Getting Started with C++: Essential Concepts (Part 2)
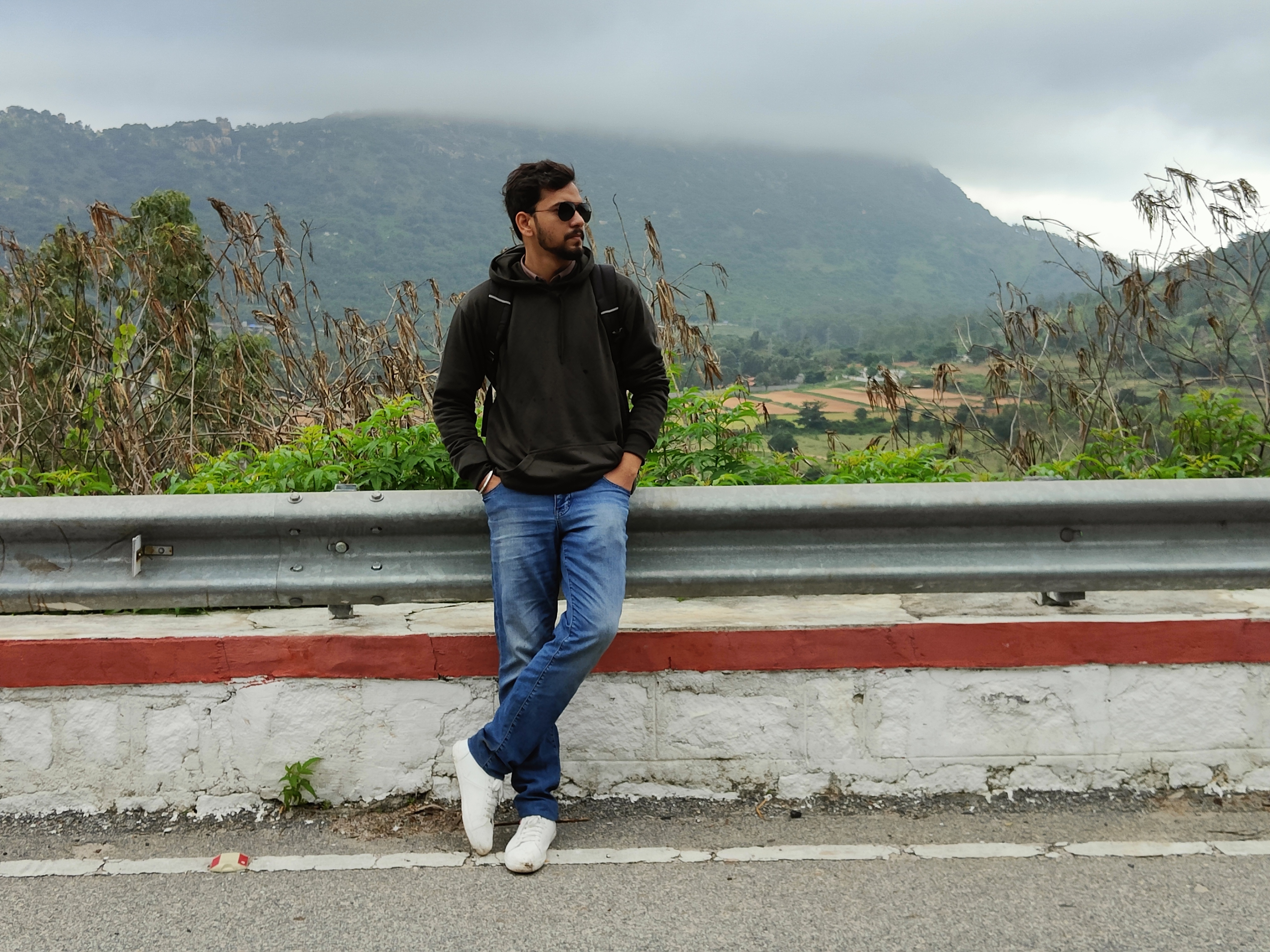
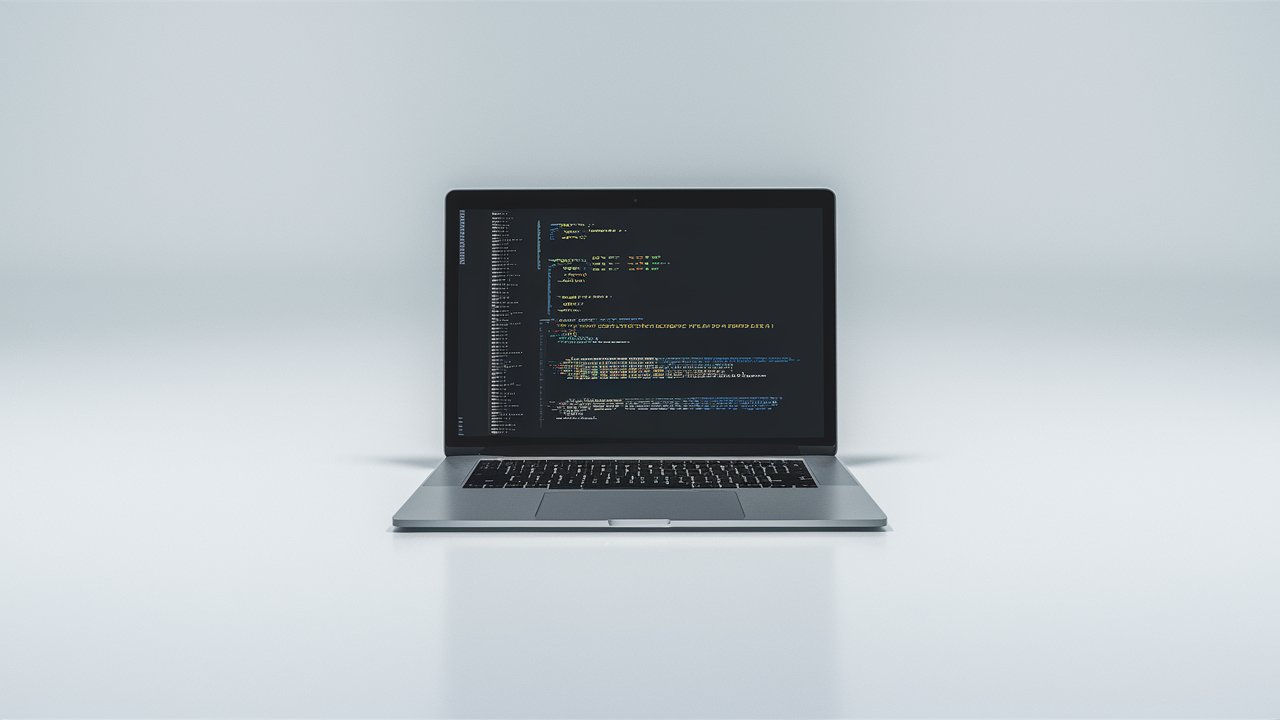
LOOPS
Why Loops?
A loop in programming is a fundamental concept used to repeat a block of code multiple times. There are different types of loops, each serving various purposes and offering different ways to control the repetition process. In C++ there are different types of loops each serving different purposes.
'for loop': The 'for loop' is typically used when the number of iterations is known beforehand. It initializes a counter, checks a condition before each iteration, and updates a counter at the end of each iteration.
In the above code, the counter starts at 0. It checks a condition and, if true, runs the code inside the curly brackets. Then, it increments the counter by 1 and checks the condition again. This process repeats until the condition is no longer met. Output for the above code is given below:
Output:
- 'while loop': The while loop continues to execute a block of code as long as the specified condition is true. It is useful when the number of iterations is not known and depends on a certain condition.
It continues to execute code blocks until a certain condition is met.
Output:
'do-while loop': The do-while is similar to the while loop, but guarantees that the block of code is executed at least once before checking the condition.
Output:
Why loops are necessary?
Automation: They automate repetitive tasks, reducing the risks of errors and saving time compared to manual repetition.
Efficiency: They can iterate over data structures like arrays, vectors, or links making it easy to process or manipulate large sets of data.
