A Step-by-Step Guide to AWS Key Management Service (KMS) with DynamoDB Global Tables

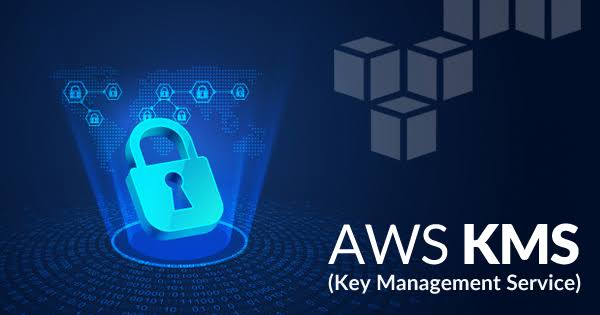
In today's digital landscape, data security is paramount. AWS Key Management Service (KMS) offers a scalable solution for encrypting your data. This blog post will walk you through a practical application of AWS KMS by using it in conjunction with Amazon DynamoDB Global Tables, highlighting how to securely manage and replicate data across multiple regions.
What is AWS KMS?
AWS Key Management Service (KMS) is a managed service that makes it easy to create and control the cryptographic keys used to encrypt your data. KMS integrates with other AWS services to simplify the encryption and decryption process, ensuring your data remains secure.
Understanding the Workflow
The image provided illustrates a common use case where a client application encrypts a record using KMS before storing it in DynamoDB. This data is then replicated to another region using DynamoDB Global Tables, where it can be decrypted and accessed by another client application. Let's break down the process step by step.
Step-by-Step Explanation
Step 1: Encrypt Record with Primary MRK
Region:
us-east-1
Action: The client application uses AWS KMS to encrypt a record with the primary Multi-Region Key (MRK).
Details:
KMS Primary MRK: This is the primary key used for encryption in the
us-east-1
region.Client Application: Initiates the encryption request.
import boto3
# Create a KMS client
kms_client = boto3.client('kms', region_name='us-east-1')
# Encrypt data
plaintext = b'Hello, world!'
response = kms_client.encrypt(
KeyId='alias/your-key-alias',
Plaintext=plaintext
)
ciphertext_blob = response['CiphertextBlob']
Step 2: Put Encrypted Record in DynamoDB
Region:
us-east-1
Action: The encrypted record is stored in a DynamoDB table.
Details:
DynamoDB: A fully managed NoSQL database service that supports key-value and document data structures.
Client Application: Writes the encrypted data to DynamoDB.
import boto3
# Create a DynamoDB client
dynamodb = boto3.resource('dynamodb', region_name='us-east-1')
table = dynamodb.Table('YourTableName')
# Store encrypted data
table.put_item(
Item={
'id': '123',
'encrypted_data': ciphertext_blob
}
)
Step 3: Global Table Replication
Action: The encrypted record is replicated to another region (
us-west-2
) using DynamoDB Global Tables.Details:
- Global Tables: Enable automatic replication of DynamoDB tables across multiple AWS regions.
Step 4: Get Encrypted Record from DynamoDB
Region:
us-west-2
Action: The client application retrieves the encrypted record from the replicated DynamoDB table.
Details:
DynamoDB: The service in the
us-west-2
region from which the encrypted data is fetched.Client Application: Reads the encrypted data from DynamoDB.
# Create a DynamoDB client in us-west-2
dynamodb = boto3.resource('dynamodb', region_name='us-west-2')
table = dynamodb.Table('YourTableName')
# Retrieve encrypted data
response = table.get_item(
Key={
'id': '123'
}
)
encrypted_data = response['Item']['encrypted_data']
Step 5: Decrypt Record with Replica MRK
Region:
us-west-2
Action: The client application uses AWS KMS to decrypt the record with the replica MRK.
Details:
KMS Replica MRK: This is the replica key used for decryption in the
us-west-2
region.Client Application: Initiates the decryption request.
# Create a KMS client in us-west-2
kms_client = boto3.client('kms', region_name='us-west-2')
# Decrypt data
response = kms_client.decrypt(
CiphertextBlob=encrypted_data,
EncryptionContext={
'aws:replica': 'us-west-2'
}
)
plaintext = response['Plaintext']
print(plaintext.decode('utf-8'))
Conclusion
AWS KMS, when used with DynamoDB Global Tables, offers a robust solution for secure and scalable data management across multiple regions. This setup ensures that your data remains encrypted during transit and at rest, providing a high level of security and reliability.
By following this step-by-step guide, you can implement a similar architecture in your AWS environment, leveraging the power of KMS and DynamoDB to protect and manage your data effectively.
Subscribe to my newsletter
Read articles from ANSAR SHAIK directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

ANSAR SHAIK
ANSAR SHAIK
AWS DevOps Engineer