Exploring Java : My Learning Adventure Part - 02
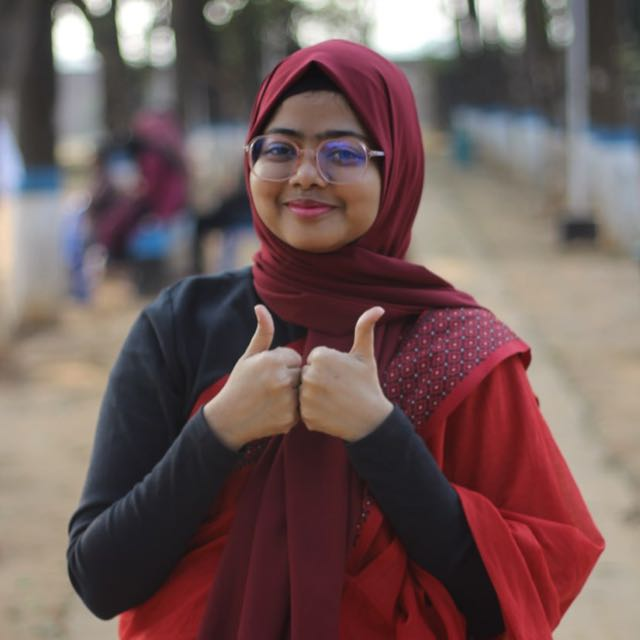
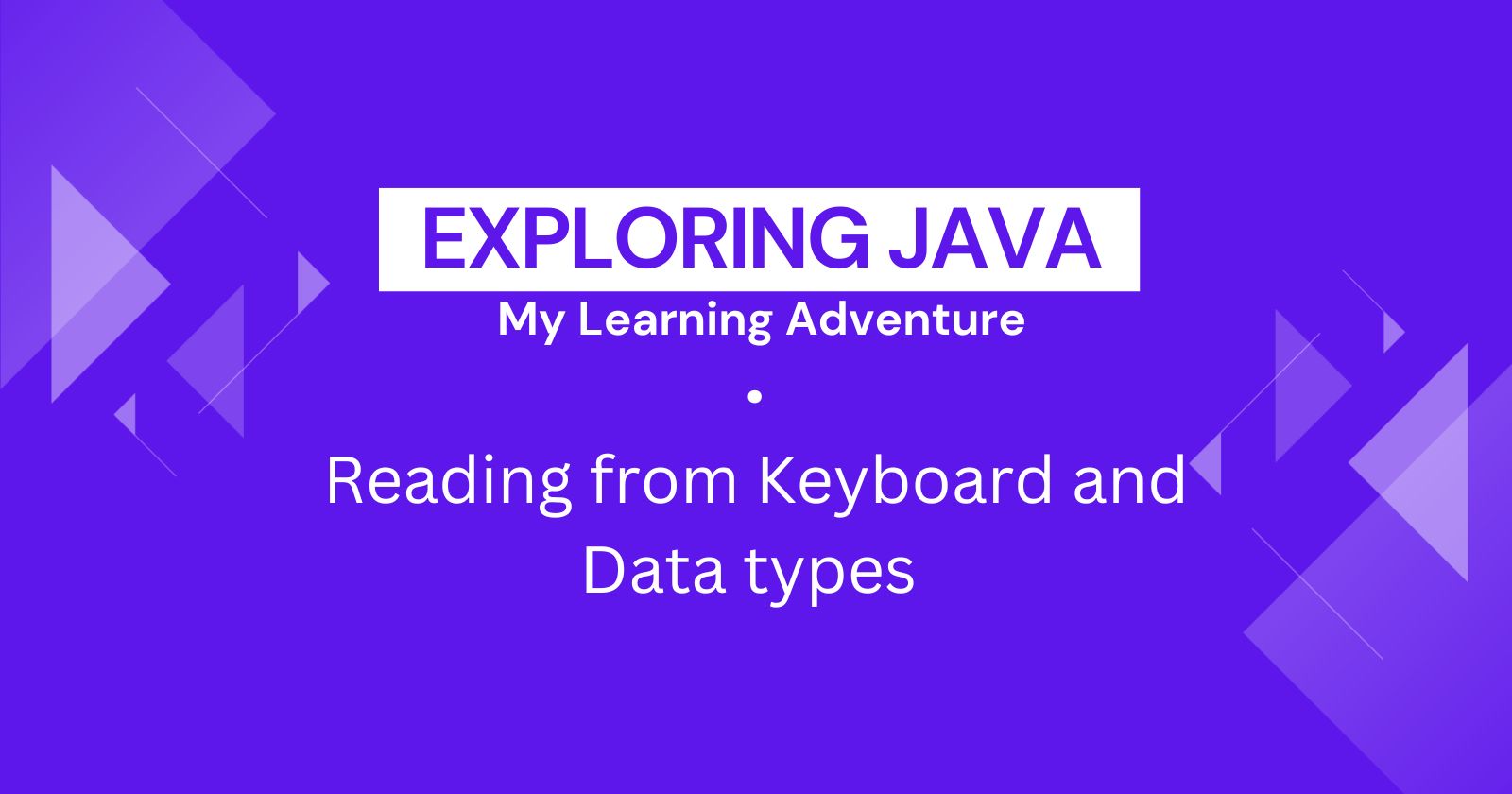
Welcome back! I hope you have read my first blog on Java. If not, here's the link to the blog https://mahia.hashnode.dev/exploring-java-my-learning-adventure
Okay so let's start with reading from keyboard
Reading from Keyboard
Reading from the keyboard means capturing user input during the execution of a program.Just like in C language scanf
is used for reading data, in C++ cin
is used for reading data, in Python input()
is used for reading data, similarly, in Java Scanner
is used for reading data. Scanner is basically a class that will allow you to read data from different sources. Let's focus on keyboard being the source of data here.
