Tutorial : How to get Recent Blogs using Hashnode API 2024 Updated
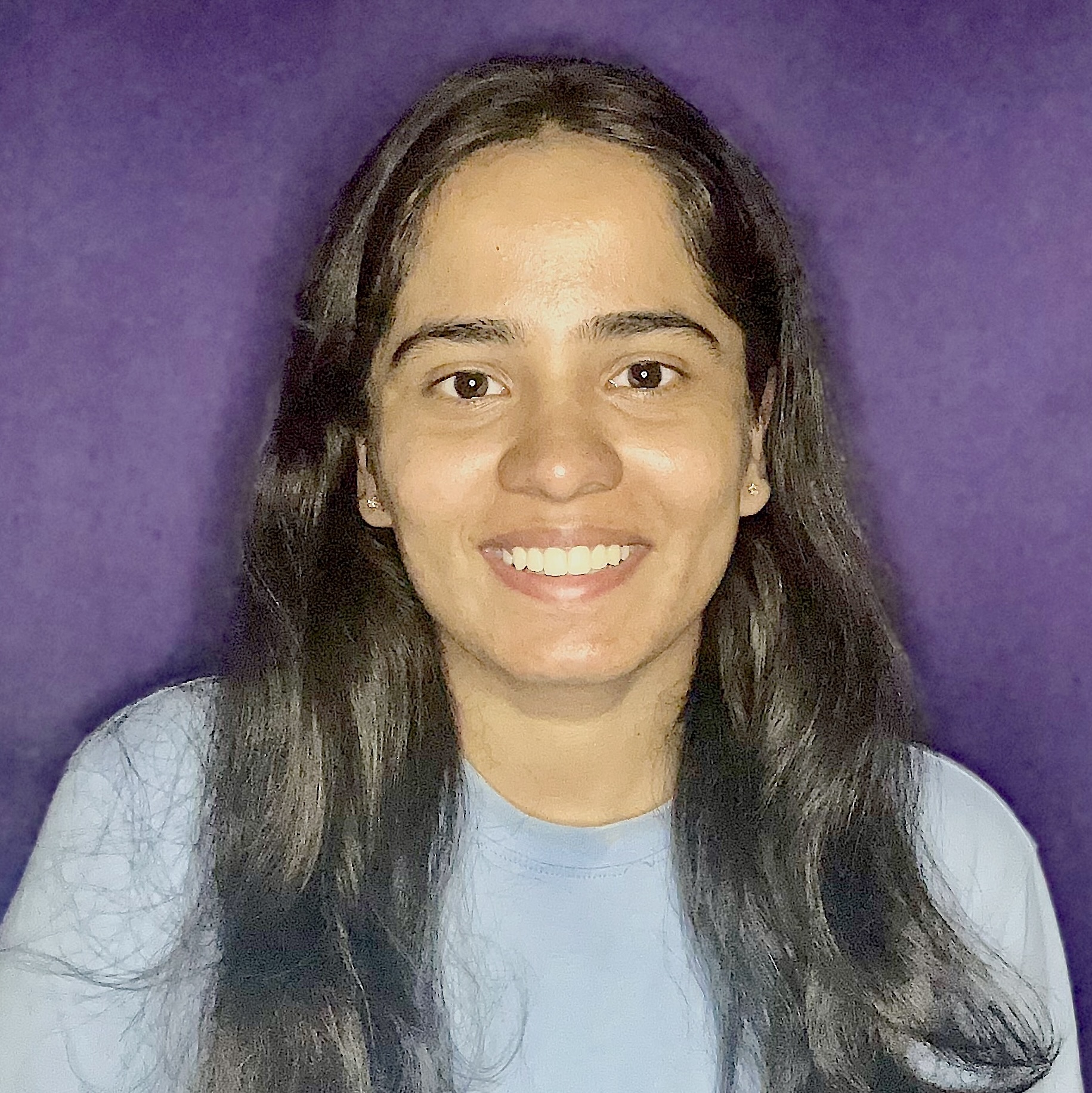
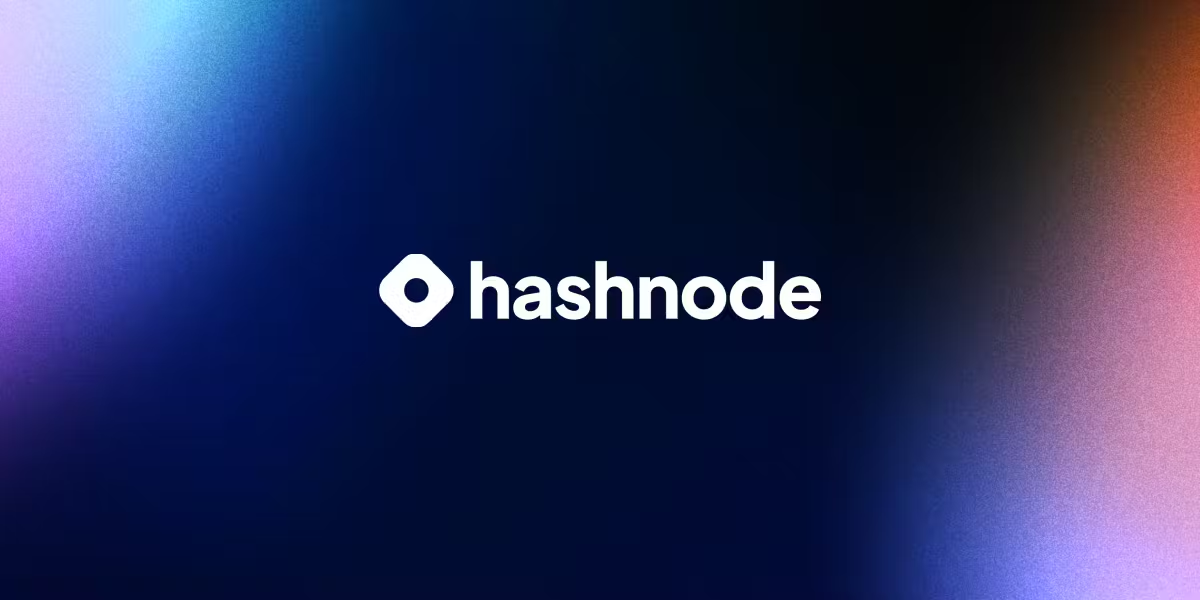
Whether you're looking to showcase your latest posts on your portfolio website or simply want to streamline your content management, this tutorial will guide you through the process with ease. In this article, I will be sharing how you can fetch all the blog posts of a user using Hashnode API version 2.0.
What you'll need:
Knowledge of HTML,CSS & JS
Basic Understanding of GraphQL
In this tutorial, I will be using my own user and will be adding 3 Most Recent Posts to my portfolio website. You can check it out here.
Step 1: Fetching data using GraphQL queries
Firstly, we will start by writing some GraphQL queries to fetch all data we need. Hashnode provides a GraphQL Playground where you can run those queries before adding to your code.
Playground link ๐๐ป https://gql.hashnode.com
To fetch these posts, We can use either a hostname or host id. We will be using hostname in this but you can do the same with host id as well.
Hostname - It will be either "username.hashnode.dev" or "blog.username.dev"
Host ID - It can be fetched by using hostname in below query.
query Publication($host: String = "tanujabhatnagar.hashnode.dev") {
publication(host: $host) {
id
}
}
You can also find your hostId by navigating to your dashboard url
You can provide your own value to the variable $host using String = "yourvalue".
For adding cards to my website, I will be fetching below columns :
Title
Brief
Slug
URL
query Publication(
$id: ObjectId="631db6be3e8d6f3497ad5d20"
) {
publication(
id: $id
) {
posts(first:3) {
edges {
node {
title,
brief,
slug,
url
}
}
}
}
}
Step 2: Creating JS and adding query.
