Exploring Solidity: Understanding and Using State Variables
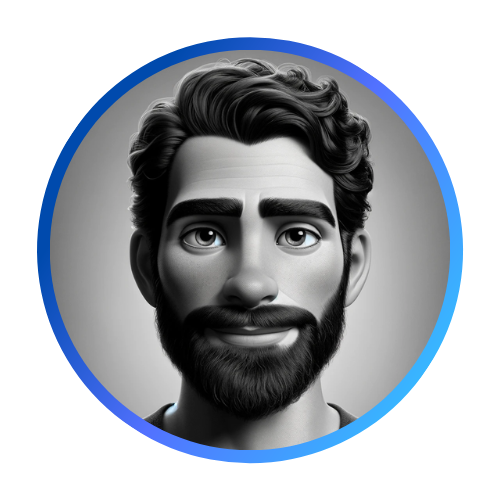
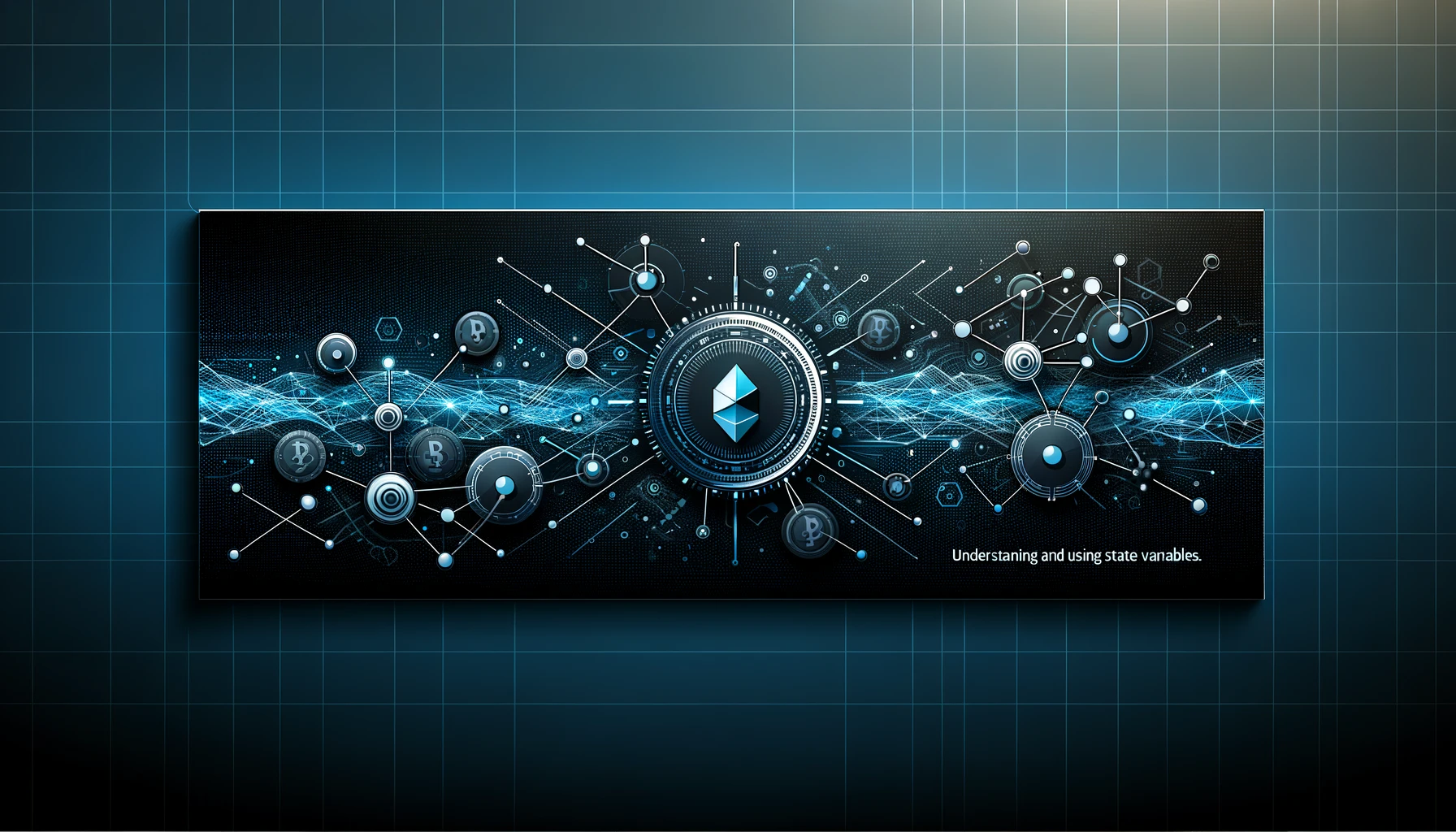
State variables in Solidity are akin to the backbone of your smart contracts, storing data on the blockchain permanently. If you're diving into Solidity, understanding state variables is crucial. In this tutorial, we’ll walk through what state variables are and how to use them, with hands-on examples to illustrate their functionality.
What Are State Variables?
State variables are variables whose values are stored on the blockchain. These values persist between function calls and transactions, making them essential for maintaining the state of your contract.
Example Contract with State Variables
Let's start with a simple example to demonstrate how state variables work. Here’s the Solidity code:
// SPDX-License-Identifier: MIT
pragma solidity >=0.5.0 <0.9.0;
contract StateVariable {
uint public age; // Public state variable
// Constructor to initialize the state variable
constructor() public {
age = 2;
}
// Function to set a new age
function setAge(uint _age) public {
age = _age;
}
}
Understanding the Code
The SPDX
license identifier at the top of the code ensures clarity about the license type, while the pragma
directive specifies the Solidity version for which the code is written, ensuring compatibility with different compiler versions.
Next, we declare our contract with contract StateVariable {