Break keyword:
The 'break' keyword in C++ is a powerful control statement used to terminate the execution of the nearest enclosing loop or switch statement. When the 'break' keyword is encountered within a loop, such as a 'for', 'while', or 'do-while' loop, or within a 'switch' statement, the control flow is immediately transferred to the statement following the terminated loop or switch. This means that the remaining iterations of the loop or the remaining cases of the switch statement are skipped, and the program continues executing from the next statement after the loop or switch.
Using the 'break' keyword can be particularly useful in various scenarios. For instance, it can be employed to exit a loop when a certain condition is met, thereby preventing unnecessary iterations and enhancing the efficiency of the code. In a 'switch' statement, 'break' is essential to ensure that only the matching case is executed and to avoid the fall-through behavior, where multiple cases are executed in sequence.
Output:
Continue keyword:
The 'continue' keyword in C++ is used within loops to skip the remaining statements in the current iteration and immediately proceed to the next iteration of the loop. It is particularly useful when you need to bypass specific parts of the loop code under certain conditions without terminating the entire loop.
Output:
'nested loops': Nested loops in C++ involve placing one loop inside another loop. This allows you to perform repeated operations. Over a multi-dimensional structure or handle more complex iteration logic. Each time the outer loop executes, the inner loop will run from start to finish.
