Optimizing E-Commerce Communication: Implementing Email Notifications with AWS SES
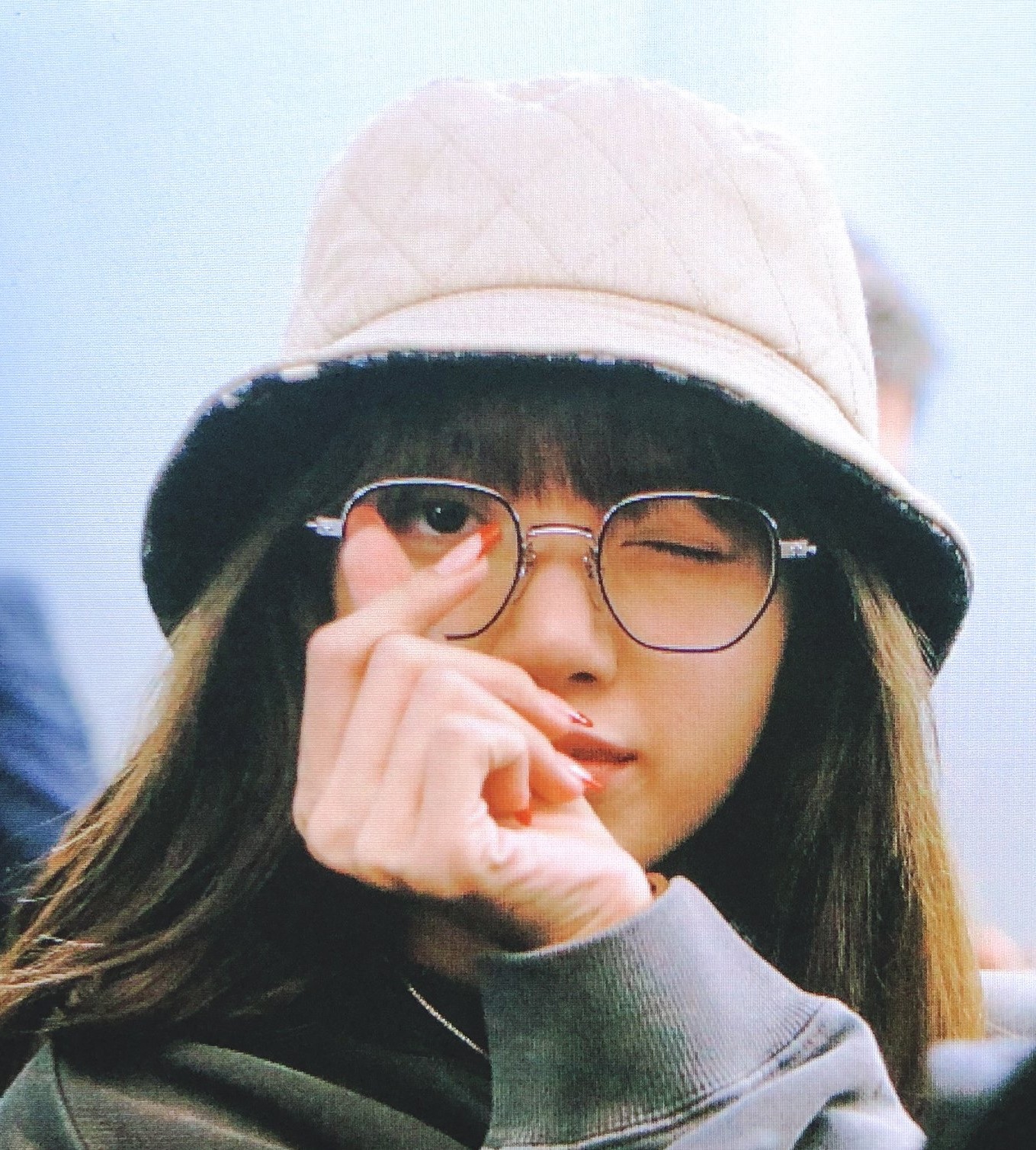
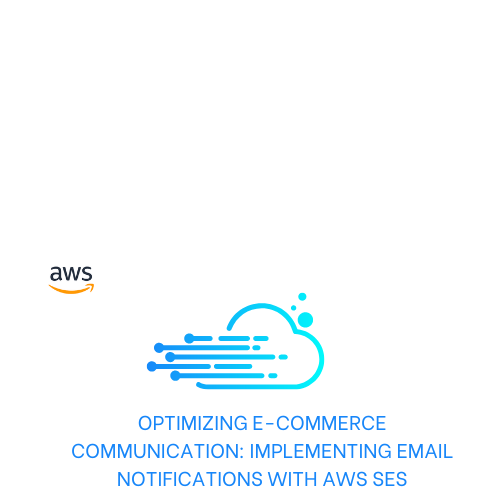
Introduction:
Welcome to the next installment of my series on architecting a cutting-edge e-commerce platform. In this post, I'll delve into the role of AWS Simple Email Service (SES) in my project. I'll walk you through on how SES helps me efficiently send invoices to users, ensuring timely and reliable communication. I'll also provide a step-by-step guide to setting up and configuring SES, share best practices, and discuss the challenges I faced along the way.
