Mastering the useEffect Hook in React: Understanding Component Lifecycle
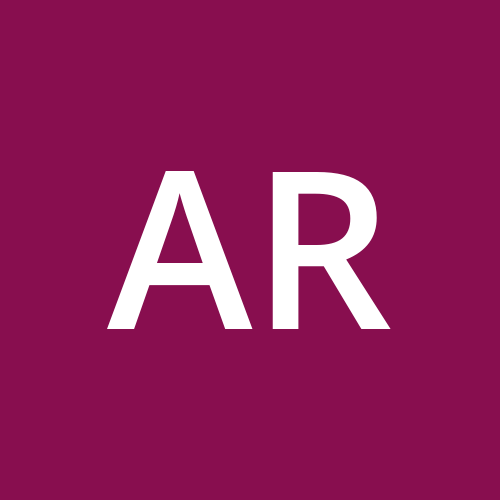
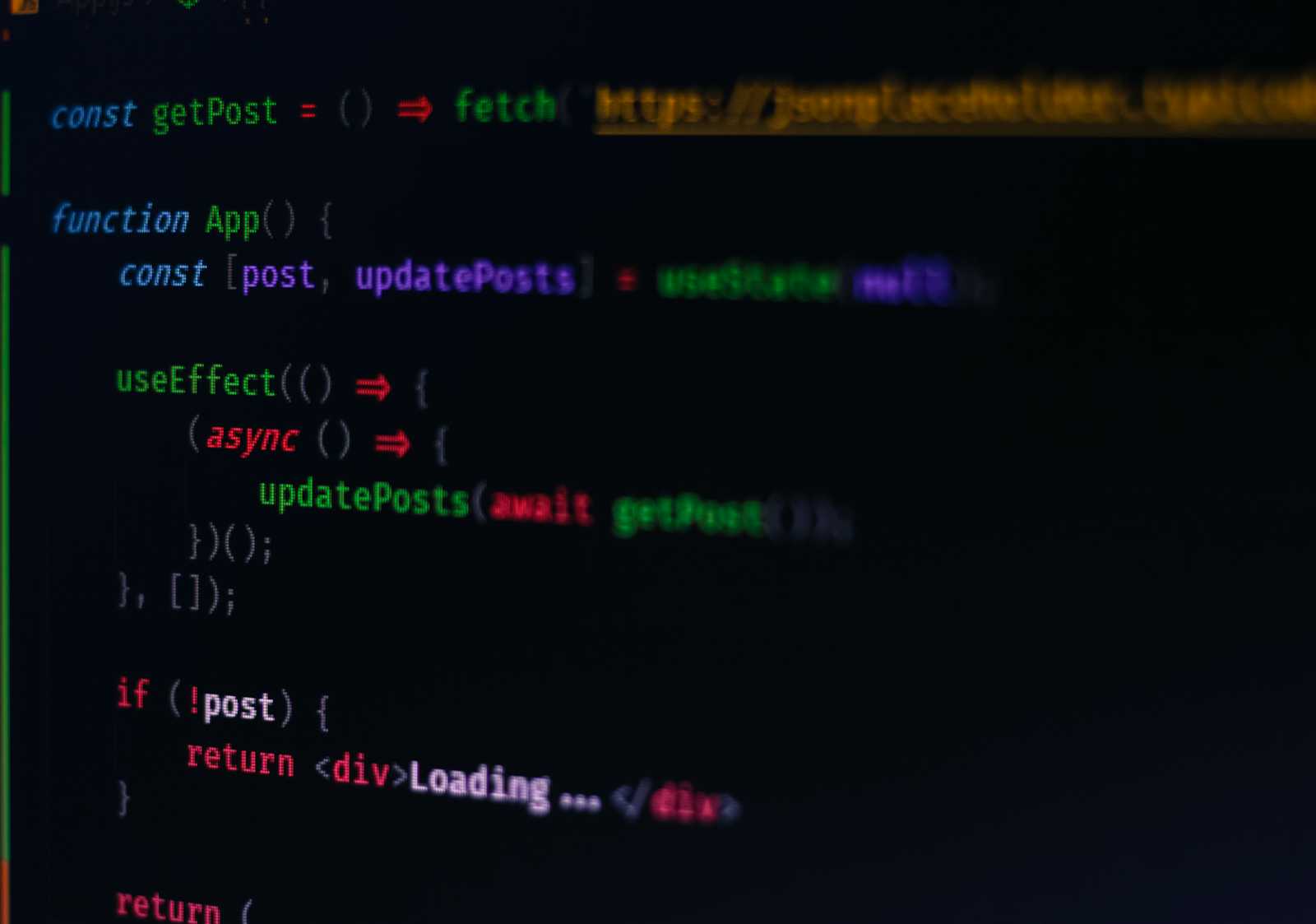
The useEffect
hook is one of the most powerful tools in React's functional components, providing a way to handle side effects and manage component lifecycles. This article will guide you through using the useEffect
hook effectively, helping you understand how it integrates with the component lifecycle in React.