Scanner
is a part of the util
package. To use the class, first import the util
package, import java.util.Scanner;
, then create an object. (I have attached demo, scroll down)
The Scanner
class in Java has has various methods to read different types of data from the keyboard. Let me show you some :
next()
Usage:
String input =
scanner.next
();
Description: Reads the next token (word) from the keyboard.
nextInt()
Usage:
int number = scanner.nextInt();
Description: Reads the next token as an integer. If the input is not a valid integer, it throws an
InputMismatchException
.
nextFloat()
Usage:
float number = scanner.nextFloat();
Description: Reads the next token as a float. If the input is not a valid float, it throws an
InputMismatchException
.
nextBoolean()
Usage:
boolean value = scanner.nextBoolean();
Description: Reads the next token as a boolean (
true
orfalse
). If the input is not a valid boolean, it throws anInputMismatchException
.
nextDouble()
Usage:
double number = scanner.nextDouble();
Description: Reads the next token as a double. If the input is not a valid double, it throws an
InputMismatchException
.
nextLine()
Usage:
String input = scanner.nextLine();
Description: Reads a full line of text from the keyboard until the user presses Enter.
hasNextInt()
Usage:
boolean hasInt = scanner.hasNextInt();
Description: Checks if the next token is an integer. Returns
true
if it is,false
otherwise.
hasNextFloat()
Usage:
boolean hasInt = scanner.hasNextFloat();
Description: Checks if the next token is an float. Returns
true
if it is,false
otherwise.
hasNextDouble()
Usage:
boolean hasDouble = scanner.hasNextDouble();
Description: Checks if the next token is a double. Returns
true
if it is,false
otherwise.
nextLong()
Usage:
long number = scanner.nextLong();
Description: Reads the next token as a long. If the input is not a valid long, it throws an
InputMismatchException
.
nextShort()
Usage:
short number = scanner.nextShort();
Description: Reads the next token as a short. If the input is not a valid short, it throws an
InputMismatchException
.
nextByte()
Usage:
byte number = scanner.nextByte();
Description: Reads the next token as a byte. If the input is not a valid byte, it throws an
InputMismatchException
Let's write a code in Java using nextInt()
Scanner method.
import java.util.*,
class Keyboard
{
public static void main(String[] args)
{
Scanner sc = new Scanner(System.in); // Declaring variable
int a,b,c ;
System.out.println('Enter two numbers)
a = sc.nextInt();
b = sc.nextInt();
c = a+b ;
System.out.println("Sum is " + c) ;
}
}
Basically, whatever you type it comes through the Scanner class. Scanner class is the bridge between your program and keyboard.
Data types
Data is the main part of a program. When a program runs in the memory, it holds the data temporarily in the memory rather than storing it permanently on the hard disk. So where is the data being stored? It is being stored in variables. Variables are meant for storing data.
Primitive data are built-in data types. They are predefined by the language.
Java has eight primitive data types. Each has a specific purpose, size, and value range. Here’s a brief overview of each:
1. byte
Size: 8-bit (1 byte)
Range: -128 to 127
Default Value: 0
Usage: Useful for saving memory in large arrays where the memory savings are most needed.
2. short
Size: 16-bit (2 bytes)
Range: -32,768 to 32,767
Default Value: 0
Usage: Can be used to save memory in large arrays, similar to
byte
.
3. int
Size: 32-bit ( 4 bytes)
Range: -2^31 to 2^31-1 (-2,147,483,648 to 2,147,483,647)
Default Value: 0
Usage: Most commonly used integer type for general-purpose integer arithmetic.
