From Frustration to Proficiency: Solving GitHub’s Toughest Challenges (Part 1)
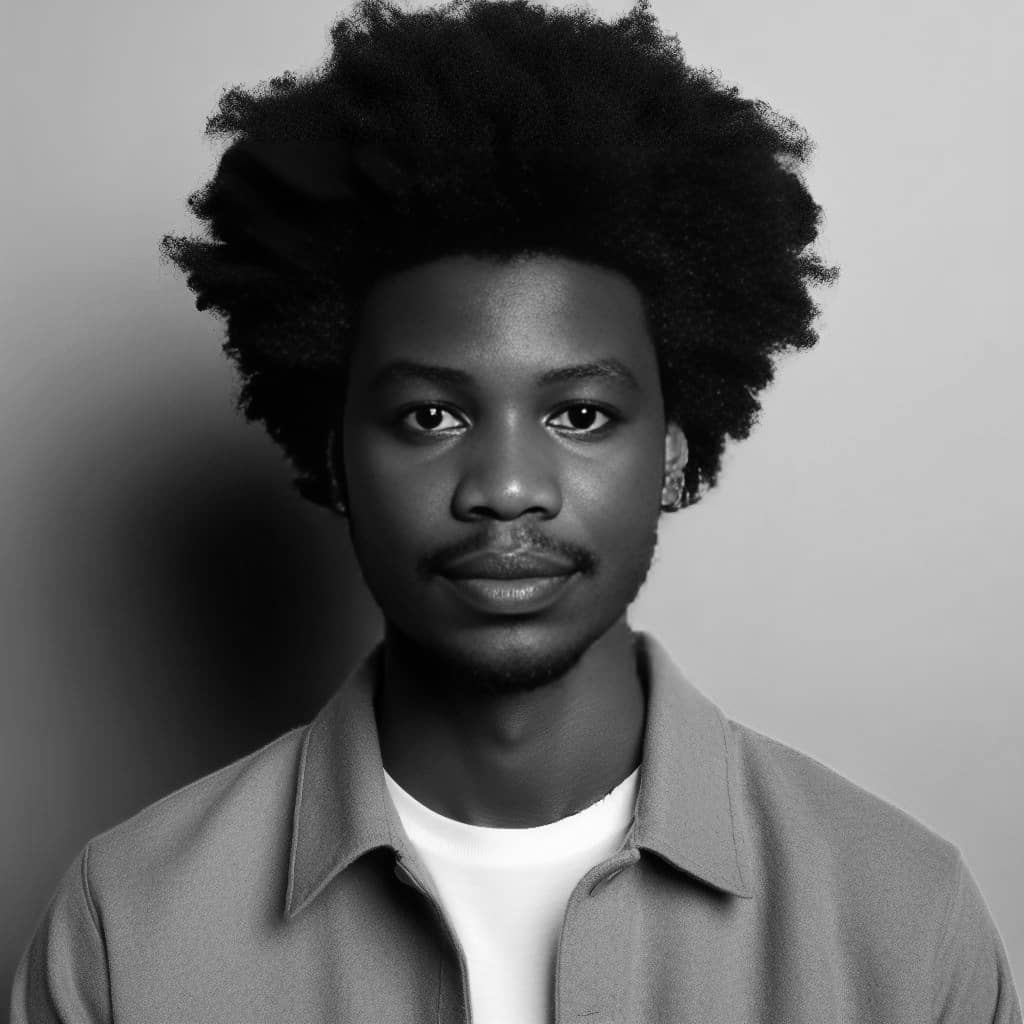
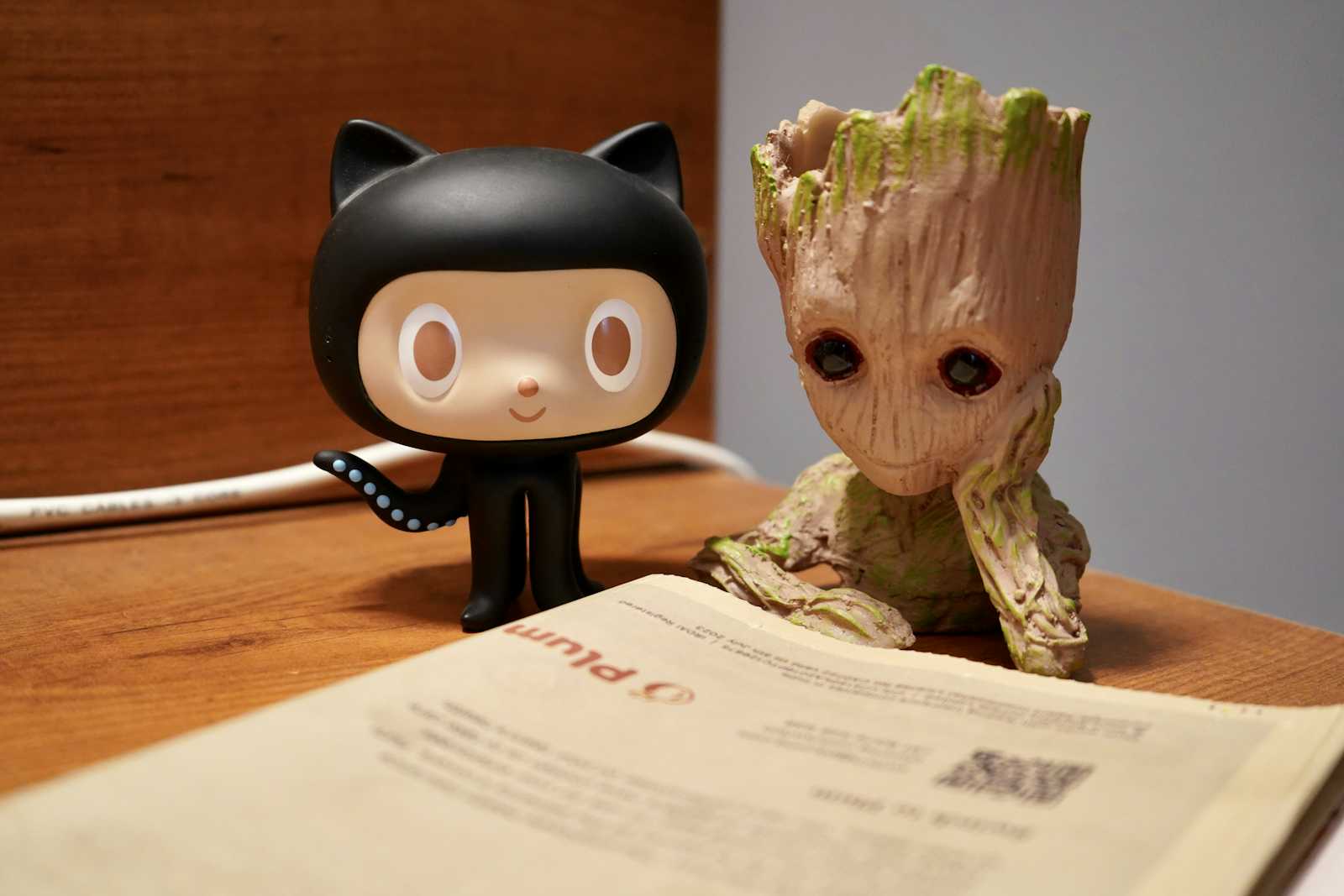
Welcome to the inaugural installment of "From Frustration to Proficiency: Solving GitHub’s Toughest Challenges" on the DevOps/Cloud with Qurtana blog! Whether you're a seasoned developer or just beginning your coding journey, GitHub can often seem like an intricate puzzle waiting to be solved. But fret not, dear reader, for this series is your compass in the GitHub wilderness.
Here, we go beyond mere solutions to common GitHub hurdles; we unveil the secrets to conquering every coder's nightmares. From navigating the labyrinth of version control to mastering collaborative workflows, we're here to guide you every step of the way.
So, grab your favorite beverage, settle into your coding throne, and prepare to embark on a journey of enlightenment. Together, we'll turn frustration into proficiency, relishing every challenge GitHub throws our way.
As we delve into this adventure, remember that every stumble is an opportunity for growth. Whether you're grappling with authentication quandaries or wrestling with the intricacies of version control, know that you're not alone. Through shared experiences and practical solutions, we'll navigate these challenges together, emerging stronger and more adept in the world of GitHub.
Common Challenges Developers May Encounter on Their Journey Through GitHub
Challenge 1: Navigating Authentication and Secure Connections
Authentication serves as the gateway to GitHub's expansive universe of code and collaboration. Without it, accessing this realm becomes an arduous task, leaving you on the sidelines of the coding community.