Email Notifications: A Key Feature
A crucial feature of our e-commerce platform is the ability to send order invoices to users. This functionality not only enhances the user experience but also builds trust by providing clear and timely transaction records. When a user completes a purchase, an invoice detailing the items purchased, date and time of the transaction, and user profile details is generated and sent to their registered email address.
Leveraging AWS SES for Invoices
AWS SES is a cost-effective, flexible, and scalable email service that enables us to send transactional emails like invoices. Here’s how we use it in our project:
- Generating Invoices: When a user checks out, our system collects order details and user information. This data is processed in the background to create an invoice.
Sending Emails: The generated invoice is then sent to the user's registered email address using AWS SES, ensuring that users receive timely confirmation of their purchase.
As part of AWS Free Tier, SES offers a flexible free tier which enables you to try the SES email features you need, free of charge. Free tier customers receive up to 3,000 message charges free each month for the first 12 months after you start using SES.
Setting Up and Configuring AWS SES
To integrate AWS SES into our e-commerce platform, follow these steps:
Verify Your Domain and Email Addresses: Start by verifying the email addresses or domains from which you plan to send emails. This is crucial for ensuring that your emails are delivered successfully.
Configure IAM Roles: Set up IAM roles and policies to grant your application the necessary permissions to send emails via SES.
Generalized Explanations and Concepts
AWS Simple Email Service (SES) is a cloud-based email sending service provided by Amazon Web Services (AWS). It allows you to send and receive emails from your applications using SMTP or API calls. SES provides a scalable and cost-effective solution for sending transactional emails, marketing campaigns, and other types of email communications.
To interact with AWS SES from a web application, you can use the AWS SDK for your preferred programming language. In this case, the application is using the AWS SDK for JavaScript (aws-sdk).
AWS SDK Configuration: Before you can interact with AWS services, you need to configure the AWS SDK with your AWS credentials and other settings. This typically involves providing your Access Key ID, Secret Access Key, and the desired AWS region.
AWS.config.update({ accessKeyId: 'YOUR_ACCESS_KEY_ID', secretAccessKey: 'YOUR_SECRET_ACCESS_KEY', region: 'us-east-1', });
Sending Emails with AWS SES: To send an email using AWS SES, you typically create an instance of the AWS.SES service and use the
sendEmail
method, which takes an object with parameters such as the source (sender) email address, recipient email addresses, subject, and email body.const ses = new AWS.SES(); const params = { Source: 'sender@example.com', Destination: { ToAddresses: ['recipient@example.com'], }, Message: { Subject: { Data: 'Email Subject', }, Body: { Text: { Data: 'Email Body', }, }, }, }; ses.sendEmail(params, (err, data) => { if (err) { console.error('Error sending email:', err); } else { console.log('Email sent:', data); } });
Project-Specific Implementation
In our project, the code related to sending an invoice email using AWS SES is located in the sendInvoiceEmail
function, which is imported in the Checkout.js
file:
Here is the JavaScript file named sendInvoiceEmail.js
.
