Understanding Why We Use LinkedList<Integer> Instead of LinkedList<int> in Java
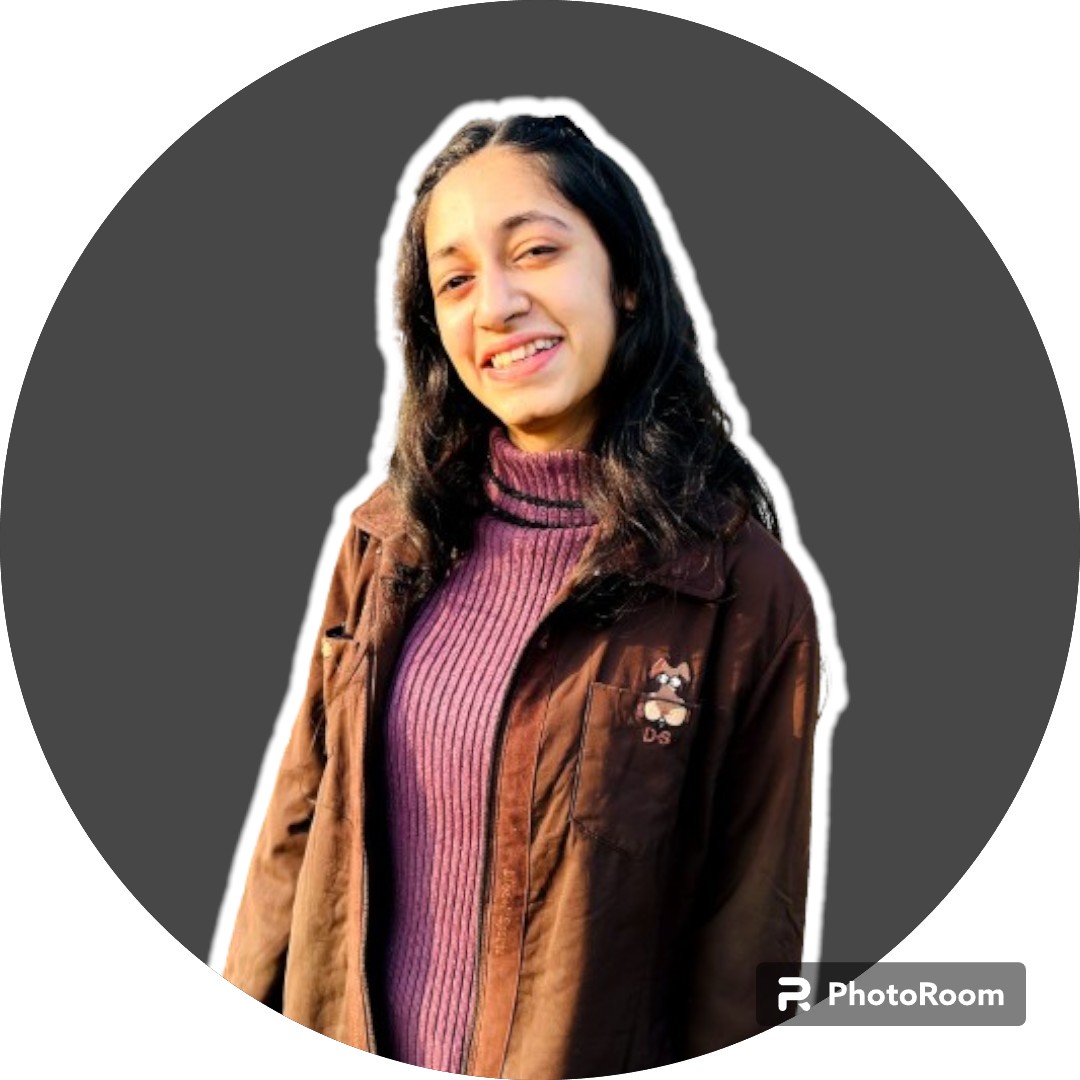
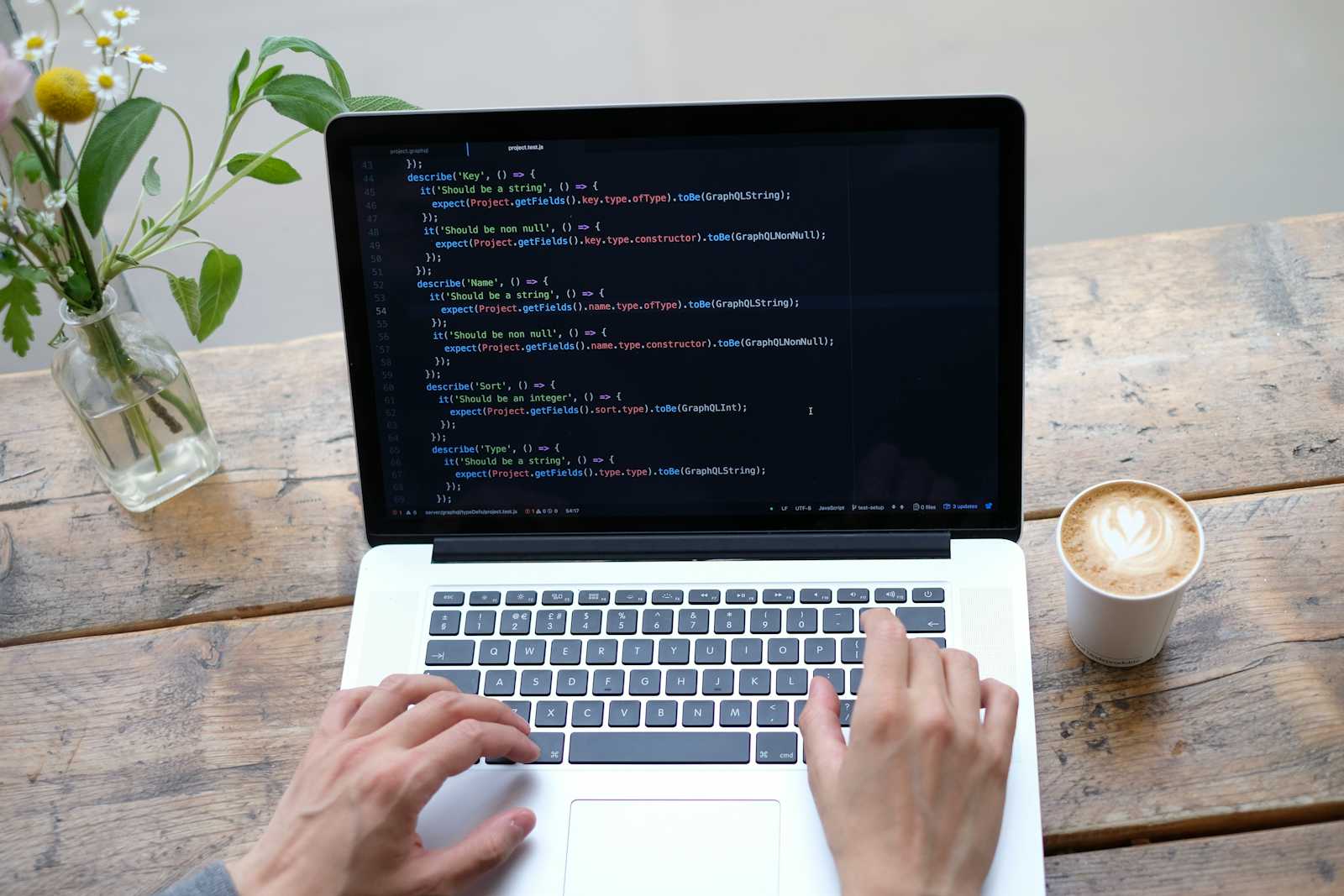
When diving into Java programming, especially when dealing with collections, one might wonder why we use LinkedList<Integer>
instead of LinkedList<int>
. This question touches on some fundamental concepts in Java that revolve around generics, primitive types, and wrapper classes. Let’s explore these concepts and understand the rationale behind this design choice.