4. long
Size: 64-bit (8 bytes)
Range: -2^63 to 2^63-1 (-9,223,372,036,854,775,808 to 9,223,372,036,854,775,807)
Default Value: 0L you have to append L to let the compiler know
Usage: Used when a wider range than
int
is needed.
5. float
Size: 32-bit IEEE 754 floating point
Range: Approximately ±3.40282347E+38F (6-7 significant decimal digits)
Default Value: 0.0f you have to append f to let the compiler know
Usage: Use for saving memory in large arrays of floating point numbers. Not recommended for precise values such as currency.
6. double
Size: 64-bit IEEE 754 floating point
Range: Approximately ±1.79769313486231570E+308 (15 significant decimal digits)
Default Value: 0.0d you have to append d to let the compiler know
Usage: Default data type for decimal values. Generally, the default choice for decimal values.
7. boolean
Size: Not precisely defined (depends on JVM)
Range:
true
orfalse
Default Value:
false
Usage: Use for simple flags that track true/false conditions.
8. char
Size: 16-bit Unicode character
Range: '\u0000' (or 0) to '\uffff' (or 65,535)
Default Value: '\u0000'
Usage: Used to store any character.
Variables
What are Variables?
Variables are names given to data. Each variable must have a specific data type, like byte
, int
, float
, etc. The data type must be declared before the variable can be used. For example, you cannot write x int = 20
; you need to declare the data type first, like this: int x = 20
.
The variable will take up space as per to its data type. byte
takes up 8 bits where int
takes up 32 bits, got it?
Variable naming rules
Case sensitive : Lower case and upper case are different
Symbols : Alphabet, numbers and only two special character
_
and$
can be used. No other that thisStart : variable name must start with a alphabet
Should not be a keywords
Don't use class name
it’s good practice to use descriptive names that indicate the variable's purpose.
Follow the Camel cases
Literals
literals are the fixed values assigned to variables. They represent the constant values assigned in the code. 5 is an int literal. Any value with a decimal point is double. Any value without a decimal is an integer. 'A' is a char literal, and "A" is a string literal. The difference lies within the quotes. If it's a single quote, then it's a character, and if it's double, then it's a string.
Literals | types |
byte | int |
short | int |
int | int |
long | L or l |
float | F or f |
double | D or d |
char | ' ' |
Boolean | True/False |
byte b = 5;
is a byte data type. short s = 25;
is a short data type. But what about int? How do you state an int number? Okay, first, let's see other data type declarations. If you have any int that ends with 5L
, then it's not an int, it's a long literal. 2.5
is by default a double literal. To make it float, you have to append F/f, 2.5F
. That's how you declare literals. Even float f='p';
is possible too.
import java.lang.*;
class Literal
{
public static void main(String[] args)
{
byte b = 5;
System.out.println(b);
int x = 5555;
System.out.println(x);
short s = 5;
System.out.println(s);
long l = 9999999999999999999999999999999999999999999999999999999999999L
System.out.println(l);
float f = 12.23F
System.out.println(f);
double d = 12.23
System.out.println(d);
}
}
That's it for today and be sure to try them out.
In the next blog, i will talk about Operator and Expression.
In the meantime, don't forget to stay hydrated! Happy learning!
Subscribe to my newsletter
Read articles from Fatima Jannet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
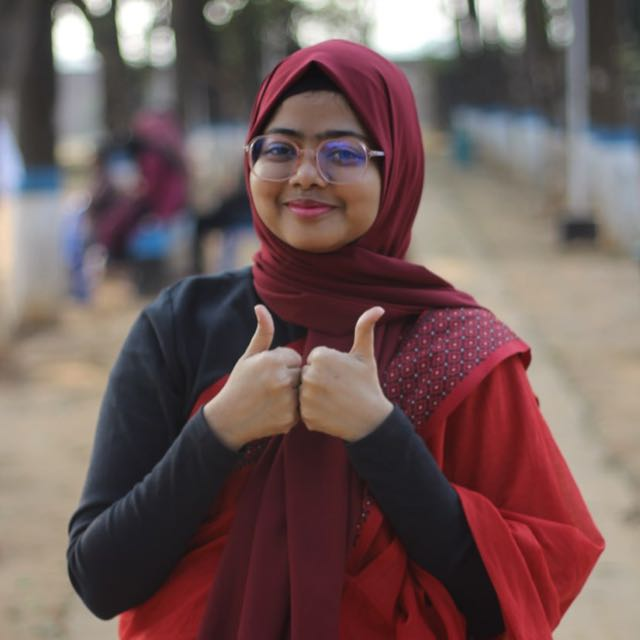