My First React Mini Project: Weather & Notepad App!

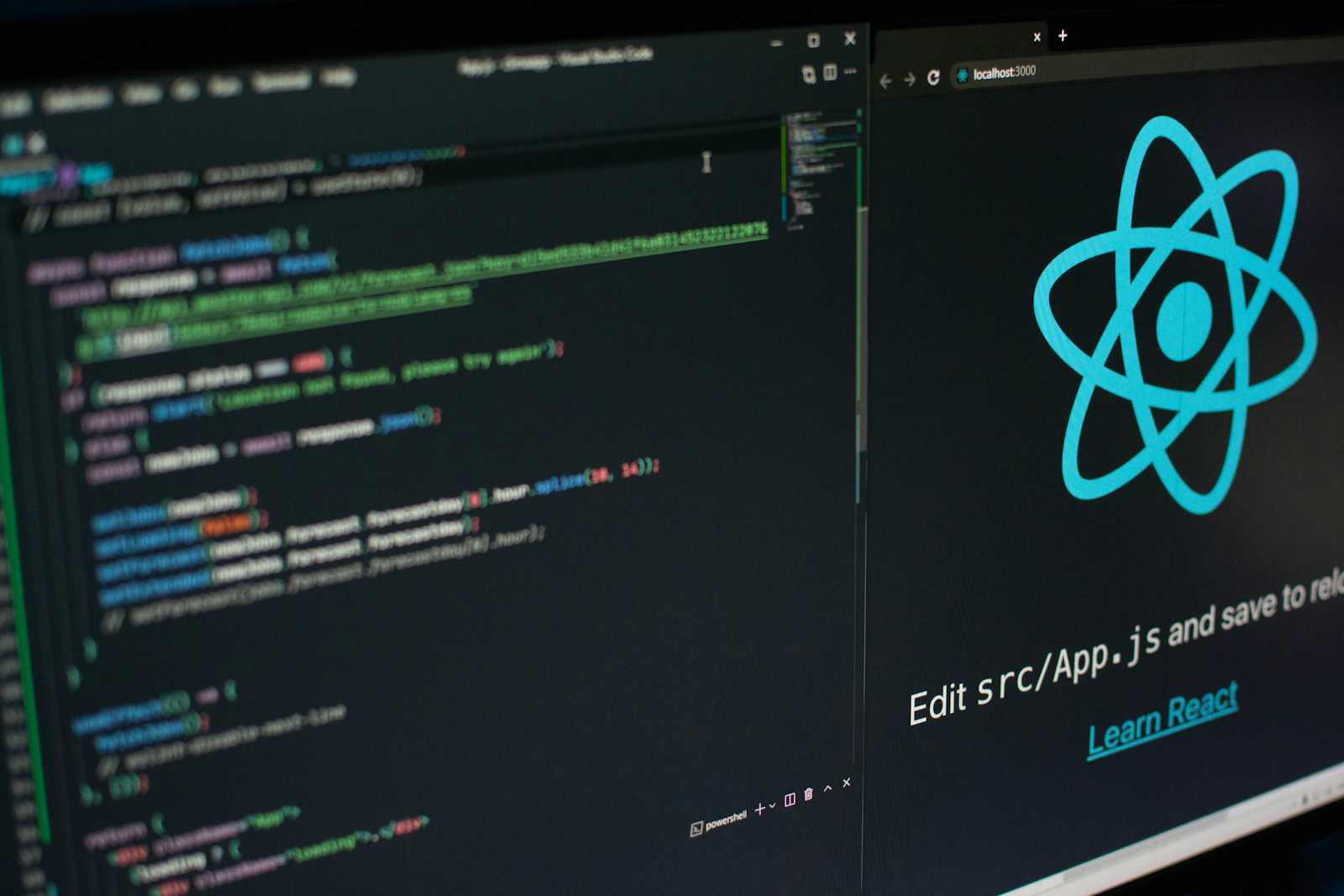
I'm thrilled to share my first mini project using React! This application combines real-time weather data with a simple notepad feature. It was a fantastic learning experience, and I'm excited to walk you through it.
