Day 15 - Python Libraries for DevOps
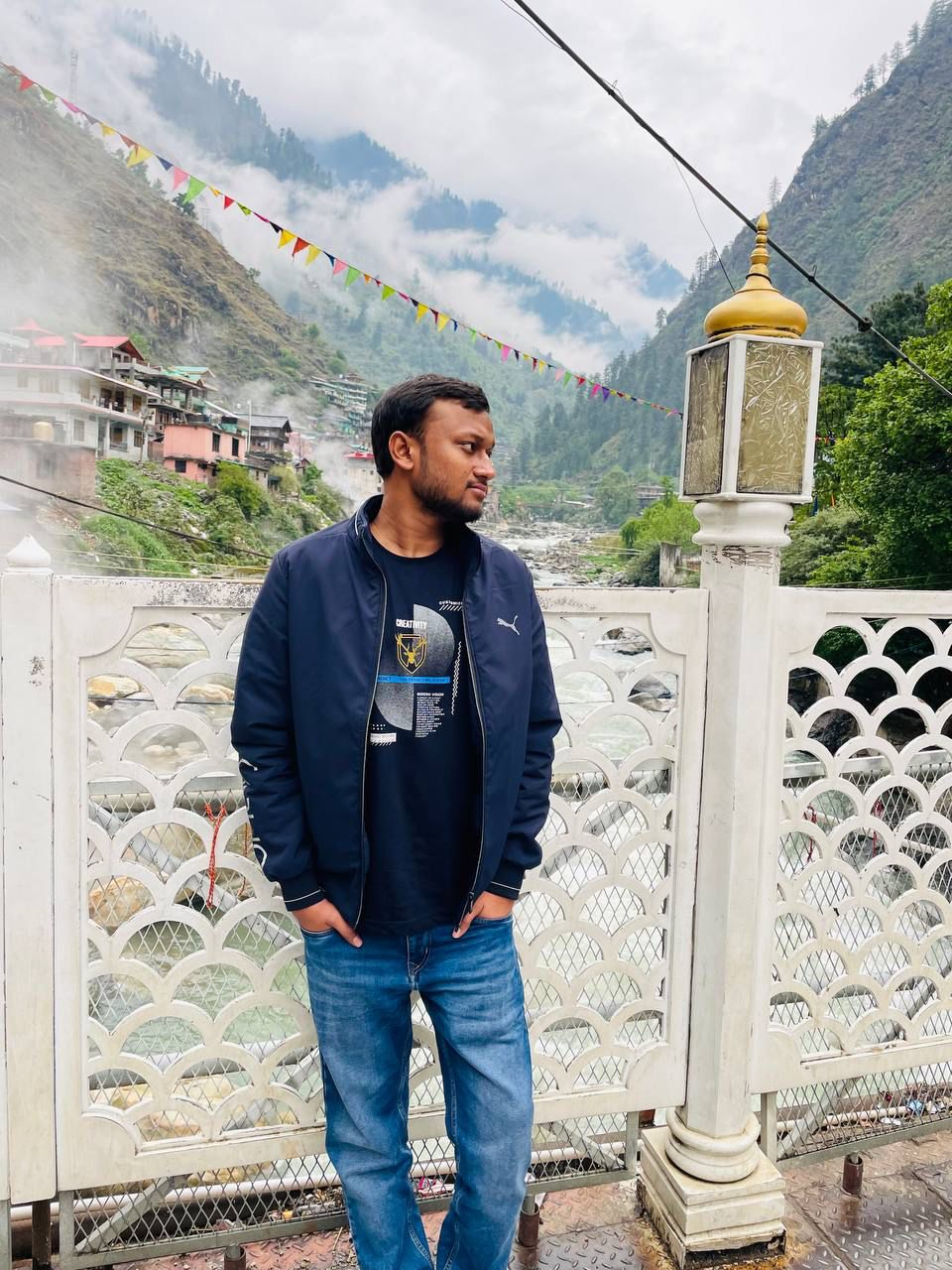
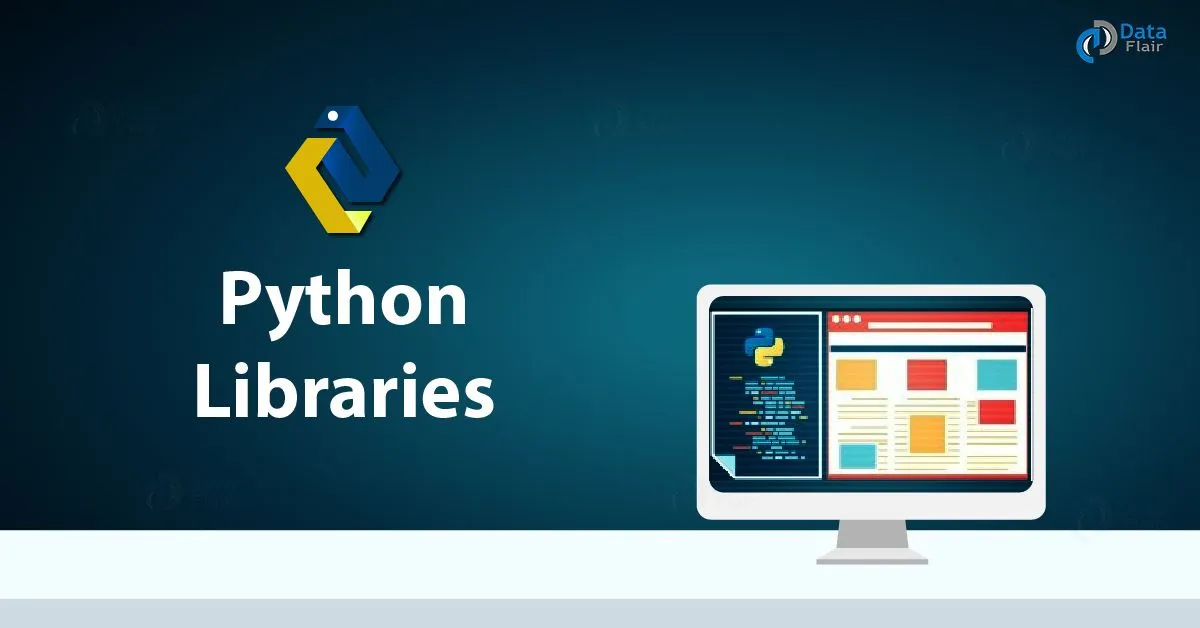
Introduction
As a DevOps Engineer, handling various file formats is part of your daily routine. Among the most common formats you will encounter are JSON and YAML. Python, with its extensive library ecosystem, makes it easy to parse and manipulate these files. In this blog post, we will explore how to work with JSON and YAML files in Python, crucial for automating and streamlining DevOps workflows.
Creating and Writing JSON Files
JSON (JavaScript Object Notation) is a lightweight data-interchange format that is easy for humans to read and write and for machines to parse and generate. Python's built-in json
module provides a simple interface to work with JSON data.
Here’s how you can create a dictionary in Python and write it to a JSON file:
import json
# Create a dictionary
data = {
"aws": "ec2",
"azure": "VM",
"gcp": "compute engine"
}
# Write the dictionary to a JSON file
with open('services.json', 'w') as json_file:
json.dump(data, json_file, indent=4)
print("Dictionary written to services.json")
Reading and Printing JSON Files
Reading a JSON file and extracting its content is straightforward. Here’s an example where we read a JSON file and print out the service names for each cloud provider:
import json
# Read the JSON file
with open('services.json', 'r') as json_file:
services = json.load(json_file)
# Print the service names
for provider, service in services.items():
print(f"{provider} : {service}")
Handling YAML Files
YAML (YAML Ain't Markup Language) is another human-readable data format often used for configuration files. The PyYAML
library in Python allows you to read and write YAML files with ease.
First, install PyYAML
:
pip install pyyaml
Then, use the following script to read a YAML file and convert its content to JSON:
