JavaScript Basics: Understanding let, var, and const for Freshers
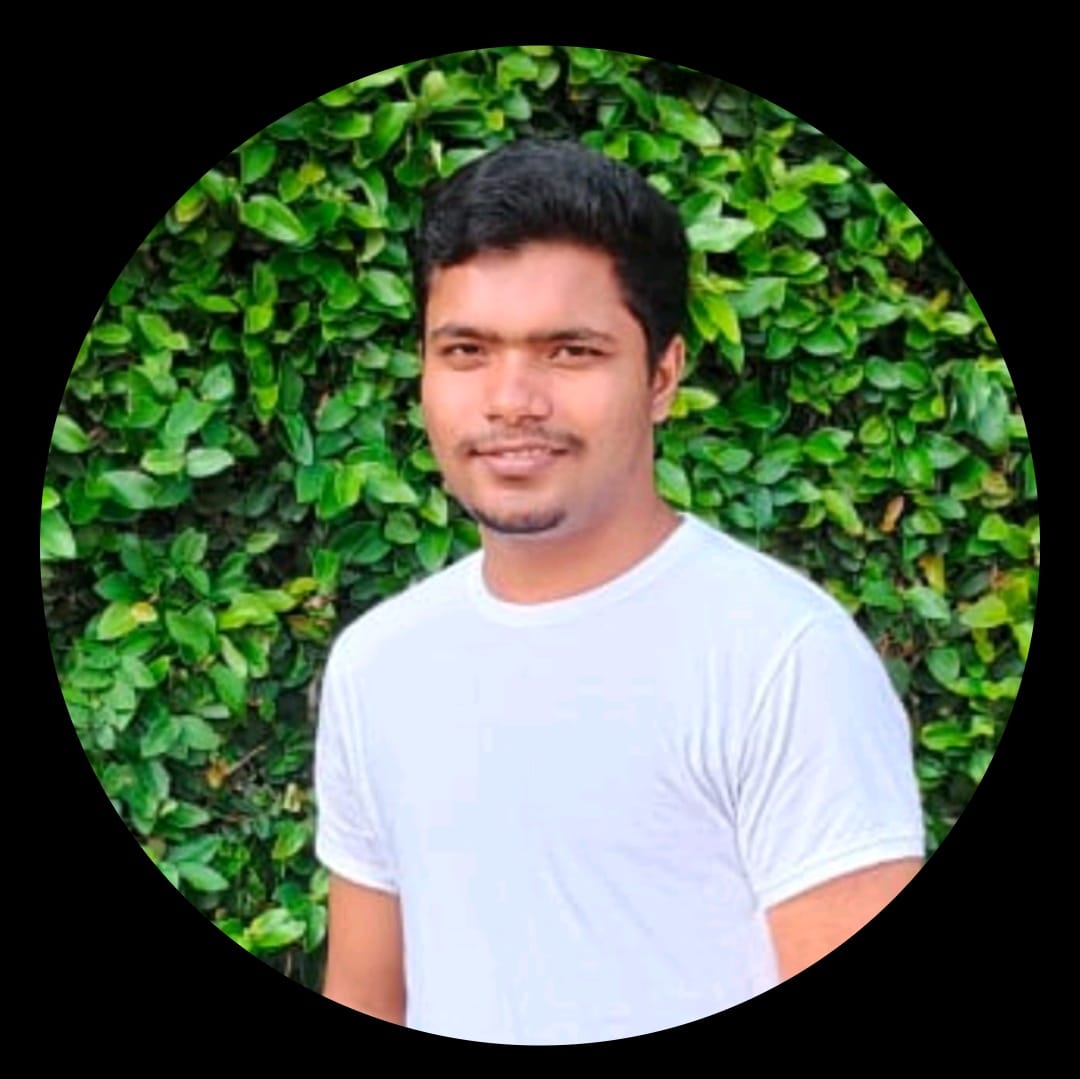
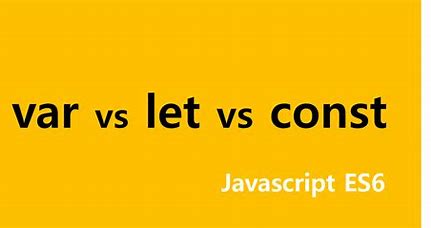
As a fresher preparing for frontend interviews, having a solid understanding of JavaScript's variable declarations—let
, var
, and const
—is crucial. These concepts form the backbone of JavaScript's variable management, scoping, and behavior during code execution.