Common use cases for nested loops:
Iterating through multi-dimensional Arrays or matrices.
- Generating permutations or combinations.
Output:
Operators in C++:
An operator is a symbol that operates on a value to perform specific mathematical or logical computations. They form the foundation of any programming language.
Unary Operators :
Unary operators in C++ are operators that operate on a single operand to produce a new value. They are used to perform various operations such as incrementing/decrementing a value, negating a value, or checking a value's properties.
Increment('++'):
Increases the value of the operand by 1. It has two forms: prefix & postfix
Prefix('++a'): Increments the value before using it in an expression.
Postfix('a++''): Increments the value after using it in an expression.
Output:
Decrement('--'):
Decreases the value of the operand by 1. It has two forms:
Prefix('--a'): Decrements the value before using it in an expression.
Postfix('a--'): Decrements the value after using it in an expression.
Output:
Binary Operators:
Arithmetic Operators: These operators perform basic arithmetic operations.
Note: int/int gives value in int, float/int gives value in float, double/int gives value in double.
Output:
Relational Operators: These operators compare two values and return a boolean result.
Output:
Logical Operators: These operators perform logical operations on boolean values.
Output:
Assignment Operators: These operators assign values to variables, possibly modifying the variable's current value.
Bitwise Operator:
Bitwise Operators in C++ are used to perform operations on the binary representations of integers. These operators work directly on the bits of the integer values.
Bitwise AND('&'):
Performs a logical AND operation on each pair of corresponding bits of two integers. The result is 1 if both bits are 1 otherwise 0.
Bitwise OR ('|'):
This operator compares each bit of its operands and sets the corresponding bit in the result to '1'. If either of the operand bits is '1'. Otherwise, the result bit is set to '0'.
Bitwise NOT('~'):
The bitwise NOT operation is performed using the '~' operator. This operator inverts each bit of its operand, turning '0' bit into '1' and '1' bits into '0'.
Left Shift ('<<'):
It shifts the bits of its left-hand operand to the left by the number of positions specified by its right-hand operand. Each left shift operation effectively multiplies the number by 2pow(number of shifts).
The initial value of a =5
Decimal: 5, Binary 0000 0101
Result of a << 1 (left shift by 1 position):
Shift left by 1: 0000 1010
Decimal: 10
Right Shift('>>'):
The right shift operator ('>>') shifts the bits of its left-hand operand to the right by the number of positions specified by its right-hand operand. For unsigned types, the rightmost bits are discarded and zero bits are shifted in from the left. The right shift operation effectively divides the number by 2pow(number of shifts).
Initial value of a = 20
Decimal: 20, Binary: 0001 0100
Result of a >> 1
Shift right by 1: 0000 1010
Decimal: 10
XOR('^'):
XOR operator is denoted by '^'. It performs a bitwise XOR operation and sets a bit to '1'. If the corresponding bits of the operands are different and to '0' if they are the same.
Let's write functions():
Functions in C++ are a fundamental part of the language, allowing you to encapsulate code into reusable blocks. Here is an overview of functions in C++, including how to declare, define, and use them.
Actual Parameter: The actual parameters are the values or variables passed to a function when it is called. These are also known as arguments.
Formal Parameter: The formal parameters are the variables defined by the function that receive the values of the actual parameters when the function is called.
Parameter Passing:
Pass by value: Copies the actual value of an argument into the formal parameter of the function.
Changes made to the parameter inside the function do not affect the argument.
Pass by reference:
Copies the address of an argument into the formal parameter. Changes made to the parameter affect the argument.
Number System in C++:
In C++ you often need to work with different number systems primarily binary & decimal number systems.
Decimal Number system:
The decimal number system is the standard system for denoting Integer and Non-Integer numbers. It's based on 10 different digits 0-9.
Binary Number system:
The binary number system is based on 2 digits 0 & 1.
Conversion between binary and decimal numbers is a fundamental concept in Computer Science and programming.
Binary to Decimal conversion:
Binary = 1101
Binary number and its positional values:
1 * 2 power(3) = 8
1 * 2 power(2) = 4
0 * 2 power(1) = 0
1 * 2 power(0) = 1
sum = 8+4+0+1 = 13
Decimal to Binary conversion:
To convert a decimal number to a binary number, repeatedly divide the number by 2 and record the remainder.
Decimal number = 13
Divide 13 by 2: quotient = 6, remainder = 1
Divide 6 by 2: quotient = 3, remainder = 0
Divide 3 by 2: quotient = 1, remainder =1
Divide 1 by 2: quotient = 0, remainder = 1
TypeCasting in C++:
Typecasting in C++ is a way to convert a variable from 1 datatype to another. There are different methods to perform typecasting, each suited to different needs and ensuring type safety.
