in tech meetups.