Polyfills for Map , Filter & Reduce in Javascript
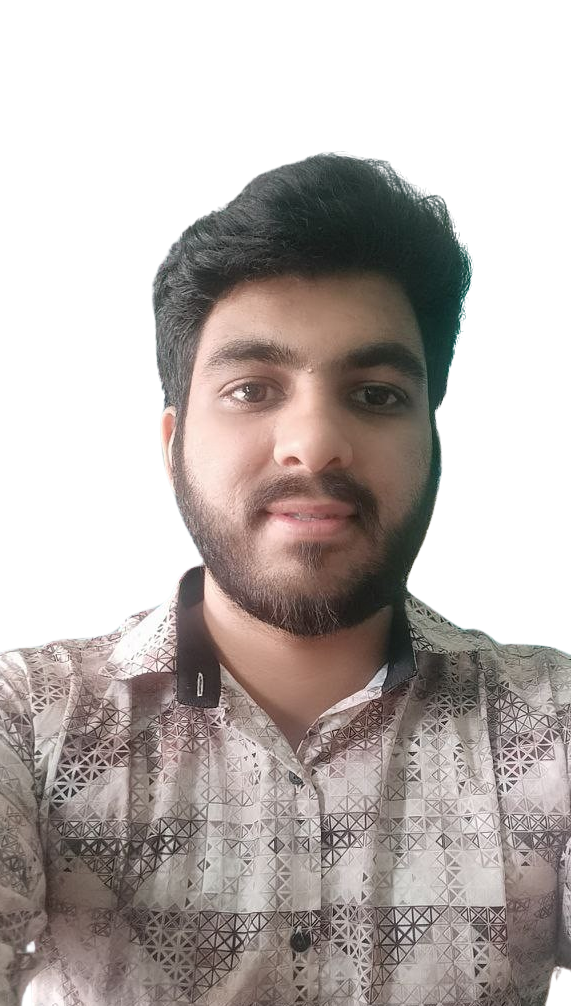
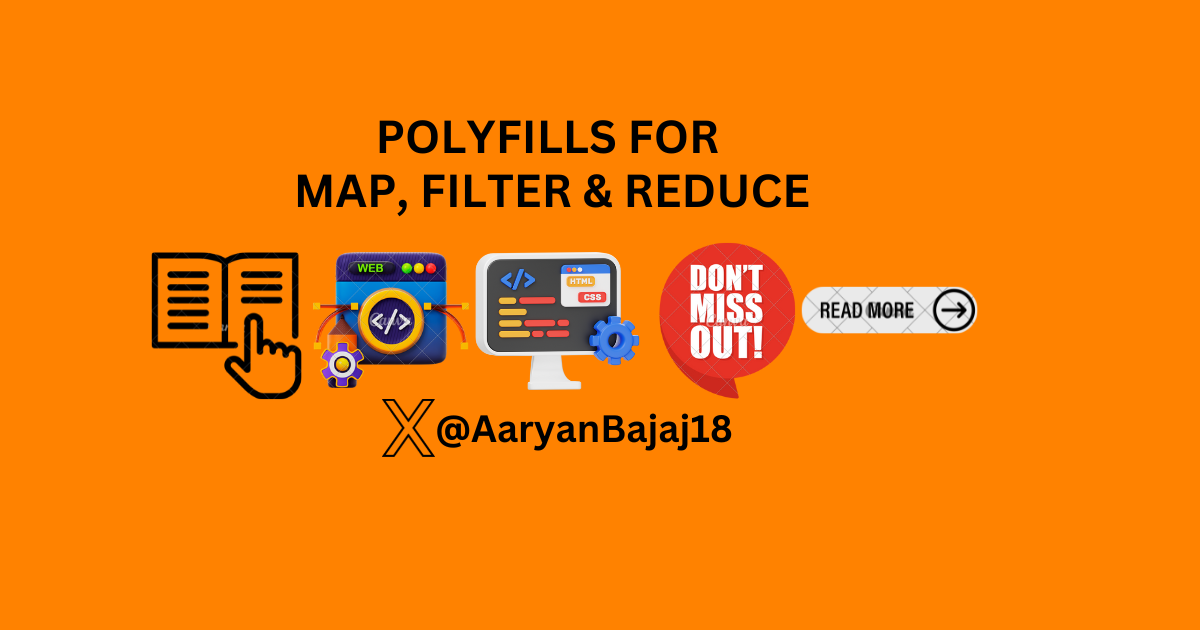
Introduction
Ever found yourself working on a project only to realise your JavaScript methods arenโt supported in older browsers? ๐ค
Let's talk about how polyfills can be your rescue squad!