GitHub presents three primary authentication methods: SSH, GitHub CLI, and HTTPS, each with it's own unique benefits. Among them, SSH authentication shines as the gold standard for secure connections.
Using SSH Authentication
SSH authentication offers a secure pathway to GitHub repositories, yet its setup can be daunting for novices. Follow these steps to navigate SSH authentication successfully:
Generating SSH Keys: Begin by generating a new SSH key via your terminal:
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
Follow the prompts to specify the file location and passphrase for your SSH key.
Adding SSH Key to the ssh-agent: To ensure seamless authentication, add your SSH key to the ssh-agent:
eval "$(ssh-agent -s)"
Then, include your SSH private key:
ssh-add ~/.ssh/id_rsa
Adding SSH Key to GitHub: With your SSH key ready, navigate to your GitHub account settings and paste the public key into the SSH and GPG keys section.
Cloning Repositories with SSH: Finally, clone repositories effortlessly using the following command:
git clone git@github.com:user/repository.git
Replace
user/repository
with the appropriate username and repository name.
Using GitHub CLI
GitHub CLI streamlines the authentication process, enabling you to execute GitHub actions directly from the command line. Here's how to authenticate and clone repositories using the GitHub CLI:
Install GitHub CLI: Follow GitHub's installation instructions for your operating system.
Authenticate with GitHub: Open your terminal and execute the command:
gh auth login
Follow the prompts to authenticate with your GitHub account.
Clone a Repository: Once authenticated, clone repositories effortlessly:
gh repo clone owner/repository
Replace
owner/repository
with the desired username and repository name.
For HTTPS authentication, similar steps apply. Remember to safeguard your SSH private key with a passphrase and periodically rotate SSH keys for enhanced security.
Conclusion
Authentication marks the initial stride in your GitHub expedition, with SSH authentication, GitHub CLI, and HTTPS serving as trusty companions for secure connections. By adhering to these steps and best practices, you'll navigate GitHub's terrain with confidence, embarking on a coding odyssey like no other.
For further insights, consult the GitHub documentation on SSH authentication and GitHub CLI.
Challenge 2: Managing Branches
As a newcomer to the realm of software development, mastering the basics of GitHub may seem like a conquerable feat. However, the landscape drastically changes when you're tasked with navigating the intricacies of multiple branches. Suddenly, what once felt like a manageable journey becomes a labyrinth of confusion and stress.
At its core, GitHub's branch system allows developers to work on separate strands of code without affecting the main project. Each branch represents a unique avenue for development, enabling collaboration among team members without the risk of disrupting the master codebase.
For the uninitiated, branching can quickly spiral into chaos without proper oversight. It's all too easy to lose track of changes, merge conflicts, or inadvertently overwrite crucial code. As a result, what should be a streamlined development process transforms into a tangled web of frustration.
Fear not, intrepid coder! With the right approach, managing branches can become second nature. Let's dive into some essential commands and their contexts:
Creating a New Branch:
Command:
git checkout -b new-branch-name
Context: Imagine you're tasked with adding a new feature to your project. Instead of making changes directly to the main codebase, you create a new branch named "feature-xyz" using the command above. This isolates your work from the main project until it's ready to be merged.
Switching Between Branches:
Command:
git checkout branch-name
Context: While working on your "feature-xyz" branch, you receive a critical bug report in the production code. You switch to the main branch (
git checkout main
) to address the issue without affecting your feature development.
Merging Branches:
Command:
git merge source-branch
Context: After thoroughly testing your new feature on the "feature-xyz" branch, you're ready to integrate it into the main codebase. You switch to the main branch (
git checkout main
) and merge your feature branch using the command above.
Deleting a Branch:
Command:
git branch -d branch-name
Context: Once your feature has been successfully merged into the main codebase, you no longer need the "feature-xyz" branch. You delete it to keep the repository clean and tidy, using the command above.
Remember to communicate effectively with your team to ensure alignment on branch objectives and timelines. Regularly review and merge branches to keep the codebase up-to-date and minimize merge conflicts. Document branch changes and rationale to provide context for future reference and troubleshooting.
While managing branches may initially seem daunting, with patience and practice, you'll soon navigate the branching landscape with confidence. By implementing sound branching strategies and adhering to best practices, you'll unlock the full potential of GitHub's collaborative ecosystem, paving the way for seamless development and innovation.
Challenge 3: Handling Merge Conflicts
Merge conflicts are a common hurdle in collaborative development, occurring when Git is unable to automatically merge changes from different branches. These conflicts arise when two branches have diverged and Git cannot determine which changes to incorporate into the final merge.