In this blog post, we’ll delve into these keywords, their differences, and important concepts like variable shadowing, hoisting, and the temporal dead zone (TDZ).
The Basics: let
, var
, and const
var
Scope: Function-scoped.
Hoisting: Declarations are hoisted and initialized with
undefined
.Reassignment: Can be reassigned.
Redeclaration: Can be redeclared within the same scope.
Example:
var x = 10;
console.log(x); // 10
if (true) {
var x = 20;
console.log(x); // 20
}
console.log(x); // 20 (variable is function-scoped, not block-scoped)
let
Scope: Block-scoped.
Hoisting: Declarations are hoisted but not initialized, leading to TDZ until the declaration is encountered.
Reassignment: Can be reassigned.
Redeclaration: Cannot be redeclared within the same scope.
Example:
let y = 10;
console.log(y); // 10
if (true) {
let y = 20;
console.log(y); // 20
}
console.log(y); // 10 (block-scoped)
const
Scope: Block-scoped.
Hoisting: Declarations are hoisted but not initialized, leading to TDZ until the declaration is encountered.
Reassignment: Cannot be reassigned.
Redeclaration: Cannot be redeclared within the same scope.
Example:
const z = 10;
console.log(z); // 10
if (true) {
const z = 20;
console.log(z); // 20
}
console.log(z); // 10 (block-scoped)
Something I found on the internet to summarize the above points.
Variable Shadowing
Variable shadowing occurs when a variable declared within a certain scope has the same name as a variable declared in an outer scope. The inner variable "shadows" the outer variable, making the outer variable inaccessible within the inner scope.
Example:
let a = 5;
function shadowExample() {
let a = 10; // Shadows the outer 'a'
console.log(a); // 10
}
shadowExample();
console.log(a); // 5 (outer variable is unaffected)
Shadowing with var
:
var b = 5;
function varShadowExample() {
var b = 10; // Shadows the outer 'b'
console.log(b); // 10
}
varShadowExample();
console.log(b); // 5 (outer variable is unaffected)
Hoisting
Hoisting is JavaScript's default behavior of moving declarations to the top of the scope before code execution. This applies to both variables and functions but behaves differently for var
, let
, const
, and functions.
var
Hoisting
Variables declared with var
are hoisted to the top of their scope and initialized with undefined
.
Example:
console.log(c); // undefined
var c = 10;
console.log(c); // 10
let
and const
Hoisting
Variables declared with let
and const
are hoisted but not initialized, leading to the Temporal Dead Zone (TDZ).
Example:
console.log(d); // ReferenceError: Cannot access 'd' before initialization
let d = 10;
console.log(d); // 10
console.log(e); // ReferenceError: Cannot access 'e' before initialization
const e = 20;
console.log(e); // 20
Function Hoisting
Function declarations are hoisted entirely, meaning you can call the function before its declaration.
Example:
hoistedFunction(); // "Hello from hoisted function!"
function hoistedFunction() {
console.log("Hello from hoisted function!");
}
Function expressions and arrow functions assigned to variables are not hoisted.
Example:
nonHoistedFunction(); // TypeError: nonHoistedFunction is not a function
var nonHoistedFunction = function() {
console.log("Hello from non-hoisted function!");
};
Temporal Dead Zone (TDZ)
The TDZ is a behavior in JavaScript where variables declared with let
and const
are in a state where they cannot be accessed until they are initialized. This prevents the use of the variable before the actual declaration is encountered in the code.
Example:
console.log(f); // ReferenceError: Cannot access 'f' before initialization
let f = 10;
console.log(f); // 10
console.log(g); // ReferenceError: Cannot access 'g' before initialization
const g = 20;
console.log(g); // 20
Summary
var
: Function-scoped, hoisted withundefined
initialization, allows redeclaration.let
: Block-scoped, hoisted but not initialized (TDZ), no redeclaration.const
: Block-scoped, hoisted but not initialized (TDZ), no redeclaration, no reassignment.Variable Shadowing: Inner variables can shadow outer variables of the same name.
Hoisting: Declarations are moved to the top of their scope;
var
is initialized withundefined
,let
andconst
are not initialized.TDZ: Temporal Dead Zone prevents accessing
let
andconst
variables before their declaration.
