(lt.39)Mastering Regular Expressions and Efficient Use of Spread & Rest Operators in JavaScript

4 min read
Table of contents
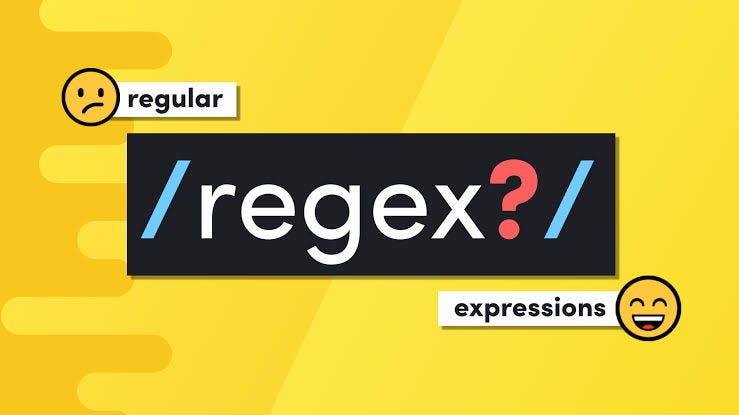
A regular expression (regex) in JavaScript is a pattern used to match character combinations in strings. They consist of a sequence of characters and special metacharacters that define the criteria for the search.
// let pattern = "hello"
// //converted to regular expression
// // let regx = new RegExp(pattern) // this conversion is optional
// let flag = "gi" // g resembles global and i resembles index
// // check
// let regx1 = new RegExp(pattern,flag)
// // const check ="hello1 himansh2u"
// const check ="helilo abhhello himansh2u"
// const result = regx1.test(check)
// // const result = pattern.test(check)
// // // .test will not work if you have not converted both into regexp
// console.log(result)
// console.log(regx1)
/*+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*/
// // method 2
// let regx2 = /himanshu/gi
// const check ="hello1 himansh2u"
// // const check ="hello874hi himanshu_89585649hj"
// // const check ="hel4lohello 67himanshu_89585649hj"
// const result1 = regx2.test(check)
// // // //ye test method tabhi kaam karega jab regular expression ban chuka hoga aur result boolean value m hi dega
// console.log(result1)
/*++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ */
// let regx2 = /himanshu/gi
// const check ="hello himanshu how are u say hel6lo hi 1ii_himanshu again HIMANSHui90i means hello hi means hell9o"
// const otherresult = check.match(regx2)
// console.log(otherresult)
// const replaceresult = check.replace(regx2,'h-elo')
// console.log(replaceresult)
/*+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*/
// // // checking urls
const weburl ="https://anon30ymo3030us.30@gmail.com"
const urlresult =weburl.replace(/30/,"-")
console.log(urlresult)
const urlresult1 =weburl.replace(/\d\d\d\d/,"-")
console.log(urlresult1)
Spread and rest operator
The spread operator unpacks an iterable (like an array or string) into individual elements.
It's commonly used in:
Combining Arrays:
const numbers1 = [1, 2, 3]; const numbers2 = [4, 5, 6]; const combinedNumbers = [...numbers1, ...numbers2]
- Passing Arguments to Functions:
function sum(x, y, z) {
return x + y + z;
}
const n = [11, 2, 3];
const result = sum(...n);
console.log(result)
Copying Arrays:
const originalArray = [1, 2, 3]; const copiedArray = [...originalArray];
Rest operator: The rest parameter gathers remaining elements into an array within function parameters or array destructuring.It's used for:
1. Variable-Length Function Arguments:
2. Array Destructuring:
const [first, ...remaining] = [1,8 ,4,5,2, 3, 4];
console.log(first)
console.log(remaining)
Coding implementation:
// let a = [1, 2, 3,4]
// let b = [5,6,7,8]
// // let c = a.concat(b)
// // console.log(c)
// // let d = [ a , b]
// // console.log(d)
// // // //spread and rest operator
// // the below code is both spred and rest if depends on the use case
// const e = [...a , ...b]
// // here this is spread
// console.log(e)
/**+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ */
// converting objects to array//
// TYPE 1: CONVERTING ARRAY TO ARGUMENTS//
// function hello(){
// let a = Array.from(arguments)
// let b = a.map(e=>e)
// console.log(b)
// }
// hello(1,2,3)
// TYPE 2: DIRECTLY CONVERTING INTO ARRAY
// function hii(...a)
// {
// let b = a.map(e=>e)
// console.log(b);
// }
// hii(2,3,6,4)
/*--------------------*/
// rest operator : when we have different values in arguments
// and we have to combine them then we can use rest operator
// spread operator : when we have to combine two array then we have
// to either use concat otherwise the output is array inside array
// so to overcome it and for seperation we have to use spread operator
/**/
// more exapmles of sperad operator
// const name = ["g" , 'gg']
// const name2 = ["h" , ...name,'hh']
// console.log(name2)
// const name3 = "hello howare you"
// console.log([...name3])
// console.log(...name3)
// example of rest operator
// function testrest (...a){
// return a
// }
// console.log(testrest(1,2,3))
/*
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*/
// let set1= new Set() // declaration of set
// console.log(set1.size)
// let array1 =[1,2,3,3,,4,5,3,2]
// // set allows only unique values
// // //TO CREATE A SET
// const newset = new Set(array1)
// console.log(newset)
// console.log(newset.size)
// // //TO ADD A NEW NUMBER
// newset.add(9)
// console.log(newset)
// // // TO CHECK WHETEHER THE LEMENET IS PRESENT OR NOT
// console.log(newset.has(9))
// // //TO CLEAR THE ELEMENTS
// newset.clear()
// console.log(newset)
// //set difference
function setdiff(set1, set2)
{
if (!(set1 instanceof Set) || !(set2 instanceof Set)) {
throw new Error('Both setA and setB must be Sets+++++.');
}
return new Set ( [...set1].filter(element => !set2.has(element)))
}
// const set11 = new Set([1, 2, 3]);
// const set2 = new Set([2, 3, 4]);
// const diffSet = setdiff(set11, set2);
// console.log(diffSet)
// const set1 = new Set([1, 2, 3]);
// const array2 = [2, 3, 4];
// const diffSet = setdiff(set1, array2);
// console.log(diffSet);
// //MAP
let map = new Map()
console.log(map.size)
let array =[
[1,"give"],
[2,"krishna"],
[3,"thor"],
[4,"haryana"]
];
// let c =array.map((arrayelement)=>map.set(arrayelement[0],arrayelement[1]))
// console.log(c)
array.map((arrayelement) => map.set(arrayelement[0], arrayelement[1]));
console.log(map);
lt.38: link:https://hashnode.com/preview/662a992439252d00cfb66c8a
0
Subscribe to my newsletter
Read articles from himanshu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