Java Generics: The Backbone of Type Safety
Java introduced generics to ensure type safety and to eliminate the need for type casting. When we declare a generic collection like LinkedList<T>
, we’re defining a collection that can hold elements of any specified type T
. However, there’s a catch: generics in Java work only with reference types (objects) and not with primitive types.
Primitive Types vs. Reference Types
Java distinguishes between primitive types (like int
, char
, boolean
) and reference types (objects). Primitive types are basic data types that are not objects and hold their values directly in memory. Reference types, on the other hand, refer to objects that are instances of classes.
The Role of Wrapper Classes
To bridge the gap between primitive types and the need for objects in generics, Java provides wrapper classes for each primitive type. These wrapper classes are part of the java.lang
package and encapsulate primitive values in an object. For instance:
int
->Integer
char
->Character
boolean
->Boolean
Autoboxing and Unboxing: Making Life Easier
Java simplifies the conversion between primitives and their corresponding wrapper objects through autoboxing and unboxing. Autoboxing automatically converts a primitive value to its wrapper class object when needed, while unboxing converts the wrapper class object back to a primitive value.
Consider this example:
LinkedList<Integer> list = new LinkedList<>();
list.add(5); // Autoboxing: converts int 5 to Integer.valueOf(5)
int value = list.get(0); // Unboxing: converts Integer back to int
In this example, Java handles the conversion between int
and Integer
seamlessly, allowing us to work with primitive values in a generic collection without manually wrapping and unwrapping them.
Practical Example: Using LinkedList<Integer>
Here’s how you can use LinkedList<Integer>
in a real-world scenario:
import java.util.LinkedList;
public class Main {
public static void main(String[] args) {
// Create a LinkedList of Integer
LinkedList<Integer> list = new LinkedList<>();
// Add elements to the list
list.add(1); // Autoboxing converts int to Integer
list.add(2);
list.add(3);
// Access elements from the list
int firstElement = list.get(0); // Unboxing converts Integer to int
int secondElement = list.get(1);
int thirdElement = list.get(2);
// Print elements
System.out.println(firstElement); // Output: 1
System.out.println(secondElement); // Output: 2
System.out.println(thirdElement); // Output: 3
}
}
Why Not LinkedList<int>
?
The fundamental reason we cannot use LinkedList<int>
is that generics in Java are designed to work with objects, not primitives. Since int
is a primitive type, it cannot be used directly as a type parameter in a generic class. Instead, we use Integer
, the wrapper class for int
, which satisfies the requirement for a reference type.
Conclusion
Understanding the distinction between primitive types and reference types, along with the roles of wrapper classes and the concepts of autoboxing and unboxing, clarifies why LinkedList<Integer>
is used instead of LinkedList<int>
. This design ensures type safety, leverages the power of generics, and maintains the flexibility and robustness of Java’s type system.
So, the next time you’re working with collections in Java, you’ll know exactly why LinkedList<Integer>
is the way to go when dealing with integer values. Happy coding!