Introduction to useEffect Hook
The useEffect
hook allows you to perform side effects in function components, such as data fetching, subscriptions, and manually changing the DOM. It helps manage the component's lifecycle, taking over the roles that lifecycle methods had in class components.
Basic Usage
The basic syntax of useEffect
is as follows:
import React, { useEffect } from 'react';
const MyComponent = () => {
useEffect(() => {
// Your side effect code here
return () => {
// Cleanup code here
};
}, [dependencies]); // Dependency array
return <div>My Component</div>;
};
Effect Function: The first argument is a function where you put your side effect code. This function runs after every render by default.
Cleanup Function: The effect function can optionally return a function. This cleanup function runs before the component is removed from the DOM (unmounts) or before the effect runs again on subsequent renders.
Dependency Array: The second argument is an array of dependencies. The effect only runs if one of these dependencies changes. If you provide an empty array, the effect runs only once after the initial render.
Understanding Component Lifecycle
React's component lifecycle consists of different stages that a component goes through from its creation to its removal. Knowing these stages is essential for managing state and ensuring your app runs smoothly. In class components, you would handle these tasks in lifecycle methods like componentDidMount
, componentDidUpdate
, and componentWillUnmount
. The useEffect
hook combines all these capabilities into a single API.
In React, a component's lifecycle consists of three main phases: Mounting, Updating, and Unmounting.
Mounting: This is when a component is being created and inserted into the DOM. During this phase, you can set up any initial state or side effects that your component needs to function.
Updating: This phase occurs whenever a component's state or props change, causing a re-render. It's crucial to manage these updates efficiently to keep your application responsive and performant.
Unmounting: This is when a component is being removed from the DOM. During this phase, you should clean up any side effects or subscriptions that were set up during the component's lifecycle to prevent memory leaks.
Here's an example that fetches data from an API when the component mounts:
import React, { useState, useEffect } from 'react';
const MyComponent = () => {
const [data, setData] = useState(null);
useEffect(() => {
const fetchData = async () => {
const response = await fetch('https://api.example.com/data');
const result = await response.json();
setData(result);
};
fetchData();
}, []); // Empty array ensures this runs only once on mount
return (
<div>
{data ? <p>Data: {data}</p> : <p>Loading...</p>}
</div>
);
};
Mounting Phase
In the mounting phase, the component is initially added to the DOM. This is the perfect time to set up any initial state or side effects that your component needs to function, similar to what you would do in componentDidMount
in class components. It’s ideal for tasks like fetching data, setting up subscriptions, or initiating timers. By passing an empty array as the second argument, you ensure that this effect runs only once, when the component mounts.