After that, you need to create a .js file and add the query to a variable.
const GET_USER_ARTICLES = `query Publication(
$id: ObjectId="631db6be3e8d6f3497ad5d20"
) {
publication(
id: $id
) {
posts(first:5) {
edges {
node {
title,
brief,
slug,
url
}
}
}
}
}`;
Then, we will create a function that will make a call to Hashnode's GraphQL Playground and return json data.
async function gql(query) {
const data = await fetch('https://gql.hashnode.com/', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
query
})
});
return data.json();
}
Step 3: Creating HTML
Then, we will create an HTML body inside which we will create a div with class "app
". We also need to add tag with id "articles
".
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>App Page</title>
</head>
<body>
<div class="app">
</div>
<script type="text/javascript" id="articles" src="app.js"></script>
<script>
</body>
</html>
Step 4 : Populating result in HTML using JS
Now, we just need to fetch the result and create cards for our website using below code.
gql(GET_USER_ARTICLES, { page: 0 }).then(result => {
const articles = result.data.publication.posts.edges;
let container = document.createElement('div');
container.setAttribute("id", "card");
articles.forEach(article => {
let title = document.createElement('h3');
title.innerText = article.node.title;
let brief = document.createElement('p');
brief.innerText = article.node.brief;
let link = document.createElement('a');
link.innerText = 'Read more...';
link.href = `https://tanujabhatnagar.hashnode.dev/${article.node.slug}`;
container.appendChild(title);
container.appendChild(brief);
container.appendChild(link);
});
document.querySelector('.app').appendChild(container);
});
And Voila ๐
If you find this tutorial helpful, don't forget to hit the โค๏ธ button.