In this blog, we'll explore
What polyfills are
Why they are essential
How to create polyfills for
map
,filter
, andreduce
methods in JavaScript
Map Method
The map
method creates a new array populated with the results of calling a provided function on every element in the calling array.
const numbers = [1, 2, 3, 4];
const doubled = numbers.map(num => num * 2);
console.log(doubled); // [2, 4, 6, 8]
Filter Method
The filter
method creates a new array with all elements that pass the test implemented by the provided function.
const numbers = [1, 2, 3, 4];
const even = numbers.filter(num => num % 2 === 0);
console.log(even); // [2, 4]
Reduce Method
The reduce
method executes a reducer function (that you provide) on each element of the array, resulting in a single output value.
const numbers = [1, 2, 3, 4];
const sum = numbers.reduce((acc, num) => acc + num, 0);
console.log(sum); // 10
Need For Polyfills
Browser Compatibility
Older browsers may not support these methods, causing your code to break. For instance, Internet Explorer does not support
map
,filter
, andreduce
. This is where polyfills come into play.Importance of Polyfills
Polyfills allow you to add functionality to older browsers that natively support newer JavaScript methods. This ensures your code runs consistently across all environments.
Creating Polyfills
Map Polyfill
Array.prototype.myMap = function(callback) {
let arr = [];
for (let i = 0; i < this.length; i++) {
arr.push(callback(this[i], i, this));
}
return arr;
};
Purpose: This code defines a custom
myMap
method on theArray
prototype, which mimics the behaviour of the nativeArray.prototype.map
method.Method Definition:
- Adds a method
myMap
toArray.prototype
, making it available to all array instances.
- Adds a method
Parameters:
- The
myMap
method takes one parameter:callback
, which is a function to execute on each element in the array.
- The
Variable Declaration:
let arr: any[] = [];
Initializes an empty array
arr
to store the results of applying the callback function.
Looping through the Array:
for (let i: number = 0; i < this.length; i++) { ... }
Iterates over each element of the array using a
for
loop.
Callback Execution and Result Storage:
Inside the loop:
arr.push(callback(this[i], i, this));
Executes the
callback
function on each element of the array (this[i]
), passing three arguments:The current element (
this[i]
).The index of the current element (
i
).The entire array (
this
).
The result of the callback function is pushed into the
arr
array.
Return Statement:
return arr;
Returns the new array
arr
containing the results of the callback function applied to each element of the original array.
Filter Polyfill
Array.prototype.myFilter = function(callback) {
let arr = [];
for (let i = 0; i < this.length; i++) {
if (callback(this[i], i, this)) {
arr.push(this[i]);
}
}
return arr;
};
Purpose: This code defines a custom
myFilter
method on theArray
prototype, which mimics the behaviour of the nativeArray.prototype.filter
method.Method Definition:
- Adds a method
myFilter
toArray.prototype
, making it available to all array instances.
- Adds a method
Parameters:
- The
myFilter
method takes one parameter:callback
, which is a function to execute on each element in the array.
- The
Variable Declaration:
let arr: any[] = [];
Initialises an empty array
arr
to store the elements that pass the test implemented by the callback function.
Looping through the Array:
for (let i: number = 0; i < this.length; i++) { ... }
Iterates over each element of the array using a
for
loop.
Callback Execution and Result Storage:
Inside the loop:
if (callback(this[i], i, this)) { arr.push(this[i]); }
Executes the
callback
function on each element of the array (this[i]
), passing three arguments:The current element (
this[i]
).The index of the current element (
i
).The entire array (
this
).
If the callback function returns
true
, the current element (this[i]
) is pushed into thearr
array.
Return Statement:
return arr;
Returns the new array
arr
containing the elements that passed the test implemented by the callback function.