import React, { useEffect } from 'react';
const MyComponent = () => {
useEffect(() => {
console.log('Component mounted');
// Perform setup actions like fetching data or setting up subscriptions
}, []); // Empty dependency array ensures this runs only once on mount
return <div>My Component</div>;
};
Performance Considerations During the Mounting Phase
When a component mounts, it's crucial to ensure that any operations performed during this phase are efficient and do not degrade the performance of your application. Here are key considerations to keep in mind:
Avoid Blocking the Main Thread
The main thread is responsible for rendering the UI and handling user interactions. If you perform heavy operations, such as complex calculations or synchronous API calls, during the mounting phase, it can block the main thread. This results in a sluggish user experience, with delayed interactions and rendering.
useEffect(() => {
// Simulating a heavy synchronous operation
const heavyOperation = () => {
for (let i = 0; i < 1e9; i++) {
// Intensive loop
}
console.log('Heavy operation completed');
};
heavyOperation(); // This blocks the main thread
}, []);
To avoid blocking the main thread, perform heavy operations asynchronously. This can be achieved using setTimeout
, requestAnimationFrame
, or asynchronous functions.
Minimize DOM Manipulations
Direct DOM manipulations can be expensive and should be minimized. Use React’s declarative approach to update the DOM efficiently. In a declarative approach, you describe what the UI should look like for a given state, and React handles the underlying DOM manipulations.
const MyComponent = () => {
const [color, setColor] = useState('blue');
const [text, setText] = useState('Hello, World!');
const divRef = useRef(null);
// Correct Declarative Approach
const changeTextAndColorDeclarative = () => {
setColor('green');
setText('Updated Text');
};
// Incorrect Imperative Approach
const changeTextAndColorImperative = () => {
if (divRef.current) {
divRef.current.style.backgroundColor = 'green';
divRef.current.innerText = 'Updated Text';
}
};
useEffect(() => {
if (divRef.current) {
// Initial setup for the incorrect approach
divRef.current.style.backgroundColor = 'blue';
divRef.current.innerText = 'Hello, World!';
}
}, []);
};
Lazy Initialization
For state that requires heavy computations, use lazy initialization to defer the computation until it is actually needed. When you have a piece of state that requires a lot of time or resources to compute, you don't want this computation to happen during the initial render. This can slow down the rendering of your component, making your app feel sluggish.
import React, { useState, useEffect } from 'react';
const MyComponent = () => {
// Define the heavy computation function
const computeExpensiveValue = () => {
console.log('Performing heavy computation...');
let someValue = 0;
for (let i = 0; i < 1e7; i++) {
someValue += i;
}
return someValue;
};
// Use lazy initialization with useState
const [expensiveState] = useState(() => {
return computeExpensiveValue();
});
useEffect(() => {
// Setup side effects if any
}, []);
return <div>Expensive State: {expensiveState}</div>;
};
export default MyComponent;
By using lazy initialization, you ensure that the heavy computation only happens when it’s necessary, not right away when the component is first created.
Updating Phase
During the updating phase, the useEffect
hook handles side effects based on changes in state or props. This allows you to perform actions whenever specific values change, similar to the componentDidUpdate
lifecycle method in class components.
import React, { useState, useEffect } from 'react';
const MyComponent = () => {
const [count, setCount] = useState(0);
useEffect(() => {
console.log('Count changed:', count);
// Perform actions that depend on `count`
}, [count]); // Dependency array with `count` ensures this runs only when `count` changes
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
export default MyComponent;cy array with `count` ensures this runs when `count` changes
The second argument to useEffect
is a dependency array. By including count
in this array, you tell React to run the effect only when count
changes. This optimizes performance by preventing unnecessary re-renders.
There are several reasons why updates may happen in a React component:
Parent Component Re-renders: If the parent component re-renders, it can cause child components to re-render as well.
State Changes: When the state within the component changes, React re-renders the component to reflect the new state.
Context Changes: If the component consumes context and the context value changes, it triggers a re-render.