🔧 Features
Weather Data Integration
The app fetches weather data using Axios and integrates with the Weatherbit API. It displays:
Average temperature for the week
Average rainfall for the week
Average humidity for the week
Current temperature
A bar chart showing the temperature for each day of the previous week
Responsive UI
Using Material-UI, I built a clean and responsive interface that adapts well to different screen sizes.
Interactive Charts
I used Recharts to create a dynamic bar chart that visualizes the weekly temperature data.
Notepad Functionality
The app includes a notepad where users can:
Add new notes
Delete existing notes
Project Links
Check out the project on GitHub and see it live on Vercel:
GitHub: Weather & Notepad App
Vercel: Live demo
Technologies Used
React: For building the user interface
Axios: For making HTTP requests
Weatherbit API: For fetching weather data
Material-UI: For UI components
Recharts: For data visualization
Code Overview
Fetching Weather Data
Using Axios, I fetch the weather data from the Weatherbit API:
useEffect(() => {
const fetchWeatherData = async () => {
const API_KEY = 'your_api_key';
const CITY = 'london';
const URL = `https://api.weatherbit.io/v2.0/forecast/daily?city=${CITY}&key=${API_KEY}&days=7&units=M`;
try {
const response = await axios.get(URL);
const data = response.data;
setWeatherData({
avgTemp: data.data.reduce((acc, day) => acc + day.temp, 0) / data.data.length,
avgRainfall: data.data.reduce((acc, day) => acc + (day.precip || 0), 0) / data.data.length,
avgHumidity: data.data.reduce((acc, day) => acc + day.rh, 0) / data.data.length,
currentTemp: data.data[0].temp,
weeklyTemp: data.data.map((day, index) => ({
day: `Day ${index + 1}`,
temp: day.temp
}))
});
} catch (error) {
console.error('Error fetching weather data', error);
}
};
fetchWeatherData();
}, []);
UI Components
I used Material-UI for building a responsive and visually appealing interface:
<Grid container spacing={2}>
<Grid item xs={3}>
<Card className="card animated fadeInLeft">
<CardContent>
<Typography variant="h6">Avg Temp of Week</Typography>
<Typography variant="h4">{weatherData.avgTemp.toFixed(2)}°C</Typography>
</CardContent>
</Card>
</Grid>
{/* More cards here for Rainfall, Humidity, and Current Temp */}
</Grid>
Notepad Functionality
Users can add and delete notes:
const handleDeleteNote = (index) => {
setNotes(notes.filter((_, i) => i !== index));
};
const handleAddNote = () => {
if (newNote.trim()) {
if (newNote.length > 200) {
alert("Note cannot exceed 200 characters.");
} else {
setNotes([...notes, newNote.trim()]);
setNewNote('');
}
} else {
alert("Note cannot be empty.");
}
};
Conclusion
Building this Weather & Notepad app has been a great learning experience. I've enhanced my skills in React, API integration, and data visualization. I look forward to building more projects and sharing my journey with you all!
Feel free to check out the code on GitHub and try the live demo on Vercel. Let me know your thoughts and any feedback you might have!
Happy coding! 🚀
#React #WebDevelopment #JavaScript #FirstProject #APIIntegration #MaterialUI #Recharts #Hashnode
Subscribe to my newsletter
Read articles from Bhavesh Jadhav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Bhavesh Jadhav
Bhavesh Jadhav
I am a passionate web developer from India, currently pursuing an MCA from Government College of Engineering, Aurangabad. With a strong foundation in HTML, CSS, JavaScript, and expertise in frameworks like React and Node.js, I create dynamic and responsive web applications. My skill set extends to backend development with PHP, MySQL, and MongoDB. I also have experience with AJAX, jQuery, and I am proficient in Python and Java. As an AI enthusiast, I enjoy leveraging AI tools and LLMs to enhance my projects. Eager to expand my horizons, I am delving into DevOps to optimize development workflows and improve deployment processes. Always keen to learn and adapt, I strive to integrate cutting-edge technologies into my work. Let's connect and explore the world of technology together!