import yaml
import json
# Read the YAML file
with open('services.yaml', 'r') as yaml_file:
yaml_content = yaml.safe_load(yaml_file)
# Convert YAML content to JSON
json_content = json.dumps(yaml_content, indent=4)
# Print JSON content
print(json_content)
Task 1: Create a Dictionary in Python and Write it to a JSON File
First, we create a dictionary and write it to a JSON file.
import json
# Create a dictionary
data = {
"aws": "ec2",
"azure": "VM",
"gcp": "compute engine"
}
# Write the dictionary to a JSON file
with open('services.json', 'w') as json_file:
json.dump(data, json_file, indent=4)
print("Dictionary written to services.json")
Task 2: Read a JSON File and Print Service Names
Next, we read a JSON file services.json
and print the service names for each cloud service provider.
import json
# Read the JSON file
with open('services.json', 'r') as json_file:
services = json.load(json_file)
# Print the service names
for provider, service in services.items():
print(f"{provider} : {service}")
Task 3: Read YAML File and Convert to JSON
For reading a YAML file and converting its contents to JSON, we will use the PyYAML
library.
First, make sure to install the PyYAML library if you haven't already:
pip install pyyaml
Here's the Python script to read a YAML file and convert it to JSON:
import yaml
import json
# Read the YAML file
with open('services.yaml', 'r') as yaml_file:
yaml_content = yaml.safe_load(yaml_file)
# Convert YAML content to JSON
json_content = json.dumps(yaml_content, indent=4)
# Print JSON content
print(json_content)
Conclusion
Mastering these file formats and libraries is essential for any DevOps engineer. Whether you are configuring infrastructure as code, managing deployment scripts, or automating monitoring setups, Python’s json
and PyYAML
libraries provide the tools you need to handle these tasks efficiently.