Implicit Typecasting:
As known as Automatic type conversion. The compiler automatically converts one data type to another during an operation. This happens when you perform operations involving variables of different datatypes and the compiler promotes one type to a larger type to maintain precision.
For more info on ASCII Table check this link:
Explicit type conversion:
As known as manual type conversion. Allows you to explicitly specify the desired datatype during an assignment or operation. You use the Casting operator which is represented by parentheses containing the target data type.
Throughout this series, we've covered all the essential topics to give you a comprehensive understanding of C++. With this knowledge, you are well-equipped to tackle real-world programming challenges and continue your journey toward becoming a proficient C++ developer. Thank you for joining us, and happy coding!
Subscribe to my newsletter
Read articles from Rishabh Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
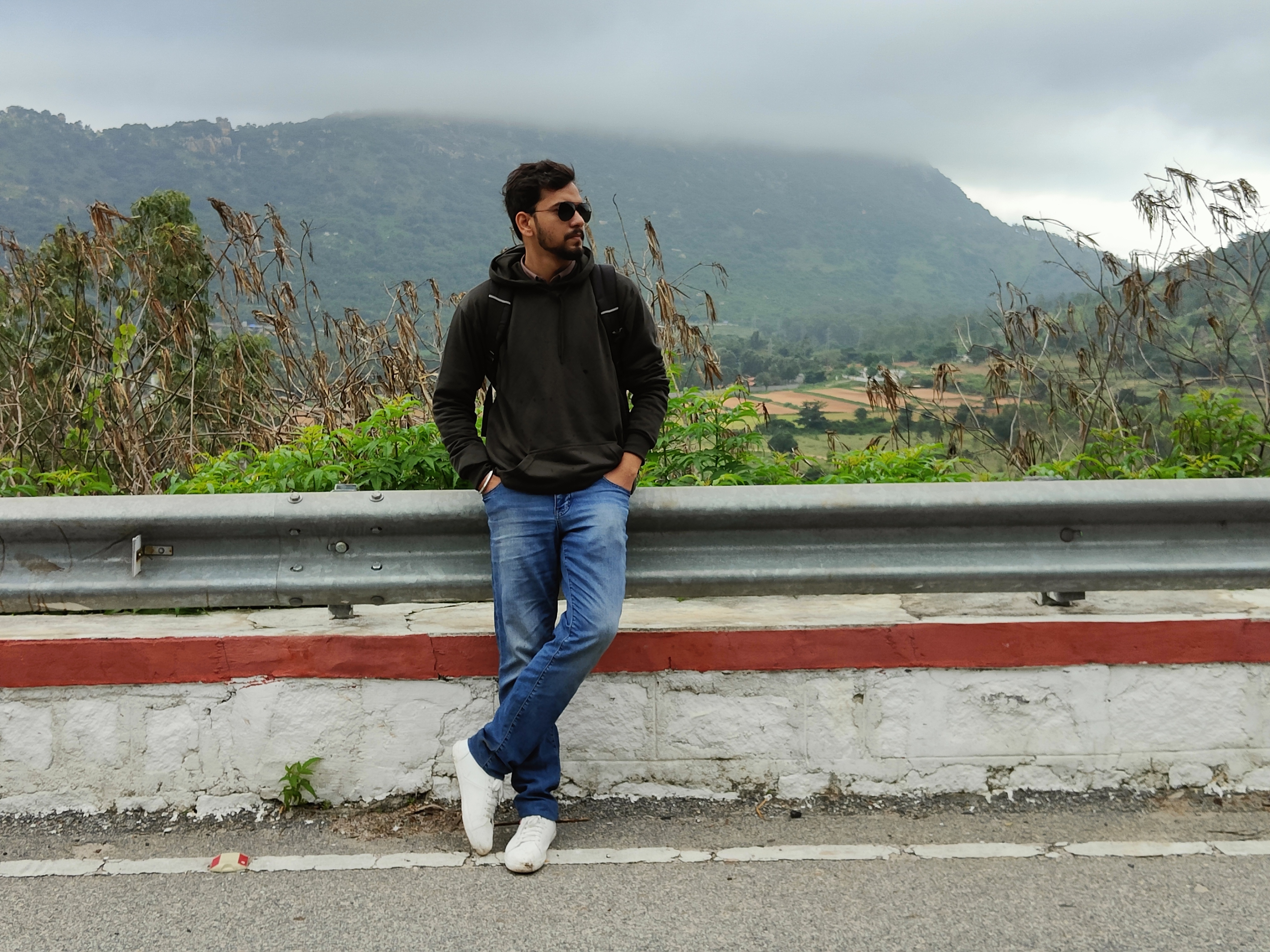