import AWS from 'aws-sdk';
import ReactDOMServer from 'react-dom/server';
import Invoice from '../services/Invoice';
// Configure AWS with your credentials and region
AWS.config.update({
accessKeyId: 'YOUR_ACCESS_KEY_ID',
secretAccessKey: 'YOUR_SECRET_ACCESS_KEY',
region: 'us-east-1',
});
const ses = new AWS.SES();
This section involves importing aws-sdk
for AWS interactions, react-dom/server
for server-side React rendering, and an Invoice
component from ../services/Invoice
. It configures the AWS SDK with credentials and a region, then creates an AWS.SES
instance for email sending via AWS SES.
const sendInvoiceEmail = async (to, orderDetails) => {
try {
// Calculate the total outside the component
const total = orderDetails.items.reduce(
(acc, item) => acc + item.price * item.quantity,
0
).toFixed(2);
// Generate the invoice HTML using ReactDOMServer.renderToString
const invoiceHTML = ReactDOMServer.renderToString(
<Invoice orderDetails={orderDetails} total={total} isEmailRendering={true} />
);
// Prepare the email parameters
const params = {
Destination: {
ToAddresses: [to],
},
Message: {
Body: {
Html: {
Charset: 'UTF-8',
Data: `
<!DOCTYPE html>
<html>
<head>
<title>Invoice</title>
</head>
<body>
${invoiceHTML}
</body>
</html>
`,
},
},
Subject: {
Charset: 'UTF-8',
Data: `Invoice for Order ${orderDetails.orderId}`,
},
},
Source: 'Your verified email address',
};
// Send the email using AWS SES
await ses.sendEmail(params).promise();
console.log('Invoice email sent successfully');
} catch (error) {
console.error('Error sending invoice email:', error);
}
};
export default sendInvoiceEmail;
The sendInvoiceEmail
function computes the total order cost, renders an Invoice component server-side, prepares email details including recipient, HTML body, and subject, sends the email via AWS SES with error management, and logs outcomes. This method employs react-dom/server
for dynamic invoice email generation, enhancing visual appeal and personalization.
Now, we'll incorporate the sendInvoiceEmail
function into our checkout.js
file.
Imports :
import AWS from 'aws-sdk'; // For interacting with AWS SES import sendInvoiceEmail from './sendInvoiceEmail'; // Imports the sendInvoiceEmail function
AWS
: Used to interact with AWS services, including SES.sendInvoiceEmail
: Imports the function responsible for constructing and sending invoice emails via SES.
handleSubmit
Function :const handleSubmit = async (e) => { e.preventDefault(); // ... (form validation) // Send invoice email using sendInvoiceEmail function await sendInvoiceEmail(userData.email, orderDetails); // ... (other actions after sending invoice email) };
Within the
handleSubmit
function, after form validation:The
sendInvoiceEmail
function is called with two arguments:userData.email
: The user's email address obtained from the checkout form.orderDetails
: An object containing the order details (likely defined elsewhere in the code).
This function likely constructs the invoice email content (using the template from the invoice file created) and sends it via SES.
Upon form submission, handleSubmit
triggers form validation; post-validation, sendInvoiceEmail
constructs and sends an invoice email via AWS SES, allowing subsequent actions like displaying a confirmation message & clearing the cart.
Best Practices for Email Communication :
Optimize Email Content: Ensure that your emails are well-formatted and provide all necessary information clearly.
Monitor Deliverability: Use SES metrics to monitor the deliverability of your emails and address any issues promptly.
Handle Bounces and Complaints: Configure bounce and complaint notifications to maintain your sender reputation and address any issues proactively.
Challenges and Solutions :
During the configuration of AWS SES, our initial challenge was integrating the SDK, leading to numerous errors. We started with SMTP for setup, but later switched to the SDK for email configuration. Overcoming these hurdles required leveraging AI tools and YouTube tutorials, which proved invaluable in troubleshooting and resolving the configuration issues, ultimately enabling us to successfully implement the invoice emailing feature.
Conclusion:
In this comprehensive exploration, I delve into the strategic use of AWS Simple Email Service (SES) to streamline the delivery of order invoices to our valued customers, thereby enriching their overall shopping journey through efficient and secure communication channels.
Concluding our series on AWS services, the strategic use of SES for secure, reliable invoicing significantly enhanced our project's customer engagement and operational efficiency, underscoring our commitment to innovation and excellence in e-commerce solutions.
