Passing data between components

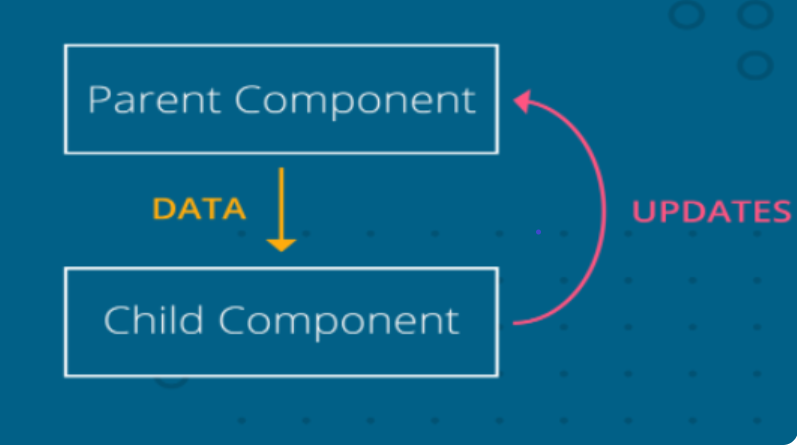
In React sharing data between components is common ,There are several ways to do so :-
Props
Context API
Redux or any other state management Tool
Although In this blog we will be studying about Props and How to pass data between Components using Props
Unidirectional Binding
Unidirectional Binding means data will flow in only one direction that is from parent component to child component. Although there are ways to send data from child component to parent component which we study later.
The data from the parent is known as props.
Data with props is read-only, i.e the data coming from the parent component must not be changed or altered by the child components. This makes sure that a clean data flow architecture is followed. This also means that you can control the data flow better.
Props
Props offer a method to transfer data between components. They are passed to a component similarly to how arguments are passed to a function.
They are used the same way attributes are used in HTML.
One thing to note about Props is Props are immutable by design. This means that once a prop is passed to a component, it cannot be changed by that component.
To Know more about Props , Read My blog on Props Link
Passing Data from Parent to Child
In this the flow of data is top-down.
Data is passed from Parent to Child using Props,Props are used the same way attributes are used in HTML.
function ParentComponent(){
var obj={
name:"Max",
age:12}
return(
<>
<ChildComponent data1={obj} data1="send any data you want"/>
</>
)}
function ChildComponent ({data1,data2}){
return(
<>
{data1.name}//Max
{data2}//send any data you want
</>
)
}
Passing Data from Child to Parent
Now that we can send data from our parent component to our child let's see how it would work the other way around.
React does not allow the bottom-up flow of data as it will not be unidirectional.
What we can do is pass a callback function to child component as prop and recieve data from that callback function.
This might be confusing so here are the steps to follow:-
Create a callback in the parent component which takes in the data needed as a parameter.
Pass this callback as a prop to the child component.
Send data from the child component using the callback.
import React, { useState } from 'react';
function ParentComponent() {
const [DataFromChild, setDataFromChild] = useState("I am Parent");
let callBack = (dataFromChild) => {
setDataFromChild(dataFromChild);
};
return (
<>
{DataFromChild}
<ChildComponent callbackfn={callBack} />
</>
);
}
function ChildComponent({ callbackfn }) {
return (
<>
<button onClick={() => { callbackfn("I am Child"); }}>Click me</button>
</>
);
}
so this is how we can send data from child to parent.
Thanks for Reading!!!
Kindly Share, like and comment your thoughts!
Follow me on LinkedIn
Subscribe to my newsletter
Read articles from Abhinandan Vashisht directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Abhinandan Vashisht
Abhinandan Vashisht
I am a computer science student who is passionate about web devlopment. I have expertise in MERN stack and is looking forward to sharing my knowledge