Reduce Polyfill
Array.prototype.myReduce = function(callback, initialValue) {
let accumulator = initialValue;
if (!initialValue) {
accumulator = this[0];
for (let i = 1; i < this.length; i++) {
accumulator = callback(accumulator, this[i], i, this);
}
} else {
for (let i = 0; i < this.length; i++) {
accumulator = callback(accumulator, this[i], i, this);
}
}
return accumulator;
};
Purpose: This code defines a custom
myReduce
method on theArray
prototype, which mimics the behaviour of the nativeArray.prototype.reduce
method.Method Definition:
- Adds a method
myReduce
toArray.prototype
, making it available to all array instances.
- Adds a method
Parameters:
The
myReduce
method takes two parameters:callback
: a function to execute on each element in the array.initialValue
: an initial value to use as the first argument to the first call of the callback.
Variable Declaration:
let accumulator = initialValue;
Initialises the
accumulator
variable to the value ofinitialValue
.
Conditional Check for Initial Value:
if (!initialValue) { ... }
Checks if
initialValue
is not provided.
Handling No Initial Value:
accumulator = this[0];
Sets
accumulator
to the first element of the array.Starts the loop from the second element (
i = 1
).for (let i: number = 1; i < this.length; i++) { ... }
Iterates over each element of the array starting from the second element.
accumulator = callback(accumulator, this[i], i, this);
Executes the
callback
function with theaccumulator
, current element, index, and the entire array as arguments. Updates theaccumulator
with the result.
Handling Provided Initial Value:
else { ... }
If
initialValue
is provided, starts the loop from the first element (i = 0
).for (let i = 0; i < this.length; i++) { ... }
Iterates over each element of the array starting from the first element.
accumulator = callback(accumulator, this[i], i, this);
Executes the
callback
function with theaccumulator
, current element, index, and the entire array as arguments. Updates theaccumulator
with the result.
Return Statement:
return accumulator;
Returns the final value of the
accumulator
.
Thank You Everyone For The Read ....
Happy Coding! ๐ค๐ป
๐ Hello, I'm Aaryan Bajaj .
๐ฅฐ If you liked this article, consider sharing it.
Subscribe to my newsletter
Read articles from AARYAN BAJAJ directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
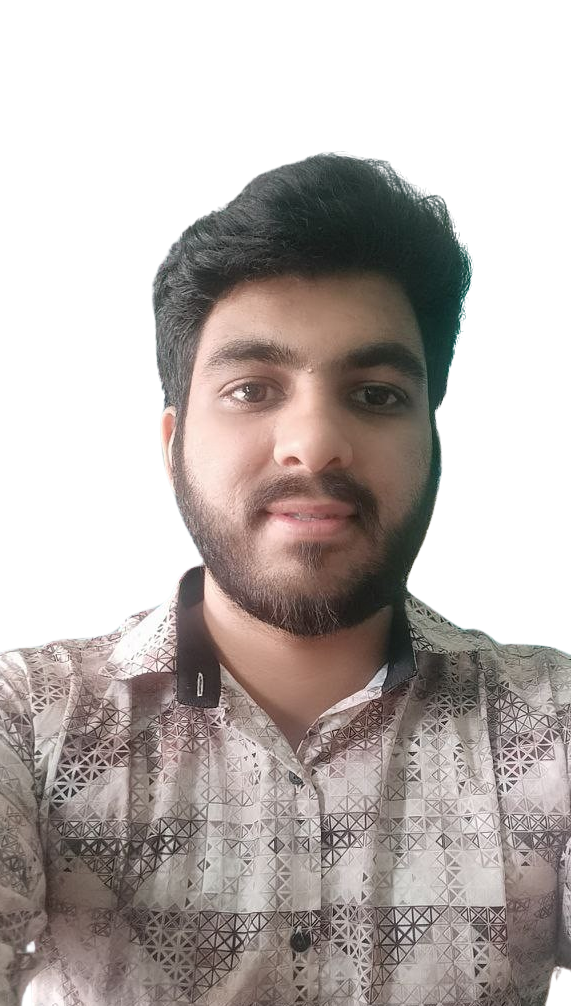
AARYAN BAJAJ
AARYAN BAJAJ
Hi Everyone ๐๐ป , I'm Aaryan Bajaj , a Full Stack Web Developer from India(๐ฎ๐ณ). I share my knowledge through engaging technical blogs on Hashnode, covering web development.