Subscribe to my newsletter
Read articles from Sri Durgesh V directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
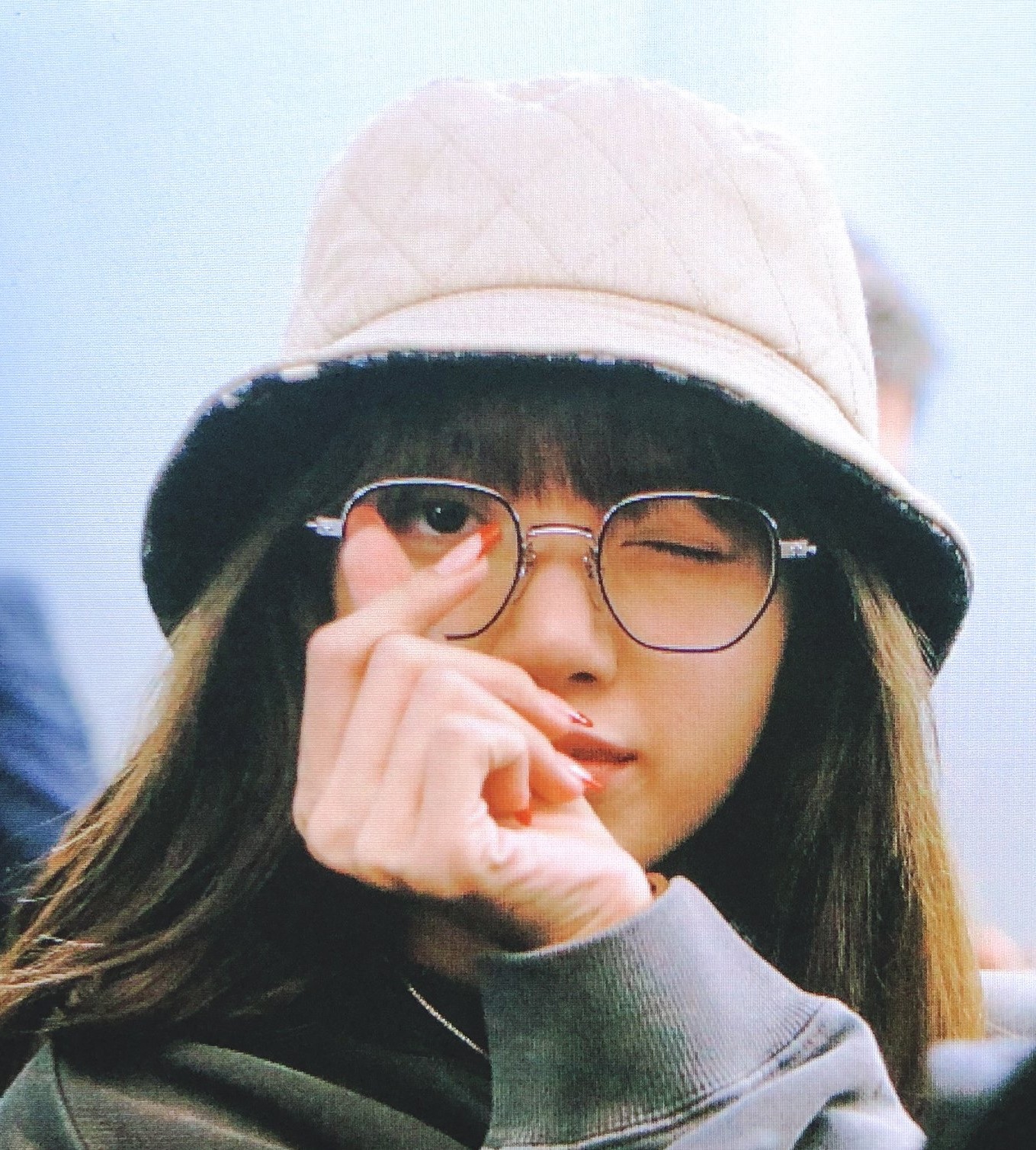
Sri Durgesh V
Sri Durgesh V
As a Cloud Enthusiast, this blog documents my journey with Amazon Web Services (AWS), sharing insights and project developments. Discover my experiences and learn useful information about different AWS cloud services.