Check out my website here and feel free to connect.
Happy Coding! ๐ฉ๐ปโ๐ป
Subscribe to my newsletter
Read articles from Tanuja Bhatnagar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
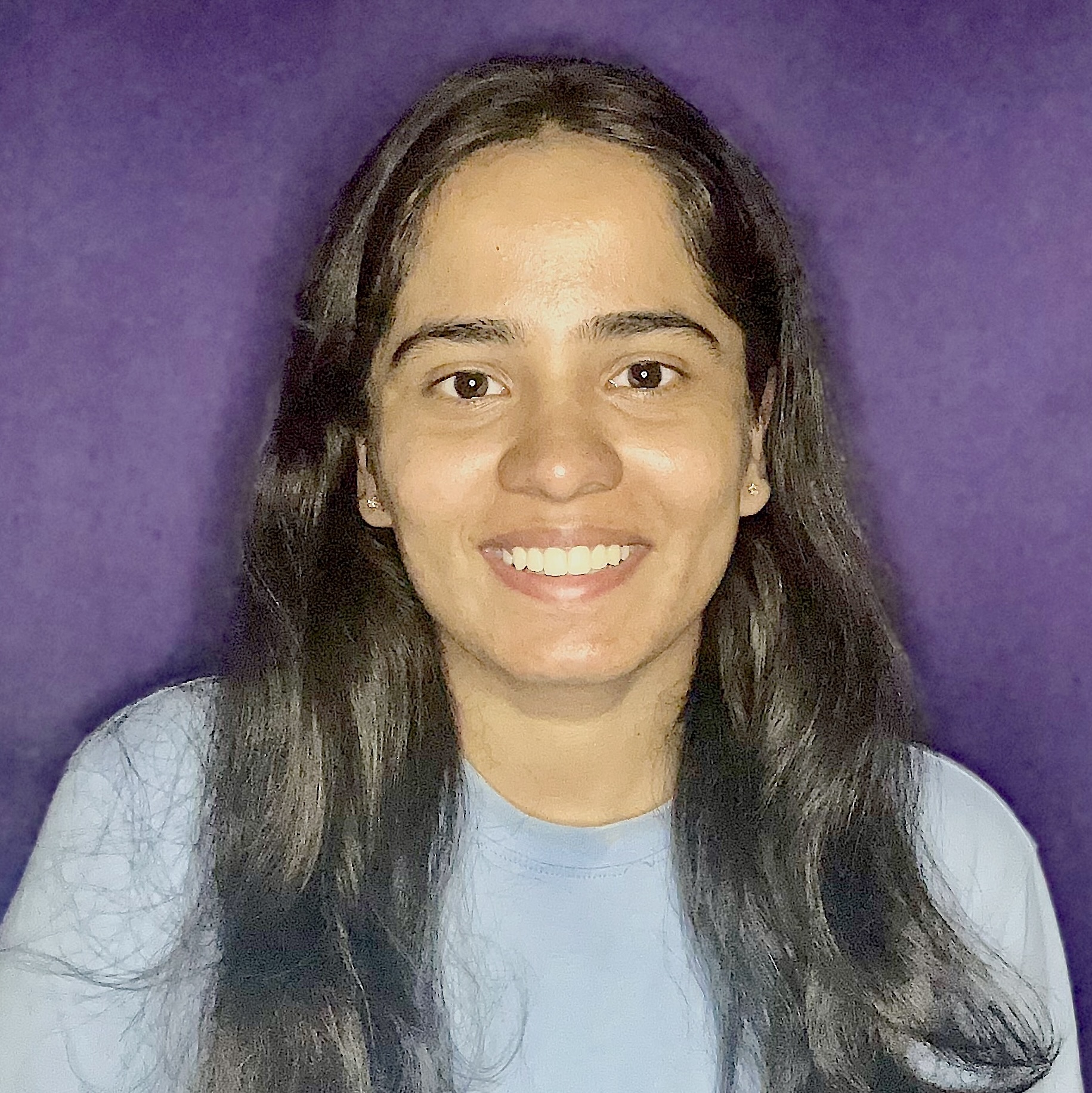
Tanuja Bhatnagar
Tanuja Bhatnagar
Programmer and a tech enthusiast