Feel free to share your thoughts or ask any questions in the comments. Let’s continue exploring the fascinating world of Java programming together! #Java #Programming #Generics #LinkedList #Hashnode
Subscribe to my newsletter
Read articles from Mahak Pandey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
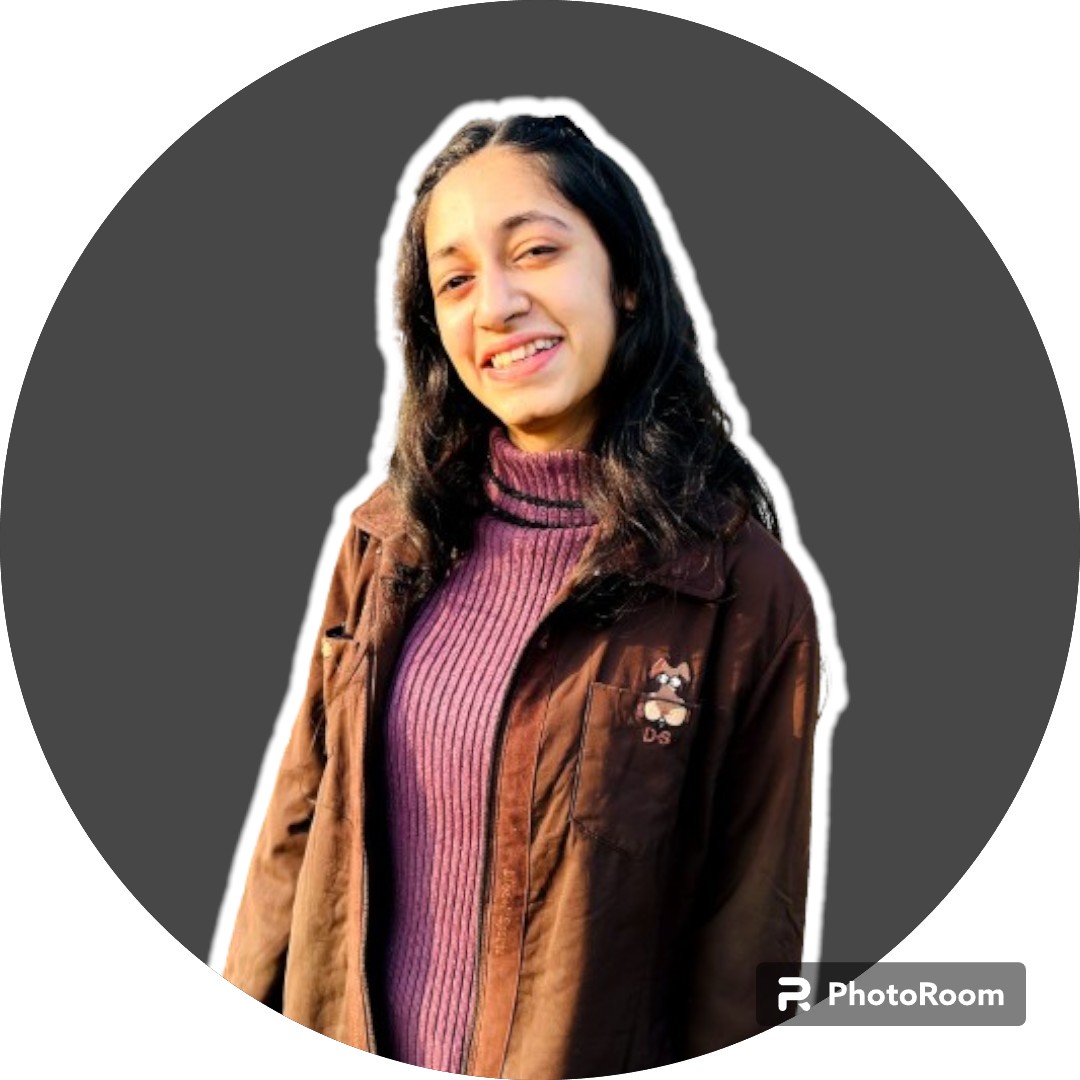
Mahak Pandey
Mahak Pandey
Hey, I am currently a 4th year student majoring in Computer science & Information Technology. I have a strong academic background with coursework in software development, database management, Operating System, OOPS and cybersecurity, and I’ve maintained a CGPA of 9.28. I am proficient in Java, HTML, CSS, Figma, JavaScript, Learning React and core Knowledge Of MERN STack. Additionally, I have hands-on experience with version control systems like Git, and I am familiar with Visual studio code IDE, working experience with Blender for 3D modeling and rendering, Figma for UI design and Prototyping, Canva for Designing assets. Here to share knowledge i've learned and learning. I recently publish the "Cyber Security" Book as a Co-Author. I like to learn and build in public. If you want to connect with me do follow my socials.