Understanding React Composition: The Superior Alternative to Prop Drilling and Context API

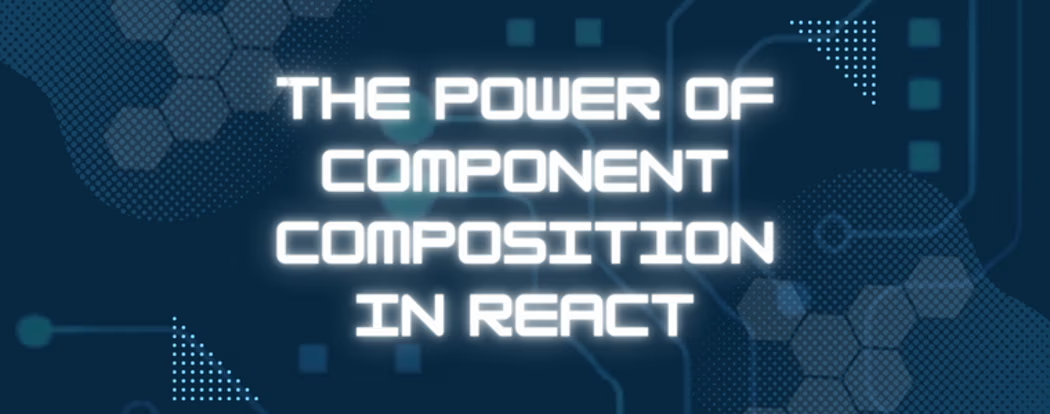
React composition is a powerful design pattern that allows you to build complex UIs by combining smaller, reusable components. Instead of relying on prop drilling, the Context API, or state management libraries like Redux, React encourages composition to promote code reusability and maintainability. In this blog post, we'll explore the basics of React composition, and its benefits over other state management techniques, and demonstrate its use with example code.
What is React Composition?
Composition in React refers to the practice of building components by combining multiple smaller components. It allows you to create flexible and reusable components by passing data and functions down through props, enabling you to assemble complex UIs from simple building blocks.
Why Choose Composition Over Prop Drilling, Context API, or Redux?
Prop Drilling
Prop drilling involves passing data through multiple layers of components, even if intermediate components don't need the data. This can lead to cluttered code and make it hard to manage and maintain.
Disadvantages of Prop Drilling:
Hard to maintain as the app grows
Increased coupling between components
Prop chains can become long and unwieldy
Context API
Context API provides a way to pass data through the component tree without having to pass props down manually at every level. While useful, it has its own downsides.
Disadvantages of Context API:
Overuse can lead to performance issues
Makes component reuse more difficult
Can make the code harder to understand and debug
Redux
Redux is a state management library that helps manage the state of the application in a single place. It's powerful but can be overkill for simple state management.
Disadvantages of Redux:
Requires boilerplate code
The steep learning curve for beginners
Adds complexity to the project
Benefits of Composition
Reusability: Small, focused components can be reused across different parts of your application.
Maintainability: Smaller components are easier to understand, test, and maintain.
Separation of Concerns: Each component handles a specific part of the UI, making your codebase more modular.
Flexibility: Components can be composed in various ways to create different UIs without changing the underlying components.
Simplicity: Composition simplifies state management by limiting the state to where it is needed.
Example: Building a Modal Component with Composition
Let's create a reusable Modal
component using composition to illustrate its benefits.
Step 1: Create the Modal Component
First, we'll create a Modal
component that serves as a container for its children.
// Modal.js
import React from 'react';
import './Modal.css'; // Assuming you have some basic styling
const Modal = ({ children, isOpen, onClose }) => {
if (!isOpen) return null;
return (
<div className="modal-overlay" onClick={onClose}>
<div className="modal-content" onClick={e => e.stopPropagation()}>
{children}
</div>
</div>
);
};
export default Modal;
Step 2: Create Child Components
Next, we'll create some child components to be used inside the Modal
.
// ModalHeader.js
import React from 'react';
const ModalHeader = ({ title }) => {
return <div className="modal-header"><h2>{title}</h2></div>;
};
export default ModalHeader;
// ModalBody.js
import React from 'react';
const ModalBody = ({ children }) => {
return <div className="modal-body">{children}</div>;
};
export default ModalBody;
// ModalFooter.js
import React from 'react';
const ModalFooter = ({ children }) => {
return <div className="modal-footer">{children}</div>;
};
export default ModalFooter;
Step 3: Compose the Components
Now, we'll compose the Modal
with the child components to create a complete UI.
// App.js
import React, { useState } from 'react';
import Modal from './Modal';
import ModalHeader from './ModalHeader';
import ModalBody from './ModalBody';
import ModalFooter from './ModalFooter';
const App = () => {
const [isModalOpen, setModalOpen] = useState(false);
const openModal = () => setModalOpen(true);
const closeModal = () => setModalOpen(false);
return (
<div>
<button onClick={openModal}>Open Modal</button>
<Modal isOpen={isModalOpen} onClose={closeModal}>
<ModalHeader title="Modal Title" />
<ModalBody>
<p>This is the body of the modal.</p>
</ModalBody>
<ModalFooter>
<button onClick={closeModal}>Close</button>
</ModalFooter>
</Modal>
</div>
);
};
export default App;
Step 4: Add Some Styling
Finally, let's add some basic CSS to style our modal.
/* Modal.css */
.modal-overlay {
position: fixed;
top: 0;
left: 0;
right: 0;
bottom: 0;
background: rgba(0, 0, 0, 0.5);
display: flex;
justify-content: center;
align-items: center;
}
.modal-content {
background: white;
padding: 20px;
border-radius: 8px;
max-width: 500px;
width: 100%;
}
.modal-header,
.modal-body,
.modal-footer {
margin-bottom: 10px;
}
Final Output
When you run your application, you should see a button that opens a modal with a header, body, and footer, all composed from separate components.
Conclusion
React composition offers a clean and efficient way to build complex UIs by combining simple, reusable components. It avoids the pitfalls of prop drilling, the Context API, and heavy state management libraries like Redux, making your code more maintainable, flexible, and easier to understand. By leveraging composition, you can create powerful and reusable components that enhance the overall quality of your React applications.
Happy coding!
Subscribe to my newsletter
Read articles from akash javali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

akash javali
akash javali
A passionate 'Web Developer' with a Master's degree in Electronics and Communication Engineering who chose passion as a career. I like to keep it simple. My goals are to focus on typography, and content and convey the message that you want to send. Well-organized person, problem solver, & currently a 'Senior Software Engineer' at an IT firm for the past few years. I enjoy traveling, watching TV series & movies, hitting the gym, or online gaming.