Unmounting Phase
In the unmounting phase, useEffect
handles cleanup actions when a component is about to be removed from the DOM. This is equivalent to the componentWillUnmount
lifecycle method in class components. The cleanup function inside useEffect
runs before the component unmounts, ensuring that any necessary cleanup actions, such as removing event listeners or canceling network requests, are performed.
import React, { useEffect } from 'react';
const MyComponent = () => {
useEffect(() => {
console.log('Component mounted');
return () => {
console.log('Component will unmount');
// Cleanup actions like removing event listeners
};
}, []); // Empty dependency array ensures this runs only once on mount and cleanup on unmount
return <div>My Component</div>;
};
export default MyComponent;
Usage Scenarios for Cleanup
Removing Event Listeners: If you add event listeners when the component mounts, you should remove them when the component unmounts to prevent memory leaks.
Canceling Network Requests: If you initiate network requests when the component mounts, you should cancel any ongoing requests when the component unmounts to avoid potential issues.
Cleaning Up Subscriptions: If you set up subscriptions (e.g., WebSocket connections) when the component mounts, you should close them when the component unmounts.
Clear Timers or Intervals: Any
setTimeout
orsetInterval
calls should be cleared.
Proper cleanup ensures that resources are freed up and the application remains efficient without unintended side effects.
Handling Multiple Effects
You can use multiple useEffect
hooks to handle different side effects separately. This improves code organization and readability, allowing you to manage side effects related to different states independently. By separating concerns, you can make your codebase easier to maintain and debug.
import React, { useState, useEffect } from 'react';
const MyComponent = () => {
const [count, setCount] = useState(0);
const [name, setName] = useState('John');
// Effect for count
useEffect(() => {
console.log('Effect for count:', count);
// Perform actions that depend on `count`
return () => {
console.log('Cleanup for count effect');
// Cleanup actions related to `count`
};
}, [count]);
// Effect for name
useEffect(() => {
console.log('Effect for name:', name);
// Perform actions that depend on `name`
return () => {
console.log('Cleanup for name effect');
// Cleanup actions related to `name`
};
}, [name]);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment Count</button>
<p>Name: {name}</p>
<button onClick={() => setName('Doe')}>Change Name</button>
</div>
);
};
export default MyComponent;
Managing side effects independently can lead to better performance. For example, effects will only re-run when their specific dependencies change, reducing unnecessary computations and re-renders.
Best Practices
Dependencies: Always specify dependencies to avoid infinite loops and unnecessary re-renders.
Cleanup: Use cleanup functions to prevent memory leaks and ensure proper resource management.
Separation of Concerns: Use multiple useEffect
hooks for different side effects to keep your code organized.
Conclusion
The useEffect
hook is a versatile tool that simplifies handling side effects and managing the component lifecycle in React functional components. By understanding its usage patterns and best practices, you can create efficient, clean, and maintainable React applications.
Mastering useEffect
will significantly enhance your React development skills, enabling you to manage component lifecycles effectively. If you have any questions or topics you'd like to explore further, feel free to reach out!
If you liked this post, please give it a like and share it with others who might find it helpful!
Subscribe to my newsletter
Read articles from Artur directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
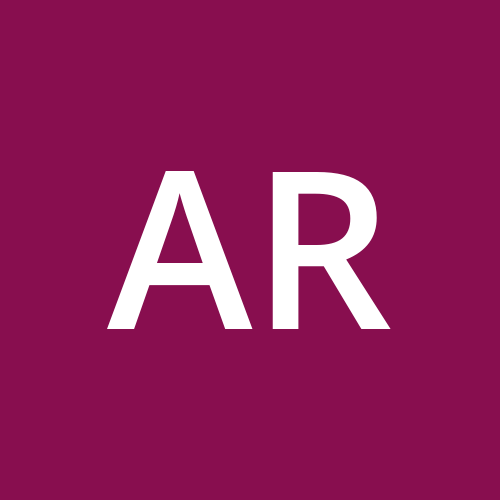