Imagine you're working on a project with a team, and you’re all working on different features in separate branches. You're excited to merge your changes into the main branch, but then Git throws a curveball—merge conflict detected. This usually happens when changes are made to the same part of a file in both branches, and Git doesn't know which changes to keep.
Let's walk through an example to make this clearer. Suppose you and a teammate are working on the same project. You're adding a new feature in a branch called feature-login
, and your teammate is fixing a bug in a branch called bugfix-header
. You both make changes to the app.js
file, but in different parts of the file. When you try to merge bugfix-header
into main
, Git is fine with it. However, when you try to merge feature-login
into main
, Git finds a conflict because both changes affect the same lines in app.js
.
When you encounter a merge conflict, Git will mark the conflicting sections within the affected files. These conflict markers usually look like this:
<<<<<<< HEAD
console.log('This is the main branch');
=======
console.log('This is the feature-login branch');
>>>>>>> feature-login
The code between <<<<<<< HEAD
and =======
is from the current branch (the branch you are merging into), and the code between =======
and >>>>>>> feature-login
is from the branch you are trying to merge.
To resolve this, you need to manually edit the file to keep the changes you want and remove the conflict markers. For example, you might decide to keep both log statements for debugging:
console.log('This is the main branch');
console.log('This is the feature-login branch');
Once you've resolved the conflicts, you need to stage the changes and commit them. Here are the commands to use:
git add app.js
git commit -m "Resolved merge conflict in app.js"
In some scenarios, resolving conflicts can be more complex and require more than just combining code. For example, if two different logic implementations were added, you might need to discuss with your teammate the best way to integrate both changes. Effective communication and collaboration are crucial here.
Regularly merging branches and using feature flags can minimize conflicts. For instance, if your team frequently merges changes into the main
branch, conflicts are less likely to build up. Feature flags allow you to merge incomplete features into the main branch without affecting the live code, making it easier to integrate changes continuously.
Another helpful command is git mergetool
, which opens a graphical interface to help resolve conflicts. It's particularly useful for complex conflicts:
git mergetool
When you run this command, Git will open a merge tool to help you resolve conflicts. Common merge tools include kdiff3
, Meld
, and Beyond Compare
. You can configure Git to use your preferred merge tool by setting the merge.tool
configuration:
git config --global merge.tool meld
Replace meld
with your preferred merge tool. When a conflict occurs, running git mergetool
will open the tool, allowing you to visually compare the conflicting changes and choose the ones you want to keep.
Using VS Code to Resolve Merge Conflicts
VS Code is a popular code editor that simplifies resolving merge conflicts. When you open a file with conflicts, VS Code highlights the conflicting sections and provides options to accept the changes from either branch or both. Here’s how to use it:
Open the repository in VS Code: Navigate to your repository and open it in VS Code:
code .
Identify Conflicting Files: VS Code will highlight files with conflicts in the Explorer panel. Click on a conflicted file to open it.
Resolve the Conflict: VS Code displays conflict markers and provides options to resolve them. You can accept changes from the current branch (HEAD), incoming changes, or both. Use the buttons provided by VS Code to choose the desired changes.
Save and Commit: After resolving the conflicts, save the file and commit the changes:
git add app.js git commit -m "Resolved merge conflict in app.js using VS Code"
It's important to test the merged code thoroughly to ensure everything works as expected. Merge conflicts can sometimes introduce subtle bugs that only surface under specific conditions. Running your project's test suite and manually testing critical paths can help catch these issues early.
Remember, merge conflicts are a natural part of collaborative development. By staying calm, communicating effectively with your team, and following best practices, you can handle them smoothly and keep your project moving forward.
For more detailed guidance, refer to the official Git documentation on merge conflicts and VS Code's guide on resolving merge conflicts.
Conclusion and Teaser for Part 2
Navigating GitHub’s complexities, from authentication and branch management to handling merge conflicts, is crucial for efficient and collaborative development. Here are the key takeaways from Part 1:
Authentication: Master SSH, GitHub CLI, and HTTPS for secure and efficient access.
Branch Management: Utilize effective branching strategies to manage code changes without disrupting the main project.
Merge Conflicts: Approach merge conflicts calmly, use tools like VS Code and
git mergetool
, and communicate with your team for smooth conflict resolution.