By incorporating these libraries into your workflow, you can enhance your ability to manage and automate various DevOps tasks, ensuring smooth and reliable operations.
Thank you for reading our DevOps blog post. We hope you found it informative and helpful. If you have any questions or feedback, please don't hesitate to contact us.
I hope this helps!
Happy Learning✨
Subscribe to my newsletter
Read articles from Rahul Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
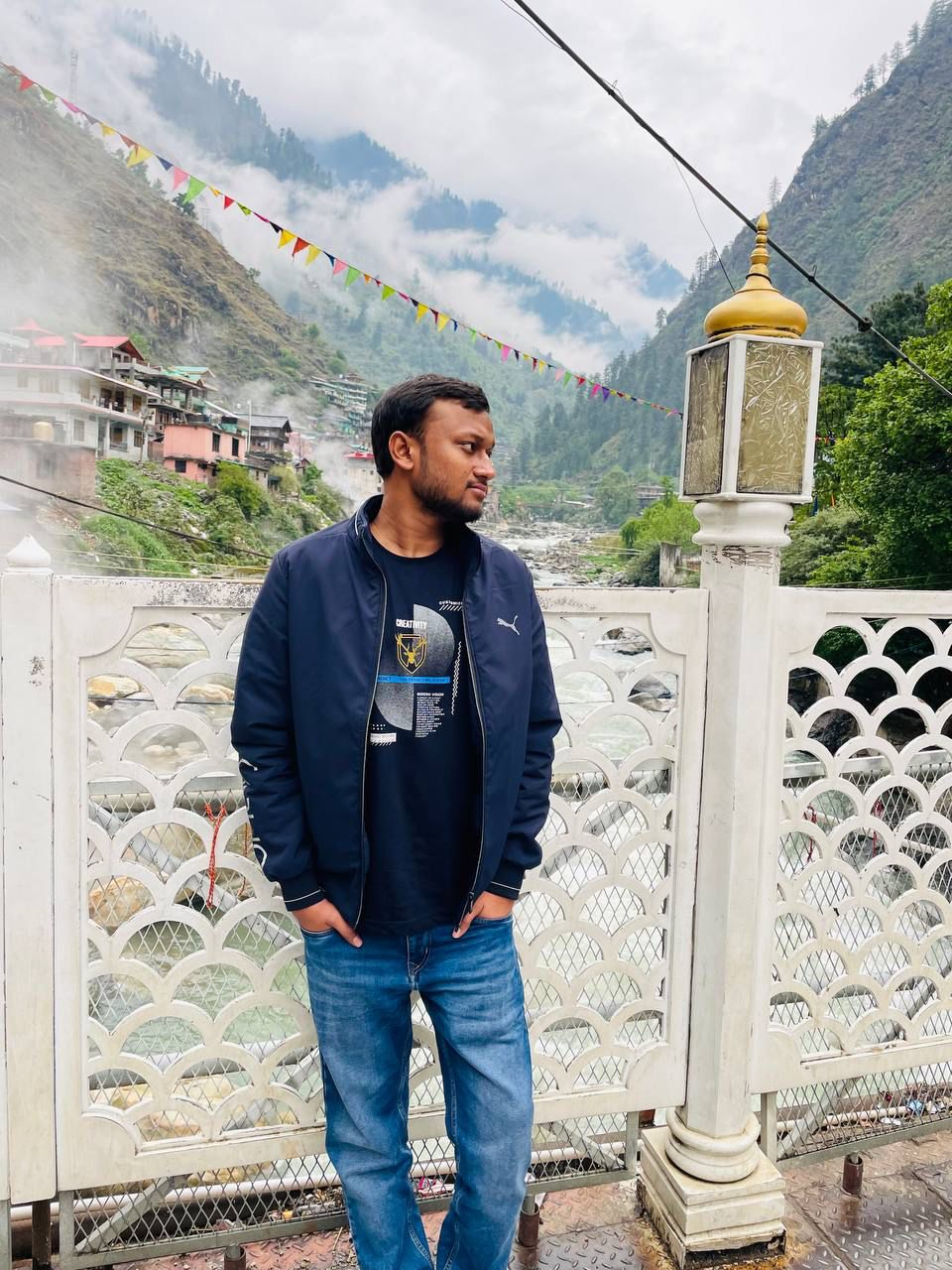
Rahul Gupta
Rahul Gupta
Hey there! 👋 I'm Rahul Gupta, a DevOps Engineer passionate about all things AWS DevOps Technology. Currently, on a learning adventure, I'm here to share my journey and Blogs in the world of cloud and DevOps. 🛠️ My focus? Making sense of AWS services, improving CI/CD, and diving into infrastructure as code. Whether you're fellow interns or curious enthusiasts, let's grow together in the vibrant DevOps space. 🌐 Connect with me for friendly chats, shared experiences, and learning moments. Here's to embracing the learning curve and thriving in the exciting world of AWS DevOps Technology!