Understanding these concepts is fundamental for writing efficient and bug-free JavaScript code. As you prepare for interviews, practice these concepts through coding exercises and real-world examples to solidify your understanding.
Happy Coding!
Subscribe to my newsletter
Read articles from Tahmeer Pasha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
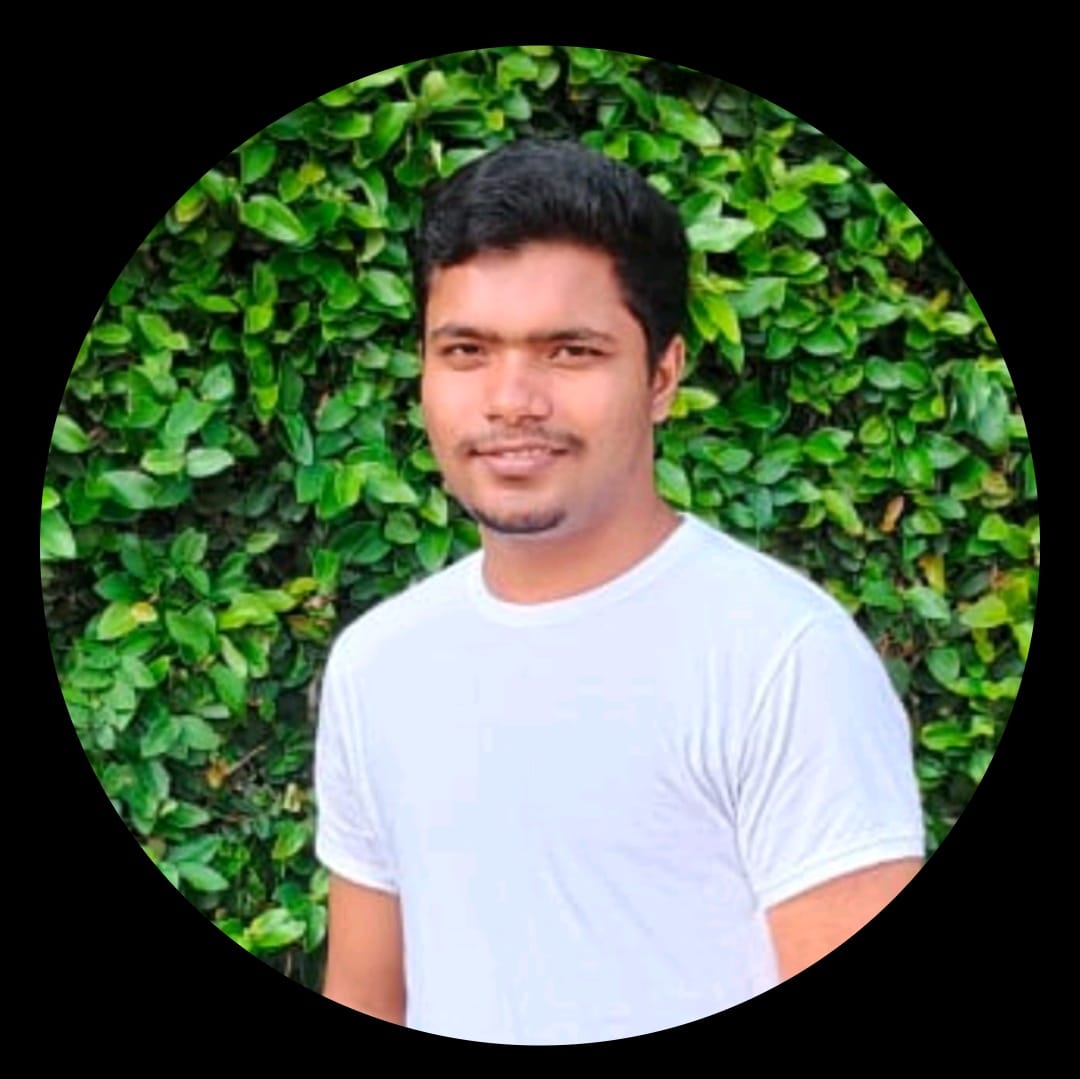
Tahmeer Pasha
Tahmeer Pasha
Hi there! My name is Tahmeer Pasha, I'm a full stack developer specializing in JavaScript and Java based technologies. I will be sharing my experiences in my blogs, do check them out!