As you continue your GitHub journey, remember that practice and exploration are your best allies. Every challenge is an opportunity to learn and grow as a developer.
Stay tuned for Part 2, where we will dive into "Managing Commit History" as our next challenge. Get ready to refine your "commit" practices and keep your project history clean and meaningful. Until then, happy coding!
By following these guidelines, you'll transform GitHub frustrations into a journey of mastery and collaboration. Keep practicing, exploring, and pushing the boundaries of your coding skills.
Subscribe to my newsletter
Read articles from Ayodeji Hamed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
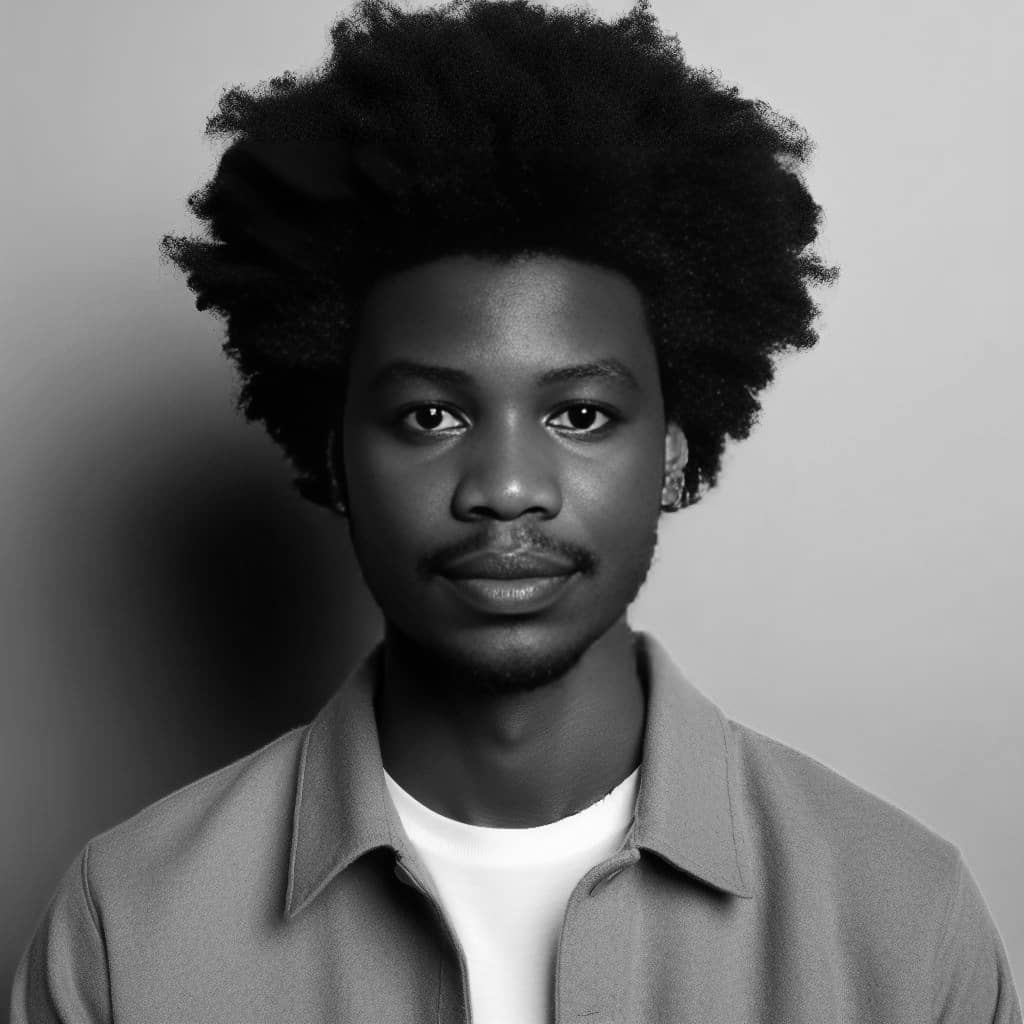
Ayodeji Hamed
Ayodeji Hamed
🚀 Welcome to my tech playground on Hashnode! I'm Hamed Ayodeji, a DevOps/Cloud Engineer with a passion for all things innovative and cutting-edge in the world of technology. Join me as I explore the latest trends, share insights, and embark on exciting coding adventures. Let's connect, learn, and grow together in this ever-evolving digital landscape! #DevOps #CloudEngineering #Innovation