, laying down the foundation for our smart contract named StateVariable
.
Inside the contract, we define our state variable with uint public age;
. This line sets up a public state variable, which automatically generates a getter function, allowing other contracts and external users to access its value.
The constructor, constructor() public { age = 2; }
, initializes the age
variable when the contract is deployed, setting its value to 2. Constructors are special functions that run only once during the contract deployment.
Finally, the function function setAge(uint _age) public { age = _age; }
allows updating the value of the age
variable. It takes a new age as an input and updates the state variable accordingly.
Wrapping Up
State variables are a fundamental aspect of Solidity and smart contract development. They store persistent data on the blockchain, making them essential for creating robust and functional smart contracts. As you continue your journey into blockchain development, understanding how to use state variables efficiently will be invaluable.
References:
Written by ljb630 | Trying to find my place in the web3 community! | Technical Writer & Researcher | Team Lead
Subscribe to my newsletter
Read articles from ljb630 directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
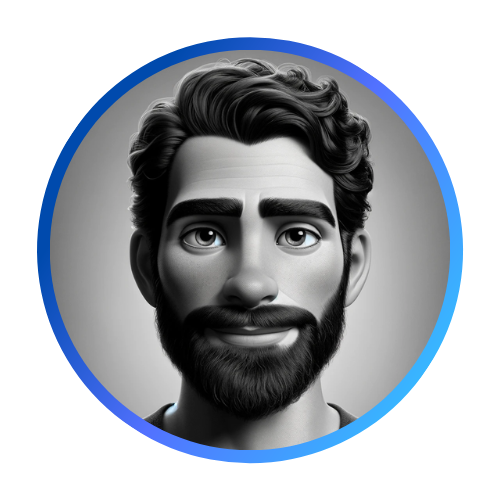
ljb630
ljb630
Trying to find my place in the web3 community! | Technical Writer & Researcher | Team Lead